Mastering React Print: A Comprehensive Guide
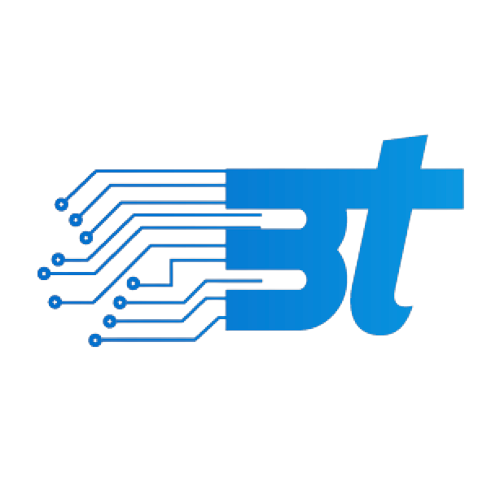
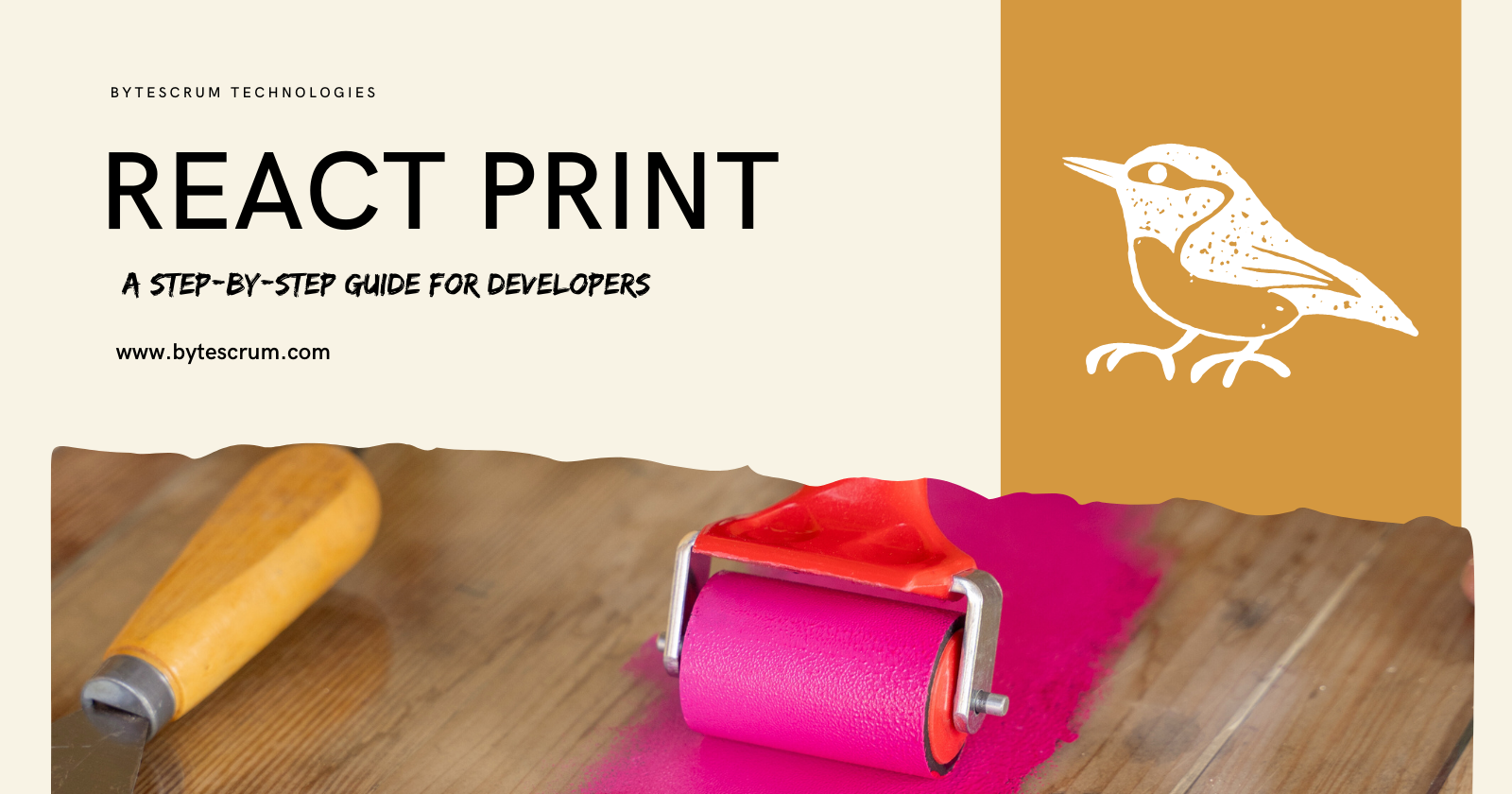
In the world of web development, printing content directly from web applications can be a challenging yet essential task. React, a popular JavaScript library for building user interfaces, offers various ways to handle printing. This guide provides an in-depth look at how to implement printing in a React application, including the use of the react-to-print
library, styling for print, handling print-related issues, and best practices for ensuring your printed content looks professional.
Introduction to React Printing
Printing directly from a React application involves transforming web-based content into a format that can be printed or saved as a PDF. React's component-based architecture makes it easy to manage and control what gets printed. However, achieving a clean and well-formatted printout requires careful handling of styles, content layout, and print-specific configurations.
