'this' Keyword in JS - Detailed Explanation

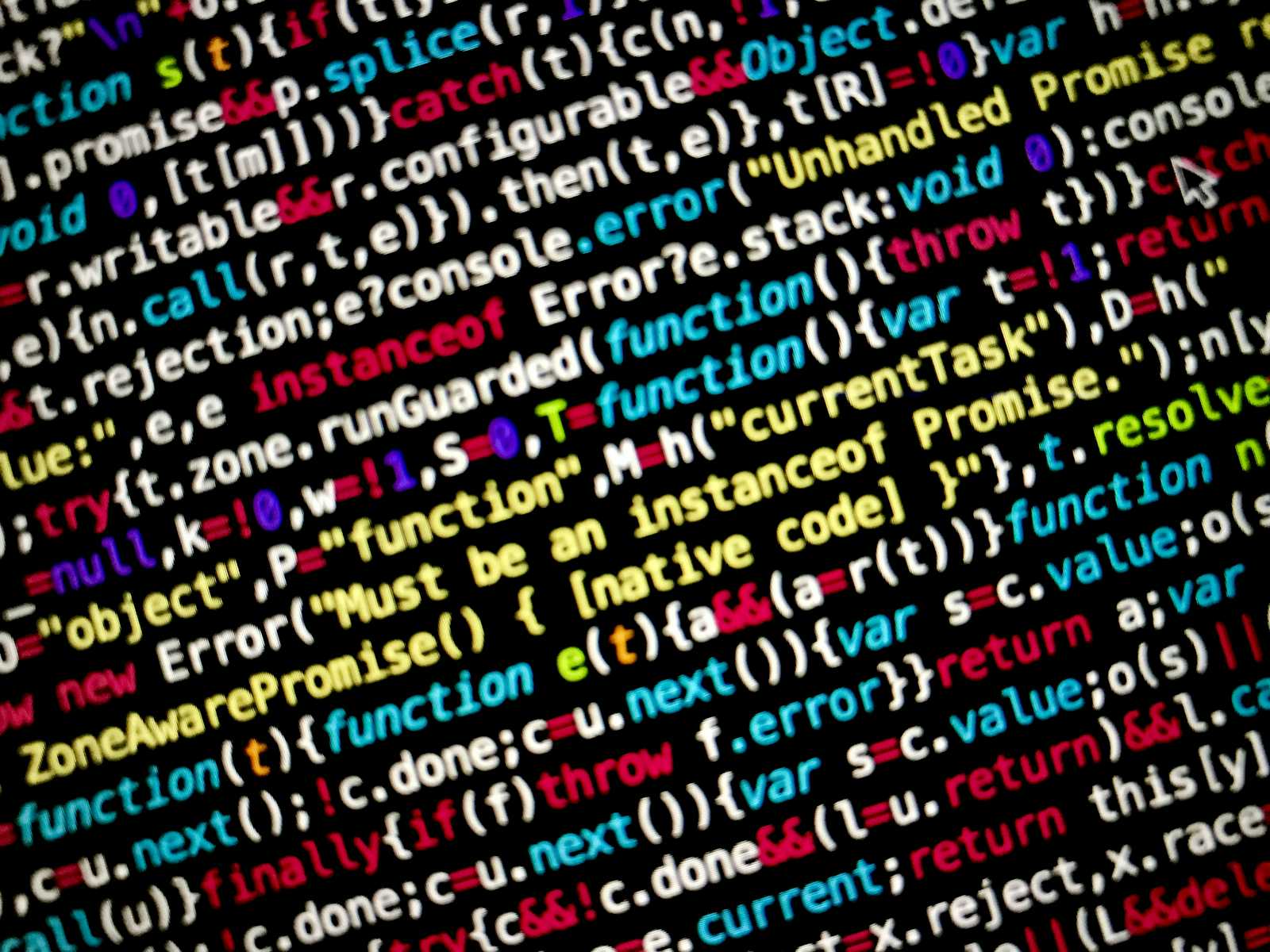
Understanding the 'this' Keyword in JavaScript
This Post contains examples and explanations of how this
keyword behaves in different contexts in JavaScript.
example: https://github.com/arunkumar201/JS-Practice/tree/Main/this-in-js
Table of Contents
Global Context
In the global execution context, this
refers to the global object:
In a browser, it's the
window
object.In Node.js, it's the
global
object.
console.log(this); // Window object in browser
console.log(this === window); // true in browser
Regular Function Context
In a regular function, this
behaves differently depending on strict mode:
In non-strict mode:
this
refers to the global object.In strict mode:
this
isundefined
.
function displayThis() {
console.log(this);
}
displayThis(); // Window (non-strict) or undefined (strict)
Object Method Context
When used inside an object's method, this
refers to the object itself:
const obj = {
name: "John",
greet: function() {
console.log(`Hello, my name is ${this.name}`);
}
};
obj.greet(); // "Hello, my name is John"
Arrow Function Context
Arrow functions don't have their own this
. They inherit this
from the enclosing scope:
const person = {
name: "Jack",
greet: () => {
console.log(this.name); // undefined (this refers to global object)
}
};
Constructor Context
In a constructor function, this
refers to the newly created instance:
function Student(name) {
this.name = name;
}
const student1 = new Student("John");
console.log(student1.name); // "John"
Event Handler Context
In an event handler, this
typically refers to the element that triggered the event:
button.addEventListener("click", function() {
console.log(this); // refers to the button element
});
Additional Notes
The behavior of
this
can be explicitly set usingcall()
,apply()
, orbind()
methods.Arrow functions, introduced in ES6, provide a way to lexically bind the
this
value, which can be useful in certain scenarios.
For more detailed explanations and examples, please refer to the source code in this repository.
GitHub: https://github.com/arunkumar201/JS-Practice/tree/Main/this-in-js
Subscribe to my newsletter
Read articles from Arun Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
