Kotlin Learning Path
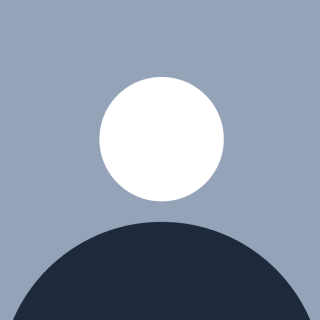
Table of contents
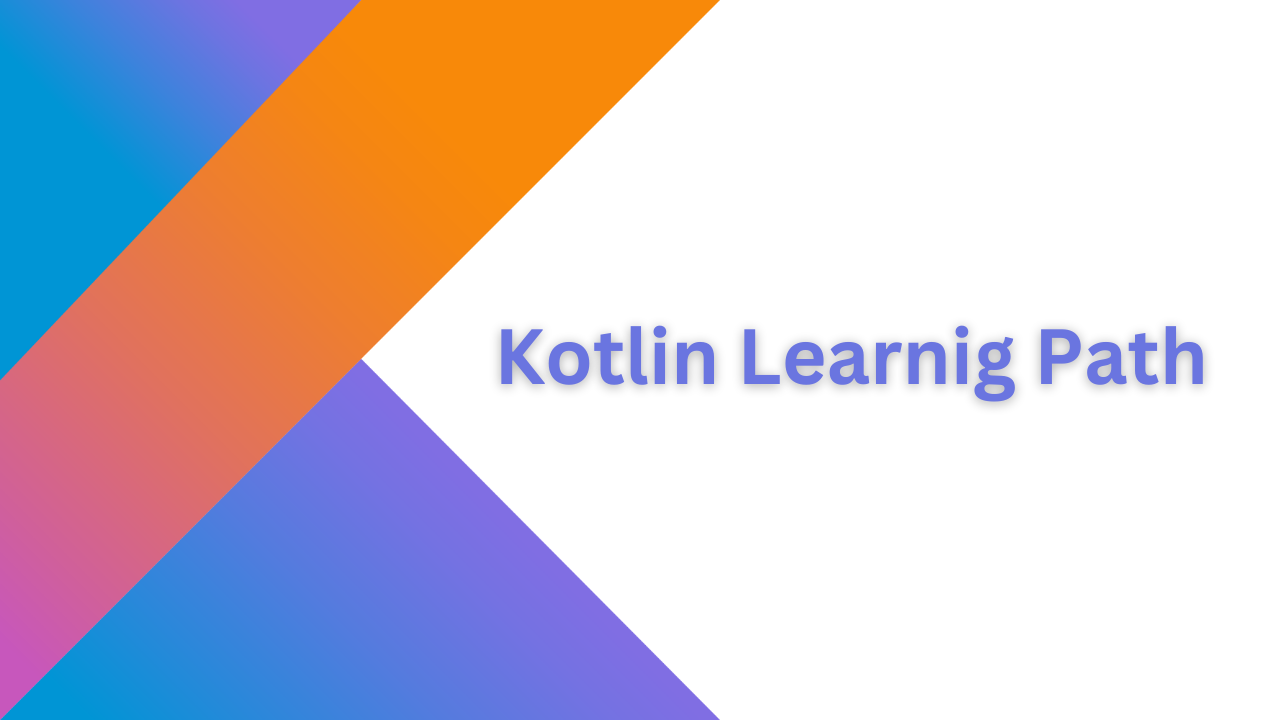
Kotlin Index
Kotlin Language Basics Start with the syntax and fundamental building blocks of the Kotlin language.
Kotlin main() Function
Kotlin Comments
Kotlin Packages
Kotlin Tokens
Kotlin Keywords and Identifiers
Java Variables and Literals
Kotlin Data Types
Kotlin Type Casting
Kotlin Operators
Java Basic Input/Output
Kotlin Expressions, Statements and Blocks
Pass-by-value or Pass-by-reference
Flow Control Statements Learn to write statements and control the flow of the programs.
Kotlin if-else
Kotlin when
Ternary Operator
Kotlin for loop
Kotlin for-each loop
Kotlin while loop
Kotlin do-while loop
Kotlin break Keyword
Kotlin continue Keyword
Labeled Statements
Kotlin OOP Learn to create, arrange, and manage objects and their relationships in Kotlin.
Building Blocks of OOP These concepts are the building blocks and pillars for object-oriented programming.
Intro to Object-Oriented Programming
Association, Aggregation, and Composition
Guide to Abstraction
Guide to Inheritance
Guide to Polymorphism
Multiple Inheritance in Kotlin
OOP in Kotlin The given articles describe how Kotlin supports the OOP paradigm.
Constructors in Kotlin
Kotlin Access Modifiers
Kotlin
object
KeywordKotlin
final
KeywordKotlin Initializer Blocks
Kotlin
is
OperatorOverriding final static method in Kotlin
Difference Between Go through the differences between some very similar concepts to understand them even better.
Overloading vs Overriding in Kotlin
Encapsulation vs Abstraction in Kotlin
Interface vs Abstract Class in Kotlin
Kotlin
extends
vsimplements
Keywords
Kotlin Strings Strings are always the most used constructs in any programming language. Learn to work on String in Kotlin.
Guide to String Class
Kotlin String Pool
Why Strings are Immutable in Kotlin?
Splitting a String
Joining the Strings
Kotlin Exceptions A program can raise errors due to errors in the program as well as runtime errors in the execution environment. Learn to handle both.
Kotlin try-catch-finally blocks
Kotlin Try with Resources
Kotlin throw and throws Keywords
Checked and Unchecked Exceptions
Kotlin Custom Exceptions
Kotlin Synchronous and Asynchronous Exceptions
Kotlin NullPointerException
Kotlin Suppressed Exceptions
Exception Handling Best Practices
Kotlin Array Arrays are the most basic container of multiple elements. Learn to work on arrays in Kotlin.
Guide to Array in Kotlin
Printing an Array
Cloning an Array
Copying Array Range
Intersection of Two Arrays
Union of Two Arrays
Kotlin Collections Apart from the array, Kotlin supports more advanced collection types.
Kotlin List
Kotlin Map
Kotlin Comparable
Kotlin Comparator
Kotlin Iterator
Kotlin Generics
Kotlin Sorting
Kotlin IO Learn the most basic read-and-write operations on files in Kotlin.
How Kotlin IO Works Internally?
Create a File
Read a File
Write to a File
Append to a File
Kotlin Coroutines Coroutines are a powerful feature in Kotlin for asynchronous programming.
Introduction to Coroutines
Coroutine Builders
Suspending Functions
Coroutine Context and Dispatchers
Structured Concurrency
Exception Handling in Coroutines
Kotlin Streams Streams are a rather new addition to the language but have made it super easy.
Kotlin Stream API
Functional Interfaces
Default Methods
Lambda Expressions
Kotlin Runtime Learn to set up and interact with the Kotlin development environment.
JDK vs. JRE vs. JVM
Kotlin 32 bit vs 64 bit
kotlin.exe vs kotlinw.exe
Kotlin Classpath
System Properties
Command-Line Arguments
Little-Endien vs Big-Endien
Generating Bytecode
Misc Topics
Builder Pattern for Kotlin Data Classes
Kotlin Annotations Tutorial
Guide to Kotlin Cloning
Kotlin Versions and Features
Kotlin Examples
Printf-Style Output Formatting
Rounding off to 2 Decimal Places
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer