How to Handle File Uploads in JavaScript: A Detailed Guide with Examples
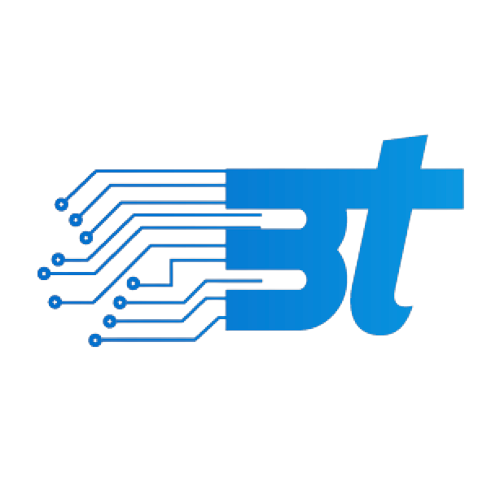
Table of contents
- 1. Setting Up a Basic File Upload Form in HTML
- Explanation:
- 2. Handling File Uploads with JavaScript
- Explanation:
- 3. Validating Files on the Client Side
- Explanation:
- 4. Uploading Files to the Server Using Fetch
- Key Points:
- 5. Handling File Uploads on the Server (Node.js Example)
- Server Code (Node.js with Express)
- Explanation:
- 6. Enhancing User Experience with Progress Indicators
- Explanation:
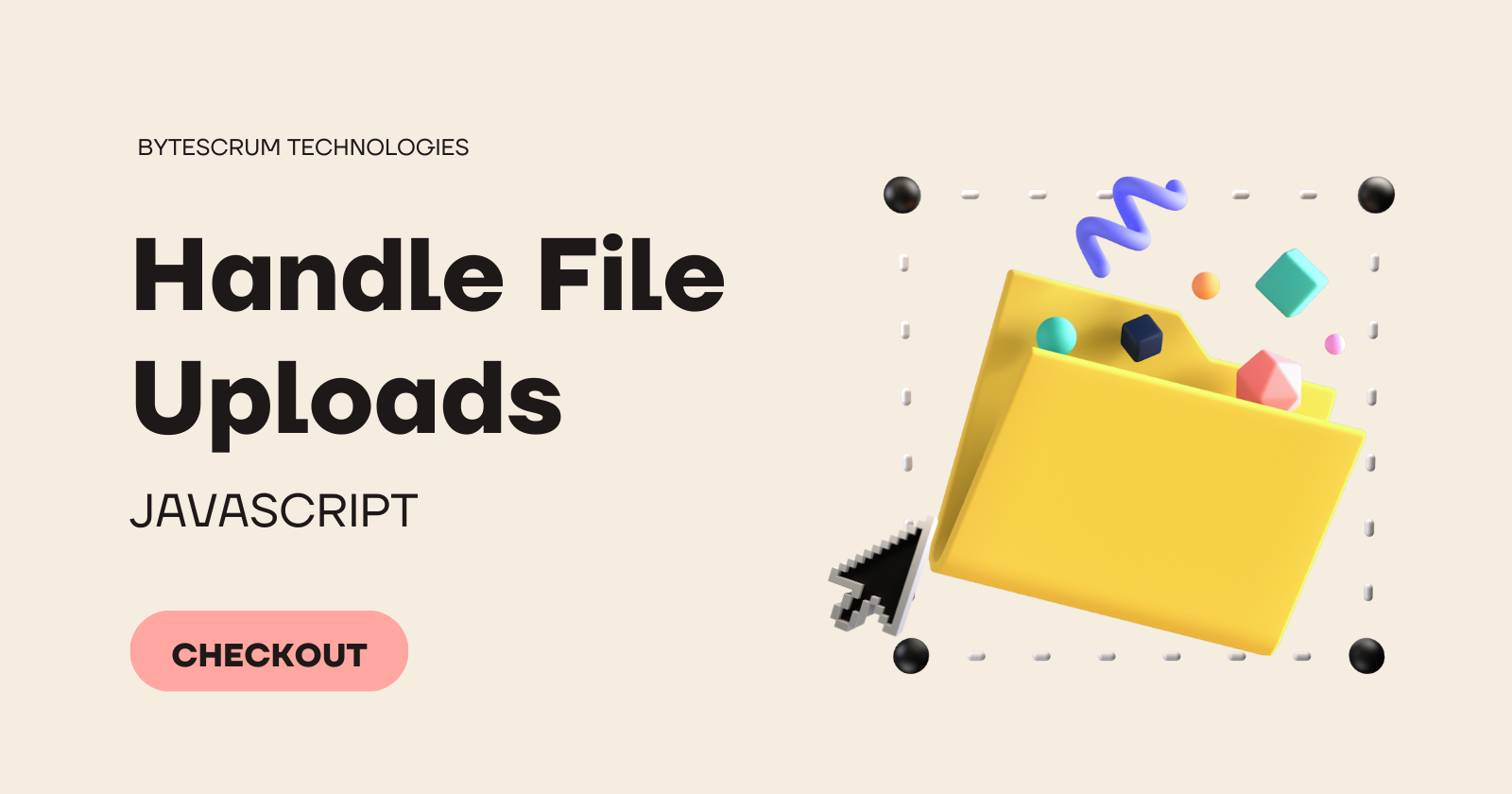
File uploads are a crucial part of many web applications, whether it's for user profiles, documents, or images. With JavaScript, you can create a seamless and efficient file upload experience for your users. This comprehensive guide will take you through the process of handling file uploads in JavaScript, from creating a simple HTML form to processing files on the server.
