Wrapping Server Components in Client Components: What Happens?

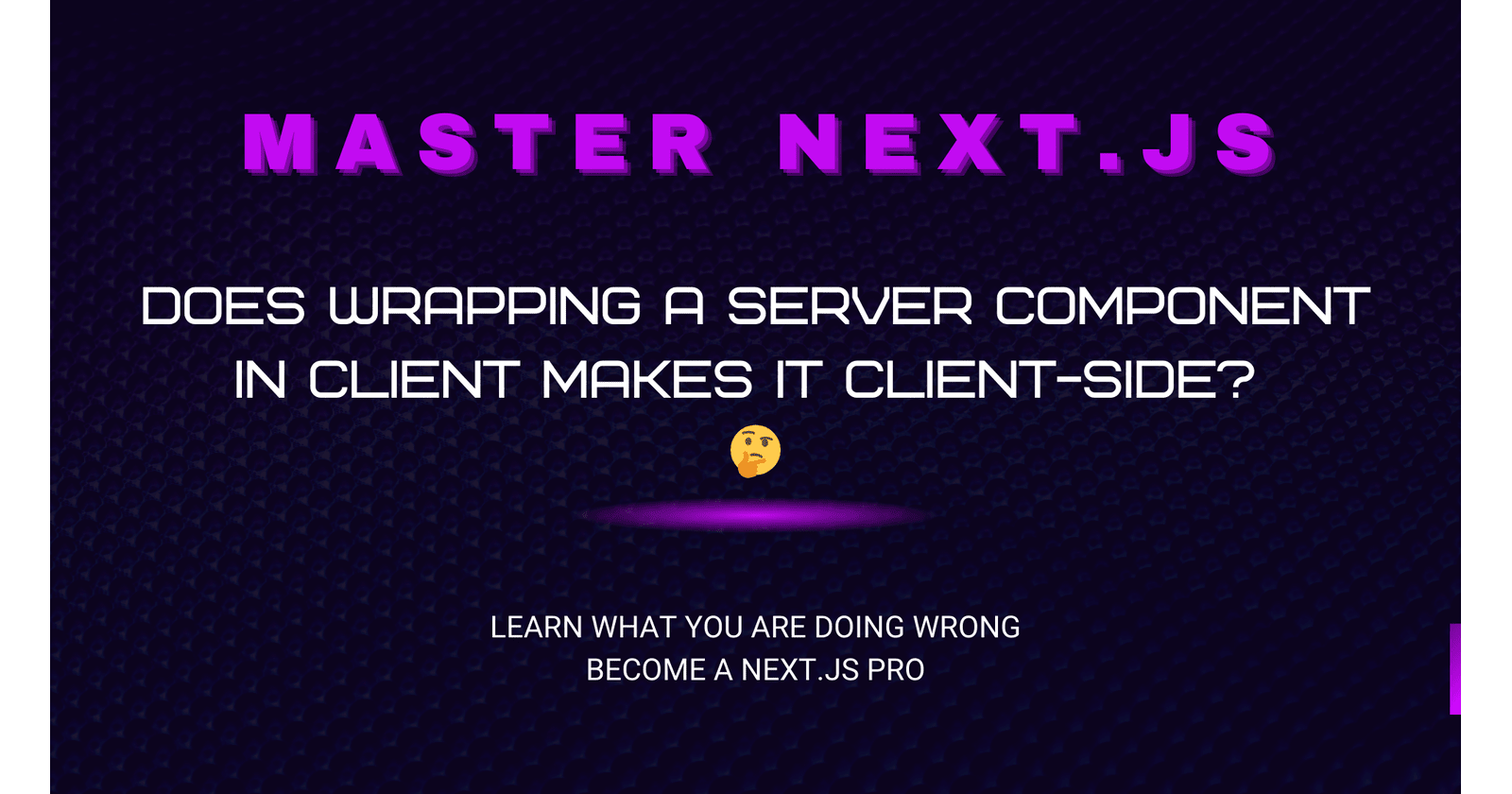
One of the most common mistakes developers make in Next.js is the assumption that if you wrap a server component inside a client component, it automatically becomes a client component. This misunderstanding often arises from the import behavior in Next.js, where importing components into a file marked as 'use client'
at the top turns any imported components into client components.
