Understanding Vue.js ( emit ): A Complete Guide

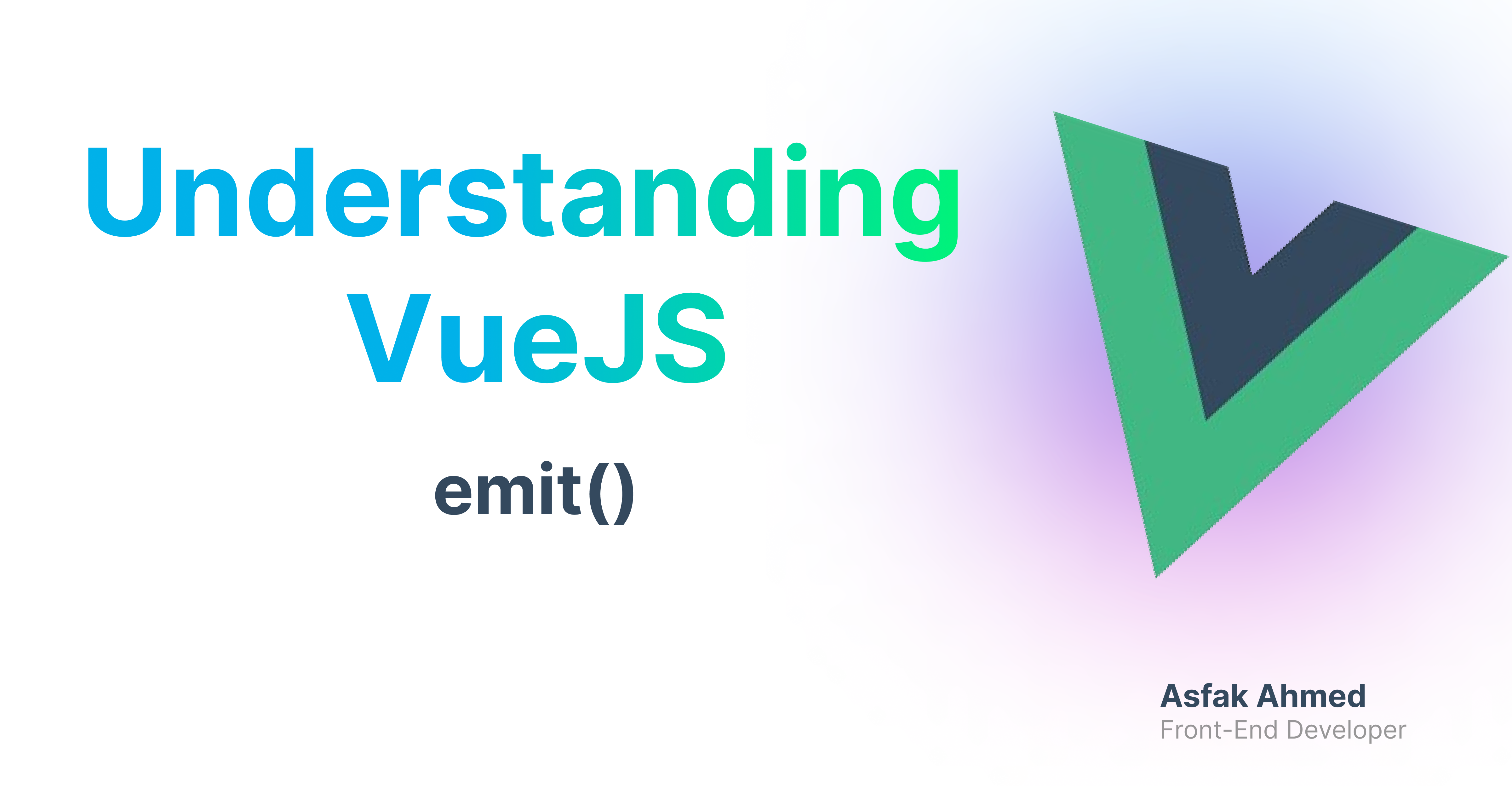
Vue.js of the core concepts in Vue is the communication between components, and emit
is the key tool for sending events from child components to parent components. In this blog post, we will explore what emit
is, why it is important, when to use it, and how it works.
What is emit
?
In Vue.js, the emit
function is used to send custom events from a child component to its parent component. Vue's component system promotes a "one-way data flow," where data flows from parent to child via props
. However, there are many cases where a child component needs to notify the parent component of an event, such as a button click, a form submission, or a value change. This is where emit
plays a vital role.
