How I Manage Django Settings
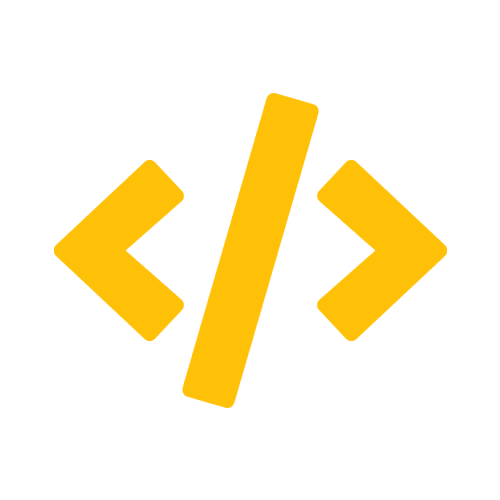
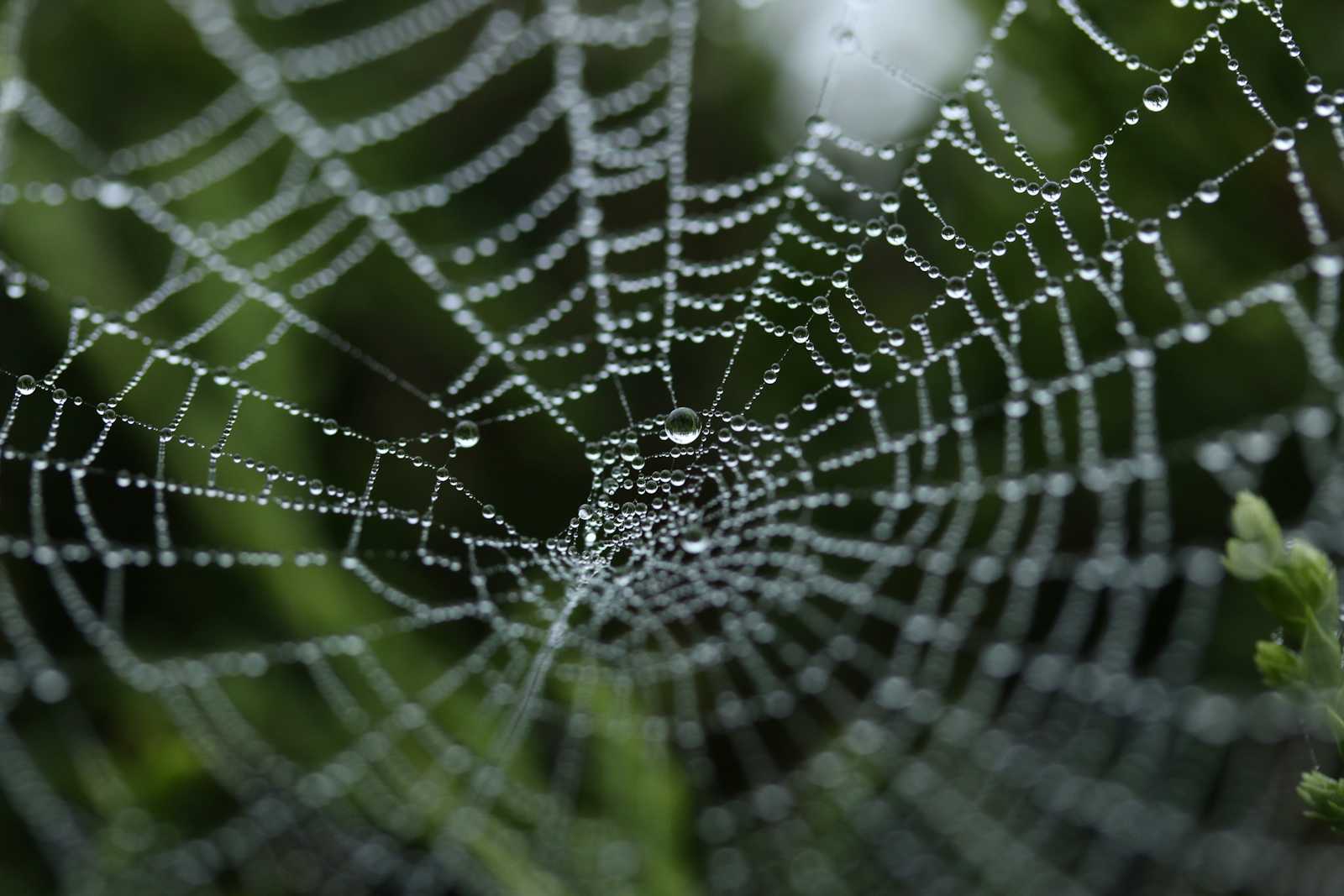
Building a Django app is fun until you realize you need different settings for development and production. One day, I accidentally shared my secret keys with the whole internet. Oops.
