Fetch vs Axios: Choosing the Right API Tool in JavaScript
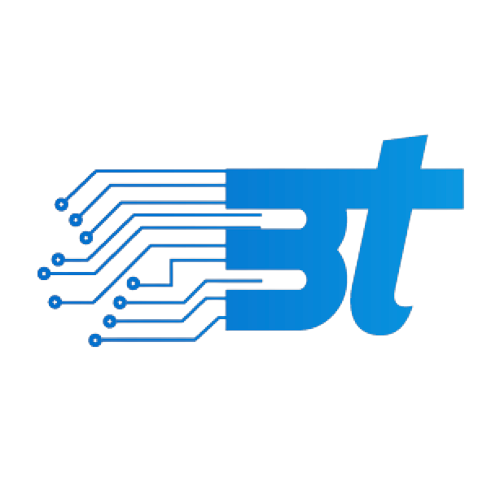
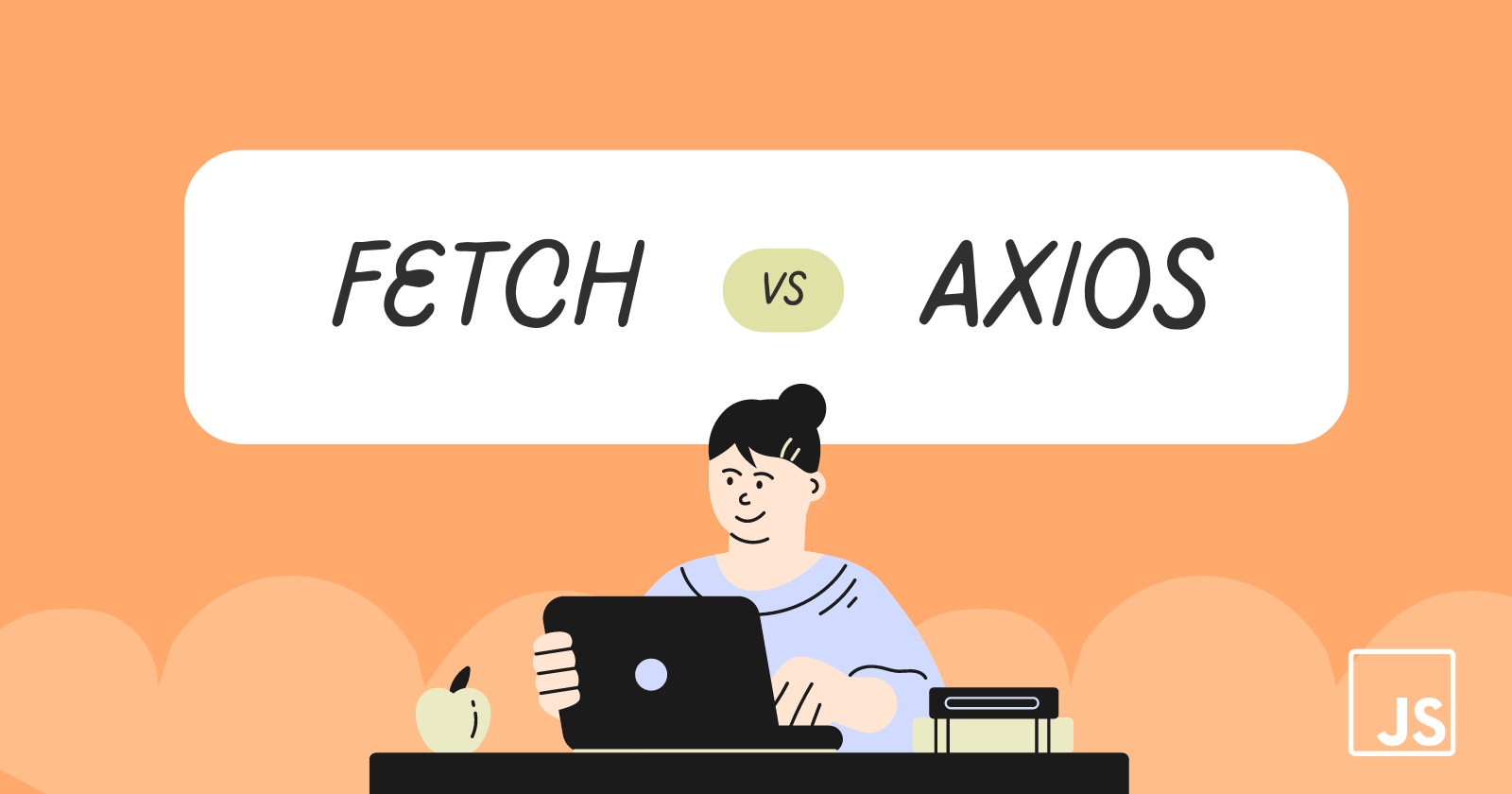
When you’re building a web application, interacting with APIs is almost inevitable. Whether you’re pulling in data from an external source or sending information to a server, making HTTP requests is essential. In JavaScript, two popular ways to make these requests are Fetch and Axios.
