Transforming Document Summarization: A Deep Dive into Sentence Embeddings, Clustering, and Summarization
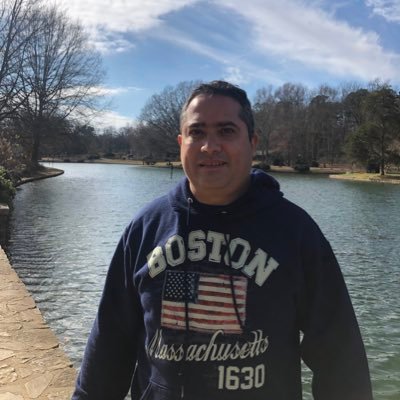
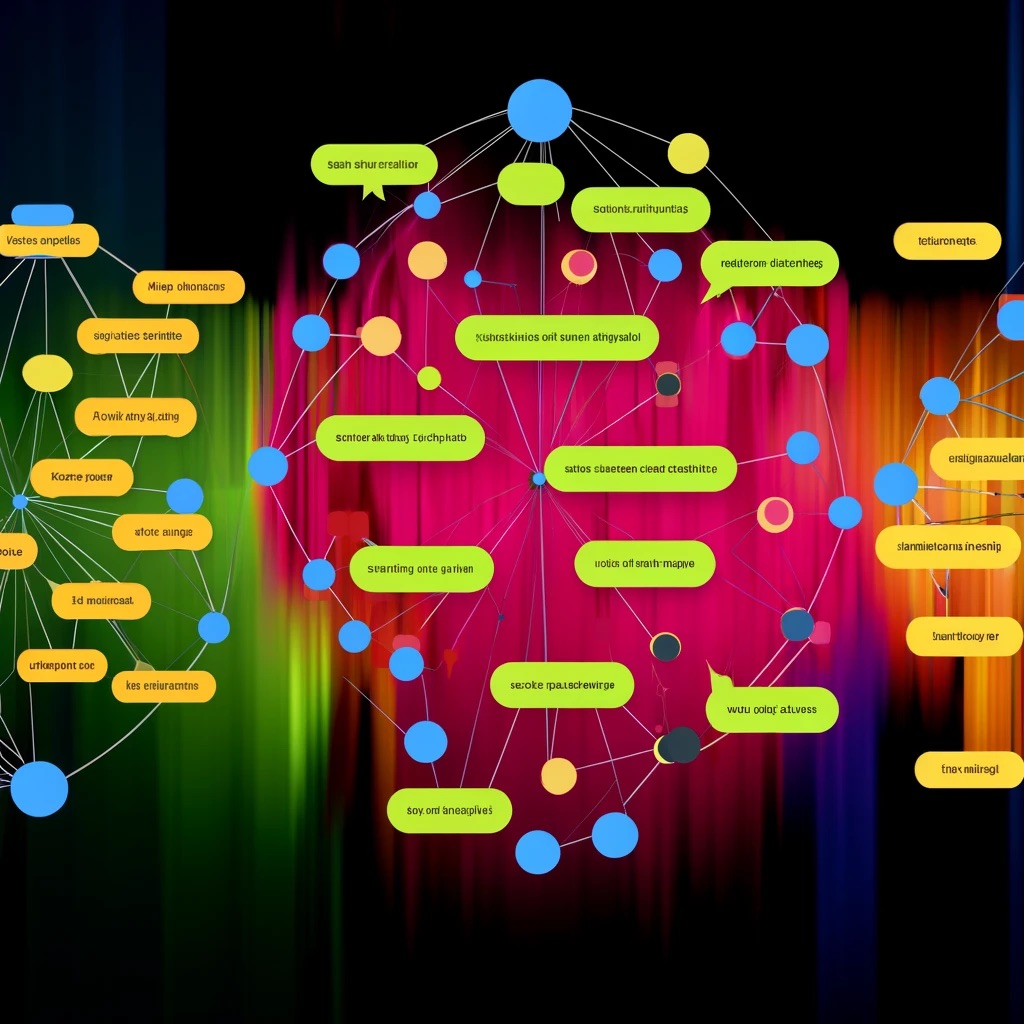
Ever felt lost in a sea of information, struggling to extract meaningful insights from lengthy documents?
You're not alone.
In today's fast-paced world, wading through pages of text to find critical data can be a daunting and time-consuming task.
