Building an Easybank Landing Page with React

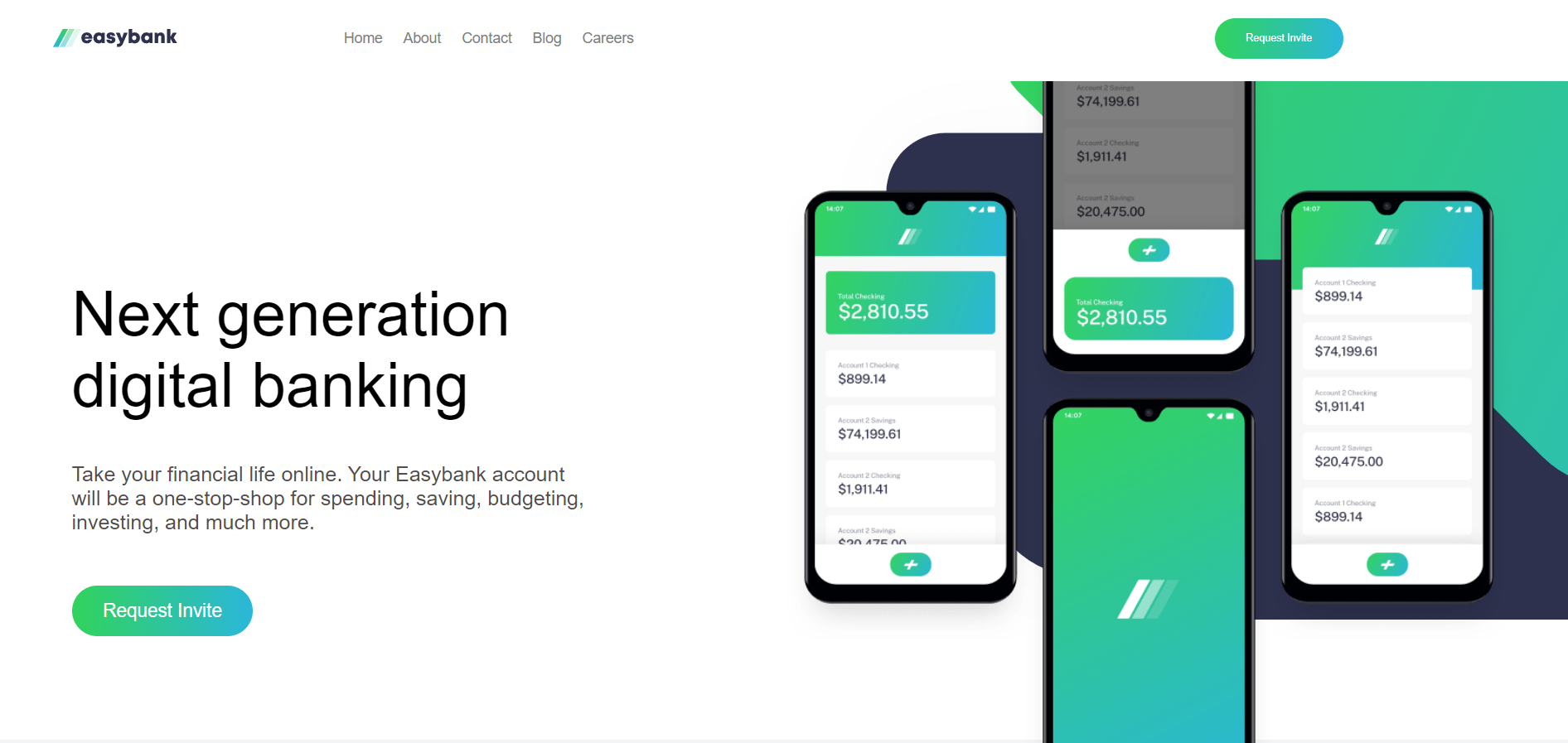
Introduction
In this blog post, I’ll walk you through the development of a modern and visually appealing landing page for a fictional digital banking service, Easybank, using React. This project showcases essential React components and CSS styling to create a responsive and interactive user interface.
