Accelerating Remote Sensing and GIS with GPUs: A Case Study in Climate Data Analytics

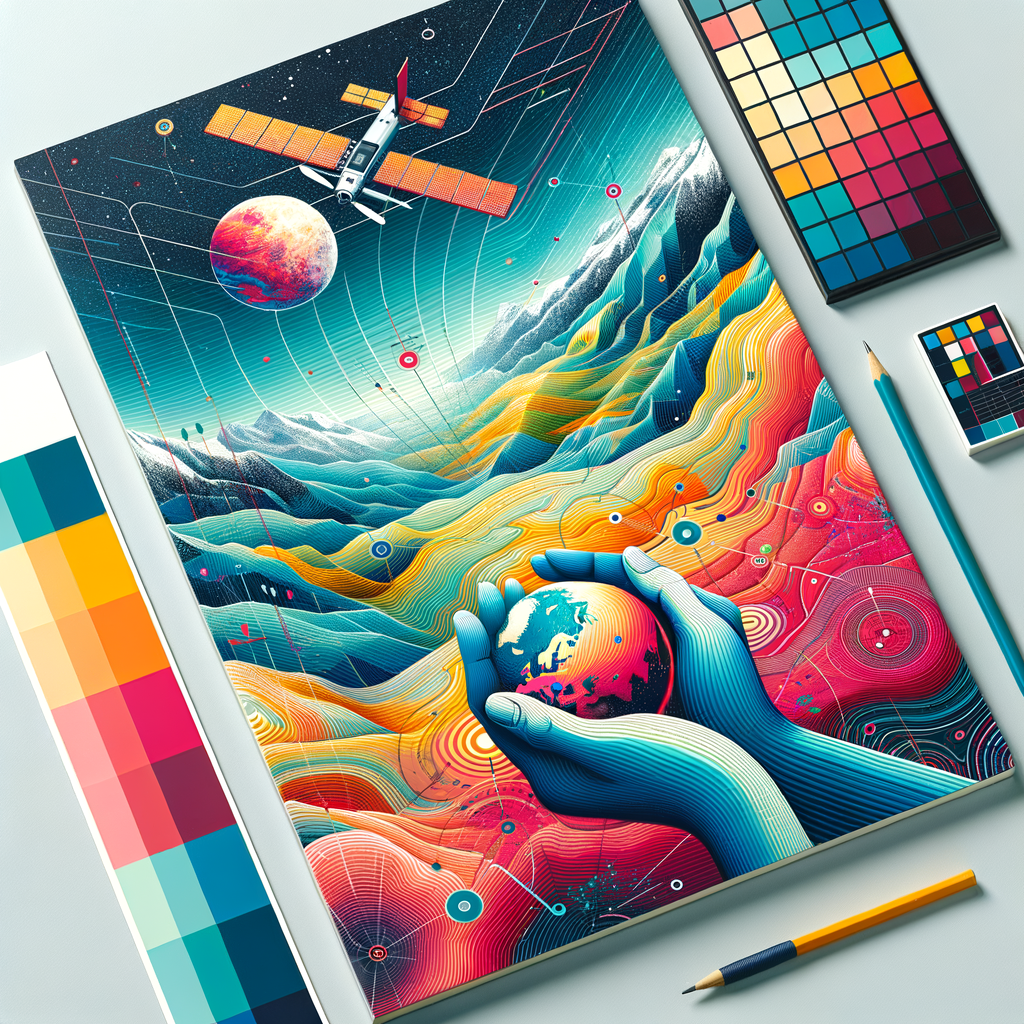
Ever since I became a part of the NASA’s PACE program, I am in awe of what potential hyperspectral earth observation can bring as value to humanity. Remote sensing and Geographic Information Systems (GIS) have transformed how we collect, visualize, and analyze environmental and climate data. With the massive amount of satellite imagery and climate datasets available, processing these large datasets can become computationally expensive and slow. Now, enter the GPU—a powerful tool for accelerating geospatial analysis and remote sensing operations. If we combine all the powers of observation, computing and human ingenuity, we have an extra-ordinary outcome. I seldom have come across GPUs being used for a use case on remote sensing, especially on Hyperspectral earth observation. Hence I have come up with this article.
Note: I have used GPT to help me improve and comment my code shared in this article here.
In this blog, we’ll dive into how GPUs can be leveraged to accelerate a remote sensing and GIS-based climate data analytics pipeline. The use case will focus on extracting and processing data from hyperspectral satellite images for monitoring climate-related phenomena, such as deforestation, urban heat islands, or crop health. We’ll write code snippets along the way, showing how GPUs can expedite the process.
