10 Performance Optimization Techniques for Next.js Applications
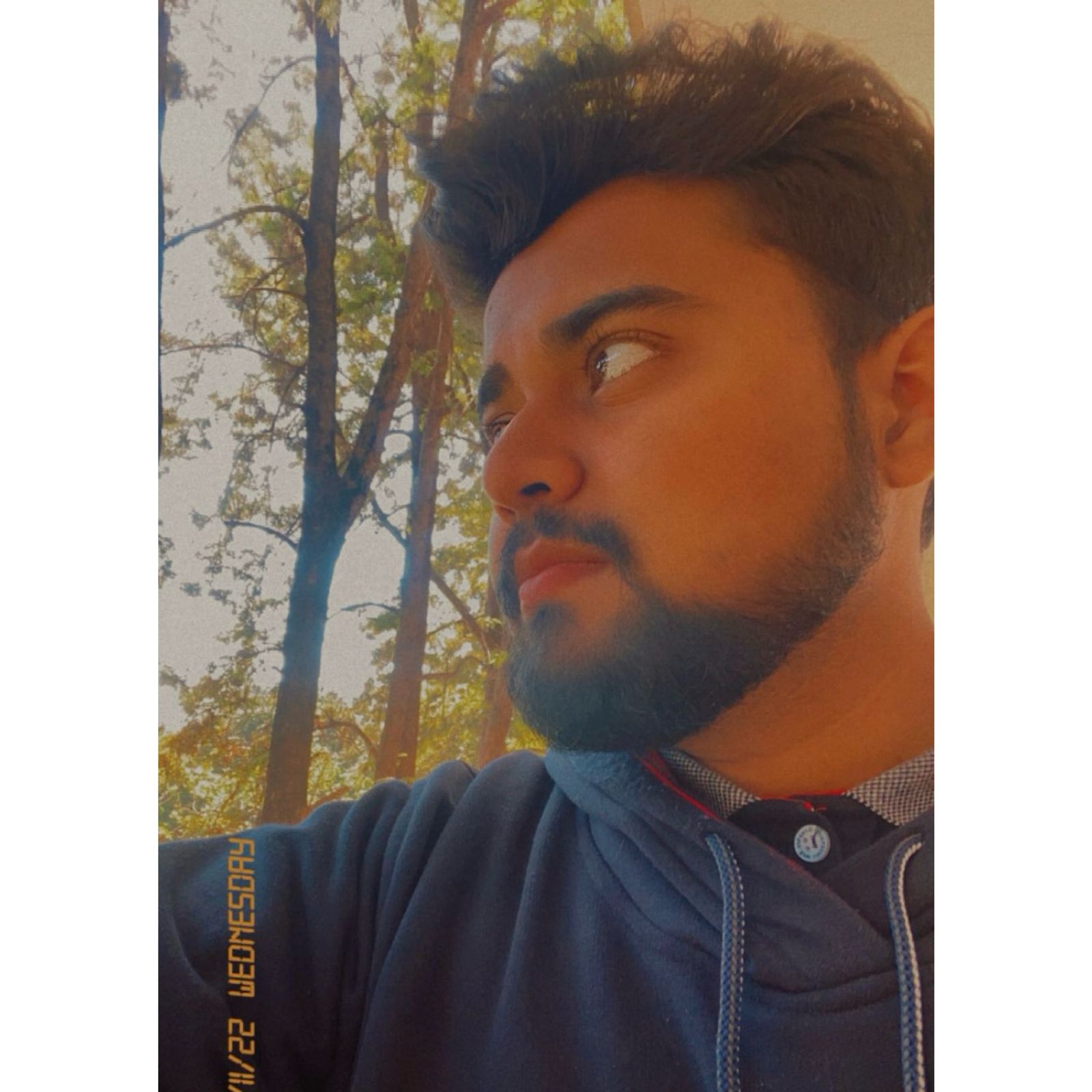
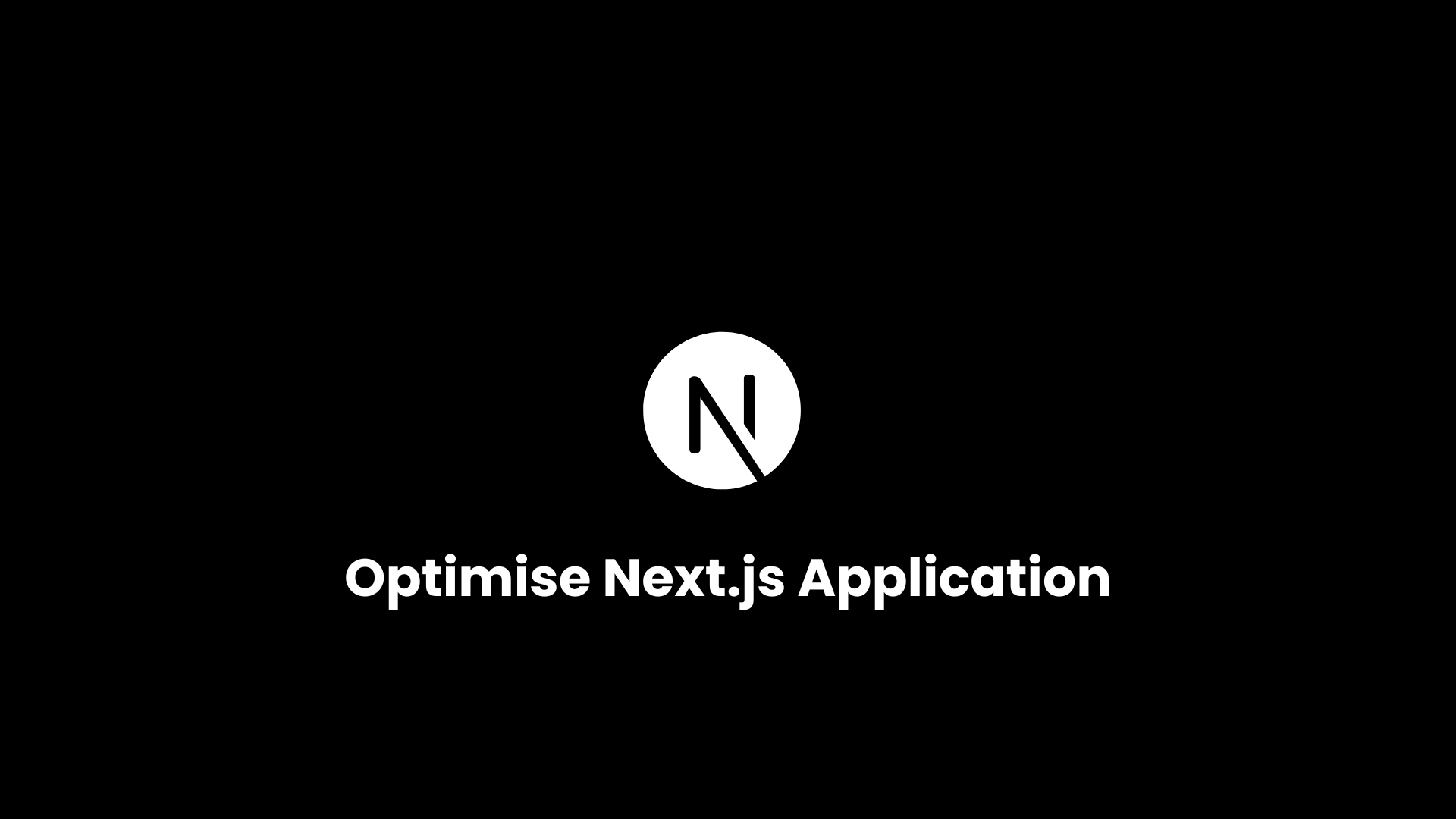
Next.js, a popular React framework, is known for its server-side rendering capabilities, built-in optimization features, and flexibility for creating high-performance web applications. However, building a performant Next.js application still requires careful consideration of several optimization strategies.
This article provides 10 essential techniques to help you improve the performance of your Next.js applications, ensuring faster load times, improved user experience, and better SEO rankings.
1. Use Image Optimization
Next.js offers built-in image optimization that helps you automatically serve optimized images. The <Image>
component provided by Next.js offers features such as lazy loading, responsive images, and automatic resizing.
How to Use:
/Replace the standard <img>
tags with Next.js <Image>
component:
import Image from 'next/image';
const HomePage = () => (
<div>
<Image
src="/images/sample.jpg"
alt="Sample Image"
width={800}
height={600}
layout="responsive"
/>
</div>
);
The <Image>
component automatically optimizes images for faster load times by using modern formats like WebP and serving the right size for different devices.
2. Enable Static Site Generation (SSG) and Incremental Static Regeneration (ISR)
Static Site Generation (SSG) pre-renders pages at build time, resulting in faster page loads. Incremental Static Regeneration (ISR) allows you to update static content after the build process, which is beneficial for content that changes frequently.
