Kotlin Keywords and Identifiers
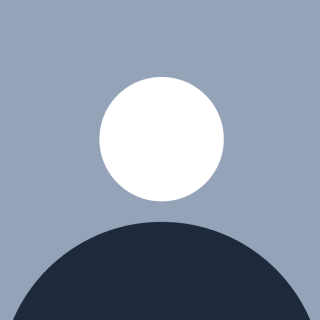
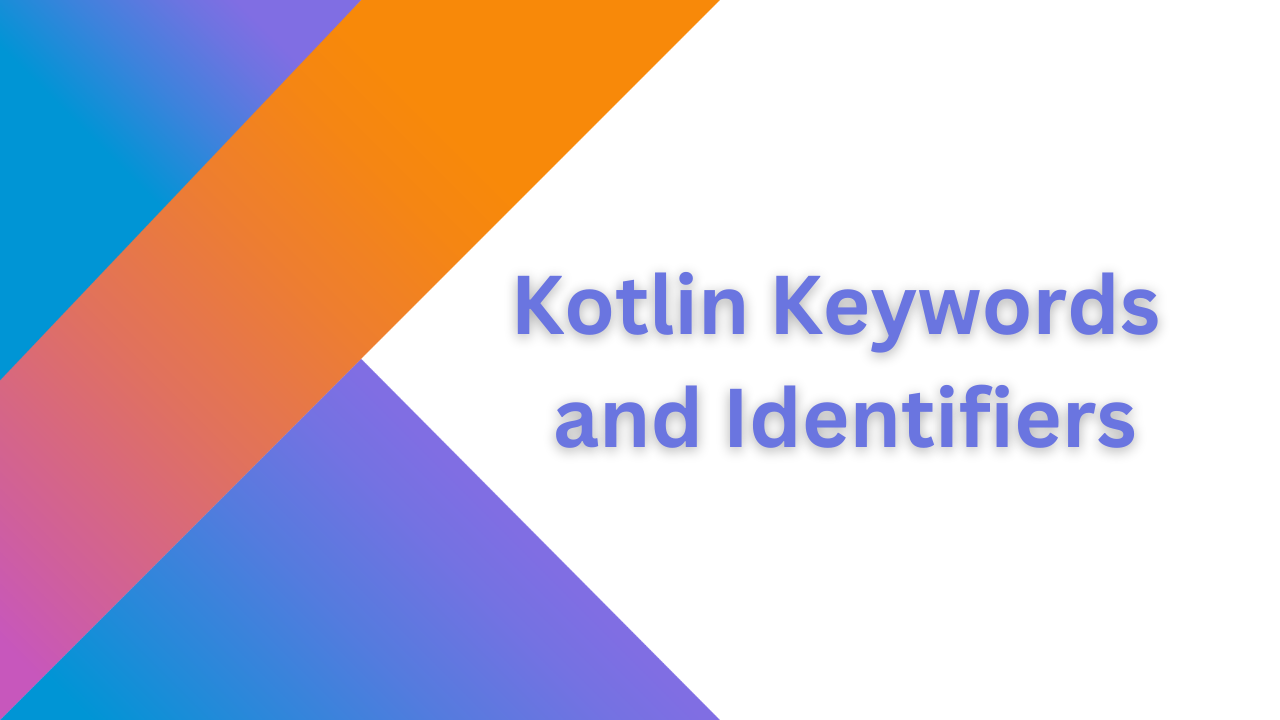
Keywords in any programming language are predefined, reserved words with specific meanings for the compiler whereas Identifiers are unique names created by programmers to represent variables, functions, classes, or other entities. In Kotlin, understanding these keywords is essential for writing clean and effective code.
1. What are Keywords in Kotlin?
Keywords are tokens that are reserved and have a special meaning in a given programming language that cannot be used as identifiers (like variable names, method names, or class names) in your code. Using a keyword as an identifier will cause compilation errors. Kotlin has a set of keywords that serve different purposes within the language.
