Shell Scripting (PART - 1)

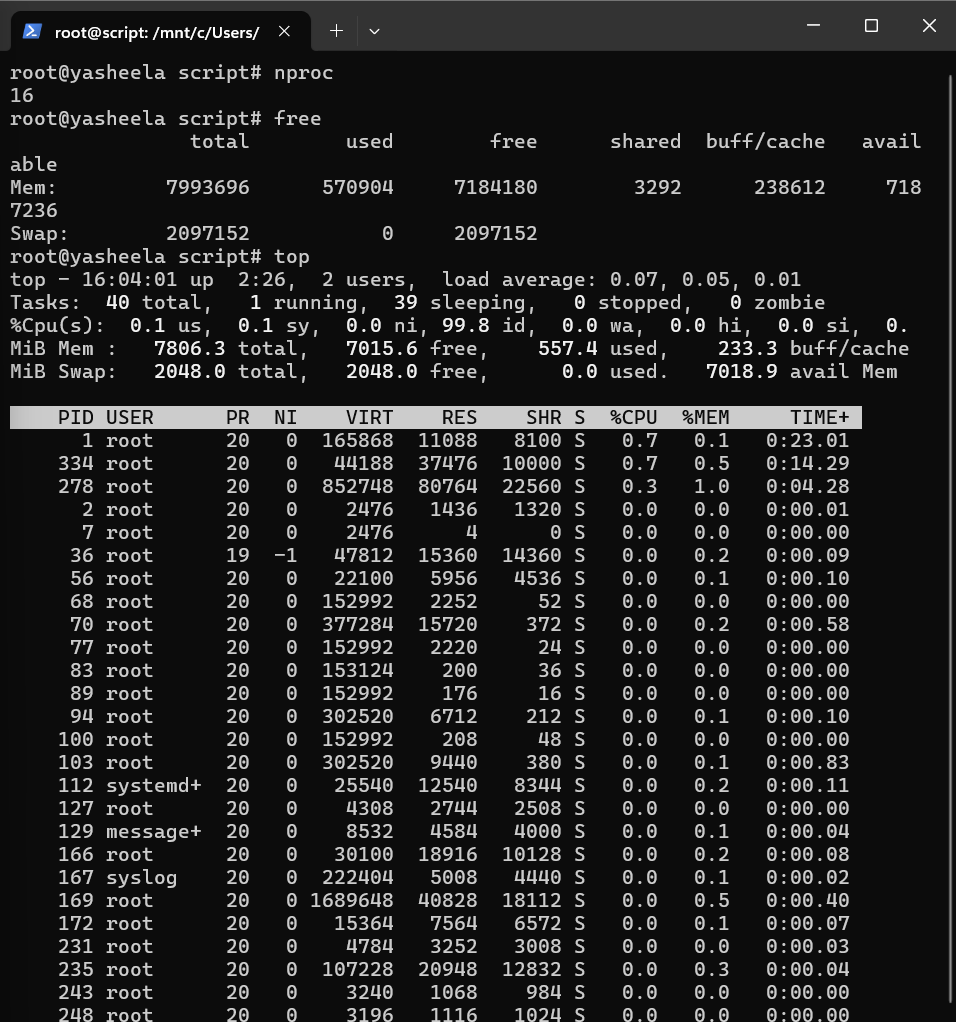
Shell scripting is a powerful way to automate tasks in Unix-like operating systems, such as Linux and macOS. By writing scripts, you can simplify complex tasks, automate repetitive actions, and even manage system configurations with just a few lines of code. In this blog post, we'll explore the basics of shell scripting in a way that's easy to understand, even if you're new to programming.
What is Shell Scripting?
A shell script is a text file that contains a sequence of commands for a Unix-based shell (like Bash, Zsh, or Sh) to execute. Shell scripts are commonly used to automate tasks that you would normally run in a terminal.
For example, if you often find yourself typing the same set of commands to set up your development environment, you can write a script to do it for you in one go.
Why Use Shell Scripting?
