Mastering the Art of React Component Libraries
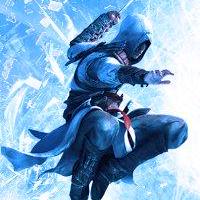
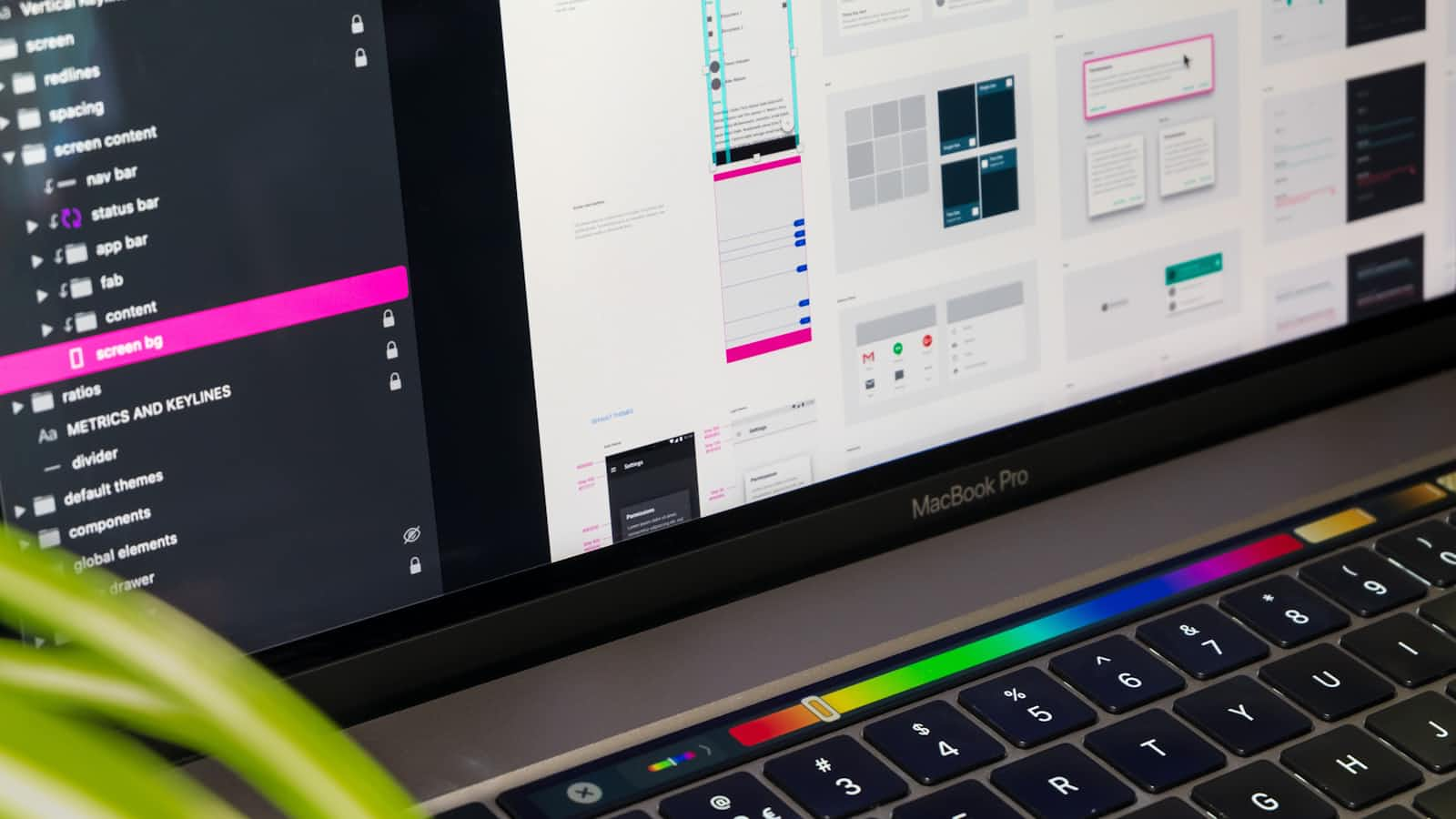
As a frontend developer, Today, I'm excited to share insights on building a React component library that not only meets current needs but scales gracefully with your project. We'll explore key decisions that enhance scalability, maintainability, and developer experience.