For example, consider the following code snippet:
Here, String
is a keyword that indicates the variable name
is of string type. Attempting to use String
as a variable name would lead to a compilation error.
2. Types of Keywords in Kotlin
There are basically four types of keywords in Kotlin:
Hard keywords
Soft keywords
Modifier keyword
Special identifiers
Operators and special symbols (NOT A KEYWORD)
1. Hard Keywords
Hard keywords are strictly reserved and cannot be used as identifiers (such as variable names, function names, or class names) under any circumstances.
They have predefined meanings in the language.
Here are the 24 hard keywords in Kotlin:
Keyword | Description |
as | Used for type casts. |
as? | Used for safe type casts. |
break | Terminates the execution of a loop. |
class | Declares a class. |
continue | Proceeds to the next step of the nearest enclosing loop. |
do | Begins a do/while loop (a loop with a postcondition). |
else | Defines the branch of an if expression that is executed when the condition is false. |
false | Specifies the ‘false’ value of the Boolean type. |
for | Begins a for loop. |
fun | Declares a function. |
if | Begins an if expression. |
in | Specifies the object being iterated in a for loop. |
interface | Declares an interface. |
is | Checks that a value has a certain type. |
null | Represents an object reference that doesn’t point to any object. |
object | Declares a class and its instance at the same time. |
package | Specifies the package for the current file. |
return | Returns from the nearest enclosing function or anonymous function. |
super | Refers to the superclass implementation of a method or property. |
this | Refers to the current receiver. |
throw | Throws an exception. |
true | Specifies the ‘true’ value of the Boolean type. |
try | Begins an exception-handling block. |
typealias | Declares a type alias. |
val | Declares a read-only property or local variable. |
var | Declares a mutable property or local variable. |
when | Begins a when expression (executes one of the given branches). |
while | Begins a while loop (a loop with a precondition). |
2. Soft Keywords
Soft keywords act as keywords in specific contexts but can be used as identifiers in other situations. These tokens have dual roles.
These tokens act as keywords in specific contexts but can be used as identifiers in other contexts.
Here are 17 soft keywords in Kotlin:
Keyword | Description |
by | Delegates the implementation of an interface to another object. Also used for property delegation. |
catch | Begins a block that handles a specific exception type. |
constructor | Declares a primary or secondary constructor. |
delegate | Used as an annotation use-site target. |
dynamic | References a dynamic type in Kotlin/JS code. |
field | Used as an annotation use-site target. |
file | Used as an annotation use-site target. |
finally | Begins a block that is always executed when a try block exits. |
get | Declares the getter of a property. Also used as an annotation use-site target. |
import | Imports a declaration from another package into the current file. |
init | Begins an initializer block. |
param | Used as an annotation use-site target. |
property | Used as an annotation use-site target. |
receiver | Used as an annotation use-site target. |
set | Declares the setter of a property. Also used as an annotation use-site target. |
setparam | Used as an annotation use-site target. |
value | With the class keyword, declares an inline class. |
where | Specifies the constraints for a generic type parameter. |
3. Modifier Keywords
Modifier keywords affect the visibility, behavior, or properties of declarations. They can be used as identifiers in other contexts.
Modifier keywords appear in modifier lists of declarations. While they serve as keywords in those contexts, they can also be used as regular identifiers elsewhere.