In this guide, we’ll take a closer look at these tools, breaking down when and why you should use each one, and how they differ in real-world scenarios.
What is Fetch?
Fetch is the native way to make HTTP requests in JavaScript. Introduced in modern browsers, Fetch allows you to perform asynchronous operations using Promises. Unlike its predecessor, XMLHttpRequest, Fetch is much cleaner and more flexible for handling requests.
Why Use Fetch?
Fetch is built directly into the browser, so you don’t need to install any libraries or dependencies to use it. It’s perfect for straightforward API requests and works seamlessly with JavaScript’s Promise system.
Key Features of Fetch:
Promise-Based: Fetch uses Promises, so handling asynchronous responses becomes much easier and more readable compared to older methods.
No Installation: Since Fetch is native to JavaScript, you don’t need to install any external libraries, making it a lightweight option.
Supports Modern Browsers: Fetch is supported by all modern browsers, so it’s perfect for projects that target modern environments.
Limitations of Fetch:
Basic Error Handling: Fetch considers any request that reaches the server as “successful” even if the server returns an error like 404 or 500. You have to manually check the response status to handle errors.
Manual JSON Parsing: Fetch doesn’t automatically convert response bodies into JSON. You need to explicitly call
.json()
on the response object.No Built-in Timeout Support: Fetch doesn’t provide a native way to set request timeouts. If your API request takes too long, you need to implement custom timeout logic.
Basic Fetch Example:
Here’s a simple Fetch example for making a GET request:
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) { // Manually check if the response was successful
throw new Error('Network response was not ok');
}
return response.json(); // Convert response to JSON
})
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
In this example, Fetch is used to send a GET request to an API endpoint. The .json()
method is manually called to parse the response, and error handling requires checking the response.ok
property to detect non-200 status codes.
What is Axios?
Axios is a popular JavaScript library for making HTTP requests. It’s not built into the browser like Fetch, so you’ll need to install it, but Axios comes with a wide range of features that can make working with APIs easier.
Why Use Axios?
Axios is a great option for developers who need more robust error handling, automatic JSON parsing, and additional features like request timeouts. It works well for more complex API interactions and supports both the browser and Node.js environments.
Key Features of Axios:
Automatic JSON Parsing: Unlike Fetch, Axios automatically converts JSON responses, saving you the extra step.
Better Error Handling: Axios will throw an error for any response that isn’t in the 2xx range (e.g., 404, 500), making it easier to handle server-side errors.
Request Timeouts: Axios has built-in support for timeouts, which is useful for ensuring your API calls don’t hang indefinitely.
Interceptors: Axios lets you intercept requests and responses to modify or log them, which is great for debugging or modifying headers.
More Flexible Configuration: Axios allows more complex request configurations, including custom headers, query parameters, and more.
Limitations of Axios:
External Dependency: Unlike Fetch, you need to install Axios as a dependency, adding extra weight to your project.
Slightly Larger Size: Since Axios is a third-party library, it adds more code to your project compared to Fetch.