This often leads to missed opportunities and wasted resources.
Imagine having a tool that can effortlessly segment and summarize these documents, pinpointing key points and making information retrieval a breeze.
This article addresses the common challenge of extracting valuable insights from large documents using advanced techniques like sentence embeddings, clustering, and AI-driven summarization.
By the end of this article, you'll learn how to implement a powerful method to segment and summarize any document efficiently, saving you time and enhancing your productivity.
Dive in to discover how these cutting-edge tools can transform your data processing approach.
Understanding the Core Concepts
Before we dive into the technical details, let's familiarize ourselves with some key concepts:
Sentence Embeddings
Sentence embeddings are vector representations of sentences in a high-dimensional space.
These vectors capture semantic meaning, allowing us to mathematically compare and analyze sentences.
In our summarization technique, we use the SentenceTransformer library to generate these embeddings.
Gap Scores
Gap scores measure the semantic similarity between adjacent groups of sentences.
By analyzing these scores, we can identify potential topic boundaries within a document.
Lower gap scores often indicate a shift in topic or theme.
K-means Clustering
K-means is an unsupervised machine learning algorithm used for clustering similar data points.
In our context, we use it to group semantically related segments of text.
This clustering helps in organizing the document's content into coherent themes.
Implementing Sentence Embeddings
Document Preprocessing
document = """
This is a sample document. It contains multiple sentences.
Each sentence is separated by a period."""
sentences = [s.strip() for s in document.split('.') if s.strip()]
The first step is to break down the document into individual sentences.
We use a simple split on periods, followed by stripping whitespace and removing any empty sentences.
This gives us a clean list of sentences to work with.
Generating Embeddings
from sentence_transformers import SentenceTransformer
# Initialize the SBERT model
model = SentenceTransformer('paraphrase-MiniLM-L6-v2')
# Generate embeddings for each sentence
embeddings = model.encode(sentences)
We use the SentenceTransformer
library to generate embeddings.
The model paraphrase-MiniLM-L6-v2
provides a balance between performance and speed.
Calculating Gap Scores
Gap scores measure the similarity between consecutive sentence embeddings, helping to identify potential segment boundaries.
Gap scores highlight transitions between different topics or sections in a document.
Low similarity scores indicate a change in topic, which can be used to segment the document.
We calculate gap scores by comparing the cosine similarity between adjacent groups of sentences.
from sklearn.metrics.pairwise import cosine_similarity
import numpy as np
# Define the parameter n
n = 2
# Calculate gap scores
gap_scores = []
for i in range(len(embeddings) - n):
similarity = cosine_similarity(
embeddings[i:i+n], embeddings[i+n:i+2*n],
)
gap_scores.append(np.mean(similarity))
Smoothing Gap Scores
Smoothing the gap scores helps reduce noise and highlight significant changes in the document.
Raw gap scores can be noisy.
Smoothing them helps to reveal underlying patterns and significant boundaries more clearly.
To reduce noise and highlight more significant trends, we apply a simple moving average to smooth the gap scores.
The parameter 'k' determines the size of the smoothing window.
# Define the window size k
k = 3
# Smoothing the gap scores
smoothed_gap_scores = np.convolve(
gap_scores,
np.ones(k)/k,
mode='valid',
)
Detecting Local Minima
Local minima in the smoothed gap scores indicate potential segment boundaries.
Local minima represent points where the similarity between consecutive segments is lowest, indicating a potential change in topic.
We use a clever trick with numpy's diff function to identify these points efficiently.
local_minima = (
np.diff(np.sign(np.diff(smoothed_gap_scores))) > 0
).nonzero()[0] + 1
Identifying Significant Boundaries
Not all local minima are significant.
We filter out insignificant ones using a threshold based on the mean and standard deviation of the smoothed gap scores.
The parameter 'c' controls the sensitivity of this filtering.
# Setting the threshold c
c = 1.5
# Identifying significant boundaries
significant_boundaries = [
i for i in local_minima
if smoothed_gap_scores[i] < \
np.mean(smoothed_gap_scores) - c * np.std(smoothed_gap_scores)
]
# Ensure the boundaries cover the entire document
significant_boundaries = [0] + significant_boundaries + [len(sentences)]
Clustering Segment Embeddings
We use KMeans clustering to group similar segments together.
