Mastering State Management in Flutter: A Comprehensive Guide

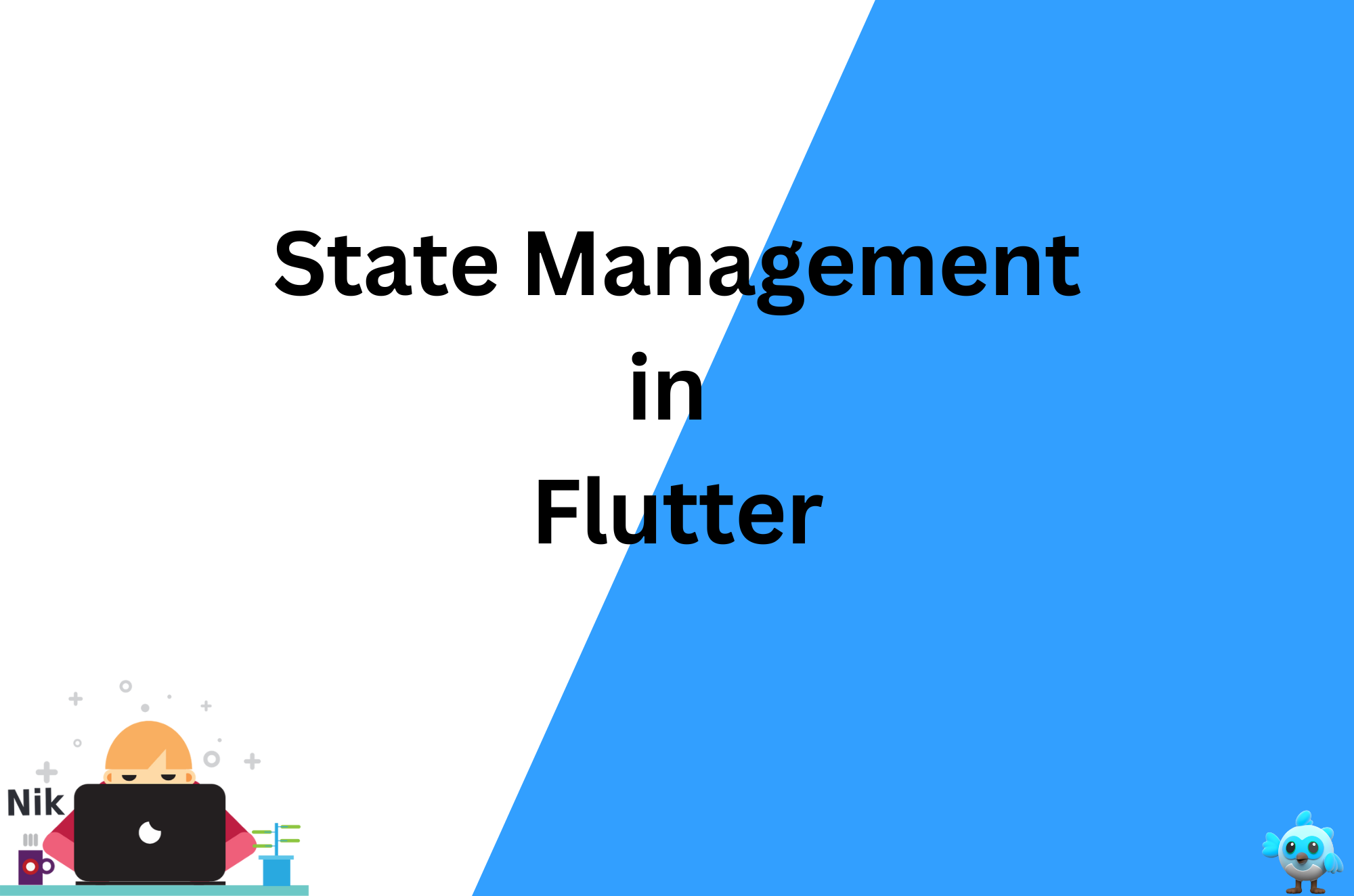
Flutter has revolutionized the way we build cross-platform applications with its powerful and expressive framework. One of the most critical aspects of building a robust Flutter application is managing state effectively. In this blog, we'll dive into state management in Flutter, exploring various approaches, their benefits, and when to use each.
Understanding State Management
State management refers to the way an application handles and maintains the state of its user interface and data. In Flutter, managing state efficiently is crucial for creating responsive and interactive applications. Poor state management can lead to complex code, performance issues, and a less maintainable codebase.
Popular State Management Solutions in Flutter
Flutter offers several state management solutions, each with its strengths and use cases. Let's explore the most popular ones:
1. setState
setState
is the simplest and most straightforward way to manage state in Flutter. Itβs built into Flutter and is ideal for managing state within a single widget.