Harnessing the Power of Scoped Styling
When building a component library, one of the most impactful decisions you can make is how to handle styling. We've found that combining twin.macro and Styled Components offers a robust solution that brings several advantages:
Elimination of CSS leakage: By using CSS-in-JS via Styled Components, we ensure that styles remain encapsulated within their respective components. This prevents unintended side effects and makes our components more predictable and easier to reason about.
Rapid development with utility classes: twin.macro allows us to leverage Tailwind's utility classes directly in our JavaScript. This speeds up development while maintaining the benefits of scoped styles.
Type safety: Both tools work seamlessly with TypeScript, providing excellent type checking and autocompletion.
Performance: Styled Components' ability to critical CSS extraction helps optimize our application's loading times.
Here's a simple example of how we use twin.macro with Styled Components:
import tw, {styled} from 'twin.macro';
// Using twin.macro for inline styles
const Button = tw.button`
bg-blue-500 text-white px-4 py-2 rounded
hover:bg-blue-600
`;
// Combining twin.macro with Styled Components
const Card = styled.div`
${tw`bg-white rounded-lg shadow-md p-6`}
max-width: 300px;
`;
// Usage
const Example = () => (
<Card>
<h2 tw="text-xl font-bold">Card Title</h2>
<p tw="text-gray-600">Card content goes here.</p>
<Button>Click me</Button>
</Card>
);
By adopting this approach, we've eliminated the headaches associated with global CSS conflicts and simplified our styling workflow.
Architecting for Complexity: Atomic Design and Compound Components
As component libraries grow, managing complexity becomes a key concern. We've found great success in adopting two complementary patterns: Atomic Design and Compound Components.
Atomic Design provides a mental model for breaking down complex UIs into smaller, more manageable pieces. By thinking in terms of atoms, molecules, and organisms, we create a more modular and scalable system. This approach offers several benefits:
- Improved reusability: Smaller, atomic components are easier to reuse across different contexts.
- Easier maintenance: When changes are needed, they can often be made at the atomic level, reducing the risk of unintended side effects.
- Better collaboration: The clear hierarchy makes it easier for designers and developers to communicate and work together.
Compound Components, on the other hand, allow us to compose complex components from smaller parts while encapsulating the overall component's logic. This pattern:
- Enhances flexibility: Consumers of our library can easily customize the composition of complex components.
- Improves readability: The relationship between parent and child components becomes more explicit.
- Facilitates testing: Smaller, focused components are generally easier to test in isolation.
Here's a basic example of how we implement Compound Components:
import React, {useState} from 'react';
// Parent component that manages the state
const Accordion = ({children}) => {
const [isOpen, setIsOpen] = useState(false);
// Function to toggle open/close state
const toggleAccordion = () => {
setIsOpen(!isOpen);
};
// Clone children to pass down props (isOpen and toggleAccordion)
return React.Children.map(children, (child) => React.cloneElement(child, {isOpen, toggleAccordion}));
};
// Toggle button for Accordion
Accordion.Toggle = ({toggleAccordion, children}) => {
return <button onClick={toggleAccordion}>{children}</button>;
};
// Content of Accordion
Accordion.Content = ({isOpen, children}) => {
return isOpen ? <div>{children}</div> : null;
};
// Example usage of the Accordion component
const App = () => {
return (
<Accordion>
<Accordion.Toggle>Click to Toggle</Accordion.Toggle>
<Accordion.Content>This is the content</Accordion.Content>
</Accordion>
);
};
export default App;
By combining these patterns, we've created a component library that's both flexible and maintainable, even as it grows in complexity.
Ensuring Consistency with a Centralized Theme
A cohesive design system relies on consistency. That's why we've implemented a Theme Provider as a single source of truth for our design tokens. This decision brings several advantages:
- Centralized configuration: All design tokens - colors, sizes, fonts, etc. - are defined in one place, making updates and maintenance straightforward.
- Easy theming: Switching between different themes (e.g., light and dark mode) becomes a matter of swapping out the theme object.
- Type safety: By leveraging TypeScript, we ensure that our theme is used correctly throughout the application.
- Performance: With all theme values readily available via React's Context API, we avoid unnecessary prop drilling.
