Day-1 JavaScript Notes 2024

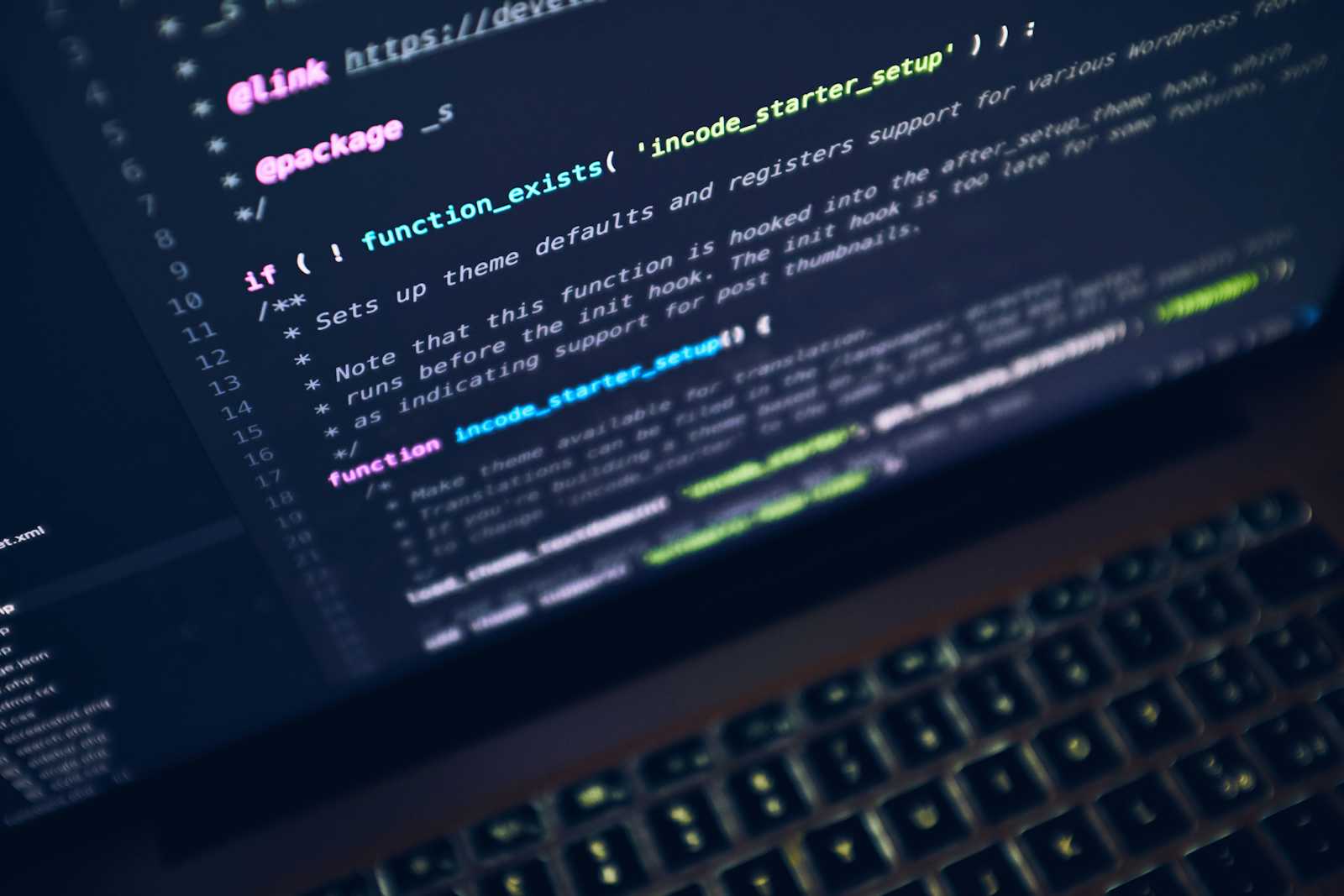
The basic JavaScript concepts, along with examples and a summary table for revision:
1. Variables
Definition: Variables store data values. In JavaScript, you can declare variables using
var
,let
, orconst
.var
: Function-scoped.let
: Block-scoped.const
: Block-scoped, but the value cannot be reassigned.