How to Implement:
Use the getStaticProps
function to generate static pages:
export async function getStaticProps() {
const data = await fetch('https://api.example.com/data');
return {
props: { data },
revalidate: 10, // Revalidate every 10 seconds
};
}
With ISR, you can serve static content while keeping it up-to-date without having to rebuild the entire site.
3. Use Code Splitting and Dynamic Imports
Code splitting is a technique that splits your code into smaller bundles that are loaded only when necessary. Next.js supports dynamic imports that allow you to import components only when they are needed.
How to Use:
To dynamically import a component, use next/dynamic
:
import dynamic from 'next/dynamic';
const HeavyComponent = dynamic(() => import('../components/HeavyComponent'), {
ssr: false,
});
const HomePage = () => (
<div>
<h1>Welcome to My Site</h1>
<HeavyComponent />
</div>
);
This will load HeavyComponent
only on the client side, improving the initial load time.
4. Optimize Fonts with next/font
Web fonts can be a major contributor to slow page loads. Next.js provides a built-in solution to optimize custom fonts by loading them in a way that improves both performance and layout stability.
How to Use:
Utilize the built-in next/font
package to manage custom fonts:
import { Inter } from 'next/font/google';
const inter = Inter({ subsets: ['latin'] });
const HomePage = () => (
<main className={inter.className}>
<h1>Hello, Next.js</h1>
</main>
);
This method ensures that fonts are loaded efficiently and without blocking rendering.
5. Enable Compression with gzip or Brotli
Enable HTTP compression on the server side to reduce the size of the files sent to the client. This can be done by setting up gzip or Brotli compression in your hosting provider or custom server configuration.
How to Implement:
If you are deploying on Vercel or another cloud provider, compression is usually enabled by default. For a custom server, use a middleware like compression
for Express:
const compression = require('compression');
const express = require('express');
const next = require('next');
const app = next({ dev });
const handle = app.getRequestHandler();
const server = express();
server.use(compression());
server.get('*', (req, res) => handle(req, res));
server.listen(3000, () => console.log('Server running on port 3000'));
6. Leverage Content Delivery Network (CDN) for Static Assets
Serving static assets such as images, stylesheets, and scripts via a CDN can significantly reduce load times by caching them closer to the user.
How to Use:
Most hosting providers like Vercel, Netlify, and AWS automatically provide CDN capabilities. Make sure to store your static files in the /public
folder so they can be effectively cached and served by the CDN.
7. Use React.memo and useMemo
for Component Optimization
React provides React.memo
and useMemo
hooks to memoize components and values, reducing unnecessary re-renders and boosting performance.
How to Use:
Wrap components with React.memo
to prevent them from re-rendering unless their props change:
const MemoizedComponent = React.memo(function MyComponent({ data }) {
return <div>{data}</div>;
});
Use useMemo
for expensive calculations or derived data:
const memoizedValue = useMemo(() => computeExpensiveValue(data), [data]);
8. Pre-fetch Data and Links
Next.js has built-in support for link prefetching. When a link is visible in the viewport, Next.js will automatically pre-fetch the linked page in the background.
How to Use:
Use the <Link>
component with the prefetch
prop:
import Link from 'next/link';
const HomePage = () => (
<Link href="/about" prefetch={true}>
About Us
</Link>
);
This reduces the load time when a user navigates to a new page.
9. Implement Lazy Loading for Non-Essential Components
Lazy loading defers the loading of non-essential components until they are needed, which can improve initial load time.
How to Implement:
Use dynamic imports with React’s Suspense
:
import dynamic from 'next/dynamic';
const LazyComponent = dynamic(() => import('../components/LazyComponent'));
const HomePage = () => (
<div>
<h1>My Next.js Application</h1>
<LazyComponent />
</div>
);
This loads LazyComponent
only when it is needed, improving performance.
10. Analyze and Monitor Performance with Lighthouse and Web Vitals
Regularly analyze and monitor your Next.js app’s performance using tools like Google Lighthouse and Web Vitals.
How to Use:
Use Google Lighthouse in Chrome DevTools to get a performance report.
Use Next.js Analytics to measure Web Vitals in production.
This data helps identify bottlenecks and guide future optimizations.
Conclusion
Optimizing a Next.js application is an ongoing process that involves a combination of built-in features and additional techniques to improve speed, responsiveness, and user experience. By implementing these 10 optimization strategies, you can ensure that your Next.js applications run efficiently and effectively, delighting users and improving your website's SEO performance.