In this post, I’ll show you how I manage Django settings. It's a lifesaver when you have to deal with different environments.
Why Bother with Django Settings?
Imagine working on your app locally, everything looks perfect. Then you push it to production, and boom! Something breaks. Maybe you left debug mode on, or worse, exposed all your secrets.
Managing settings is like having different outfits for different occasions. You wouldn’t wear pajamas to a job interview, right? Same with Django, your settings should change depending on where you’re running your app.
Using Environment Variables: Keep Your Secrets Hidden
You don’t want your secret keys, database passwords, or API keys lying around in your code for everyone to see. Trust me, it's not a good look.
I use python-decouple
to handle this. It helps me load environment variables and keep my settings clean.
First, install it:
pip install python-decouple
Now, in settings.py
, grab your environment variables:
# settings.py
from decouple import config
SECRET_KEY = config('SECRET_KEY')
DEBUG = config('DEBUG', cast=bool)
Store the real secrets in a .env
file. Don’t forget to add this file to your .gitignore
. You don’t want to share your secrets like I did.
# .env
SECRET_KEY='supersecretkey'
DEBUG=True
Congrats! Now, your sensitive data is safe from prying eyes.
Handling Multiple Environments (Because One is Never Enough)
You probably have different setups for development and production. In development, you might use SQLite. But in production, you need something fancier like PostgreSQL.
Here’s how I handle multiple environments without losing my sanity.
Step 1: Split Settings
First, I split my settings into three files:
base.py
for shared settings.development.py
for local work.production.py
for the big leagues (aka production).
It looks something like this:
/project
├── settings/
│ ├── __init__.py
│ ├── base.py
│ ├── development.py
│ └── production.py
Step 2: Common Settings in base.py
In base.py
, I put everything that’s the same for all environments.
# settings/base.py
from decouple import config
INSTALLED_APPS = [
# Your apps here
]
SECRET_KEY = config('SECRET_KEY')
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': config('DB_NAME', default='db.sqlite3'),
}
}
Step 3: Custom Settings for Each Environment
In development.py
, I set up things like debugging and using SQLite.
# settings/development.py
from .base import *
DEBUG = True
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': config('DB_NAME', default='db.sqlite3'),
}
}
In production.py
, I switch to PostgreSQL and turn off debugging. No need to let the world see your errors!
# settings/production.py
from .base import *
DEBUG = False
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.postgresql',
'NAME': config('DB_NAME'),
'USER': config('DB_USER'),
'PASSWORD': config('DB_PASSWORD'),
}
}
Step 4: Load the Right Settings Automatically
In settings/__init__.py
, I decide which settings to use based on an environment variable. Fancy, huh?
# settings/__init__.py
from decouple import config
ENV = config('DJANGO_ENV', default='development')
if ENV == 'production':
from .production import *
else:
from .development import *
In your .env
, you can set the environment like this:
# .env
DJANGO_ENV='development' # Or 'production'
Now, when you deploy, Django will use the right settings without any extra effort from you.
Static and Media Files: The Pesky Duo
Managing static and media files in different environments can be annoying. Locally, I just serve them from my app. In production, I need something more serious like AWS S3.
For development:
# settings/development.py
STATIC_URL = '/static/'
MEDIA_URL = '/media/'
For production:
# settings/production.py
STATIC_URL = '/static/'
STATIC_ROOT = '/path/to/static/'
MEDIA_URL = '/media/'
MEDIA_ROOT = '/path/to/media/'
Now, my files work everywhere without me worrying about broken images or missing styles.
Keep Secrets Out of Git (Seriously)
Make sure your .env
file is in .gitignore
. I learned this the hard way. Once, I committed my secret keys to GitHub, and bots immediately started sniffing around my repo.
# .gitignore
.env
Don’t be like me. Keep your secrets secret.
Subscribe to my newsletter
Read articles from Arjun Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
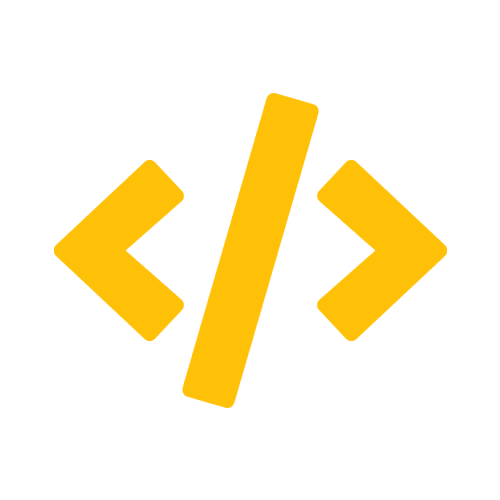