How to Build a Custom JavaScript Slider from Scratch
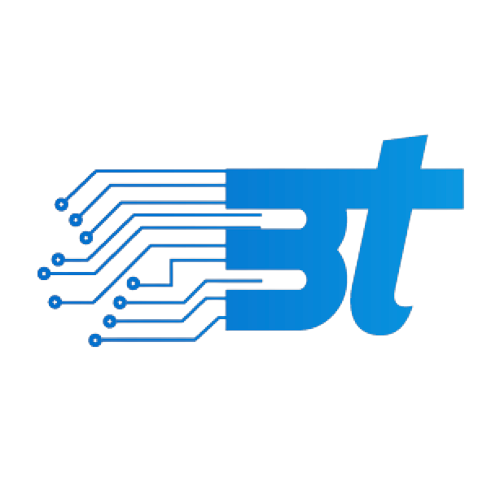

Modern web development is all about creating interactive and dynamic user experiences, and custom JavaScript components are a key part of that. While libraries like jQuery, React, or Vue offer pre-built components, there’s great value in understanding how to build them yourself from scratch.
In this guide, we’ll walk through the process of building a simple yet effective slider (also known as a carousel) using vanilla JavaScript. You’ll learn how to create a reusable slider component, understand the core concepts of component-based development, and customize the functionality.
What is a Slider?
A slider is a UI component that allows users to cycle through a set of content, typically images or cards, by moving horizontally (or vertically). Sliders are commonly used in galleries, testimonials, or product showcases to display content in an interactive, space-efficient way.
Key Features of a Slider:
Navigation Buttons: To move forward and backward through the slides.
Auto-Sliding: Slides can automatically transition after a set time.
Customizable: The slider can have various transition effects, speeds, and content types.
We’ll build a simple slider with the following features:
Navigation buttons (Next/Previous).
Automatic sliding with adjustable intervals.
Smooth transitions between slides.
Step 1: HTML Structure
Before jumping into JavaScript, let’s start by setting up the basic HTML structure for our slider. We’ll create a container to hold the slides and two buttons for navigation.
<div class="slider">
<div class="slider-wrapper">
<div class="slide">Slide 1</div>
<div class="slide">Slide 2</div>
<div class="slide">Slide 3</div>
</div>
<button class="slider-btn prev-btn">Previous</button>
<button class="slider-btn next-btn">Next</button>
</div>
Explanation:
The
slider
div serves as the main container for the component.The
slider-wrapper
holds all the slides. Each slide is represented by adiv
with the classslide
.The two buttons,
prev-btn
andnext-btn
, allow users to navigate between slides.
Step 2: CSS for Styling
Next, we’ll add some basic CSS to style the slider. We want the slides to appear in a row and hide the ones that aren’t active.
.slider {
width: 100%;
max-width: 600px;
position: relative;
overflow: hidden;
}
.slider-wrapper {
display: flex;
transition: transform 0.5s ease-in-out;
}
.slide {
min-width: 100%;
box-sizing: border-box;
padding: 20px;
text-align: center;
background-color: #f4f4f4;
}
.slider-btn {
position: absolute;
top: 50%;
transform: translateY(-50%);
background-color: rgba(0, 0, 0, 0.5);
color: #fff;
border: none;
padding: 10px;
cursor: pointer;
}
.prev-btn {
left: 10px;
}
.next-btn {
right: 10px;
}
Key Styles:
slider-wrapper is a flex container that lays out the slides horizontally.
transition on the
slider-wrapper
creates a smooth sliding effect when moving between slides.overflow: hidden on the
slider
container ensures that only one slide is visible at a time.slider-btn styles the navigation buttons, positioning them on the left and right of the slider.
Step 3: JavaScript for Functionality
Now for the fun part! We’ll write JavaScript to make the slider work. We’ll focus on creating navigation between slides and implementing automatic sliding.