Basic Axios Example:
Here’s a simple Axios example for making the same GET request:
axios.get('https://api.example.com/data')
.then(response => console.log(response.data)) // Axios automatically parses JSON
.catch(error => console.error('Error:', error)); // Axios handles errors automatically
In this example, Axios automatically handles JSON parsing and throws an error if the response status is outside the 2xx range, making the code more concise and reliable.
Fetch vs Axios: Head-to-Head Comparison
Now that we’ve seen how both Fetch and Axios work, let’s compare them directly:
1. Ease of Use:
Fetch: Great for simple, quick requests. However, it requires extra work for error handling and JSON parsing.
Axios: Provides a more streamlined experience out of the box with automatic JSON parsing and better error handling.
2. Error Handling:
Fetch: Only throws an error for network failures. You need to manually check response statuses for HTTP errors.
Axios: Automatically throws an error for non-2xx responses, making it easier to catch both network and server-side issues.
3. Timeouts:
Fetch: No built-in timeout support. You need to use additional logic to implement timeouts.
Axios: Supports timeouts natively, allowing you to set a maximum duration for your requests.
4. Interceptors:
Fetch: Does not support request/response interceptors.
Axios: Supports interceptors, which can be used to modify requests or responses before they are handled. This is especially useful for adding authentication tokens or logging requests.
5. Browser Support:
Fetch: Supported by all modern browsers, but not by older ones (such as IE11).
Axios: Works in both modern browsers and older browsers, including Internet Explorer.
6. File Uploads:
Fetch: Supports file uploads, but it can be more complicated to implement.
Axios: Provides better support for file uploads with FormData and progress tracking.
Fetch Example with Timeout:
If you want to set a timeout with Fetch, you have to use extra code:
const controller = new AbortController();
const timeout = setTimeout(() => controller.abort(), 5000); // 5-second timeout
fetch('https://api.example.com/data', { signal: controller.signal })
.then(response => response.json())
.then(data => console.log(data))
.catch(error => {
if (error.name === 'AbortError') {
console.error('Request timed out');
} else {
console.error('Error:', error);
}
});
Axios Example with Timeout:
In Axios, timeout support is built-in, making it easier to use:
axios.get('https://api.example.com/data', { timeout: 5000 }) // 5-second timeout
.then(response => console.log(response.data))
.catch(error => {
if (error.code === 'ECONNABORTED') {
console.error('Request timed out');
} else {
console.error('Error:', error);
}
});
When to Use Fetch:
If your project is small and simple: For basic GET or POST requests, Fetch is lightweight and gets the job done without adding extra dependencies.
When you don’t want to rely on external libraries: Fetch is built-in, so it’s perfect if you want to minimize external packages.
When to Use Axios:
For more complex API interactions: If you’re working on a larger project with more complicated requirements like timeouts, error handling, or request interceptors, Axios provides more features.
When you need better error handling: Axios makes it easier to handle HTTP errors and provides useful defaults for handling common API patterns.
If you need cross-browser support: Axios works well across all browsers, including older ones like Internet Explorer.
Conclusion
Understanding when to use each tool will help you make better decisions when working with APIs in your JavaScript projects.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
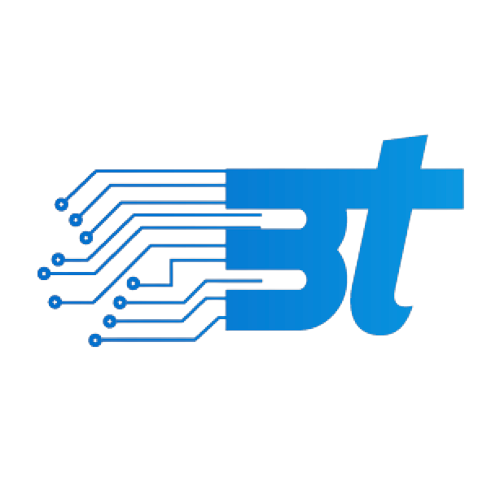
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.