Example:
var name = "Srinidhi"; let age = 21; const country = "India"; console.log(name); console.log(age); console.log(country); /* Output: Srinidhi 21 India */
2. Data Types
Definition: JavaScript has dynamic types, which means the same variable can hold different types of data. Common data types include:
Primitive Types: Number, String, Boolean, Null, Undefined, Symbol, BigInt.
Non-Primitive Types: Object (including Arrays and Functions).
Example:
let name = "Srinidhi"; // String let age = 21; // Number let isStudent = true; // Boolean let skills = ["JavaScript", "HTML", "CSS"]; // Array let person = { firstName: "John", lastName: "Doe" }; // Object let notAssigned; // Undefined let empty = null; // Null // accessing array and object elemnts will be in Day 3 blog
3. Operators
Arithmetic Operators:
+
,-
,*
,/
,%
,++
,--
.// Arithmetic Operators //+, -, *, /, %, ++, -- (Pre,Post) let a = 10; let b = 5; console.log("Arithmetic Operators:"); console.log('a + b = ${a + b} observe single quote and cross quote closings while logging');//output is: a + b = ${a + b} console.log(`a + b = ${a + b}`); // Addition: 10 + 5 = 15 console.log(`a - b = ${a - b}`); // Subtraction: 10 - 5 = 5 console.log(`a * b = ${a * b}`); // Multiplication: 10 * 5 = 50 console.log(`a / b = ${a / b}`); // Division: 10 / 5 = 2 console.log(`a % b = ${a % b}`); // Modulus: 10 % 5 = 0 console.log(`++a = ${++a} Pre-increment: a=10 becomes 11 logs-out 11`); // Pre-increment: a=10 becomes 11 prints 11 console.log(`--b = ${--b} Pre-decrement: b=5 becomes 4 logs-out 4`); // Pre-decrement: b=5 becomes 4 prints 4 console.log(`a++ = ${a++} post inc: a=11 logs-out 11 then becomes 12`); //post inc: a=11 prints 11 then becomes 12 console.log(`b++ = ${b++} post inc: b=4 logs-out 4 then becomes 5`); //post inc: b=4 prints 4 then becomes 5 /* OutPut: Arithmetic Operators: a + b = ${a + b} observe single quote and cross quote closings while logging a + b = 15 a - b = 5 a * b = 50 a / b = 2 a % b = 0 ++a = 11 Pre-increment: a=10 becomes 11 logs-out 11 --b = 4 Pre-decrement: b=5 becomes 4 logs-out 4 a++ = 11 post inc: a=11 logs-out 11 then becomes 12 b++ = 4 post inc: b=4 logs-out 4 then becomes 5 */
Comparison Operators: ==
, ===
, !=
, !==
, >
, <
, >=
, <=
.
// Comparision Operators
//==, ===, !=, !==, >, <, >=, <=
let x = 20;
let y = 15;
let a = 3;
let b = 3;
console.log("\nComparison Operators:\nx=20, y=15, a=3, b=3");
console.log(`x == y: ${x == y}`); // Equal to: 20 == 15 => false
console.log(`x === y: ${x === y}`); // Strict equal to: 20 === 15 => false
console.log(`a == b : ${a == b}`);
console.log(`a === b : ${a === b}`);
console.log(`x != y: ${x != y}`); // Not equal to: 20 != 15 => true
console.log(`x !== y: ${x !== y}`); // Strict not equal to: 20 !== 15 => true
console.log(`a != b : ${a != b}`);
console.log(`a !== b : ${a !== b}`);
console.log(`x > y: ${x > y}`); // Greater than: 20 > 15 => true
console.log(`x < y: ${x < y}`); // Less than: 20 < 15 => false
console.log(`x >= y: ${x >= y}`); // Greater than or equal to: 20 >= 15 => true
console.log(`x <= y: ${x <= y}`); // Less than or equal to: 20 <= 15 => false
console.log('\nMore about ==, ===, !=, !===:');
console.log(`5 == '5' : ${5 == '5'} => true (string '5' is converted to number 5)`); // true (string '5' is converted to number 5)
console.log(`null == undefined : ${null == undefined} => true (null and undefined are considered equal)`); // true (null and undefined are considered equal)
console.log(`5 === '5' : ${5 === '5'} => false (different types: number vs string)`); // false (different types: number vs string)
console.log(`null === undefined : ${null === undefined} => false (different types: null vs undefined)`); // false (different types: null vs undefined)
