Optimizing SQL Queries for Better Performance
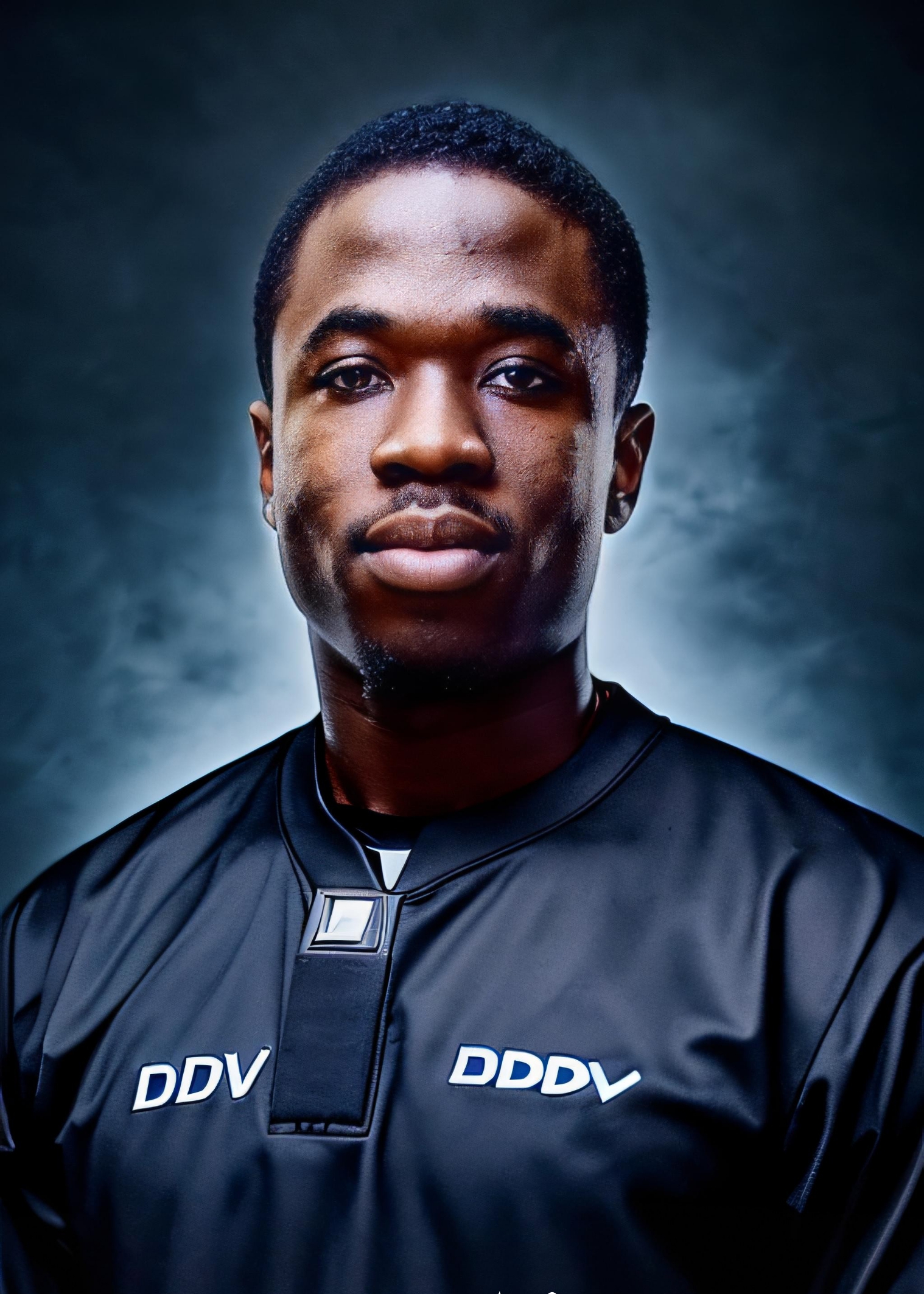
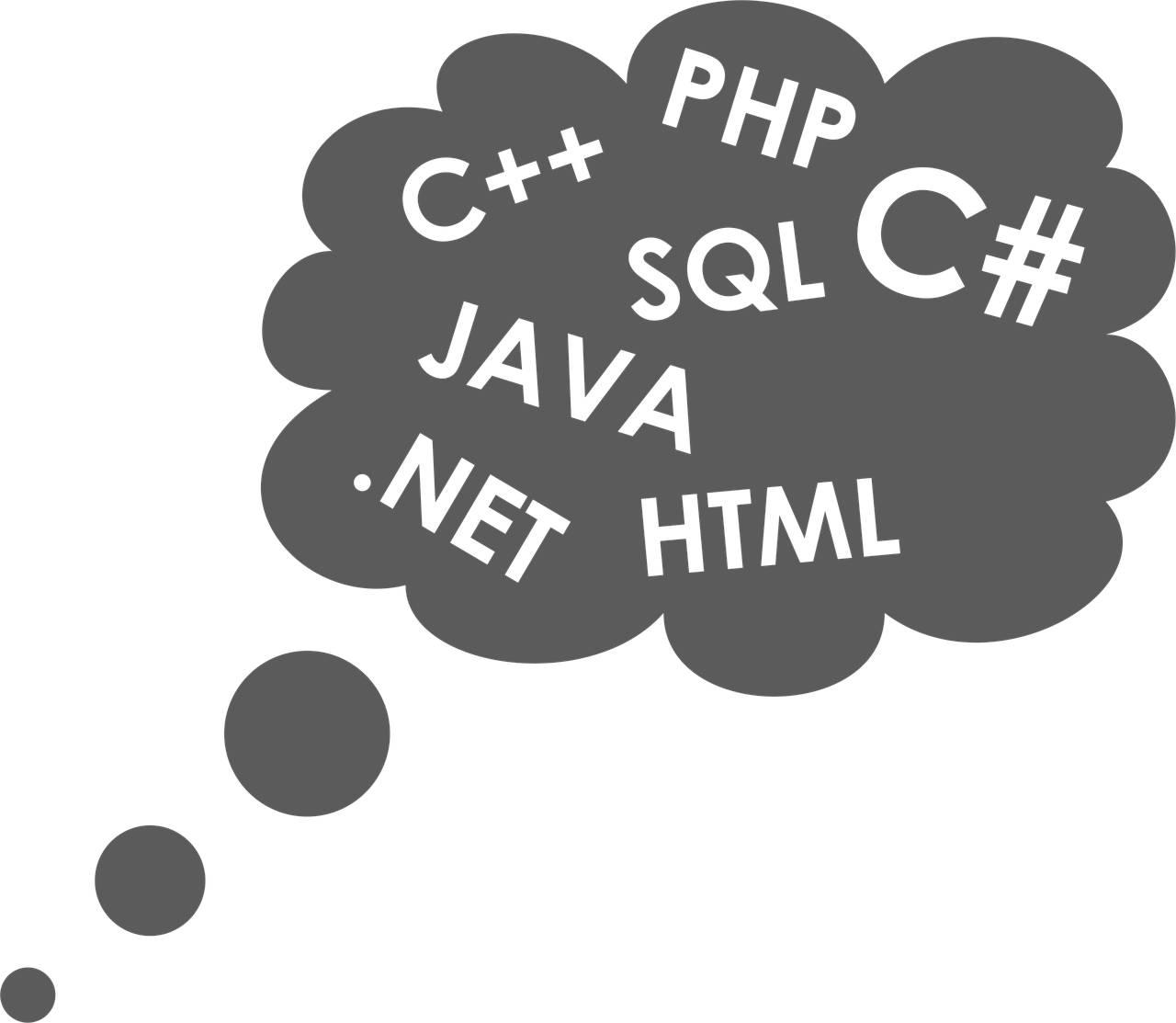
SQL (Structured Query Language) is the standard language used for interacting with relational databases. Whether you are dealing with small-scale databases or enterprise-level systems, query optimization is critical to ensure performance efficiency. Inefficient queries can lead to slow response times, higher resource consumption, and degraded performance, especially as databases grow in size.
This article provides a comprehensive guide to optimizing SQL queries, covering fundamental techniques that can make your queries more efficient and boost the overall performance of your database.
Understand the Execution Plan
Before diving into optimization techniques, understanding how SQL queries are executed is essential. The database query optimizer determines the most efficient way to execute a query by generating an execution plan. This plan provides valuable insights into how your query will access data and what operations (like joins, filters, or sorts) will be performed.
Steps to view the execution plan:
In MySQL: Use
EXPLAIN SELECT * FROM your_table;
In PostgreSQL: Use
EXPLAIN ANALYZE SELECT * FROM your_table;
Key components of an execution plan:
Table access methods: Full table scans vs. index scans.
Join methods: Nested loop joins, hash joins, or merge joins.
Sorting and filtering: Operations such as GROUP BY or WHERE filters.
Optimizing queries based on execution plans is critical. If you notice full table scans or inefficient joins, the query likely needs tuning.
Indexing
Indexes are one of the most powerful tools for speeding up query performance. Indexes allow the database to quickly locate the required rows without scanning the entire table.