However, wrapping a server component in a client component and passing it as a child doesn’t work the same way. The server component will remain a server component, even if it's wrapped by or passed into a client component. Let’s dive deeper into this misconception, clarify it with examples, and understand why it doesn't work this way.
Understanding Why Wrapping Doesn’t Change Component Behavior
The distinction is important: when you pass a server component as a child to a client component, you're not importing it into the same file. You're rendering it, but it retains its server-side nature. This behavior ensures that Next.js retains the benefits of server-side rendering (SSR) without converting everything into client-side code.
Context API Misunderstanding
The confusion often deepens when developers work with React's Context API, which is frequently used for managing global state. The pattern is to create a client component that provides context to other components, often passing them as children
.
Here’s the flow:
The Context Provider is a client component because context in React is tied to client-side state.
Inside the provider, you pass server or client components as
children
.
If Wrapping Server Components Converted Them, It Would Defeat Next.js's Purpose
Many developers think that if you wrap the entire application (or certain server components) inside this client context provider, all components—including server components—will automatically behave as client components. This is not true.
If this were the case, it would defeat the purpose of using Next.js, where server components are used to optimize SSR, reduce client-side JavaScript, and improve performance. If wrapping a server component in a client component turned everything into client-side code, Next.js would essentially function as React, with no distinction between server and client components.
Breaking Down the Import Behavior
To understand why wrapping and importing behave differently, let’s look at two examples:
Example 1: Wrapping a Server Component in a Client Component
// ClientComponent.js
'use client'; // Client-side component
export default function ClientComponent({ children }) {
return <div>{children}</div>;
}
// ServerComponent.js
export default function ServerComponent() {
return <h1>This is a server component!</h1>;
}
// App.js
import ClientComponent from './ClientComponent';
import ServerComponent from './ServerComponent';
export default function App() {
return (
<ClientComponent>
<ServerComponent />
</ClientComponent>
);
}
In this example, we are passing the ServerComponent
as a child to ClientComponent
. Despite being inside the client component, the server component does not become a client component. It remains a server-side component and continues to render on the server. Next.js ensures that the behavior is preserved, optimizing SSR where possible.
Example 2: Importing a Server Component into a Client Component
// ClientComponent.js
'use client'; // Client-side component
import ServerComponent from './ServerComponent'; // Importing server component
export default function ClientComponent() {
return <ServerComponent />;
}
In this example, the ServerComponent is imported directly into the ClientComponent file. Since the file is marked with 'use client'
, the server component is now executed on the client and behaves as a client component. This approach can potentially lead to a larger client-side bundle and increased client-side JavaScript.
Why Understanding This Is Important
Performance: Assuming server components become client components when wrapped can lead to missed SSR benefits. Server components stay server-side unless imported into a
'use client'
file.Clarity: Knowing the difference between wrapping and importing helps maintain clear code. Server components should be passed down explicitly if server-side functionality is needed.
Conclusion: Wrapping ≠ Importing
Importing a server component into a
'use client'
file makes it a client component.Passing a server component as a child to a client component keeps it as a server component, retaining SSR benefits.
Next.js boosts performance by keeping server and client components separate, sending only necessary code to the client. Understanding this distinction helps you avoid the misconception that wrapping converts server components into client ones. By preserving server components, Next.js ensures faster, more efficient applications with optimal server-side rendering and client-side interactivity.
Subscribe to my newsletter
Read articles from Rishi Bakshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rishi Bakshi
Rishi Bakshi
Full Stack Developer with experience in building end-to-end encrypted chat services. Currently dedicated in improving my DSA skills to become a better problem solver and deliver more efficient, scalable solutions.