Here’s the 29 modifier keywords in Kotlin:
Keyword | Description |
abstract | Marks a class or member as abstract. |
actual | Denotes a platform-specific implementation in multiplatform projects. |
annotation | Declares an annotation class. |
companion | Declares a companion object. |
const | Marks a property as a compile-time constant. |
crossinline | Forbids non-local returns in a lambda passed to an inline function. |
data | Instructs the compiler to generate canonical members for a class. |
enum | Declares an enumeration. |
expect | Marks a declaration as platform-specific, expecting an implementation in platform modules. |
external | Marks a declaration as implemented outside of Kotlin (accessible through JNI or in JavaScript). |
final | Forbids overriding a member. |
infix | Allows calling a function using infix notation. |
inline | Tells the compiler to inline a function and the lambdas passed to it at the call site. |
inner | Allows referring to an outer class instance from a nested class. |
internal | Marks a declaration as visible in the current module. |
lateinit | Allows initializing a non-nullable property outside of a constructor. |
noinline | Turns off inlining of a lambda passed to an inline function. |
open | Allows subclassing a class or overriding a member. |
operator | Marks a function as overloading an operator or implementing a convention. |
out | Marks a type parameter as covariant. |
override | Marks a member as an override of a superclass member. |
private | Marks a declaration as visible in the current class or file. |
protected | Marks a declaration as visible in the current class and its subclasses. |
public | Marks a declaration as visible anywhere. |
reified | Marks a type parameter of an inline function as accessible at runtime. |
sealed | Declares a sealed class (a class with restricted subclassing). |
suspend | Marks a function or lambda as suspending (usable as a coroutine). |
tailrec | Marks a function as tail-recursive (allowing the compiler to replace recursion with iteration). |
vararg | Allows passing a variable number of arguments for a parameter. |
4. Special Identifiers
- Kotlin defines two special identifiers in specific contexts. These identifiers can also be used as regular identifiers elsewhere.
Here are the special identifiers:
Keyword | Description |
field | Used inside a property accessor to refer to the backing field of the property. |
it | Used inside a lambda to refer to its parameter implicitly. |
5. Operators and special symbols
Keywords are tokens that are reserved and have a special meaning in a given programming language. Operators are keywords that can take one or more arguments. They are usually associated with the standard mathematical operations.
Operators like
new
in Java are generally considered as "Alphanumeric operator symbols" rather than keywords. Other examples aresizeof
delete
throw
instanceof
.
Keyword | Description |
+, -, *, /, % | Mathematical operators for addition, subtraction, multiplication, division, and modulo. |
* (passing to vararg) | Used to pass an array to a vararg parameter. |
\= | Assignment operator. |
?: | Elvis operator – takes the right-hand value if the left-hand value is null. |
+=, -=, *=, /=, %= | Augmented assignment operators. |
++, – | Increment and decrement operators. |
&&, | |
\==, != | Equality operators (translated to calls of equals() for non-primitive types). |
\===, !== | Referential equality operators. |
<, >, <=, >= | Comparison operators (translated to calls of compareTo() for non-primitive types). |
[, ] | Indexed access operator (translated to calls of get and set). |
!! | Asserts that an expression is non-nullable. |
?. | Performs a safe call (calls a method or accesses a property if the receiver is non-nullable). |
:: | Creates a member reference or a class reference. |
…, …< | Create ranges. |
: | Separates a name from a type in a declaration. |
? | Marks a type as nullable. |
-> | Separates the parameters and body of a lambda expression. |
@ | Introduces an annotation or references a loop label. |
$ | References a variable or expression in a string template. |
_ | Substitutes an unused parameter in a lambda expression or destructuring declaration. |
Conclusion
Keywords are reserved words with predefined meanings that cannot be used as identifiers, while identifiers are unique names created by programmers to represent various entities in the code. There are 72 keywords in Kotlin.
Keywords:
Keywords are reserved words with predefined meanings in a programming language.
They serve specific purposes and cannot be used as variable names or other identifiers.
Examples of keywords include
if
,for
, andclass
.Keywords always start with lowercase.
Identifiers:
Identifiers are unique names created by programmers to represent variables, functions, classes, or other entities.
It starts with a letter or an underscore (_). It can include letters, numbers, and underscores.
It can’t be a reserved keyword, true, false, or null**.** Whitespace is not allowed within an identifier.
Symbols like
@
or#
cannot be part of an identifier.Identifiers are case-sensitive.
The Kotlin tutorial page lists down all the important topics you can go through to get a deeper understanding of the language basics and advanced concepts.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer