Understanding Coroutines in Kotlin: Simplify Asynchronous Programming in Android
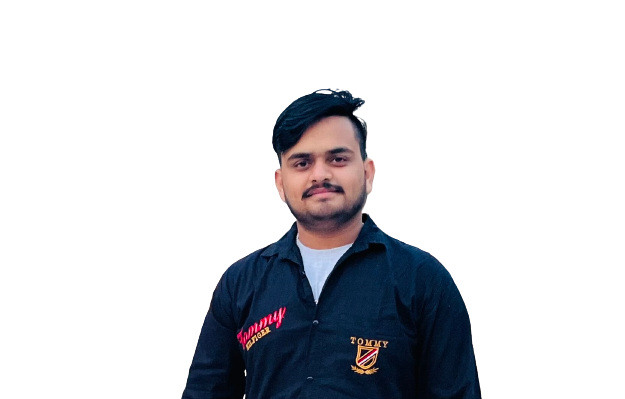
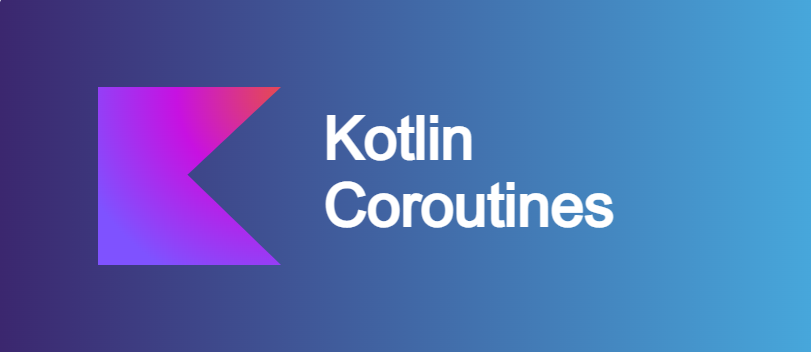
Introduction
Asynchronous programming can be challenging, especially when dealing with multiple tasks like network requests, database operations, and UI updates in Android. Kotlin Coroutines offer a solution to simplify this process, allowing you to write asynchronous code in a more readable and maintainable way. In this blog, we’ll dive into the basics of Kotlin Coroutines and how they can help you simplify asynchronous programming in Android.
What are Coroutines?
Coroutines are a Kotlin feature that helps you write asynchronous code in a sequential style. They are lightweight threads that can be suspended and resumed without blocking the main thread. This makes coroutines perfect for tasks like network calls or reading from a database, where you don’t want to block the UI thread.
Why Use Coroutines in Android?
Simplified Code: Coroutines allow you to write asynchronous code as if it were synchronous, making your code easier to read and maintain.
No Callbacks: Unlike traditional asynchronous programming with callbacks, coroutines eliminate callback hell, making your code cleaner.
Lightweight: Coroutines are lightweight, meaning you can run thousands of them without performance issues, unlike traditional threads.
Setting Up Coroutines in Android
To start using coroutines in your Android project, you need to add the necessary dependencies to your build.gradle
file:
dependencies {
implementation "org.jetbrains.kotlinx:kotlinx-coroutines-core:$latest_version"
implementation "org.jetbrains.kotlinx:kotlinx-coroutines-android:$latest_version"
}
Basic Coroutine Concepts
1. CoroutineScope and Coroutine Builders
A CoroutineScope
defines the context in which a coroutine runs. The most common coroutine builders are launch
and async
.
launch
: Launches a new coroutine without blocking the current thread. It returns aJob
that can be used to cancel the coroutine if needed.
CoroutineScope(Dispatchers.IO).launch {
// Background task
}
async
: Similar tolaunch
, but it returns aDeferred
object, which allows you to get the result usingawait()
.
val result = CoroutineScope(Dispatchers.IO).async {
// Background task
return@async "Result"
}.await()
2. Dispatchers
Dispatchers determine the thread on which the coroutine will run:
Dispatchers.Main
: Runs the coroutine on the main thread, suitable for UI operations.Dispatchers.IO
: Optimized for I/O operations like reading/writing files or making network requests.Dispatchers.Default
: Used for CPU-intensive tasks.
Example: Making a Network Request with Coroutines
Let’s see how coroutines can simplify making a network request in Android.
import kotlinx.coroutines.*
fun fetchUserData() {
CoroutineScope(Dispatchers.IO).launch {
val userData = fetchFromNetwork()
withContext(Dispatchers.Main) {
// Update UI with userData
}
}
}
suspend fun fetchFromNetwork(): String {
// Simulate network request
delay(1000L)
return "User data"
}
Explanation:
fetchUserData
: This function launches a coroutine on the IO dispatcher to fetch user data from the network. Once the data is fetched, it switches back to the main thread to update the UI usingwithContext(Dispatchers.Main)
.suspend
keyword: ThefetchFromNetwork
function is marked withsuspend
, indicating that it can be paused and resumed later. This is the essence of coroutines, allowing you to perform long-running tasks without blocking the thread.
Handling Exceptions in Coroutines
You can handle exceptions in coroutines using try-catch
blocks or using a CoroutineExceptionHandler
. Here’s an example:
val exceptionHandler = CoroutineExceptionHandler { _, throwable ->
println("Exception caught: $throwable")
}
CoroutineScope(Dispatchers.IO + exceptionHandler).launch {
try {
val data = fetchFromNetwork()
withContext(Dispatchers.Main) {
// Update UI
}
} catch (e: Exception) {
// Handle exception
}
}
Structured Concurrency
Structured concurrency ensures that new coroutines launched within a scope are properly canceled when the scope itself is canceled. This helps prevent memory leaks. A common way to achieve structured concurrency in Android is by using viewModelScope
or lifecycleScope
:
class MyViewModel : ViewModel() {
fun fetchData() {
viewModelScope.launch {
// Coroutine launched within ViewModel scope
}
}
}
Conclusion
Kotlin Coroutines provide a powerful yet simple way to handle asynchronous programming in Android. By understanding the basics of coroutines, you can write more readable, maintainable, and efficient code. Whether you’re making network requests, reading from a database, or updating the UI, coroutines can help you do it more effectively.
What’s Next?
Explore more advanced coroutine concepts like flows and channels.
Learn how to use
LiveData
with coroutines.Dive deeper into structured concurrency with coroutine scopes.
Final Thoughts
As you continue to explore coroutines, you’ll find that they greatly simplify many common programming tasks in Android. Start integrating coroutines into your projects, and see how they can make your code cleaner and more efficient.
Subscribe to my newsletter
Read articles from Mukesh Rajput directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
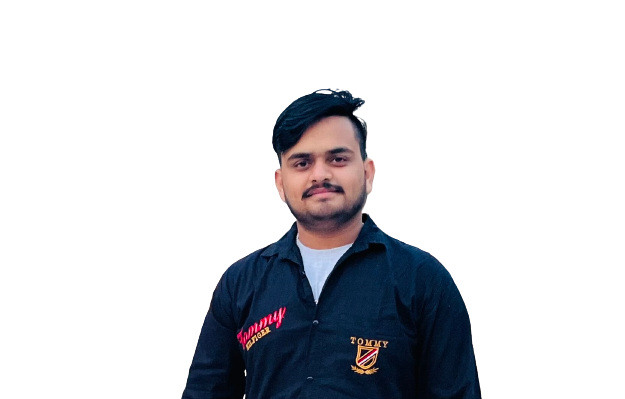
Mukesh Rajput
Mukesh Rajput
Specializing in creating scalable and maintainable applications using MVVM and Clean Architecture principles. With expertise in Ktor, Retrofit, RxJava, View Binding, Data Binding, Hilt, Koin, Coroutines, Room, Realm, and Firebase, I am committed to delivering high-quality mobile solutions that provide seamless user experiences. I thrive on new challenges and am constantly seeking opportunities to innovate in the Android development space.