This approach has eliminated inconsistencies in our design implementation and streamlined our theming process.
Elevating Developer Experience with Polymorphic Components
As our library grew, we recognized the need for more flexible component APIs. Our solution was to implement strongly-typed Polymorphic Components using TypeScript. This pattern offers several key benefits:
- Improved type safety: TypeScript ensures that the correct props are used for each component variant.
- Enhanced flexibility: Components can adapt their props based on the specific variant being used.
- Better developer experience: Autocomplete and type checking make it easier for developers to use our components correctly.
- Reduced boilerplate: We can handle multiple variants within a single component definition, reducing code duplication.
import React from 'react';
interface ButtonProps extends React.ComponentPropsWithoutRef<'button'> {
as?: React.ElementType;
children: React.ReactNode;
}
const Button = React.forwardRef<HTMLElement, ButtonProps>(({as: Component = 'button', children, ...props}, ref) => {
return (
<Component ref={ref} {...props}>
{children}
</Component>
);
});
// Usage
const Example = () => (
<>
<Button>Default Button</Button>
<Button as="a" href="https://example.com">
Link Button
</Button>
<Button as="div" role="button">
Div Button
</Button>
</>
);
export default Example;
This approach has significantly improved the usability of our component library, making it more intuitive and less error-prone for developers.
Streamlining the Build Process
In the interest of simplicity and consistency, we decided to use Vite for our entire build process. This choice has paid dividends in several ways:
- Faster build times: Vite's lightning-fast cold starts and hot module replacement have significantly sped up our development workflow.
- Simplified configuration: By using a single build tool for both our component library and Storybook, we've reduced complexity in our setup.
- Better performance: Vite's out-of-the-box optimizations help ensure our library is as performant as possible.
- Future-proofing: Vite's modern architecture positions us well for future web development trends.
This has eliminated the inconsistencies and maintenance overhead associated with using multiple build tools.
Enforcing Quality with Git Hooks and Sonarqube
To maintain high code quality and smooth collaboration, we've implemented a robust CI/CD pipeline that combines Git hooks using Husky and Sonarqube for static code review. This proactive approach offers several advantages:
Consistent commit messages: By enforcing Commitizen standards through a pre-commit hook, we ensure that our commit history is clear and useful.
Prevention of broken builds: Our pre-push hook checks that both the component library and Storybook build successfully before allowing pushes.
Faster code reviews: With consistent commit messages and guaranteed buildable code, the review process becomes more efficient.
Reduced CI/CD failures: By catching issues before they reach the CI/CD pipeline, we save time and computational resources.
Continuous visual regression testing: We've integrated Sonarqube into our CI/CD pipeline, which provides several key benefits:
- Automated visual comparisons between different versions of our components
- Early detection of unintended visual changes
- A visual history of our component evolution, aiding in debugging and decision-making
- Improved collaboration between designers and developers by providing a shared visual reference
The integration of Sonarqube with our existing Git hooks has created a powerful quality assurance system. Here's a brief overview of our workflow:
- Developers make changes and commit them, adhering to Commitizen standards (enforced by the pre-commit hook).
- Before pushing, the pre-push hook ensures all builds are successful.
- Once pushed, our CI/CD pipeline triggers Sonarqube to perform static code review.
- Reviewers can easily see diffs in the Sonarqube interface, alongside the code changes in the pull request.
This comprehensive approach to quality assurance has significantly improved our development workflow, code quality, and the overall consistency of our component library. By catching both code and visual issues early, we've reduced the time spent on bug fixes and increased our confidence in each release.
Conclusion
Building a robust React component library is a journey of continuous improvement. By making thoughtful decisions around styling, architecture, theming, component APIs, build processes, and quality enforcement, we've created a system that's scalable, maintainable, and a joy to work with. Remember, the key is to regularly reassess your needs and be willing to adapt your approach as your library evolves.
Happy coding!
Resources
Subscribe to my newsletter
Read articles from Mohammad Massoud Salem directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
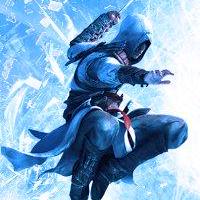
Mohammad Massoud Salem
Mohammad Massoud Salem
My goal is to create inspiring web solutions through thoughtful code and user-centered design that enhance the user experience.