Clustering helps to group similar segments, making it easier to summarize each group coherently.
We calculate the mean embedding for each segment and apply KMeans clustering.
The 'num_clusters' parameter determines how many distinct themes or topics we want to identify in the document.
from sklearn.cluster import KMeans
# Convert segments into embeddings
segment_embeddings = [
np.mean(embeddings[start:end], axis=0)
for start, end in \
zip(significant_boundaries[:-1], significant_boundaries[1:])]
# Apply clustering
num_clusters = 5
kmeans = KMeans(n_clusters=num_clusters)
clusters = kmeans.fit_predict(segment_embeddings)
Summarizing Segments
Using the significant boundaries we've identified, we now group sentences into coherent segments.
Each segment represents a portion of the document that likely covers a specific topic or theme.
Summarization models like facebook/bart-large-cnn
provide concise and coherent summaries of text segments.
We use the transformers
library to summarize each segment.
For each segment, we generate a concise summary using a pre-trained summarization model.
from transformers import pipeline
# each segment represents a specific topic or theme in the document
segments = [
' '.join(sentences[start:end])
for start, end in \
zip(significant_boundaries[:-1], significant_boundaries[1:])]
# Initialize the summarization pipeline
summarizer = pipeline("summarization", model="facebook/bart-large-cnn")
# Summarize each segment
segment_summaries = []
for segment in segments:
summary = summarizer(
segment,
max_length=30,
min_length=10,
do_sample=False,
)[0]['summary_text']
segment_summaries.append(summary)
Combining Cluster Summaries
We combine the summaries of segments within each cluster to form the final document summary.
We aggregate the summaries within each cluster and combine them.
# Organize summaries by cluster
cluster_summaries = {i: [] for i in range(num_clusters)}
for cluster, summary in zip(clusters, segment_summaries):
cluster_summaries[cluster].append(summary)
# Combine cluster summaries
final_summary = []
for cluster in sorted(cluster_summaries.keys()):
cluster_summary = " ".join(cluster_summaries[cluster])
final_summary.append(f"Cluster {cluster + 1}: {cluster_summary}")
# Output the final summary
document_summary = "\n\n".join(final_summary)
print("Document Summary:")
print(document_summary)
Advantages of This Approach
Our AI-powered summarization technique offers several key benefits:
Thematic Organization: By clustering similar segments, we present information in a structured, theme-based format. This makes it easier for readers to grasp the main topics covered in the document.
Scalability: This method can handle documents of varying lengths, from short articles to lengthy reports. The clustering approach ensures that we capture the most important information regardless of document size.
Preservation of Context: Unlike simple extractive summarization methods, our approach maintains the context and relationships between different parts of the document.
Flexibility: By adjusting parameters like the number of clusters or the significance threshold for boundaries, users can fine-tune the summarization to their specific needs.
Improved Comprehension: The combination of segmentation and summarization helps readers quickly understand the main points and structure of complex documents.
Conclusion
Automating document summarization using sentence embeddings, clustering, and summarization models can significantly enhance productivity.
This approach not only saves time but also ensures that key information is extracted accurately.
By following the steps outlined in this article, you can implement a powerful document summarization tool that leverages state-of-the-art NLP techniques.
Embrace these technologies to transform how you process and analyze large volumes of text, making your workflow more efficient and effective.
If you like this article, share it with others ♻️
Would help a lot ❤️
And feel free to follow me for articles more like this.
Subscribe to my newsletter
Read articles from Juan Carlos Olamendy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
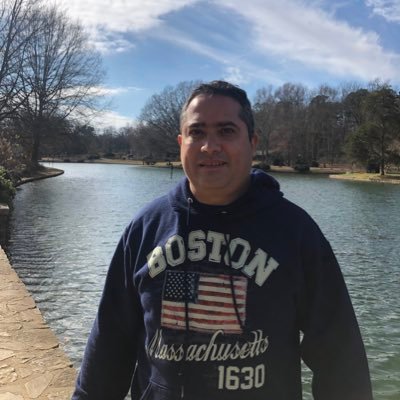
Juan Carlos Olamendy
Juan Carlos Olamendy
🤖 Talk about AI/ML · AI-preneur 🛠️ Build AI tools 🚀 Share my journey 𓀙 🔗 http://pixela.io