Automating Testing with Jest: Boosting Your Code Confidence
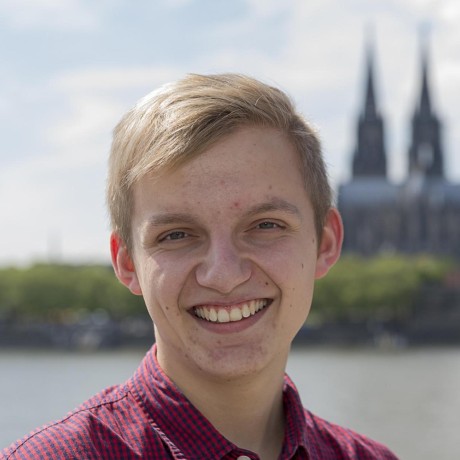
As developers, we strive to write clean, maintainable, and bug-free code. But let’s face it - no matter how experienced we are, bugs always find a way into our codebase. Unit testing can alleviate some of those woes by providing confidence that our code works as intended. And when it comes to testing in JavaScript projects, Jest is often the tool of choice.
In this article, we’ll dive into the benefits of automating testing with Jest , how to get started, and some best practices to ensure you’re getting the most out of your tests.
Why Should You Use Jest?
Jest is a popular testing framework developed and maintained by Facebook. It integrates seamlessly with most modern front-end frameworks like React, Vue, and Angular as well as Node.js backends. So why is Jest worth your time?
1. Easy Setup and Zero Configuration
Jest is designed to be out-of-the-box ready, so you don’t need a complex setup to start writing tests. With just a few commands, you can get started in minutes.
Once you install Jest, it automatically sets up the right environment for your tests, detects your test files, and provides a clean and intuitive testing experience.
2. Painless Mocking and Snapshot Testing
Jest offers powerful tools for creating unit tests by allowing you to mock functions, databases, and even entire modules. This is extremely helpful when you want to isolate your tests from external dependencies and minimize side effects.
Additionally, Jest’s snapshot testing feature helps in rendering and then comparing components. This is particularly useful in front-end development, where user interface components frequently change.
3. Excellent Performance
Jest runs tests in parallel, which improves the speed of running your tests dramatically. It uses worker threads to test multiple files simultaneously, ensuring that large test suites don’t slow down your development process.
4. Great Developer Experience
Jest provides a watch mode that reruns tests related to files that have changed, significantly speeding up your feedback loop. It also offers clear and detailed error messages, making it easier to debug failing tests.
Finally, Jest integrates seamlessly with popular CI (Continuous Integration) tools like CircleCI and Travis, making it easy to automate and scale test execution across large codebases.
Getting Started with Jest: A Simple Example
Let’s walk through a basic introduction to setting up Jest in a Node.js project, so you can see the benefits for yourself.
Step 1: Installation
Assuming you've already got a Node.js project configured, installing Jest is as simple as running:
npm install --save-dev jest
Alternatively, if you're using Yarn:
yarn add --dev jest
Once installed, add a test script in your package.json
file:
{
"scripts": {
"test": "jest"
}
}
Now, whenever you run npm test
or yarn test
, Jest will execute your test suite.
Step 2: Writing Your First Test
Let’s create a simple JavaScript file, sum.js
, that exports a small function for summing two numbers:
// sum.js
function sum(a, b) {
return a + b;
}
module.exports = sum;
Next, let’s write a test for this function. Create another file called sum.test.js
:
// sum.test.js
const sum = require('./sum');
test('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
Here, the test
function takes two arguments: a string describing what your test is doing, and a callback function for the test itself. The expect
function checks that the return value of sum(1, 2)
is equal to 3.
Step 3: Running Your Tests
Let’s run the test now by invoking the following command:
npm test
You should see the output:
PASS ./sum.test.js
✓ adds 1 + 2 to equal 3 (5ms)
Congratulations! You’ve just written your first Jest test.
Best Practices for Writing Effective Unit Tests
Now that you’re up and running with Jest, let's talk about how to get the most out of your tests. Writing tests is important, but writing good, meaningful tests is what ensures the long-term stability of your project.