Automation: Automate repetitive tasks, such as backups, file management, or system monitoring.
Efficiency: Execute multiple commands in sequence without manual intervention.
Customization: Tailor your operating system to your needs by writing scripts that run at startup or when specific conditions are met.
Simplicity: Learn to script with a relatively low learning curve compared to other programming languages.
Getting Started with Shell Scripting
Let’s start with the basics. To create a shell script, all you need is a text editor (like Vim, Nano, or even a simple notepad) and a terminal.
1. Creating Your First Shell Script
Open a Terminal:
- On your Linux or macOS system, open a terminal window.
Create a Script File:
Use a text editor to create a new file. You can use
nano
,vim
, or any other text editor.For example, using
nano
:nano myscript.sh
Write Your Script:
Start by writing a simple script that prints "Hello, World!" to the terminal.
#!/bin/bash echo "Hello, World!"
The first line
#!/bin/bash
is called a "shebang" and tells the system which interpreter to use to run the script. In this case, it's the Bash shell.
Save and Exit:
- Save the file and exit the text editor. In
nano
, you can do this by pressingCTRL + O
to save andCTRL + X
to exit.
- Save the file and exit the text editor. In
Make the Script Executable:
Before you can run your script, you need to make it executable:
chmod u+x myscript.sh
Run Your Script:
Now, run your script by typing:
./myscript.sh
You should see "Hello, World!" printed in the terminal.
2. Variables and Simple Calculations
Shell scripts can use variables to store data. Let’s see how to use variables and perform simple calculations.
#!/bin/bash
# Declare a variable
name="Yasheela"
# Use the variable
echo "Hello, $name!"
# Simple arithmetic
a=5
b=3
sum=$((a + b))
echo "The sum of $a and $b is $sum."
3. Conditional Statements
Shell scripts can make decisions based on conditions, using if
statements.
#!/bin/bash
echo "Enter a number:"
read number
if [ $number -gt 10 ]; then
echo "The number is greater than 10."
else
echo "The number is 10 or less."
fi
4. Loops in Shell Scripting
Loops allow you to repeat a block of code multiple times. Here’s an example using a for
loop:
#!/bin/bash
for i in {1..5}; do
echo "Iteration $i"
done
5. Functions in Shell Scripts
Functions let you group commands into reusable blocks.
#!/bin/bash
# Define a function
greet() {
echo "Hello, $1!"
}
# Call the function
greet "Yasheela"
Real-World Use Cases for Shell Scripting
System Backups: Automate the backup of important files to another directory or remote server.
Log Rotation: Manage and rotate log files to prevent them from consuming too much disk space.
Automated Deployments: Deploy applications by running a series of commands in a specific order.
Monitoring: Write scripts to monitor system resources like CPU usage, disk space, or memory usage, and send alerts when thresholds are exceeded.
Tips for Writing Shell Scripts
Comment Your Code: Use
#
to add comments that explain what your script does. This makes your scripts easier to understand and maintain.Use Meaningful Variable Names: Choose variable names that make it clear what the variable represents.
Test Your Scripts: Run your scripts in a controlled environment before using them in production to ensure they work as expected.
Handle Errors: Check the exit status of commands using
$?
and use conditional statements to handle errors gracefully.
Conclusion
Shell scripting is an essential skill for anyone working with Unix-like systems. Whether you're automating mundane tasks or managing complex system configurations, learning to write shell scripts can save you time and effort. Start small, experiment with the examples provided, and soon you'll be writing scripts to streamline your workflows.
Happy scripting!
Subscribe to my newsletter
Read articles from Alla Yasheela directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Alla Yasheela
Alla Yasheela
I'm Yasheela, an undergraduate with a deep interest in DevOps, and cloud technologies. Currently working on exciting projects on all things DevOps. I’m passionate about simplifying complex concepts and sharing practical insights. Through my Hashnode blog, I document my learning journey, from building scalable applications to mastering cloud services, with the goal of empowering others to grow their tech skills. Let's Learn Together !!