console.log(`5 != '5' : ${5 != '5'} => false (string '5' is converted to number 5, so they are considered equal)`); // false (string '5' is converted to number 5, so they are considered equal)
console.log(`null != undefined : ${null != undefined} => false (null and undefined are considered equal)`); // false (null and undefined are considered equal)
console.log(`5 !== '5' : ${5 !== '5'} => true (different types: number vs string)`); // true (different types: number vs string)
console.log(`null !== undefined : ${null !== undefined} => true (different types: null vs undefined)`); // true (different types: null vs undefined)
/* OutPut:
Comparison Operators:
x=20, y=15, a=3, b=3
x == y: false
x === y: false
a == b : true
a === b : true
x != y: true
x !== y: true
a != b : false
a !== b : false
x > y: true
x < y: false
x >= y: true
x <= y: false
More about ==, ===, !=, !===:
5 == '5' : true => true (string '5' is converted to number 5)
null == undefined : true => true (null and undefined are considered equal)
5 === '5' : false => false (different types: number vs string)
null === undefined : false => false (different types: null vs undefined)
5 != '5' : false => false (string '5' is converted to number 5, so they are considered equal)
null != undefined : false => false (null and undefined are considered equal)
5 !== '5' : true => true (different types: number vs string)
null !== undefined : true => true (different types: null vs undefined)
*/
Operator | Comparison Type | Type check | Example | Result |
\== | equality | yes | 5 == '5' | true, reason converts str -> int, then both be of same type hence true |
\=== | strict equality | no | 5 === '5' | false, checked as raw they are different types, hence false |
!= | inequality | yes | 5 != '5' | false, when type converts both be equal so its asking for inequality hence false |
!== | strict inequality | no | 5 !=== '5' | true checked as raw, both belong to different types so yeah they are different hence unequal hence true |
- Logical Operators:
&&
,||
,!
.
// Logical Operators
//&& || !
let a = true;
let b = false;
console.log("\nLogical Operators:");
console.log(`a && b: ${a && b}`); // AND: true && false => false
console.log(`a || b: ${a || b}`); // OR: true || false => true
console.log(`!a: ${!a}`); // NOT: !true => false
/*Output:
Logical Operators:
a && b: false
a || b: true
!a: false
*/
- Assignment Operators:
=
,+=
,-=
,*=
,/=
.
// Assignment Operators
// =, +=, -=, *=, /=
let c = 10;
console.log("\nAssignment Operators:");
c += 5; // c = c + 5
console.log(`c += 5: ${c}`); // 15
c -= 3; // c = c - 3
console.log(`c -= 3: ${c}`); // 12
c *= 2; // c = c * 2
console.log(`c *= 2: ${c}`); // 24
c /= 4; // c = c / 4
console.log(`c /= 4: ${c}`); // 6
c %= 2; // c = c % 2
console.log(`c %= 2: ${c}`); // 0
4. Control Flow
if/else: Executes a block of code if a condition is true.
switch: Performs different actions based on different conditions.
Example:
let time = 18; if (time < 12) { console.log("Good Morning"); } else { console.log("Good Evening"); } let day = "Monday"; switch(day) { case "Monday": console.log(`Start of the week! Its : ${day}`); break; case "Friday": console.log(`Weekend is near! Its : ${day}`); break; default: console.log("Just another day"); } /*Output: Good Evening Start of the week! Its : Monday */
5. Loops
for: Repeats a block of code a specified number of times.
while: Repeats a block of code while a condition is true.
Example:
console.log("For loop 0 -> 4"); for(let i=0 ; i<5 ; i++) { console.log(i); } console.log("While loop 5 -> 1"); let j = 5; while(j > 0) { console.log(j); j--; } /*Output: For loop 0 -> 4 0 1 2 3 4 While loop 5 -> 1 5 4 3 2 1 */
6. Functions
Definition: Functions are blocks of code designed to perform a particular task, invoked by a function call.
