Kotlin Naming Conventions
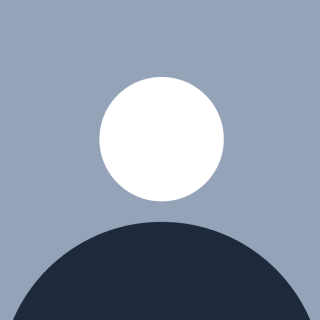
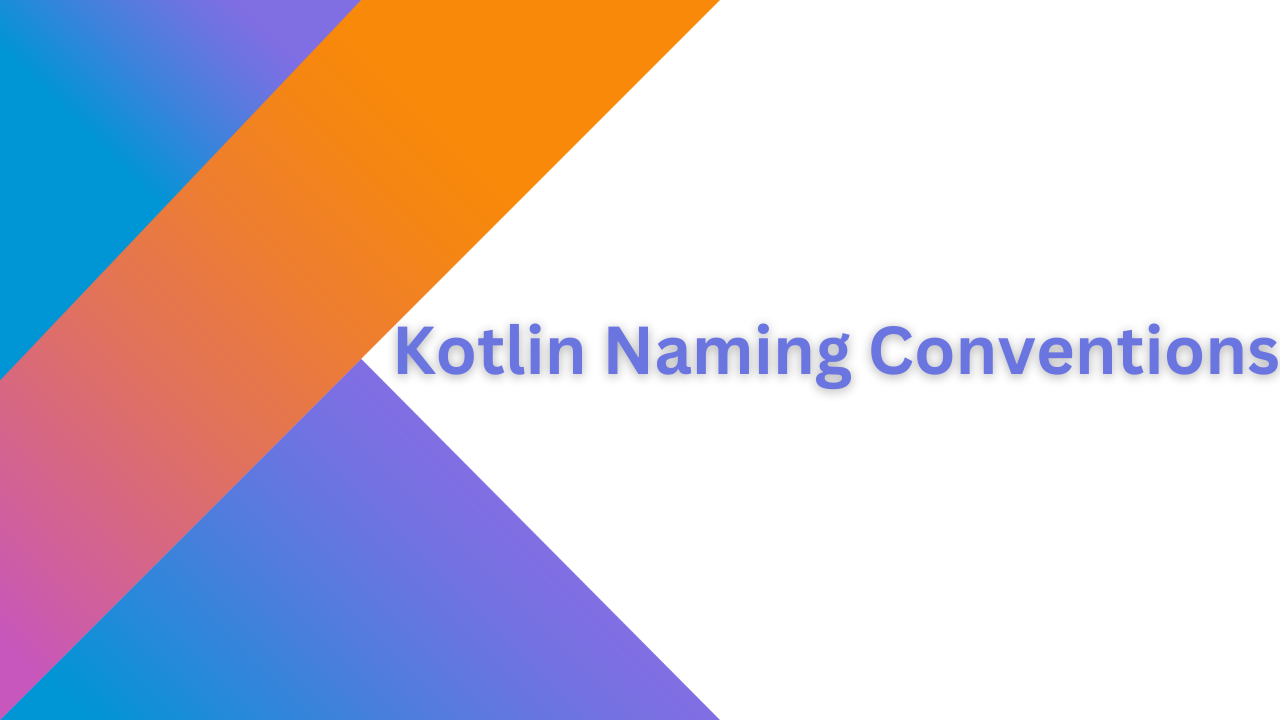
Naming conventions are essential guidelines for writing consistent and readable code. When working with Kotlin, following these conventions ensures that your code remains clear and maintainable. Let’s explore some commonly used naming conventions in Kotlin:
Package Names
Package names should start with lowercase domain names (e.g., com, org, net).
Subsequent parts of the package name can vary based on your organization’s internal conventions.
Example:
package com.dilip.webapp.controller package com.company.myapplication.web.controller package com.google.search.common
Class Names
Class names should be nouns in title case (first letter of each separate word capitalized).
Example:
class ArrayList class Employee class Record class Identity
Interface Names
Interface names should be adjectives or, in some cases, nouns representing a family of classes.
Use title case for interface names.
Example:
interface Serializable interface Clonable interface Iterable interface List
Method Names
Method names should be verbs, clearly indicating the action they perform.
Use camel case notation for method names.
Example:
fun getId(): Long fun remove(o: Object) fun update(o: Object) fun getReportById(id: Long): Report fun getReportByName(name: String): Report
Variable Names
Variable names (instance, static, and method parameters) should be in camel case.
Choose descriptive names that convey their purpose.
Example:
val id: Long val employeeDao: EmployeeDao var properties: Properties
Constant Naming Conventions
Constants should be in all uppercase with words separated by underscores.
Use the
final
modifier for constant variables.Example:
val SECURITY_TOKEN = "..." val INITIAL_SIZE = 16 val MAX_SIZE = Integer.MAX_VALUE
Naming Generic Types
Generic type parameter names should consist of uppercase single letters.
The letter ‘T’ (for type) is commonly recommended.
In JDK classes:
E
is used for collection elements.S
is used for service loaders.K
andV
are used for map keys and values.
Example:
class MyGenericClass<T> interface Map<K, V> {} interface List<E> : Collection<E> {} fun <E> Iterator<E>.iterator() {}
Naming Enums
Similar to class constants, enumeration names should be in all uppercase letters.
Example:
enum class Direction { NORTH, EAST, SOUTH, WEST }
Naming Annotations
Annotation names follow title case notation.
They can be adjectives, verbs, or nouns based on their purpose.
Example:
annotation class FunctionalInterface annotation class Deprecated annotation class Documented @Async annotation class MyAsyncAnnotation @Test annotation class MyTestAnnotation
Conclusion
In this article, we’ve discussed essential naming conventions in Kotlin. Consistently applying these conventions ensures that your code remains readable and maintainable. The Kotlin tutorial page lists down all the important topics you can go through to get a deeper understanding of the language basics and advanced concepts.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer