Pandas for Data Analysis: A Comprehensive Guide
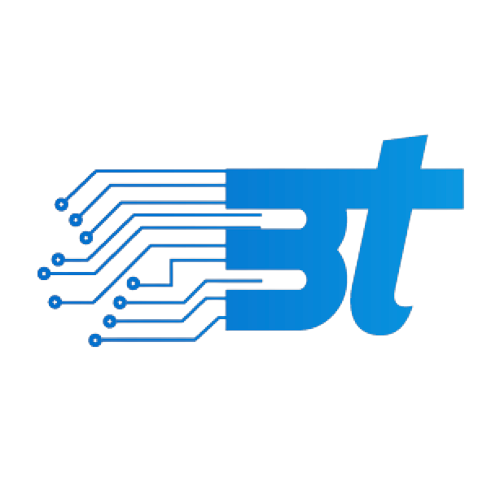
Table of contents
- 1. Introduction to Pandas
- 2. Installing Pandas
- 3. Loading Data into Pandas
- 4. Exploring DataFrames
- 5. Indexing and Selecting Data
- 6. Data Cleaning
- 7. Data Transformation
- 8. Grouping and Aggregating Data
- 9. Handling Missing Data
- 10. Merging and Joining DataFrames
- 11. Sorting Data
- 12. Visualization with Pandas
- 13. Real-World Example: Analyzing a Kaggle Dataset
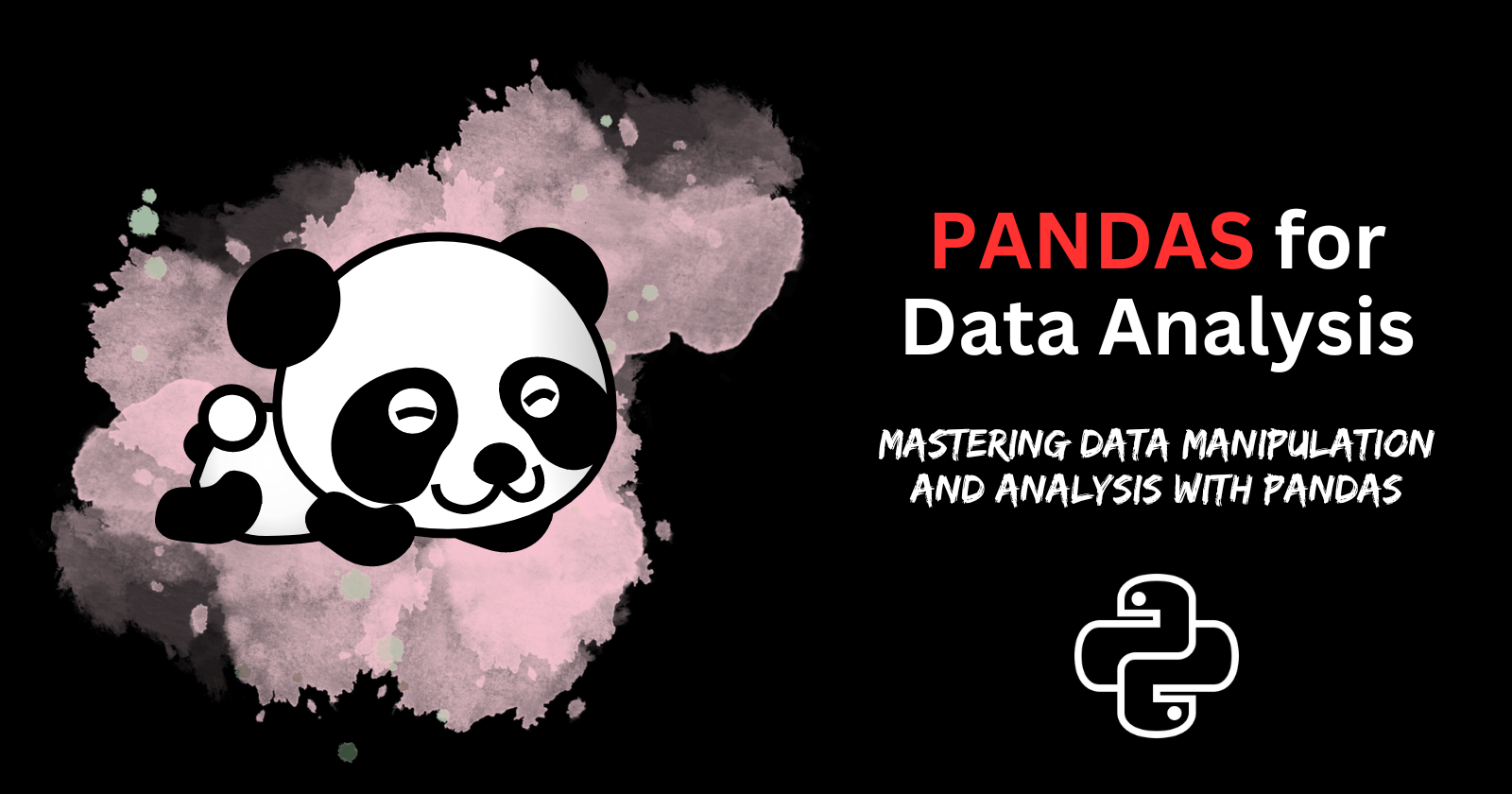
Pandas is a powerful and flexible library in Python for data manipulation and analysis. It is widely used in data science, machine learning, and statistical analysis due to its simple syntax and powerful functionalities. In this blog, we’ll dive deep into the Pandas library, covering everything from installing it, loading data, cleaning, transforming, and analyzing data efficiently. Whether you are a beginner or an experienced user, this guide will be useful to you.
1. Introduction to Pandas
Pandas provides data structures like Series
and DataFrame
for storing and manipulating data. A DataFrame
is essentially a table with rows and columns, similar to an Excel sheet, and each column is a Series
(a one-dimensional array-like object).
Pandas is built on top of NumPy, another popular Python library for numerical operations, and is designed to handle tabular data effectively.
2. Installing Pandas
Before we can start using Pandas, we need to install it. You can install it using pip
:
pip install pandas
Once installed, you can import it in your Python script:
import pandas as pd
3. Loading Data into Pandas
Pandas can load data from various sources such as CSV, Excel, JSON, SQL databases, etc. Here’s how to load a CSV file:
import pandas as pd
# Load a CSV file
df = pd.read_csv('your_file.csv')
# Load an Excel file
df = pd.read_excel('your_file.xlsx')
4. Exploring DataFrames
Once you’ve loaded your data, it's crucial to understand its structure and contents. Pandas provides several methods to do so:
# Display the first 5 rows of the DataFrame
print(df.head())
# Display the last 5 rows
print(df.tail())
# Check the shape (rows, columns)
print(df.shape)
# Get an overview of data types
print(df.info())
# Summary statistics for numerical columns
print(df.describe())
5. Indexing and Selecting Data
You can select rows and columns from the DataFrame using labels or positions. The two most common ways to do this are .loc[]
and .iloc[]
.
.loc[]
is used for label-based indexing..iloc[]
is used for position-based indexing.
# Selecting a single column
df['column_name']
# Selecting multiple columns
df[['column1', 'column2']]
# Selecting rows by index
df.loc[0:5] # Rows from index 0 to 5 (inclusive)
# Selecting specific rows and columns
df.loc[0:5, ['column1', 'column2']]
df.iloc[0:5, 0:2] # Position-based indexing
6. Data Cleaning
Data cleaning is an essential part of the data analysis process. Pandas makes it easy to clean and preprocess your data.
Renaming Columns
# Rename a single column
df.rename(columns={'old_name': 'new_name'}, inplace=True)
# Rename multiple columns
df.columns = ['new_col1', 'new_col2', 'new_col3']
Handling Duplicates
# Check for duplicate rows
df.duplicated()
# Drop duplicate rows
df.drop_duplicates(inplace=True)
7. Data Transformation
You can apply transformations on columns to modify the data:
# Apply a function to each column
df['new_column'] = df['existing_column'].apply(lambda x: x * 2)
# Apply a function to each row
df['combined_column'] = df.apply(lambda row: row['col1'] + row['col2'], axis=1)
8. Grouping and Aggregating Data
Pandas provides powerful tools for grouping and aggregating data. The groupby()
function allows you to group data by a column and apply aggregate functions like mean
, sum
, count
, etc.