1. Setting Up a Basic File Upload Form in HTML
We’ll start by creating a basic HTML form where users can select and upload a file.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>File Upload Example</title>
</head>
<body>
<h1>Upload a File</h1>
<form id="uploadForm">
<label for="fileInput">Choose a file:</label>
<input type="file" id="fileInput" name="file" required>
<button type="submit">Upload</button>
</form>
<p id="uploadStatus"></p>
<script src="app.js"></script>
</body>
</html>
Explanation:
The
input
element withtype="file"
allows the user to select a file from their device.The form will be managed via JavaScript to handle the upload process.
2. Handling File Uploads with JavaScript
Next, we'll write JavaScript to handle the file submission, prevent the default form submission behavior, and manage the file upload via AJAX.
document.getElementById('uploadForm').addEventListener('submit', function(event) {
event.preventDefault(); // Prevent the default form submission
const fileInput = document.getElementById('fileInput');
const file = fileInput.files[0];
if (!file) {
alert('Please choose a file!');
return;
}
const formData = new FormData();
formData.append('file', file);
uploadFile(formData);
});
async function uploadFile(formData) {
try {
const response = await fetch('/upload', {
method: 'POST',
body: formData
});
if (response.ok) {
document.getElementById('uploadStatus').textContent = 'File uploaded successfully!';
} else {
document.getElementById('uploadStatus').textContent = 'Upload failed.';
}
} catch (error) {
console.error('Error:', error);
document.getElementById('uploadStatus').textContent = 'An error occurred while uploading the file.';
}
}
Explanation:
We use
FormData
to encapsulate the file data in a format suitable for sending to the server.The
fetch
API is used to send the file to the server asynchronously.The script updates the
uploadStatus
text depending on whether the upload succeeds or fails.
3. Validating Files on the Client Side
Before uploading files, it’s important to validate them. We can check file size, type, or even limit the number of files.
if (file.size > 2 * 1024 * 1024) { // Limit file size to 2MB
alert('File size exceeds 2MB.');
return;
}
const validTypes = ['image/jpeg', 'image/png', 'application/pdf'];
if (!validTypes.includes(file.type)) {
alert('Only JPEG, PNG, and PDF files are allowed.');
return;
}
Explanation:
This checks the file size, ensuring it’s below 2MB.
We also verify that the file type is either a JPEG, PNG, or PDF.
4. Uploading Files to the Server Using Fetch
When the file passes validation, we send it to the server. The Fetch API simplifies handling file uploads. Here’s the uploadFile
function for reference:
async function uploadFile(formData) {
try {
const response = await fetch('/upload', {
method: 'POST',
body: formData
});
if (response.ok) {
document.getElementById('uploadStatus').textContent = 'File uploaded successfully!';
} else {
document.getElementById('uploadStatus').textContent = 'Upload failed.';
}
} catch (error) {
console.error('Error:', error);
document.getElementById('uploadStatus').textContent = 'An error occurred during the upload.';
}
}
Key Points:
- We send the file via
POST
request usingfetch
, attaching theFormData
object as the request body.
5. Handling File Uploads on the Server (Node.js Example)
Once the file reaches the server, it needs to be handled appropriately. In this example, we’ll use express
and multer
in Node.js to handle file uploads.
Server Code (Node.js with Express)
const express = require('express');
const multer = require('multer');
const path = require('path');
const app = express();
const upload = multer({ dest: 'uploads/' }); // Directory where files are stored
app.post('/upload', upload.single('file'), (req, res) => {
if (!req.file) {
return res.status(400).send('No file uploaded.');
}
res.send('File uploaded successfully!');
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
Explanation:
Multer: This middleware simplifies handling multipart/form-data, which is primarily used for file uploads.
upload.single('file')
: Handles a single file upload, matching the name of the file input element in HTML.
6. Enhancing User Experience with Progress Indicators
To further improve the user experience, you can add a progress indicator showing the upload progress. The XMLHttpRequest
object provides an event progress
that can be used to monitor upload progress.
function uploadFileWithProgress(formData) {
const xhr = new XMLHttpRequest();
xhr.open('POST', '/upload', true);
xhr.upload.onprogress = function(event) {
if (event.lengthComputable) {
const percentComplete = (event.loaded / event.total) * 100;
document.getElementById('uploadStatus').textContent = `Upload progress: ${Math.round(percentComplete)}%`;
}
};
xhr.onload = function() {
if (xhr.status === 200) {
document.getElementById('uploadStatus').textContent = 'File uploaded successfully!';
} else {
document.getElementById('uploadStatus').textContent = 'File upload failed.';
}
};
xhr.send(formData);
}
Explanation:
- The
xhr.upload.onprogress
event tracks the progress of the upload and updates the status text.
Conclusion
By following this guide, you now have a solid foundation to implement and improve file uploads in your projects.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
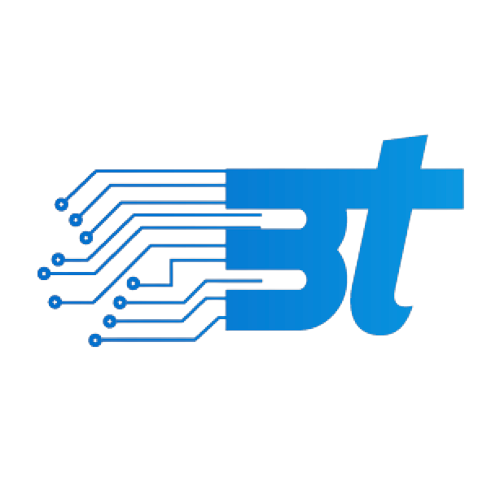
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.