Understanding Constructors in Java
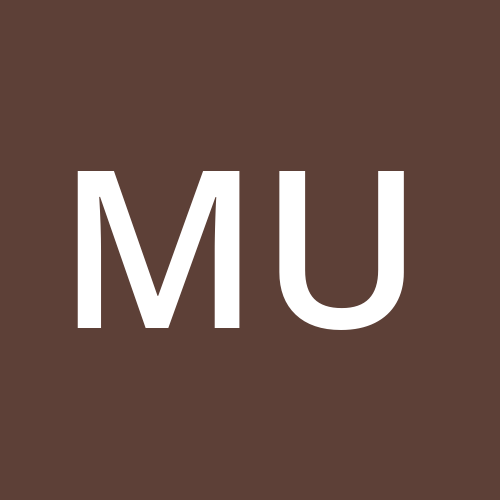
Table of contents
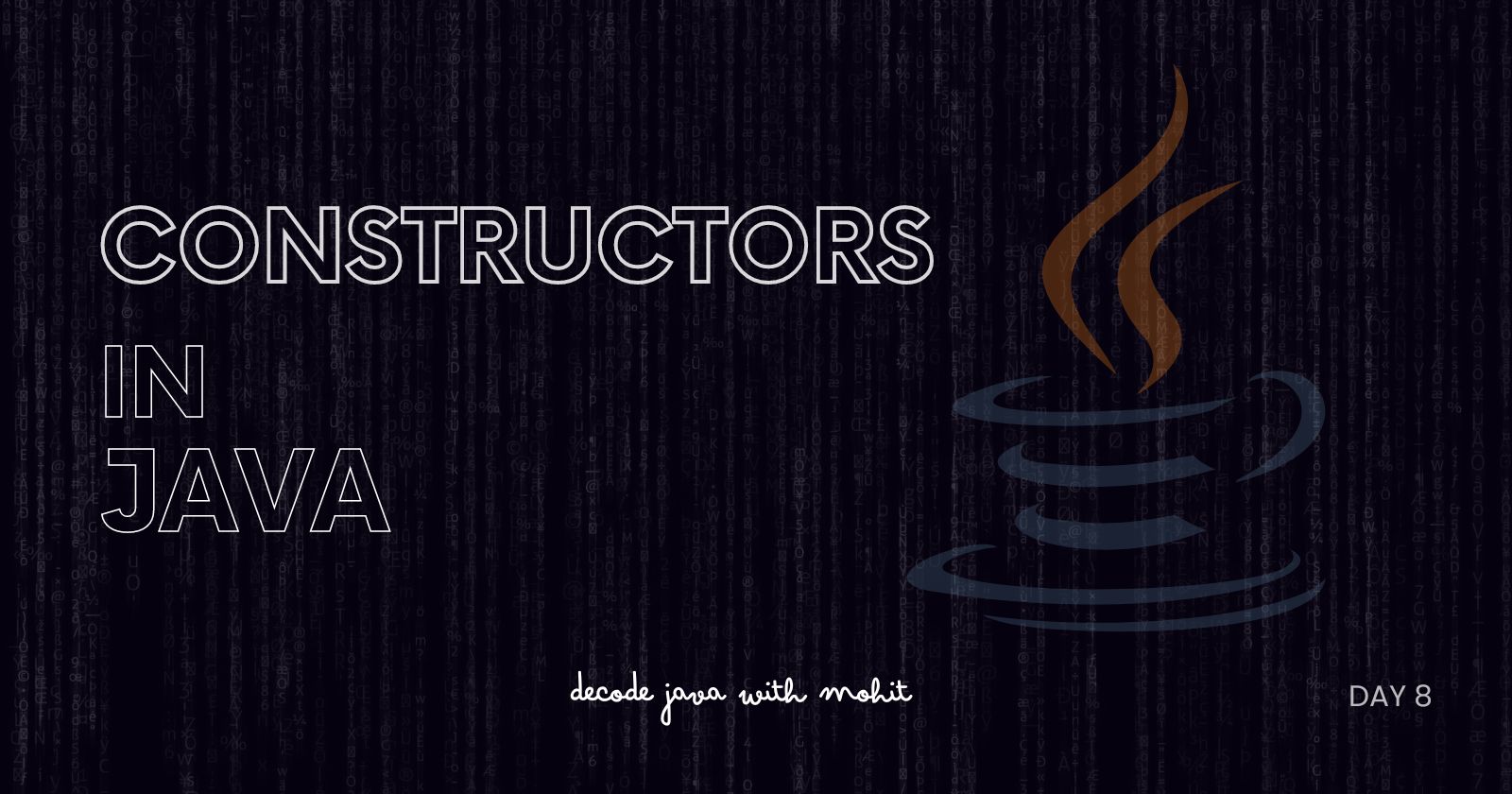
On Day 8, the focus will be on understanding constructors in Java. Constructors play a vital role in initializing objects . This lesson will cover the basics of constructors, different types, and how they are used in Object-Oriented Programming (OOPs) to enhance code functionality.
1. What is a Constructor?
A constructor is a special method in Java that is used to initialize objects. It is called automatically when an object of a class is created. Constructors have the same name as the class and do not have a return type, not even void
.
The primary function of a constructor is to set the initial values for the attributes of the class and perform necessary setup when creating an object.
2. Characteristics of Constructors
Same name as the class: The name of the constructor must match the class name exactly.
No return type: Unlike regular methods, constructors do not have a return type.
Automatically called: A constructor is called automatically when an object is instantiated using the
new
keyword.Overloading: Constructors can be overloaded, meaning a class can have multiple constructors with different parameter lists.
3. Types of Constructors
Java provides two main types of constructors:
1. Default Constructor
A default constructor is a no-argument constructor that Java provides automatically if no constructor is defined in a class.
It simply initializes the object without setting any specific values, often initializing member variables to default values like
null
,0
, etc.
Example of a Default Constructor:
public class Car {
String model;
int year;
// Default constructor (no parameters)
public Car() {
System.out.println("Default constructor called");
model = "Unknown"; // Default value
year = 2020; // Default value
}
public void displayInfo() {
System.out.println("Model: " + model + ", Year: " + year);
}
}
public class Main {
public static void main(String[] args) {
Car car1 = new Car(); // Calls the default constructor
car1.displayInfo(); // Output: Model: Unknown, Year: 2020
}
}
Explanation:
In the above example, the
Car
class has a default constructor that initializes themodel
andyear
to default values.When we create an object using
new Car()
, the default constructor is called, and themodel
andyear
attributes are initialized to "Unknown" and 2020, respectively.
2. Parameterized Constructor
A parameterized constructor allows you to pass arguments to the constructor to initialize the object with specific values.
It is used when you want to assign custom values to the object’s attributes at the time of object creation.
Example of a Parameterized Constructor:
public class Car {
String model;
int year;
// Parameterized constructor
public Car(String model, int year) {
this.model = model;
this.year = year;
}
public void displayInfo() {
System.out.println("Model: " + model + ", Year: " + year);
}
}
public class Main {
public static void main(String[] args) {
Car car1 = new Car("Tesla", 2023); // Calls parameterized constructor
car1.displayInfo(); // Output: Model: Tesla, Year: 2023
}
}
Explanation:
The parameterized constructor takes two parameters:
model
andyear
.When creating a
Car
object, specific values like "Tesla" and 2023 are passed, which are then assigned to the object's attributes.This allows objects to be initialized with custom values.
4. Constructor Overloading
In Java, constructors can be overloaded, which means you can have multiple constructors in a class, each with a different number or type of parameters. The appropriate constructor is called based on the number and type of arguments passed during object creation.
Example of Constructor Overloading:
public class Car {
String model;
int year;
// Default constructor
public Car() {
model = "Unknown";
year = 2020;
}
// Parameterized constructor
public Car(String model) {
this.model = model;
year = 2020;
}
// Another parameterized constructor
public Car(String model, int year) {
this.model = model;
this.year = year;
}
public void displayInfo() {
System.out.println("Model: " + model + ", Year: " + year);
}
}
public class Main {
public static void main(String[] args) {
Car car1 = new Car(); // Calls default constructor
Car car2 = new Car("Tesla"); // Calls constructor with 1 parameter
Car car3 = new Car("Audi", 2023); // Calls constructor with 2 parameters
car1.displayInfo(); // Output: Model: Unknown, Year: 2020
car2.displayInfo(); // Output: Model: Tesla, Year: 2020
car3.displayInfo(); // Output: Model: Audi, Year: 2023
}
}
Explanation:
Three constructors are defined in the
Car
class: one default and two parameterized constructors.Based on how the object is created, Java determines which constructor to invoke.
This provides flexibility to initialize objects in different ways based on the available information.