# Group by a column and calculate the mean
grouped_data = df.groupby('column_name').mean()
# Group by multiple columns and apply an aggregate function
grouped_data = df.groupby(['col1', 'col2']).agg({'col3': 'sum', 'col4': 'mean'})
9. Handling Missing Data
Missing data is a common issue in real-world datasets. Pandas offers several methods to handle missing values.
Checking for Missing Values
# Check for missing values
print(df.isnull().sum())
Filling or Dropping Missing Values
# Drop rows with missing values
df.dropna(inplace=True)
# Fill missing values with a constant value
df.fillna(0, inplace=True)
# Fill missing values with the mean or median of the column
df['column_name'].fillna(df['column_name'].mean(), inplace=True)
10. Merging and Joining DataFrames
Pandas allows you to merge and join multiple DataFrames, similar to SQL joins.
# Merge two DataFrames on a common column
merged_df = pd.merge(df1, df2, on='common_column')
# Join DataFrames using an index
joined_df = df1.join(df2, on='common_column')
11. Sorting Data
Sorting data is often necessary to better understand your dataset. Pandas allows sorting by values and by index.
# Sort by column values
df.sort_values(by='column_name', ascending=False, inplace=True)
# Sort by index
df.sort_index(inplace=True)
12. Visualization with Pandas
Pandas integrates well with Matplotlib, allowing you to create basic visualizations.
import matplotlib.pyplot as plt
# Plot a histogram
df['column_name'].hist()
# Plot a line graph
df.plot(x='col1', y='col2', kind='line')
# Show the plot
plt.show()
13. Real-World Example: Analyzing a Kaggle Dataset
Let’s walk through a real-world example of loading, cleaning, and analyzing a dataset from Kaggle.
We’ll use the Titanic dataset, which can be found on Kaggle.
Step 1: Load the Data
import pandas as pd
# Load the dataset
df = pd.read_csv('titanic.csv')
# Display the first few rows
print(df.head())
Step 2: Data Cleaning
We’ll drop irrelevant columns and handle missing data.
# Drop unnecessary columns
df.drop(['PassengerId', 'Name', 'Ticket', 'Cabin'], axis=1, inplace=True)
# Fill missing values
df['Age'].fillna(df['Age'].median(), inplace=True)
df['Embarked'].fillna(df['Embarked'].mode()[0], inplace=True)
Step 3: Data Transformation
Convert categorical variables to numerical values.
# Convert categorical columns to numerical values
df = pd.get_dummies(df, columns=['Sex', 'Embarked'], drop_first=True)
Step 4: Data Analysis
Now that the data is cleaned, we can analyze it.
# Group by survival status and calculate the average age
grouped_data = df.groupby('Survived')['Age'].mean()
# Plot a histogram of ages
df['Age'].hist()
plt.show()
Conclusion
Pandas is an essential tool in any data scientist's toolkit. Practice with real-world datasets (like those on Kaggle) to become proficient, and you’ll soon find yourself navigating large datasets with ease.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
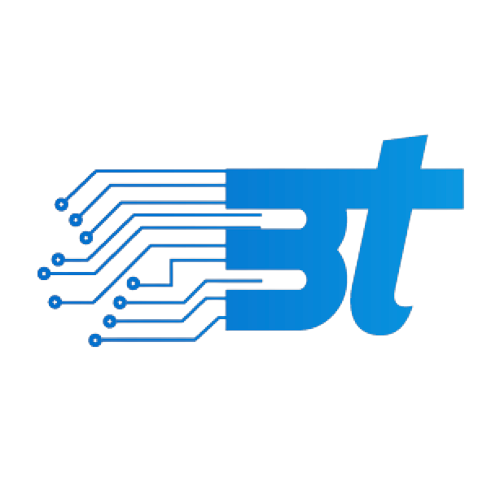
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.