Pros:
Simple to use.
Ideal for small, localized state management.
Cons:
Can become unwieldy for complex state or larger applications.
Not suitable for managing state across multiple widgets or screens.
Usage Example:
dartCopy codeclass CounterWidget extends StatefulWidget {
@override
_CounterWidgetState createState() => _CounterWidgetState();
}
class _CounterWidgetState extends State<CounterWidget> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Column(
children: <Widget>[
Text('Counter: $_counter'),
ElevatedButton(
onPressed: _incrementCounter,
child: Text('Increment'),
),
],
);
}
}
2. Provider
Provider is a popular and widely-used state management package that simplifies the process of managing state in Flutter applications. It is based on the InheritedWidget and is well-suited for medium to large-scale applications.
Pros:
Easy to understand and use.
Scales well for larger applications.
Provides support for dependency injection.
Cons:
- Can become complex with deeply nested widgets.
Usage Example:
dartCopy codeimport 'package:flutter/material.dart';
import 'package:provider/provider.dart';
void main() {
runApp(
ChangeNotifierProvider(
create: (context) => CounterModel(),
child: MyApp(),
),
);
}
class CounterModel with ChangeNotifier {
int _counter = 0;
int get counter => _counter;
void increment() {
_counter++;
notifyListeners();
}
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Provider Example')),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Consumer<CounterModel>(
builder: (context, counterModel, child) => Text(
'Counter: ${counterModel.counter}',
),
),
ElevatedButton(
onPressed: () => context.read<CounterModel>().increment(),
child: Text('Increment'),
),
],
),
),
),
);
}
}
3. Riverpod
Riverpod is a newer state management solution that builds upon the concepts of Provider but aims to address some of its limitations. It offers a more flexible and robust approach to state management.
Pros:
More flexible and modular.
Better testability.
Improves performance with lazy loading.
Cons:
- Slightly steeper learning curve compared to Provider.
Usage Example:
dartCopy codeimport 'package:flutter/material.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
final counterProvider = StateProvider<int>((ref) => 0);
void main() {
runApp(
ProviderScope(
child: MyApp(),
),
);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text('Riverpod Example')),
body: Center(
child: Consumer(
builder: (context, watch, child) {
final counter = watch(counterProvider).state;
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Counter: $counter'),
ElevatedButton(
onPressed: () => context.read(counterProvider).state++,
child: Text('Increment'),
),
],
);
},
),
),
),
);
}
}
4. Bloc
BLoC (Business Logic Component) is a powerful state management solution that helps in separating business logic from UI code. Itβs particularly useful for applications that require complex state management and asynchronous data handling.
Pros:
Clear separation of business logic and UI.
Better for complex applications with multiple streams of data.
Cons:
- Can be verbose and have a steeper learning curve.
Usage Example:
dartCopy codeimport 'package:flutter/material.dart';
import 'package:flutter_bloc/flutter_bloc.dart';
void main() {
runApp(MyApp());
}
class CounterCubit extends Cubit<int> {
CounterCubit() : super(0);
void increment() => emit(state + 1);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: BlocProvider(
create: (_) => CounterCubit(),
child: Scaffold(
appBar: AppBar(title: Text('BLoC Example')),
body: Center(
child: BlocBuilder<CounterCubit, int>(
builder: (context, count) {
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text('Counter: $count'),
ElevatedButton(
onPressed: () => context.read<CounterCubit>().increment(),
child: Text('Increment'),
),
],
);
},
),
),
),
),
);
}
}
Choosing the Right Solution
The right state management solution for your Flutter application depends on several factors, including the complexity of your application, your team's familiarity with the solution, and the specific needs of your project. For simple applications or small projects, setState might be sufficient. For medium to large applications, Provider or Riverpod could be more appropriate, while BLoC is ideal for applications with complex state requirements.
Conclusion
Effective state management is crucial for building scalable and maintainable Flutter applications. Understanding the different state management solutions and their use cases will help you make informed decisions and create better applications. Experiment with these approaches and find the one that best suits your project's needs.
Happy coding!
Subscribe to my newsletter
Read articles from Ravi Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ravi Patel
Ravi Patel
"π± Passionate Flutter Developer with 6+ years of experience, dedicated to crafting exceptional mobile applications. π Adept at turning ideas into polished, user-friendly experiences using Flutter's magic. π¨ Design enthusiast who believes in the power of aesthetics and functionality. π οΈ Expertise in translating complex requirements into clean, efficient code. π Committed to staying updated with the latest trends and continuously pushing boundaries. Let's create something extraordinary together!"