Best practices for using indexes:
Use indexes on columns in WHERE clauses: If your query filters based on certain columns, applying indexes on those columns can drastically reduce search time.
CREATE INDEX idx_column_name ON table_name(column_name);
Create composite indexes for multiple columns: If you are querying multiple columns together (e.g., in WHERE or JOIN conditions), consider a composite index.
CREATE INDEX idx_composite ON table_name(column1, column2);
Beware of over-indexing: While indexes are powerful, creating too many indexes can lead to performance issues, especially during
INSERT
,UPDATE
, andDELETE
operations. Choose indexes wisely based on your query patterns.
Optimize WHERE Clauses
The WHERE
clause is often the most critical part of query optimization. An inefficient WHERE
clause can lead to unnecessary table scans and slow performance.
Techniques to optimize WHERE
clauses:
Use indexed columns: Always try to filter based on indexed columns to speed up data retrieval.
Avoid
SELECT *
: Only select the columns you need. Selecting all columns (SELECT *
) can slow down performance, especially when you're fetching data over the network.SELECT column1, column2 FROM table_name WHERE condition;
Avoid functions on columns: Functions like
UPPER()
,LOWER()
, orSUBSTRING()
can negate the use of indexes.Inefficient query:
SELECT * FROM users WHERE UPPER(username) = 'JOHN';
Optimized query:
SELECT * FROM users WHERE username = 'john';
Leverage IN and EXISTS appropriately: If you are checking for values in another table, consider using
EXISTS
instead ofIN
, especially when dealing with large datasets.Inefficient query:
SELECT * FROM users WHERE id IN (SELECT user_id FROM orders);
Optimized query:
SELECT * FROM users WHERE EXISTS (SELECT 1 FROM orders WHERE user_id = users.id);
Optimize Joins
Joins are a common cause of slow SQL queries, especially in complex queries involving multiple tables. Optimizing joins can make a significant difference in query performance.
Best practices for optimizing joins:
Use indexed columns in JOIN conditions: Always ensure that the columns being joined are indexed. Indexing the join keys can drastically reduce the time it takes to match rows from different tables.
SELECT * FROM orders JOIN customers ON orders.customer_id = customers.id;
Use INNER JOIN over OUTER JOIN: Where possible, use
INNER JOIN
instead ofLEFT/RIGHT OUTER JOIN
as it reduces the result set size by only returning matched rows.Consider the order of joins: The order in which you join tables can impact performance. Place the smaller tables first in the join sequence to reduce the number of rows processed.
Avoid Cartesian products: Be careful to specify proper join conditions. A missing
ON
clause can lead to Cartesian products, where each row from one table is paired with every row from another, leading to a massive number of unnecessary rows.
Limit the Use of Subqueries
Subqueries (queries inside other queries) can be tempting to use but may significantly degrade performance, especially if they are uncorrelated or repeated multiple times.
Optimize subqueries with joins: Instead of relying on subqueries, try using joins to pull the required data more efficiently.
Inefficient query:
SELECT name FROM employees WHERE department_id IN (SELECT id FROM departments WHERE name = 'Engineering');
Optimized query:
SELECT employees.name
FROM employees
JOIN departments ON employees.department_id = departments.id
WHERE departments.name = 'Engineering';
Use LIMIT to Restrict Result Sets
In scenarios where you only need a subset of data, use the LIMIT
clause to restrict the number of rows returned by the query.
SELECT * FROM orders ORDER BY order_date DESC LIMIT 10;
By limiting the result set, you reduce the amount of data processed and transferred, which is especially important in large datasets.
Avoid Wildcard Searches
Using wildcards (especially leading wildcards) in LIKE
queries can prevent the use of indexes and force full table scans.
Inefficient query:
SELECT * FROM products WHERE name LIKE '%phone';
In this case, the %
before the search term forces the database to scan every row. Instead, consider designing your queries or indexing strategies to avoid leading wildcards.
Partitioning Large Tables
For very large tables, partitioning can be a powerful optimization technique. Partitioning splits a large table into smaller, more manageable chunks, allowing the database to scan only relevant partitions rather than the entire table.
Types of partitioning:
Range Partitioning: Splitting data based on ranges of values, such as date ranges.
Hash Partitioning: Distributing data based on a hash function applied to a key.
Use Caching
Sometimes, the best way to optimize a query is to avoid running it altogether. SQL query caching can save previously run query results, speeding up future executions of the same query.
Types of caching:
Application-level caching: Store query results in memory within your application.
Database-level caching: Many databases, like MySQL with its Query Cache, have built-in support for query result caching.