Using emit
, a child component can notify its parent of actions or changes, which can then trigger a function or update data in the parent component.
Why Use emit
?
Vue encourages the separation of concerns, where child components handle specific parts of the UI and the parent component maintains overall control. By using emit
, we ensure that:
Parent-Child Separation: Child components can remain independent, handling their own logic and state, but notify the parent when something significant happens.
Reusability: Child components can be more easily reused since they don't need to handle how their emitted events are consumed. The parent can decide what to do with the emitted event.
Maintainability: Instead of managing state across components, using
emit
allows event-driven communication, which simplifies managing complex applications.
When to Use emit
?
You should use emit
in the following scenarios:
User interactions: When a child component receives user input (e.g., a button click or form submission), and the parent needs to handle the logic.
Data changes: When a child component needs to notify the parent about changes in its internal state (like a counter or a selected item).
Asynchronous operations: When a child component performs an operation (e.g., an API call) and needs to notify the parent once it's complete.
How emit
Works
In Vue 3's Composition API, emit
is passed into the setup function. Here's the basic usage:
Define the event in the child component using
emit
inside thesetup
function.Handle the event in the parent component by listening to the custom event on the child component.
Example with Composition API
Let’s see a complete working example using Vue 3’s Composition API.
Step 1: Child Component ( Emitting an Event )
In this example, we’ll create a button component (MyButton.vue
) that emits an event called onClickButton
when the button is clicked.
<!-- MyButton.vue -->
<template>
<button @click="handleClick">Click Me</button>
</template>
<script setup>
import { defineEmits } from 'vue';
// Define the emit event
const emit = defineEmits(['onClickButton']);
// Method to emit event
const handleClick = () => {
emit('onClickButton', 'Button was clicked!');
};
</script>
Here’s what happens in this child component:
We use
defineEmits
to declare the custom eventonClickButton
.The
handleClick
function emits this event when the button is clicked, passing along a string message ('Button was clicked!'
).
Step 2: Parent Component ( Listening for the Event )
Now, we’ll create a parent component (ParentComponent.vue
) that listens to the onClickButton
event and responds when it’s emitted.
<!-- ParentComponent.vue -->
<template>
<div>
<h2>Message from Child: {{ message }}</h2>
<MyButton @onClickButton="handleButtonClick" />
</div>
</template>
<script setup>
import { ref } from 'vue';
import MyButton from './MyButton.vue';
// Define a reactive variable to store the message from the child component
const message = ref('No message yet');
// Define a method to handle the emitted event
const handleButtonClick = (msg) => {
message.value = msg;
};
</script>
Here’s what happens in this parent component:
We listen to the
onClickButton
event emitted byMyButton
using the@onClickButton
directive.When the event is triggered, the
handleButtonClick
method is called, updating themessage
ref with the message passed from the child.
Output:
When the button is clicked, the parent component’s message will be updated with the text Button was clicked!
.
Understanding Event Propagation
It’s important to note that the emit
method only works in one direction: from the child to the parent. This is in line with Vue’s philosophy of unidirectional data flow.
Props are used to pass data from the parent to the child.
emit is used to send events from the child to the parent.
By keeping the flow of data and events in one direction, Vue helps you write code that’s easier to maintain and reason about, especially in larger applications.
Common Use Cases of emit
Form Submission: When a child component (form) submits data, and the parent component needs to handle it.
Input Validation: When the child validates input and informs the parent about the success or failure.
Toggle State: When a child component needs to toggle something in the parent (e.g., opening/closing a modal).
Handling User Preferences: Child components can handle preferences, and emit changes to the parent, which can store or update the state globally.
Vue's emit
function plays a critical role in component communication. Especially in the Composition API, where code structure becomes more modular and easier to maintain, emit
makes it simple for child components to notify parent components about events or state changes. This enhances reusability, keeps components decoupled, and makes the application easier to manage.
When building complex Vue 3 applications, mastering emit
will allow you to create modular, event-driven systems where components can communicate effectively while maintaining clear separation of concerns.
Subscribe to my newsletter
Read articles from Asfak Ahmed directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Asfak Ahmed
Asfak Ahmed
I'm Asfak Ahmed, a Front-End Developer with 2+ years of experience, currently enhancing user experiences at Doplac CRM. As the founder of ZenUI Library, I focus on creating intuitive, user-centric interfaces. My passion lies in blending creativity with technology to craft impactful digital solutions.