5. this
Keyword in Constructors
The this
keyword refers to the current object in a method or constructor. It is often used in constructors to differentiate between class attributes and constructor parameters when they share the same name.
Example Using this
:
public class Car {
String model;
int year;
// Constructor with parameters
public Car(String model, int year) {
this.model = model; // 'this.model' refers to the instance variable
this.year = year; // 'this.year' refers to the instance variable
}
public void displayInfo() {
System.out.println("Model: " + model + ", Year: " + year);
}
}
public class Main {
public static void main(String[] args) {
Car car = new Car("BMW", 2022);
car.displayInfo(); // Output: Model: BMW, Year: 2022
}
}
Explanation:
The
this
keyword is used to refer to the instance variablesmodel
andyear
of the current object.This ensures there is no ambiguity between the instance variables and the parameters of the constructor.
6. Chaining Constructors using this()
Java allows you to call one constructor from another within the same class using the this()
syntax. This is called constructor chaining and is useful for reusing constructor logic without duplication.
Example of Constructor Chaining:
public class Car {
String model;
int year;
// Default constructor
public Car() {
this("Unknown", 2020); // Calls parameterized constructor
}
// Parameterized constructor
public Car(String model, int year) {
this.model = model;
this.year = year;
}
public void displayInfo() {
System.out.println("Model: " + model + ", Year: " + year);
}
}
public class Main {
public static void main(String[] args) {
Car car1 = new Car(); // Calls default constructor
Car car2 = new Car("Mercedes", 2022); // Calls parameterized constructor
car1.displayInfo(); // Output: Model: Unknown, Year: 2020
car2.displayInfo(); // Output: Model: Mercedes, Year: 2022
}
}
Explanation:
The default constructor calls the parameterized constructor using
this()
, passing default values.This allows the initialization logic of the parameterized constructor to be reused, reducing code duplication.
7. Copy Constructor
A copy constructor is used to create a new object by copying the attributes of another object. Java doesn’t provide a default copy constructor, but you can create one manually.
Example of Copy Constructor:
public class Car {
String model;
int year;
// Parameterized constructor
public Car(String model, int year) {
this.model = model;
this.year = year;
}
// Copy constructor
public Car(Car car) {
this.model = car.model;
this.year = car.year;
}
public void displayInfo() {
System.out.println("Model: " + model + ", Year: " + year);
}
}
public class Main {
public static void main(String[] args) {
Car car1 = new Car("Tesla", 2023);
Car car2 = new Car(car1); // Calls the copy constructor
car2.displayInfo(); // Output: Model: Tesla, Year: 2023
}
}
Explanation:
The copy constructor takes another
Car
object as an argument and copies itsmodel
andyear
attributes to the new object.This is useful when you need to create a duplicate object.
8. Best Practices for Using Constructors
Ensure proper initialization: Always use constructors to properly initialize an object's fields.
Use constructor overloading wisely: Provide constructors with different parameter lists for flexibility, but avoid too many overloaded constructors, which can lead to confusion.
Avoid heavy logic in constructors: Constructors should focus on object initialization, not performing complex business logic.
Use copy constructors for immutability: When creating immutable objects, use copy constructors to safely clone an object.
Summary
By the end of Day 8, we will have learned:
The purpose of constructors and how they work.
The difference between default and parameterized constructors.
How to overload constructors and use the
this
keyword.How to chain constructors for code reuse.
How to implement copy constructors for cloning objects.
These concepts are fundamental for understanding object initialization in Java and provide a solid foundation for further exploration into Object-Oriented Programming. So Stay tuned!!
Subscribe to my newsletter
Read articles from Mohit Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
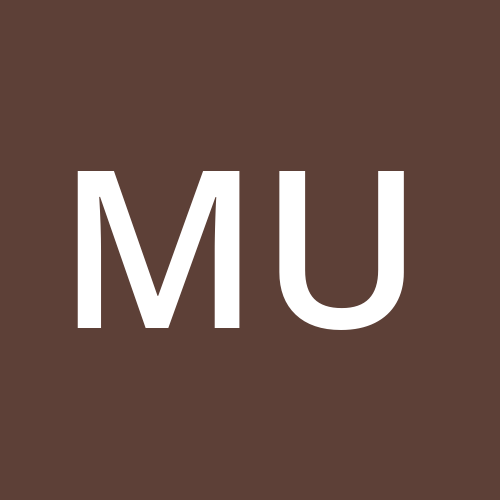