Automating Data Cleaning with Python and Machine Learning
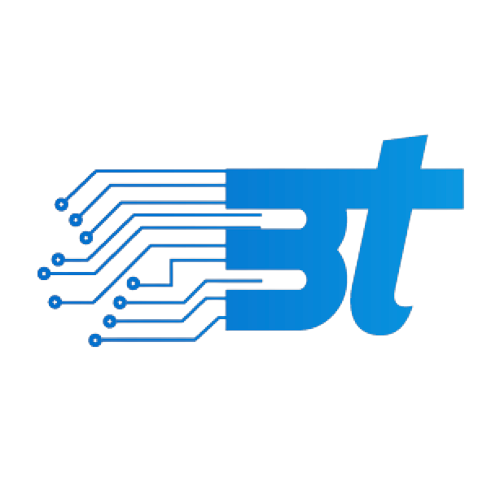
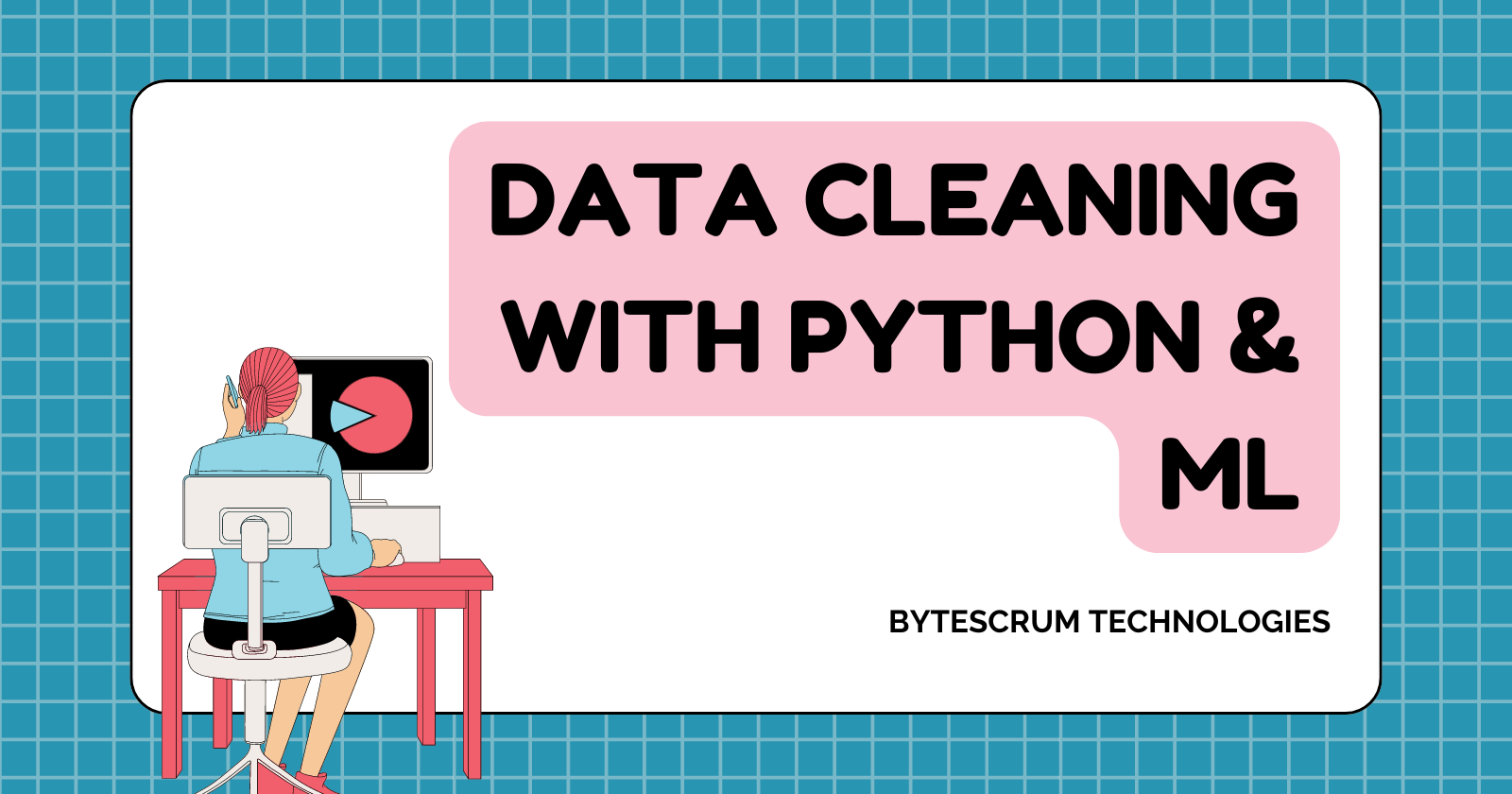
Data cleaning is an essential step in the data preprocessing pipeline, accounting for the majority of the time spent on data-related tasks. Dirty data—missing values, incorrect formats, duplicates, and outliers—can significantly affect machine learning model performance and the overall quality of analysis. Automating this process not only saves time but also ensures consistency and accuracy.
In this blog, we’ll explore how to automate data cleaning using Python, leveraging tools like pandas
, numpy
, and machine learning techniques to handle missing values, outliers, and more.
1. Setting Up the Environment
Before diving into the code, ensure that the following libraries are installed. You can install them via pip
:
pip install pandas numpy scikit-learn missingno matplotlib seaborn
pandas
: For data manipulation and analysis.numpy
: For numerical operations.scikit-learn
: For machine learning techniques.missingno
: For visualizing missing data.matplotlib
andseaborn
: For visualization.