Problem Statement: Monitoring Deforestation via Hyperspectral Remote Sensing
Let’s assume we’re working with a business that uses hyperspectral imagery to monitor deforestation trends. Deforestation, especially in tropical regions, is a significant contributor to climate change. Timely detection can help governments and conservation agencies intervene early. Hyperspectral sensors capture images in hundreds of narrow spectral bands, providing us with rich data to classify vegetation health and detect land cover changes.
However, processing hyperspectral images is computationally heavy due to the sheer volume of data and the complex models required to classify it. By using GPUs, we can drastically reduce processing times.
Step 1: Getting Satellite Imagery Data
For this example, we’ll use sample hyperspectral image datasets, which can be downloaded from NASA's AVIRIS (Airborne Visible/Infrared Imaging Spectrometer) mission or NASA’s PACE (Plankton, Aerosol, Cloud, ocean Ecosystem) satellite for climate data.
NASA Hyperspectral Data (AVIRIS): You can download AVIRIS hyperspectral imagery here.
PACE Satellite Data: PACE, launched by NASA, is crucial for understanding climate change, ocean ecology, and atmospheric composition. The data from PACE can be accessed here.
Here’s how to load a hyperspectral image using the Python rasterio
library:
import rasterio
import numpy as np
# Load hyperspectral image (assumed in GeoTIFF format)
hyperspectral_image_path = 'path_to_your_image.tif'
with rasterio.open(hyperspectral_image_path) as dataset:
hyperspectral_image = dataset.read()
# Inspect image dimensions
print(f"Image shape: {hyperspectral_image.shape}")
Typically, these images will have dimensions (bands, height, width)
, where each "band" is a specific spectral wavelength.
Step 2: Normalizing the Hyperspectral Data
Remote sensing data often requires preprocessing, such as normalization, before applying any analysis or classification. This is a great place to leverage GPU acceleration for large-scale data. Using cuPy
, a GPU-accelerated library with a NumPy-like API, we can speed up the process.
Here’s how to normalize each band of the hyperspectral image using cuPy
:
import cupy as cp
# Convert numpy array to GPU array using cuPy
hyperspectral_gpu = cp.asarray(hyperspectral_image)
# Normalize the data
def normalize(band):
return (band - cp.min(band)) / (cp.max(band) - cp.min(band))
# Normalize each spectral band
normalized_image = cp.array([normalize(hyperspectral_gpu[band, :, :]) for band in range(hyperspectral_gpu.shape[0])])
# Convert back to NumPy if needed
normalized_image_cpu = cp.asnumpy(normalized_image)
In this example, we load the hyperspectral image and normalize each band using GPU-accelerated operations. This preprocessing step can significantly speed up when applied to large-scale datasets compared to CPU-bound operations.
Step 3: Vegetation Index Calculation on GPU (NDVI)
One popular remote sensing technique is calculating vegetation indices such as the Normalized Difference Vegetation Index (NDVI). NDVI helps us measure the health of vegetation by comparing the red and near-infrared bands of an image.
For hyperspectral imagery, we can calculate NDVI using the red and NIR bands, which are typically at specific wavelengths. Let’s assume bands 30 and 60 correspond to the red and near-infrared bands, respectively.
Here’s how to calculate NDVI using cuPy
:
# Assume red and NIR bands correspond to index 30 and 60
red_band = normalized_image[30, :, :]
nir_band = normalized_image[60, :, :]
# Calculate NDVI: (NIR - Red) / (NIR + Red)
ndvi = (nir_band - red_band) / (nir_band + red_band)
# Clip NDVI values to the valid range [-1, 1]
ndvi_clipped = cp.clip(ndvi, -1, 1)
# Convert NDVI result back to CPU for visualization or further processing
ndvi_cpu = cp.asnumpy(ndvi_clipped)
Using the GPU allows us to compute NDVI much faster, especially when dealing with large images that can span several gigabytes in size.
Step 4: Classifying Deforested Areas Using a Pre-Trained Model
Let’s assume you have a pre-trained deep learning model for classifying deforested vs. non-deforested regions based on hyperspectral data. Using PyTorch
, we’ll load the model and perform inference on the GPU.