Project Overview
The Easybank landing page features a clean and modern design with a focus on simplicity and user engagement. The page is divided into several key sections:
Navbar: Provides navigation links and a request invite button.
Home: Displays a hero section with a compelling headline and a call-to-action button.
About: Highlights the key features of Easybank with attractive icons and descriptions.
Blog: Showcases the latest articles related to the banking service.
Footer: Contains social media links, company information, and a request invite button.
Features
Responsive Design: The layout adapts to various screen sizes, ensuring a consistent experience across devices.
Interactive Components: Hover effects and clickable elements enhance user interaction.
Modern Aesthetics: Utilizes a minimalist color scheme and clean typography.
Technologies Used
React: For building the component-based user interface.
CSS: For styling and responsive design.
Project Structure
The project is organized into the following components:
App.js: Main component that renders all other components.
Navbar.js: Contains the navigation bar.
Home.js: Displays the hero section of the landing page.
About.js: Provides information about the features of Easybank.
Blog.js: Lists recent articles.
Footer.js: Displays social media links and company information.
App.css: Stylesheet for the project.
Installation
To get started with this project, clone the repository and install the necessary dependencies:
git clone https://github.com/abhishekgurjar-in/Easybank-Landing-Page
cd easybank-landing-page
npm install
Usage
To run the project locally, use the following command:
npm start
This will start the development server and open the application in your default web browser.
Code Explanation
Here’s a brief overview of the main components:
- App.js:
import "./App.css"; import Navbar from "./components/Navbar"; import About from "./components/About"; import Blog from "./components/Blog"; import Footer from "./components/Footer"; import Home from "./components/Home";
const App = () => { return ( <>
</> ); };
export default App;
This is the main component that renders all the sections of the landing page.
- **Navbar.js**:
```jsx
import logo from "../assets/images/logo.svg";
const Navbar = () => {
return (
<div className='navbar'>
<img className='logo' src={logo} alt="Easybank logo" />
<div className="header">
<a href="#">Home</a>
<a href="#">About</a>
<a href="#">Contact</a>
<a href="#">Blog</a>
<a href="#">Careers</a>
</div>
<button className='request-btn'>Request Invite</button>
</div>
);
}
export default Navbar;
The Navbar component provides navigation links and a button for requesting an invite.
Home.js:
import phoneImage from "../assets/images/image-mockups.png"
const Home = () => { return (
Next generation
digital banking
Take your financial life online. Your Easybank account will be a one-stop-shop for spending, saving, budgeting, investing, and much more.
Request Invite
); };
export default Home;
The Home component showcases the primary call-to-action and an image mockup.
- **About.js**:
```jsx
import bankingImage from "../assets/images/icon-online.svg";
import onboardingImage from "../assets/images/icon-onboarding.svg";
import apiImage from "../assets/images/icon-api.svg";
const About = () => {
return (
<div className="about">
<div className="about-first">
<h1 className="about-title">Why choose Easybank?</h1>
<p>
We leverage Open Banking to turn your bank account into your financial
hub. Control your finances like never before.
</p>
</div>
<div className="about-second">
<div className="card">
<img src={bankingImage} alt="Online Banking" />
<h1>Online Banking</h1>
<p>
Our modern web and mobile applications allow you to keep track of
your finances wherever you are in the world.
</p>
</div>
<div className="card">
<img src={bankingImage} alt="Simple Budgeting" />
<h1>Simple Budgeting</h1>
<p>
See exactly where your money goes each month. Receive notifications
when you’re close to hitting your limits.
</p>
</div>
<div className="card">
<img src={onboardingImage} alt="Fast Onboarding" />
<h1>Fast Onboarding</h1>
<p>
We don’t do branches. Open your account in minutes online and start
taking control of your finances right away.