Setting Up Basic Navigation:
We’ll add event listeners to the Next and Previous buttons to move between the slides.
const sliderWrapper = document.querySelector('.slider-wrapper');
const slides = document.querySelectorAll('.slide');
const prevBtn = document.querySelector('.prev-btn');
const nextBtn = document.querySelector('.next-btn');
let currentIndex = 0; // Track the current slide index
// Function to update the slider position
function updateSliderPosition() {
const offset = currentIndex * -100; // Calculate the offset for sliding
sliderWrapper.style.transform = `translateX(${offset}%)`;
}
// Event listener for the Next button
nextBtn.addEventListener('click', () => {
currentIndex = (currentIndex + 1) % slides.length; // Loop back to the first slide
updateSliderPosition();
});
// Event listener for the Previous button
prevBtn.addEventListener('click', () => {
currentIndex = (currentIndex - 1 + slides.length) % slides.length; // Loop to the last slide
updateSliderPosition();
});
Explanation:
currentIndex keeps track of which slide is currently visible.
updateSliderPosition changes the
transform
property of theslider-wrapper
to move the slides horizontally.nextBtn and prevBtn event listeners update the
currentIndex
and callupdateSliderPosition()
to shift the slides left or right.
Step 4: Adding Auto-Slide Feature
To make our slider more dynamic, we’ll add an automatic sliding feature that advances to the next slide after a certain interval.
const slideInterval = 3000; // 3 seconds interval for auto-sliding
function startAutoSlide() {
setInterval(() => {
currentIndex = (currentIndex + 1) % slides.length;
updateSliderPosition();
}, slideInterval);
}
startAutoSlide();
Explanation:
slideInterval sets the duration between automatic slides (in milliseconds).
startAutoSlide uses
setInterval
to automatically move to the next slide every 3 seconds.
Step 5: Improving UX with Indicators
We can further enhance the slider by adding indicators (dots) that show the current slide and allow users to jump to any slide by clicking on them.
HTML for Indicators:
<div class="slider-indicators">
<span class="indicator"></span>
<span class="indicator"></span>
<span class="indicator"></span>
</div>
CSS for Indicators:
.slider-indicators {
position: absolute;
bottom: 10px;
left: 50%;
transform: translateX(-50%);
display: flex;
gap: 10px;
}
.indicator {
width: 10px;
height: 10px;
background-color: rgba(255, 255, 255, 0.5);
border-radius: 50%;
cursor: pointer;
}
.indicator.active {
background-color: rgba(255, 255, 255, 1);
}
JavaScript for Indicators:
const indicators = document.querySelectorAll('.indicator');
// Function to update the active indicator
function updateIndicators() {
indicators.forEach((indicator, index) => {
indicator.classList.toggle('active', index === currentIndex);
});
}
// Update indicators when slider position changes
function updateSliderPosition() {
const offset = currentIndex * -100;
sliderWrapper.style.transform = `translateX(${offset}%)`;
updateIndicators(); // Call the new function to update the indicator
}
// Add click event to indicators to jump to specific slide
indicators.forEach((indicator, index) => {
indicator.addEventListener('click', () => {
currentIndex = index;
updateSliderPosition();
});
});
Explanation:
updateIndicators toggles the
active
class on the indicator matching the current slide.Clicking on an indicator jumps to the corresponding slide by updating the
currentIndex
.
Step 6: Wrapping Up
By following these steps, you’ve successfully built a fully functional slider from scratch using vanilla JavaScript. This slider can be customized further with different transition effects, swipe gestures for mobile, or infinite looping.
Why Build Your Own Slider?
Learning Experience: Understanding how to build components like this from scratch helps improve your JavaScript skills.
Customization: Custom sliders give you full control over the design and functionality, without relying on third-party libraries.
Performance: Creating lightweight components without the overhead of larger libraries can improve your site’s performance.
Now you have the foundation for creating your own custom components, and you can apply this knowledge to build other dynamic features in your web applications!
Next Steps:
Experiment with different transition effects (e.g., fading or zooming).
Add touch/swipe support for mobile devices.
Expand the slider to handle dynamic content loading from APIs.
By mastering custom components, you can make your web applications stand out with unique, interactive user experiences.
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
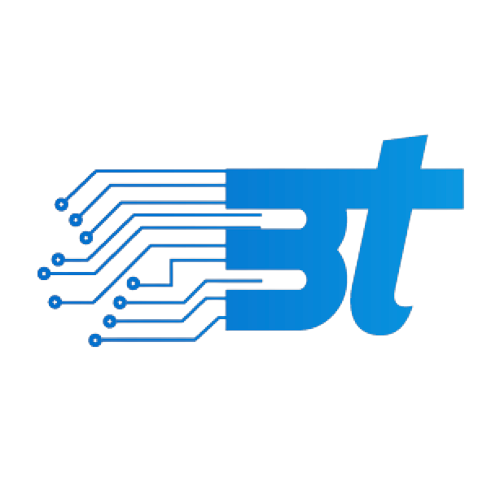
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.