import torch
import torch.nn as nn
# Assume a pre-trained model for deforestation detection
class DeforestationModel(nn.Module):
def __init__(self):
super(DeforestationModel, self).__init__()
# Example model layers
self.conv1 = nn.Conv2d(10, 32, kernel_size=3, padding=1)
self.conv2 = nn.Conv2d(32, 64, kernel_size=3, padding=1)
self.fc1 = nn.Linear(64 * 16 * 16, 2) # Binary classification (deforested/non-deforested)
def forward(self, x):
x = torch.relu(self.conv1(x))
x = torch.relu(self.conv2(x))
x = torch.flatten(x, 1)
x = self.fc1(x)
return x
# Load the model and move to GPU
model = DeforestationModel().cuda()
model.load_state_dict(torch.load('pretrained_deforestation_model.pth'))
# Assume we want to classify a patch of the hyperspectral image
# For simplicity, we're extracting the first 10 bands
image_patch = normalized_image_cpu[:10, :256, :256] # First 10 bands, patch size (256x256)
image_patch = torch.from_numpy(image_patch).unsqueeze(0).cuda() # Convert to tensor and move to GPU
# Perform inference
with torch.no_grad():
output = model(image_patch)
prediction = torch.argmax(output, dim=1).item()
print(f"Prediction: {'Deforested' if prediction == 1 else 'Not Deforested'}")
This example demonstrates how you can quickly run inference on the GPU for climate-related applications such as deforestation detection.
Step 5: Visualizing Results with GIS
Now that we’ve processed the data and run the model, it’s time to visualize the results. We can use the folium
library to create interactive maps displaying the classified regions.
import folium
import numpy as np
# Convert NDVI array to a grid for folium (assuming it's smaller for visualization)
ndvi_grid = np.random.random((100, 100)) # Replace with actual NDVI data, resized for demo purposes
# Create a folium map
m = folium.Map(location=[-15.793889, -47.882778], zoom_start=4) # Example coordinates (Brazil)
# Add NDVI grid to map
folium.raster_layers.ImageOverlay(
image=ndvi_grid,
bounds=[[-10.0, -60.0], [-5.0, -55.0]],
colormap=lambda x: (1, x, 1-x, 1) # Example colormap
).add_to(m)
# Display the map
m.save('deforestation_map.html')
In this step, we visualize the NDVI and deforestation results on an interactive map. You can modify this script to overlay classified regions for a clearer understanding of where deforestation is occurring.
Using GPUs for remote sensing and GIS applications allows businesses and organizations to process large-scale climate and environmental data efficiently. In this blog, we demonstrated how GPU acceleration can be applied to tasks like hyperspectral image normalization, vegetation index calculation, and deforestation classification using deep learning models.
From normalizing large hyperspectral images to running deep learning models, GPU acceleration is crucial for efficiently handling the computational load in climate-based use cases. Whether you’re building a business around deforestation detection, urban heat mapping, or agricultural monitoring, GPUs can take your pipeline from slow to scalable.
This is a small example on how GPUs can speed up a lot of work. I haven’t put the visualization outputs here yet but I will update them in the upcoming blog.
Interested in building climate or environment-based solutions with GPUs? Let’s discuss more! Feel free to share your own use cases and experiences below!
Now with the addition of NASA's AVIRIS hyperspectral data and [PACE satellite data](https://pace.gsfc.nasa.gov/data.html), you have real-world datasets to start with for building climate and environmental monitoring applications.
Subscribe to my newsletter
Read articles from Madhusudhan Anand directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Madhusudhan Anand
Madhusudhan Anand
Embarking on a Journey: Unveiling My Passions and Pursuits Greetings from Bangalore, India! My name is Madhusudhan Anand, and life has been a beautiful ride of experiences and challenges. Growing up, my family's nomadic nature led us to traverse various cities in Karnataka, immersing me in the rich tapestry of diverse cultures. These encounters have left an indelible mark on my journey, shaping my passions across four distinctive realms: product development, teaching, problem-solving, and writing. As the co-founder of Ambee, a vibrant climate tech startup, my forte is transforming promising ideas into tangible, revenue-generating products. I channel my creative energy, technical expertise, and entrepreneurial spirit with every project to make a meaningful impact. Teaching has become more than just a hobby—it has become a way for me to ignite a spark of knowledge and inspiration in others. Over the years, I've had the privilege of mentoring and training over 2000 programmers worldwide. Sharing my insights and empowering aspiring talents in the world of data science and programming has been a profitable endeavor. Problem-solving is the fuel that drives my passion. With an optimistic and multidimensional perspective, I approach every challenge as an opportunity for growth. From my roots in data science and remote sensing to exploring the realms of climate change, IoT, and AI, I've harnessed my problem-solving prowess to create innovative products at Ambee. Writing has always been my sanctuary—an avenue to channel my thoughts, emotions, and ideas. I am captivated by the power of the written word to inspire, educate, and connect. Through my blog, I promise to deliver authentic, informative, and infused content with my personal touch. I'll share insights from my journey, staying true to my values and unwavering commitment to honesty. As I embark on this blogging adventure, I dedicate this platform to my late father, a constant source of inspiration and strength. His memory will forever reside in my heart, guiding me to be true to myself and positively impact the world. Join me on this exhilarating journey of exploration, learning, and growth. Let's delve into the fascinating realms of technology, data science, and personal reflections. Welcome to my world!