Conclusion
Optimizing SQL queries is crucial for improving the performance of any relational database. By understanding execution plans, using appropriate indexes, writing efficient WHERE
clauses, and minimizing the complexity of joins and subqueries, you can significantly reduce query execution times. Always approach optimization with a combination of best practices and thorough testing to ensure that changes have the desired effect on performance.
Whether you're managing a small database or a complex enterprise system, applying these optimization techniques will result in faster, more scalable applications.
Subscribe to my newsletter
Read articles from Victor Uzoagba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
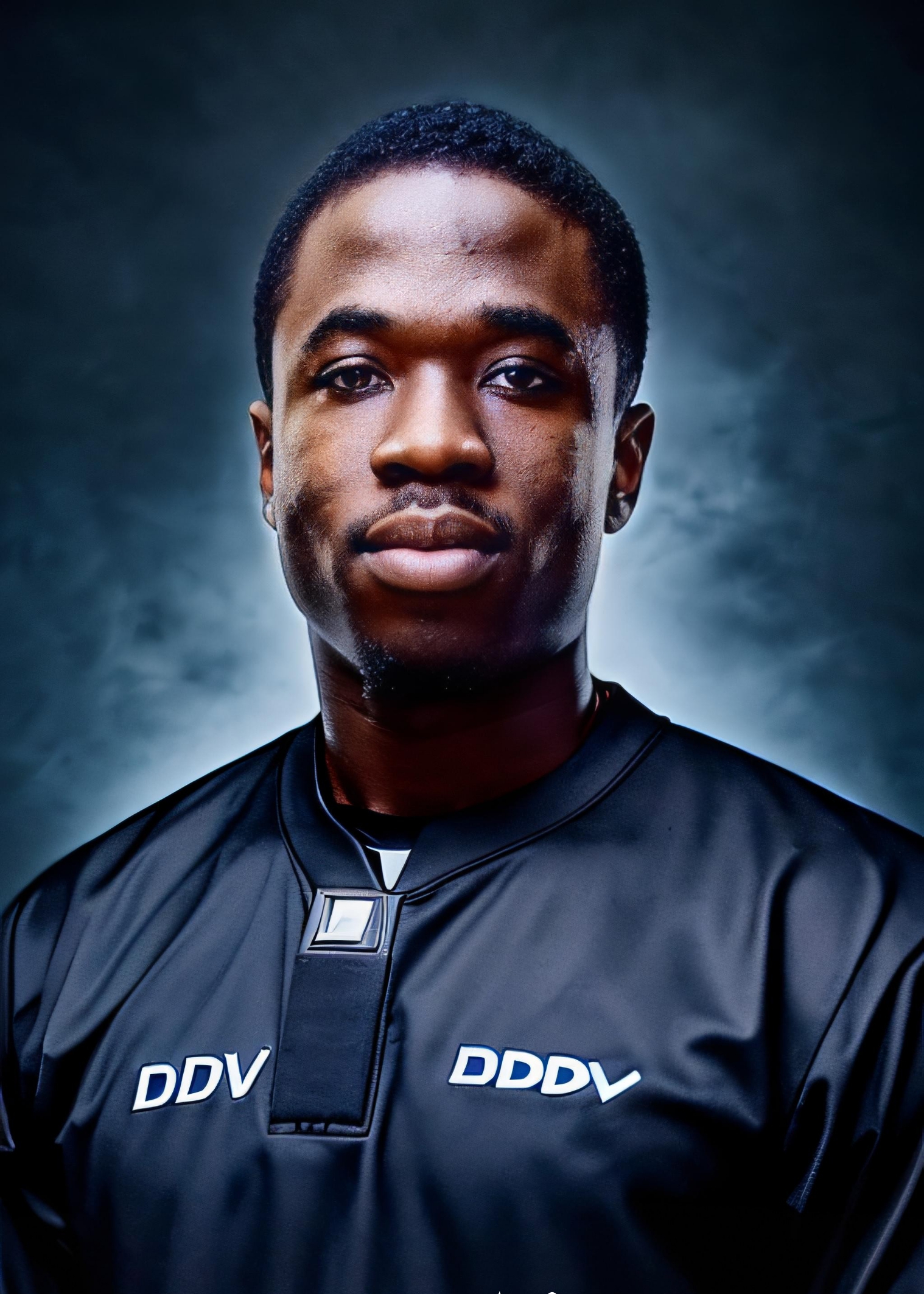
Victor Uzoagba
Victor Uzoagba
I'm a seasoned technical writer specializing in Python programming. With a keen understanding of both the technical and creative aspects of technology, I write compelling and informative content that bridges the gap between complex programming concepts and readers of all levels. Passionate about coding and communication, I deliver insightful articles, tutorials, and documentation that empower developers to harness the full potential of technology.