</p>
</div>
<div className="card">
<img src={apiImage} alt="Open API" />
<h1>Open API</h1>
<p>
Manage your savings, investments, pension, and much more from one
account. Tracking your money has never been easier.
</p>
</div>
</div>
</div>
);
};
export default About;
The About component highlights the key features of Easybank with descriptive cards.
- Blog.js:
import blog1Image from "../assets/images/image-currency.jpg"; import blog2Image from "../assets/images/image-restaurant.jpg"; import blog3Image from "../assets/images/image-plane.jpg"; import blog4Image from "../assets/images/image-confetti.jpg";
const Blog = () => { const blogData = [ { image: blog1Image, author: "Claire Robinson", title: "Receive money in any currency with no fees", description: "The world is getting smaller and we’re becoming more mobile. So why should you be forced to only receive money in a single...", }, { image: blog2Image, author: "Wilson Hutton", title: "Treat yourself without worrying about money", description: "Our simple budgeting feature allows you to separate out your spending and set realistic limits each month. That means you...", }, { image: blog3Image, author: "Wilson Hutton", title: "Take your Easybank card wherever you go", description: "We want you to enjoy your travels. This is why we don’t charge any fees on purchases while you’re abroad. We’ll even show you...", }, { image: blog4Image, author: "Claire Robinson", title: "Our invite-only Beta accounts are now live!", description: "After a lot of hard work by the whole team, we’re excited to launch our closed beta. It’s easy to request an invite through the site...", }, ];
return (
Latest Articles
{blogData.map((blog, index) => (
By {blog.author}
{blog.title}
{blog.description}
))}
); };
export default Blog;
The Blog component displays recent articles with images and brief descriptions.
- **Footer.js**:
```jsx
import Logo from "../assets/images/logo.svg";
import fb from "../assets/images/icon-facebook.svg";
import yt from "../assets/images/icon-youtube.svg";
import tw from "../assets/images/icon-twitter.svg";
import pt from "../assets/images/icon-pinterest.svg";
import ig from "../assets/images/icon-instagram.svg";
const Footer = () => {
return (
<div className="footer">
<div className="logos">
<img src={Logo} alt="" />
<div className="social-logo">
<a href="">
<img src={fb} alt="" />
</a>
<a href="">
<img src={yt} alt="" />
</a>
<a href="">
<img src={tw} alt="" />
</a>
<a href="">
<img src={pt} alt="" />
</a>
<a href="">
<img src={ig} alt="" />
</a>
</div>
</div>
<div className="aboutus">
<a href="">About Us</a>
<a href="">Contact</a>
<a href="">Blog</a>
</div>
<div className="carrers">
<a href="">Careers</a>
<a href="">Support</a>
<a href="">Privacy Policy</a>
</div>
<div className="invite">
<div className="request-btn">Request Invite</div>
<p>© Easybank. All rights reserved.</p>
<p>Made with ❤️ by Abhishek Gurjar</p>
</div>
</div>
);
};
export default Footer;
The Footer component includes navigation links, social media icons, and company information.
Live Demo
You can view the live demo of the Easybank landing page here.
Conclusion
Creating a landing page with React allows for the development of a modular and maintainable codebase. By structuring components effectively and utilizing modern CSS techniques, you can create a visually appealing and functional webpage. I hope this guide helps you in your journey to mastering React and building impressive web projects.
Credits
Design Inspiration: Easybank design from Design Course
Icons: Icons8
Images: Unsplash
Author
Abhishek Gurjar is a dedicated web developer passionate about creating practical and functional web applications. Check out more of his projects on GitHub.
Subscribe to my newsletter
Read articles from Abhishek Gurjar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Abhishek Gurjar
Abhishek Gurjar
I’m Abhishek Gurjar, a Full Stack MERN developer specializing in MongoDB, Express.js, React, and Node.js. I love creating dynamic web apps and sharing insights on web development.