Example:
function greet(name) { return `Hello, ${name}!`; } console.log(greet("Srinidhi")); // Outputs: Hello, Srinidhi!
7. Scope
Definition: Scope refers to the visibility or accessibility of variables. There are two types:
Global Scope: Variables declared outside any function/block are globally scoped.
Local Scope: Variables declared inside a function/block are locally scoped.
Example:
let globalVar = "Global"; // Global scope function checkScope() { let localVar = "Local"; // Local scope console.log(globalVar); // Accessible console.log(localVar); // Accessible } checkScope(); // This is commented bcoz it throws error, //console.log(localVar); // Error: localVar is not defined /*Actual Output Global Local */
8. Hoisting
Definition: Hoisting is JavaScript's default behavior of moving declarations to the top of the current scope before code execution. Only the declarations are hoisted, not the initializations.
Example:
console.log(myVar); // Outputs: undefined (due to hoisting) var myVar = 10; // Declaration is hoisted, but not the initialization console.log(myVar); /*output: undefined 10 */
Summary Table for Revision
Concept | Explanation | Example |
Variables | Stores data values (var , let , const ). | let name = "Srinidhi"; |
Data Types | Primitive (Number, String, etc.) and Non-Primitive (Object, Array). | let isActive = true; |
Operators | Arithmetic, Comparison, Logical, Assignment operators. | let sum = a + b; |
Control Flow | if/else and switch for decision-making. | if (x > 0) {...} else {...} |
Loops | for , while for repeating blocks of code. | for (let i = 0; i < 5; i++) {...} |
Functions | Reusable blocks of code. | function greet() {...} |
Scope | Determines the visibility of variables (Global vs Local). | let globalVar = "Global"; |
Hoisting | JavaScript moves variable declarations to the top of their scope. | console.log(x); var x = 5; |
Comprehensive Example Covering All Concepts
// Variable Declaration
let userName = "Srinidhi";
const birthYear = 2002;
var currentYear = 2024;
// Function Declaration
function calculateAge(birthYear, currentYear) {
// Using Arithmetic and Assignment Operators
let age = currentYear - birthYear;
// Control Flow with if/else
if (age >= 18) {
return `You are ${age} years old and eligible to vote.`;
} else {
return `You are ${age} years old and not eligible to vote yet.`;
}
}
// Loop through years and log eligibility
for (let year = birthYear; year <= currentYear; year++) {
console.log(`Year: ${year} - ${calculateAge(birthYear, year)}`);
}
// Switch statement for the day of the week
let day = "Saturday";
switch(day) {
case "Monday":
console.log("Start of the week!");
break;
case "Saturday":
console.log("Weekend, time to relax!");
break;
default:
console.log("Another day, keep pushing!");
}
// Scope Example
let globalScope = "I am global";
function scopeExample() {
let localScope = "I am local";
console.log(globalScope); // Accessible
console.log(localScope); // Accessible within the function
}
scopeExample();
// console.log(localScope); // Uncaught ReferenceError: localScope is not defined
// Hoisting Example
console.log(hoistedVar); // undefined due to hoisting
var hoistedVar = "Hoisted!";
// End Output
console.log("All concepts covered!");
Explanation:
Variables: Declared using
let
,const
, andvar
.Data Types: Used numbers, strings, and boolean values.
Operators: Arithmetic operators (e.g.,
-
) and comparison operators (e.g.,>=
) are used.Control Flow:
if/else
andswitch
statements manage conditional logic.Loops: A
for
loop iterates over the years.Functions: The
calculateAge
function is a reusable block of code.Scope: The
scopeExample
function demonstrates the difference between global and local scope.Hoisting: Demonstrated with
var
variable hoisting.
This example covers all the key JavaScript concepts you've asked for and demonstrates their use in a real-world scenario.
Subscribe to my newsletter
Read articles from Polkam Srinidhi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Polkam Srinidhi
Polkam Srinidhi
I am a final year student graduates on May 2024, started my journey into practical approach of learning Tech Stacks with open source contribution and Projects.