Mastering CORS in JavaScript: A Comprehensive Guide for Frontend Developers
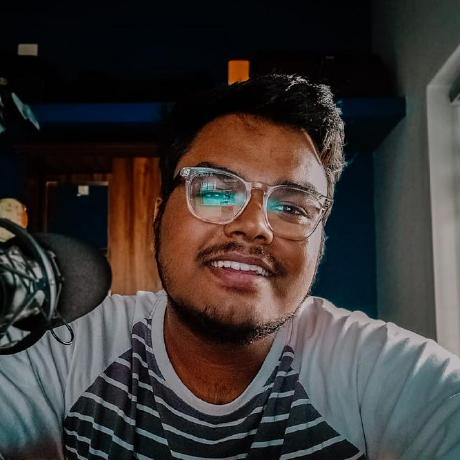
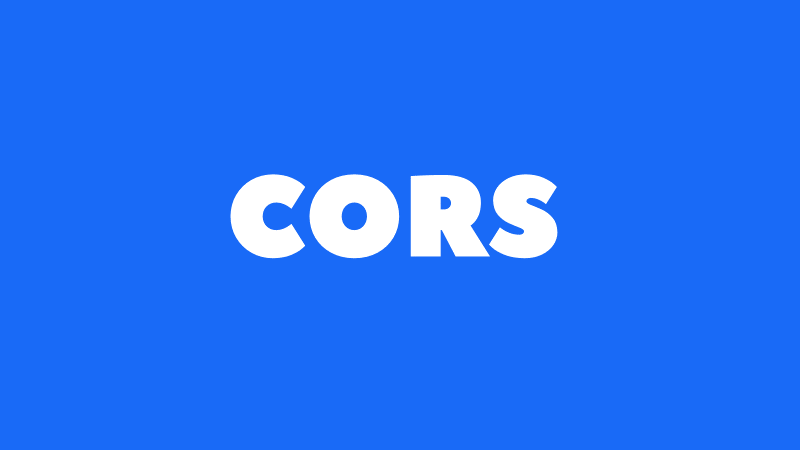
When developing web applications, security is paramount, especially when it comes to handling cross-origin requests. Browsers implement several security mechanisms to protect users from potential attacks like Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF). One of the key security features browsers use is Cross-Origin Resource Sharing (CORS). This blog will delve into how CORS works, the role of preflight requests, and the significance of the HTTP HEAD
method.
What is CORS?
Cross-Origin Resource Sharing (CORS) is a security feature implemented by browsers to restrict web pages from making requests to a different domain than the one that served the web page. This helps prevent malicious activities by enforcing a same-origin policy, where resources on a web page can only interact with resources from the same origin.
How Does CORS Work?
When a browser makes a cross-origin request, it needs to ensure that the request is safe and allowed by the server. Here's how the process works:
Preflight Request: Before making the actual cross-origin request, the browser sends an HTTP
OPTIONS
request, known as a preflight request. This request checks if the server allows the cross-origin request.Server Response: If the server permits the request, it responds with specific CORS headers such as
Access-Control-Allow-Origin
,Access-Control-Allow-Methods
, andAccess-Control-Allow-Headers
.Actual Request: After receiving the appropriate response from the server, the browser proceeds with the actual request.
This process ensures that the server explicitly allows the cross-origin request, protecting users from potential security risks.
What are Preflight Requests?
A preflight request is an HTTP OPTIONS
request sent by the browser before the actual request. Its purpose is to determine if the actual request is safe to send. The preflight request checks whether the server allows the requested method and headers.
Request Headers:
Origin
: Indicates the origin of the request.Access-Control-Request-Method
: Used in preflight requests to indicate the HTTP method for the actual request.Access-Control-Request-Headers
: Lists the headers that will be used in the actual request.
Response Headers:
Access-Control-Allow-Origin
: Specifies the allowed origin.Access-Control-Allow-Methods
: Lists the allowed HTTP methods.Access-Control-Allow-Headers
: Specifies the allowed headers.Access-Control-Allow-Credentials
: Indicates whether the request can include user credentials like cookies.Access-Control-Max-Age
: Specifies how long the results of the preflight request can be cached.
When are Preflight Requests Sent?
Preflight requests are typically sent under the following conditions:
Non-Simple Requests: If the request uses methods other than
GET
,POST
, orHEAD
, or includes custom headers, a preflight request is sent to check if these are allowed.With-Credentials Requests: When sending credentials (e.g., cookies or HTTP authentication), the browser sends a preflight request to verify that the server allows credentials to be included in the request.
Caching of Preflight Requests
To improve performance, the results of a preflight request can be cached by the browser using the Access-Control-Max-Age
header. This header indicates the number of seconds the preflight response can be cached. During this time, the browser won't send a preflight request for subsequent requests to the same resource.
The HEAD
Method in HTTP
The HTTP HEAD
method is a useful tool in web development, especially when you need to fetch metadata about a resource without downloading the entire content. It is similar to the GET
method, but the server only returns the status line and headers, without the response body.
Uses of the HEAD
Method
Checking Resource Availability: The
HEAD
method can be used to verify if a resource exists without downloading it. This is particularly useful for checking the existence of a file or endpoint.Fetching Metadata: You can use the
HEAD
method to retrieve metadata like content type, content length, and last modified date, without fetching the resource itself.Testing Links: Web crawlers and link checkers often use the
HEAD
method to verify the validity of links without downloading the linked resources.Performance Monitoring: Monitoring tools can use
HEAD
requests to check the status and performance of a server or resource.Conditional Requests: The
HEAD
method can determine if a resource has changed by examining headers likeETag
orLast-Modified
, helping clients decide whether to fetch the resource again or use a cached version.
Example: Using the HEAD
Method
fetch('https://example.com/resource', {
method: 'HEAD'
})
.then(response => {
if (response.ok) {
console.log('Resource exists');
console.log('Content-Length:', response.headers.get('Content-Length'));
console.log('Last-Modified:', response.headers.get('Last-Modified'));
} else {
console.log('Resource does not exist');
}
})
.catch(error => console.error('Error:', error));
In this example, a HEAD
request is sent to check if a resource exists on the server. If the resource is available, the metadata like Content-Length
and Last-Modified
are logged to the console.
Conclusion
Understanding CORS, preflight requests, and the HEAD
method is crucial for frontend developers, especially when dealing with cross-origin requests and server resources. These concepts not only help enhance security but also improve the performance and reliability of web applications. By mastering these tools, you'll be better prepared for frontend developer interviews and equipped to build more secure and efficient web applications.
Follow Me on Social Media:
Linkedin: https://www.linkedin.com/in/nikhil-nair27/
GitHub: https://github.com/NIKHILNAIR21
Subscribe to my newsletter
Read articles from NiKHIL NAIR directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
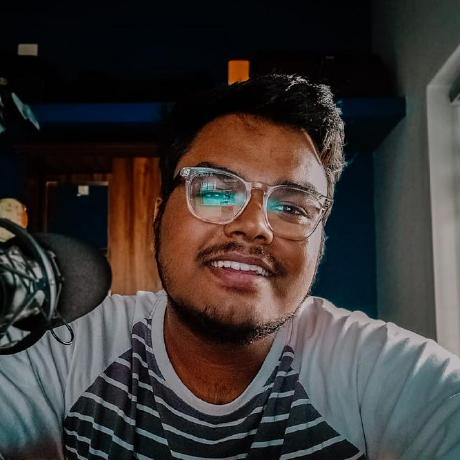