2. Loading the Data
Let’s begin by loading a sample dataset that includes common problems like missing values, duplicates, and outliers.
import pandas as pd
# Load a dataset (example: customer data)
data = pd.read_csv("customer_data.csv")
# Display the first few rows
print(data.head())
3. Handling Missing Data
Missing data is one of the most common issues in real-world datasets. We can automate the detection, visualization, and handling of missing values.
Visualizing Missing Data
You can quickly visualize the missing data using the missingno
library:
import missingno as msno
# Visualize missing data
msno.matrix(data)
Automating Missing Data Imputation
Missing data can be handled in multiple ways:
Dropping missing values: When there are few missing values.
Imputation using statistical measures: Filling in missing values with mean, median, or mode.
Using machine learning models for imputation: Predicting missing values using models.
Here’s how you can automate this:
from sklearn.impute import SimpleImputer
# Define a simple imputer to fill missing values with the mean (for numerical columns)
num_imputer = SimpleImputer(strategy='mean')
# Apply imputer to the dataset
data[['age', 'income']] = num_imputer.fit_transform(data[['age', 'income']])
# Alternatively, for categorical data, use the most frequent value
cat_imputer = SimpleImputer(strategy='most_frequent')
data[['gender', 'city']] = cat_imputer.fit_transform(data[['gender', 'city']])
For more advanced imputations, you can use regression models (e.g., Random Forest) to predict missing values.
4. Dealing with Duplicates
Duplicates can skew the analysis and model predictions. Here’s how to automate their detection and removal:
# Check for duplicates
duplicates = data.duplicated()
# Remove duplicates
data_cleaned = data.drop_duplicates()
print(f"Number of duplicates removed: {sum(duplicates)}")
5. Detecting and Handling Outliers
Outliers can distort the results of statistical analyses and machine learning models. A common technique is to use the interquartile range (IQR) or Z-scores to detect outliers.
Automating Outlier Detection using IQR
# Define a function to detect outliers using IQR
def detect_outliers_iqr(df, column):
Q1 = df[column].quantile(0.25)
Q3 = df[column].quantile(0.75)
IQR = Q3 - Q1
lower_bound = Q1 - 1.5 * IQR
upper_bound = Q3 + 1.5 * IQR
return df[(df[column] < lower_bound) | (df[column] > upper_bound)]
# Example: Detect outliers in 'income' column
outliers = detect_outliers_iqr(data_cleaned, 'income')
print(f"Number of outliers in income: {len(outliers)}")
Removing or Capping Outliers
You can either remove outliers or cap them to a specified threshold:
# Remove outliers
data_cleaned = data_cleaned[~data_cleaned.index.isin(outliers.index)]
# Alternatively, cap the outliers
data_cleaned['income'] = data_cleaned['income'].apply(lambda x: min(max(x, lower_bound), upper_bound))
6. Feature Scaling
Scaling features ensures that they are on the same scale, improving the performance of machine learning algorithms.
from sklearn.preprocessing import StandardScaler
# Apply standard scaling to numerical columns
scaler = StandardScaler()
data_cleaned[['age', 'income']] = scaler.fit_transform(data_cleaned[['age', 'income']])
7. Automating Feature Encoding
Many machine learning models require categorical variables to be converted into numerical values. We can use one-hot encoding or label encoding:
# One-hot encoding for categorical features
data_cleaned = pd.get_dummies(data_cleaned, columns=['gender', 'city'], drop_first=True)
8. Building a Machine Learning Pipeline for Automated Cleaning
You can automate all of the above steps using a machine learning pipeline:
from sklearn.pipeline import Pipeline
from sklearn.compose import ColumnTransformer
from sklearn.preprocessing import OneHotEncoder
# Define the preprocessing pipeline
numeric_features = ['age', 'income']
categorical_features = ['gender', 'city']
# Numeric preprocessing (impute missing values, scale)
numeric_transformer = Pipeline(steps=[
('imputer', SimpleImputer(strategy='mean')),
('scaler', StandardScaler())
])
# Categorical preprocessing (impute missing values, one-hot encode)
categorical_transformer = Pipeline(steps=[
('imputer', SimpleImputer(strategy='most_frequent')),
('onehot', OneHotEncoder(drop='first'))
])
# Combine numeric and categorical transformers
preprocessor = ColumnTransformer(
transformers=[
('num', numeric_transformer, numeric_features),
('cat', categorical_transformer, categorical_features)
])
# Apply the preprocessor
data_prepared = preprocessor.fit_transform(data_cleaned)
Conclusion
Automating data cleaning not only reduces manual effort but also increases productivity, enabling data scientists and engineers to focus on more critical aspects of the data pipeline.
Happy coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
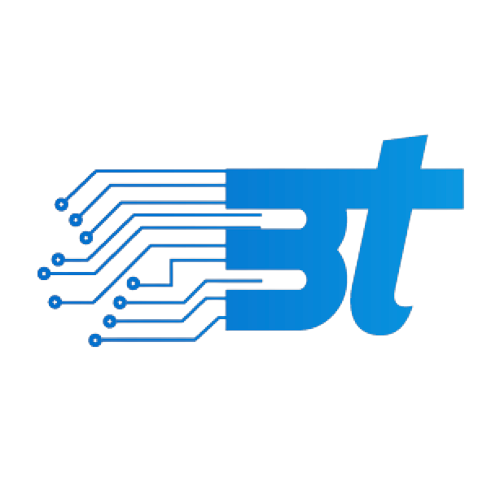
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.