Maven : Build Tool

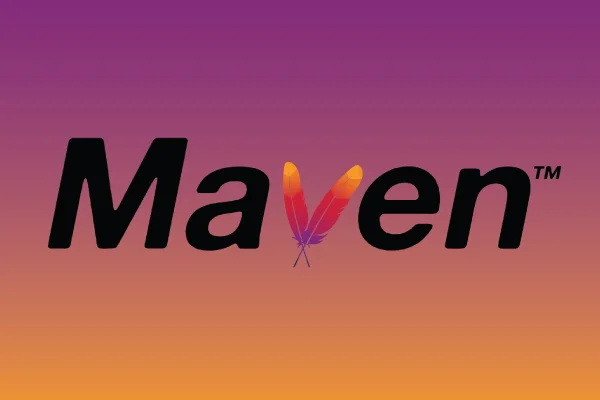
Maven is a build automation and project management tool primarily used in Java projects. It simplifies the process of managing project dependencies, builds, testing, packaging, and deployment. Maven uses an XML file called pom.xml
(Project Object Model) to manage project configurations, dependencies, plugins, and other project-related information.
Features in Maven :
Dependency Management: Maven automatically downloads and manages dependencies (libraries) for your project from a central repository (like Maven Central).
Build Automation: Maven automates the entire build process, from compiling source code to running tests, packaging the code, and deploying it.
Consistency: Using a
pom.xml
ensures that the project structure, dependencies, and configurations remain consistent across different environments.Plugin Support: Maven uses plugins for various tasks such as compiling, testing, documentation, and deployment.
Demo pom.xml file :
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.javatpoint.application1</groupId>
<artifactId>my-application1</artifactId>
<version>1.0</version>
<packaging>jar</packaging>
<name>Maven Quick Start Archetype</name>
<url>http://maven.apache.org</url>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.8.2</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
Maven Lifecycle :
validate
: Checks if the project structure is correct.compile
: Compiles the source code.test
: Runs unit tests.package
: Packages the compiled code (e.g., JAR or WAR).verify
: Checks if the code is valid and ready for release.install
: Installs the package to the local repository.deploy
: Deploys the package to a remote repository.
Let's go deep in to lifecycle steps of Maven
Validate :
deploy
When you runmvn validate
, Maven performs several checks to ensure that the project is set up correctly . Here are the key tasks involved in the validation phase:
Checks for the Presence of
pom.xml
Verifies the Correctness of
pom.xml
:<groupId>
,<artifactId>
,<version>
Dependency Version and Scope Checks
Verifies Plugin Configuration
Property Resolution
Profile Activation : Conditions for profile activation (like operating system or JDK version) are met.
Correct Directories
License and Other Metadata Checks
Compile :
Here, the raw Java source code is converted into bytecode (
.class
files), ready to be run by the Java Virtual Machine (JVM). You may also runmvn clean compile
to delete previous build artifacts (like old.class
files) before compiling again. This ensures a clean build from scratch.Test :
Compilation of Main Code (
src/main/java
).Compilation of Test Code (
src/test/java
)Test Execution : Maven uses the Surefire Plugin to run the tests.
Generating Test Reports : These reports are stored in the
target/surefire-reports
directory by default.Output : The results of the test run are displayed in the console.
Package :
Maven takes the compiled code (along with the necessary resources) and packages it into a deployable archive. Depending on the project’s
packaging
type, the result could be a:JAR (Java Archive) for standard Java applications.
WAR (Web Application Archive) for web applications.
EAR (Enterprise Archive) for enterprise applications.
This packaged file is typically placed in the
target/
directory of the project.JAR and WAR files are called archives because they bundle multiple files (code, configuration, resources) into a single compressed package.
Verify :
The
mvn verify
command in Maven is used to run integration tests and ensure that the project is fully validated and ready for release. This step is important for the projects that require additional checks or testing beyond unit tests, such as integration tests, code quality checks, or environment-specific verifications.
Difference Between
mvn test
andmvn verify
:mvn test
: Runs only unit tests using the Surefire Plugin. It focuses on testing individual components.mvn verify
: Runs integration tests (and other checks) using the Failsafe Plugin. It ensures the entire project works as expected in a more complete environment.
Install :
The
mvn install
command in Maven is used to build the project and install the resulting artifact (such as a JAR or WAR file) into the local Maven repository. This allows other Maven projects on the same machine to reference and use this artifact as a dependency without the need to rebuild it every time.Deploy :
The
mvn deploy
command in Maven is used to build the project and deploy the final packaged artifact (such as a JAR, WAR, or EAR file) to a remote repository, making it available for other developers and projects to use. It is typically the last step in the Maven build lifecycle and is used when you're ready to share your project with others outside your local environment.Typical Use Cases for
mvn deploy
:Publishing Artifacts:
- When you have completed the development and testing of your project and are ready to share it with others, you use
mvn deploy
to publish your project to a remote repository like Nexus or Maven Central.
- When you have completed the development and testing of your project and are ready to share it with others, you use
Team Collaboration:
- In large-scale projects, developers can deploy their artifacts to a shared repository so other teams can easily access and use them as dependencies without having to build the artifacts locally.
Continuous Integration (CI/CD):
mvn deploy
is commonly used in CI/CD pipelines. Once a build passes all tests, the CI/CD system like Jenkins or GitLab CI can runmvn deploy
to automatically push the build artifacts to a remote repository for production use or further testing.
Overall, Maven streamlines the build process, enforces consistency, and
facilitates project management across different environments and teams.
Subscribe to my newsletter
Read articles from saurabh dave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