1. Test Behavior, Not Implementation
When writing tests, it's important to focus on testing the behavior of your code, rather than its implementation . This means that your tests shouldn’t depend too much on the internal structure of your code, because refactoring implementations should not require you to adjust tests.
For instance, if a function is supposed to return a sum, you should test the result of the function call and not the inner workings of how the sum is calculated.
// GOOD: Testing behavior
test('returns correct sum', () => {
expect(sum(5, 3)).toBe(8);
});
// BAD: Testing internal implementation (e.g., using a loop or direct logic inspection)
test('uses addition to calculate the sum', () => {
const spy = jest.spyOn(Math, 'add');
sum(5, 3);
expect(spy).toHaveBeenCalled();
});
2. Choose Descriptive Test Names
The string you pass to the test()
function should describe what you're testing and why it's important. This will make outcomes clearer, especially when a test fails. Opt for human-readable language.
GOOD: 'calculates subtotal with multiple products'
BAD: 'subTotal function'
Choosing descriptive test names also helps you avoid meaningless messages when you run your test suite (e.g., "Test 4 failed" doesn't help anyone).
3. Avoid Testing Framework Code
Your tests should be strictly focused on application code , not the code or methods provided by the framework you’re using. For instance, if you're testing a React component, avoid testing React-specific behavior. React already has its own tests to ensure that its functionality (like useState
or useEffect
) works correctly.
4. Use Before/After Hooks to Reduce Duplication
Jest provides lifecycle hooks such as beforeEach
or afterEach
to organize test setups and minimize repetitive code. This is especially useful for code that needs to be initialized before each test:
beforeEach(() => {
initializeSettings();
});
test('should load settings properly', () => {
expect(settings.loaded).toBe(true);
});
This separates setup logic from test logic, leading to cleaner and more maintainable test suites.
5. Mock External Dependencies
When testing functions that rely on external dependencies (e.g., APIs or databases), you don’t want actual HTTP requests being made in your test suite. Instead, use Jest’s built-in mocking functions to simulate those dependencies and test in isolation.
For example, if you’re calling a REST API:
jest.mock('axios');
const axios = require('axios');
const fetchData = require('./fetchData');
// Simulate an API response
axios.get.mockResolvedValue({ data: { name: 'Sebi' } });
test('fetches the user data', async () => {
const user = await fetchData();
expect(user.name).toBe('Sebi');
});
Mocking functions prevent your tests from being dependent on external services, making them faster and more reliable.
Conclusion
Testing isn’t just an option—it’s an essential part of building maintainable and robust applications. Jest , with its ease of setup, powerful mocking, and fast execution, makes the process painless and effective.
By using the best practices we've outlined here—focusing on behavior over implementation, organizing test structure, and using mocks—you can take your testing strategy to the next level.
So, are you ready to boost your code confidence with Jest? Dive in, start small, and keep iterating on your tests—your future self will thank you!
Subscribe to my newsletter
Read articles from Sebastian Goscinski directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
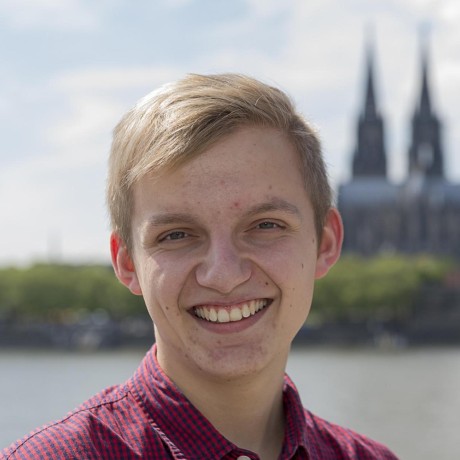
Sebastian Goscinski
Sebastian Goscinski
Hi there 👋, I´m Sebastian 👨💻 Passionate Full-Stack Developer with a knack for creating efficient and scalable applications. Enthusiastically exploring the world of web development with Next.js, React, Angular, and .NET. Join me as I share insights, tips, and best practices on building modern web applications. Let’s code something amazing together!