Setting Up Printing in React
Using react-to-print
Library
The react-to-print
library is a popular choice for implementing print functionality in React applications. It provides a simple API to handle printing of React components and supports various print configurations.
Installation
To get started, install react-to-print
using npm or yarn:
npm install react-to-print
# or
yarn add react-to-print
Basic Usage
Here's a basic example of how to use react-to-print
to print a React component:
import React, { useRef } from "react";
import { useReactToPrint } from "react-to-print";
const PrintComponent = () => {
const componentRef = useRef();
const handlePrint = useReactToPrint({
content: () => componentRef.current,
documentTitle: "Print Document",
});
return (
<div>
<button onClick={handlePrint}>Print this out!</button>
<div style={{ display: "none" }}>
<MyComponentToPrint ref={componentRef} />
</div>
</div>
);
};
const MyComponentToPrint = React.forwardRef((props, ref) => (
<div ref={ref}>
<h1>Hello, World!</h1>
</div>
));
export default PrintComponent;
In this example:
useRef
is used to create a reference to the component that will be printed.useReactToPrint
is used to handle the printing logic.MyComponentToPrint
is the component that you want to print, and it is passed tocontent
as a reference.
Creating a Print Component
Create a separate component specifically for printing purposes. This component can include the content and styles you want to print.
Example:
import React from "react";
const PrintContent = React.forwardRef((props, ref) => (
<div ref={ref}>
<h1>Printable Content</h1>
<p>This content will be printed when the button is clicked.</p>
</div>
));
export default PrintContent;
Styling for Print
CSS for Print Media
To ensure your printed content looks good, you should use CSS specifically for print media. This involves creating styles that apply only when printing.
@media print {
.printable {
width: 100%;
margin: 0 auto;
font-size: 12pt;
}
@page {
size: A4;
margin: 10mm;
}
.page-break {
page-break-before: always;
}
.avoid-break {
page-break-inside: avoid;
}
}
In this example:
@media print
specifies styles that apply when printing.@page
defines page size and margins..page-break
and.avoid-break
classes control page breaks.
Handling Page Breaks
To manage page breaks effectively, use the page-break-before
and page-break-inside
CSS properties. This ensures that content is properly split across pages and prevents awkward breaks.
.page-break {
page-break-before: always;
}
.avoid-break {
page-break-inside: avoid;
}
Apply these classes to elements where you want to control page breaks, ensuring that your content is neatly paginated.
Advanced Printing Techniques
Dynamic Content Printing
For printing dynamic content, such as user-generated reports or form submissions, you might need to adjust your component based on the content being printed.
const DynamicPrintComponent = ({ data }) => {
const componentRef = useRef();
const handlePrint = useReactToPrint({
content: () => componentRef.current,
documentTitle: "Dynamic Print Document",
});
return (
<div>
<button onClick={handlePrint}>Print Dynamic Content</button>
<div ref={componentRef}>
<h1>Report</h1>
<ul>
{data.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
</div>
</div>
);
};
This approach allows you to print different sets of data based on user interactions or other dynamic conditions.
Handling Large Content
When dealing with large content, ensure it fits well on the page by adjusting margins, font sizes, and content layout. You might also need to handle multi-page layouts effectively.
Use
page-break-before
,page-break-after
, andpage-break-inside
to manage how content flows across pages.Consider adding a header or footer that appears on every page for consistency.
Common Issues and Troubleshooting
Missing Styles
Sometimes, styles may not appear correctly in the printout. Ensure that all necessary styles are included within the @media print
block.
Content Overflow
If content overflows or doesn’t fit on the page, adjust margins, font sizes, and content width. Use CSS properties like overflow
and text-overflow
to manage overflow.
Print Preview
Always use the print preview feature of your browser to check how your content will look before printing. This helps identify any issues with layout or styling.
Best Practices
Preview Before Printing: Always preview the print layout to ensure it looks correct.
Separate Print Styles: Keep print-specific styles separate from screen styles using
@media print
.Handle Page Breaks: Use CSS properties to manage page breaks and avoid awkward splits.
Test with Various Content: Test with different types and lengths of content to ensure consistent results.
Conclusion
react-to-print
library, handling print-specific styles, and managing page breaks, you can ensure your printed content looks professional and fits well on the page.With the techniques and best practices outlined in this guide, you'll be well-equipped to handle printing in your React applications effectively. Happy coding and printing!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
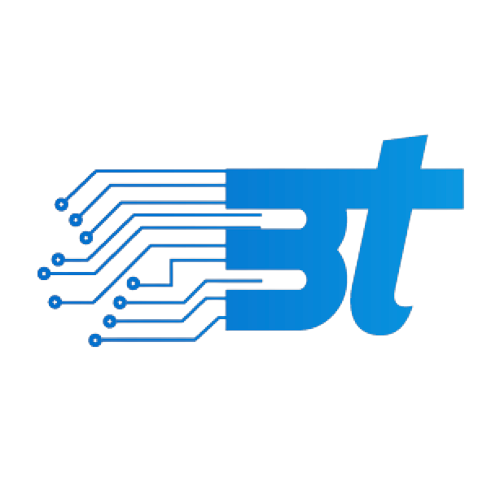
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.