Start optimizing today and take your Next.js applications to the next level! 🚀
Subscribe to my newsletter
Read articles from Anupam Shakya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
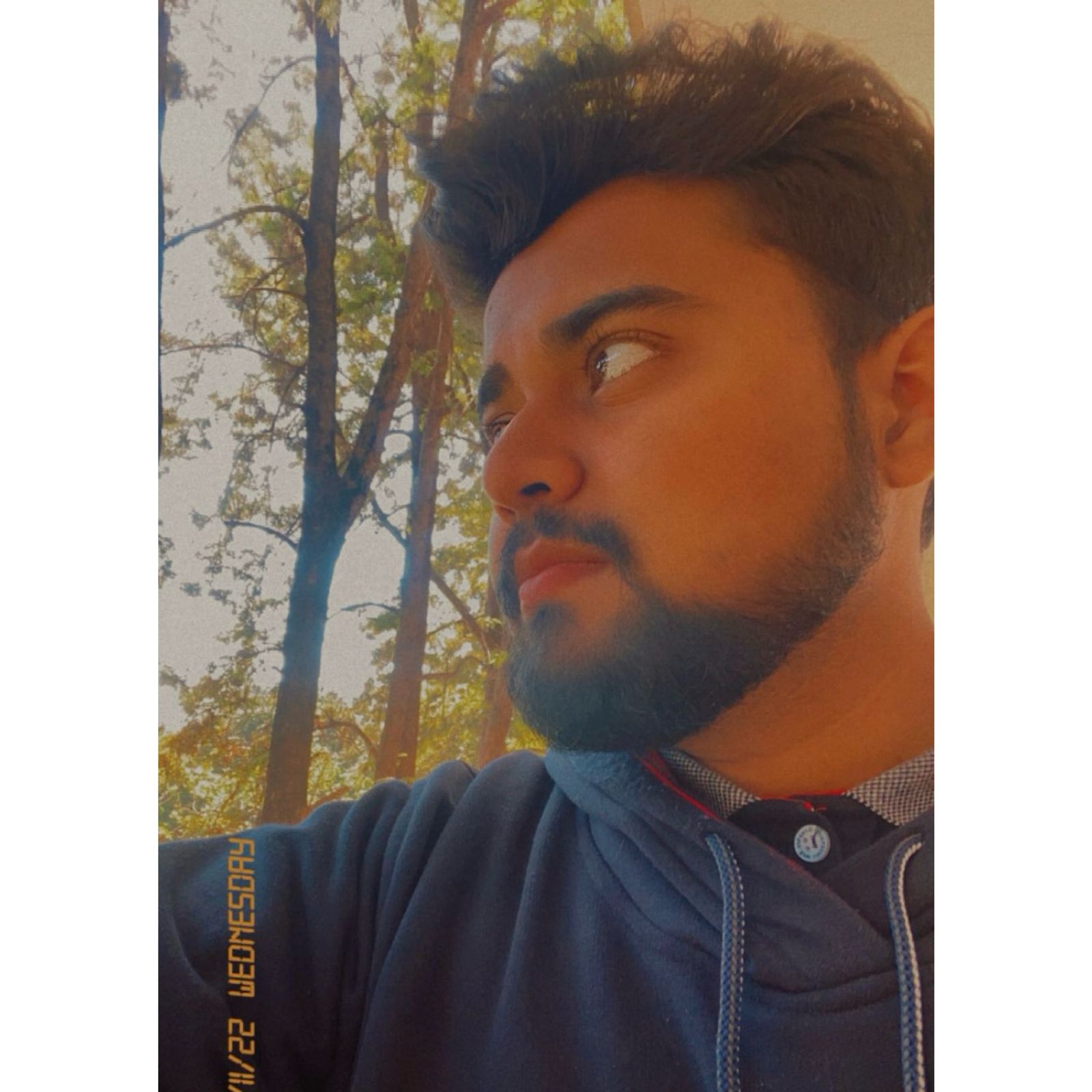
Anupam Shakya
Anupam Shakya
I am an aspiring software engineer and MCA graduate passionate about sharing my knowledge and experiences to help others advance in their careers. I am a Frontend Developer Intern at iNueron.Ai, an Ex-SDE Intern at ITJobxs, As a web developer, I have experience in Next.js, React.js, Tailwind CSS, and the MERN stack. Feel free to reach out for collaboration, mentorship, or any exciting project opportunities: Email: hello@anupamshakya.in Portfolio: https://anupamshakya.in