How URL Shorteners Work & How to Build One with Server-Side Rendering using Express and EJS
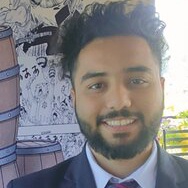
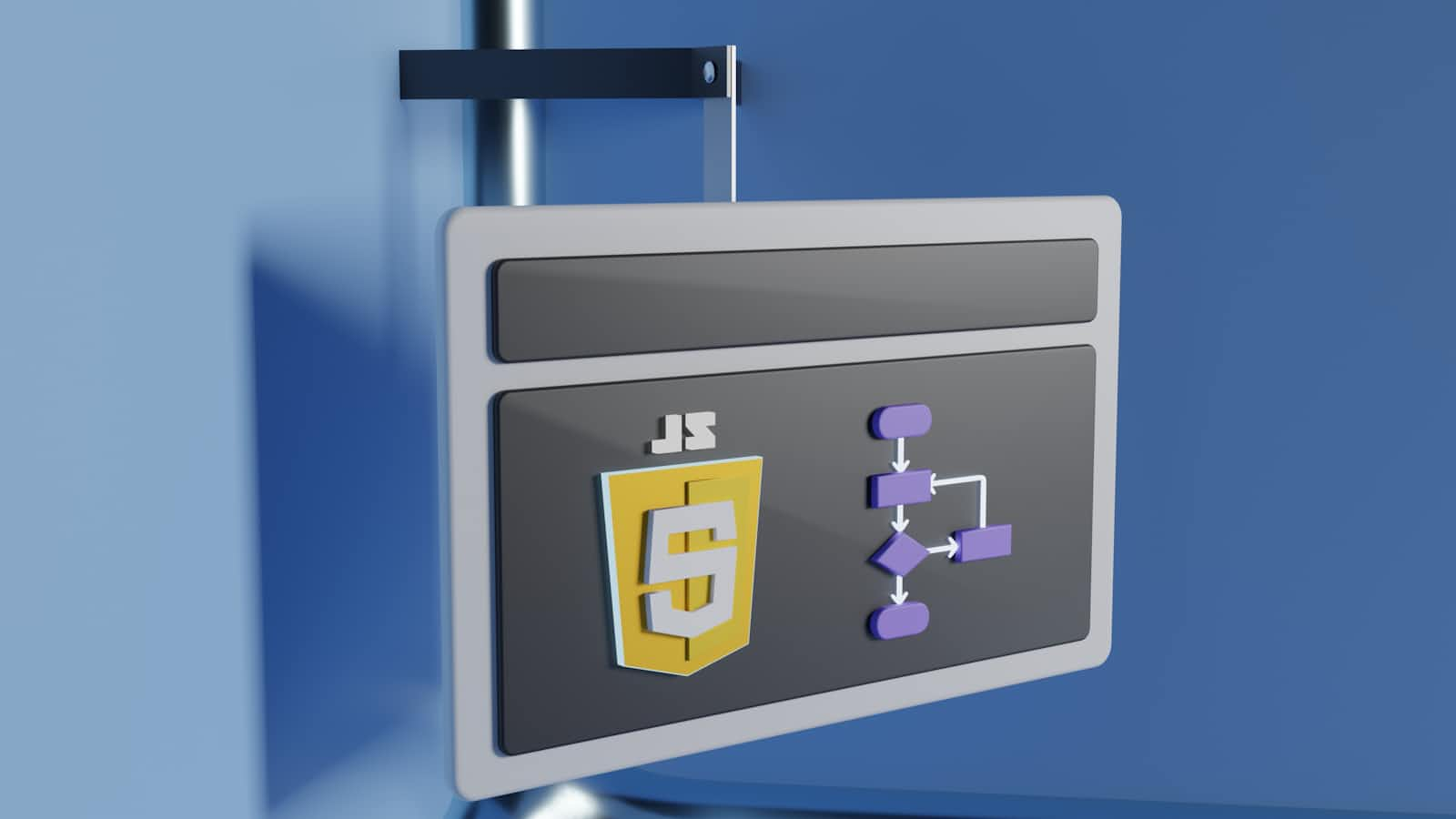
Introduction:
Have you ever wondered how those tiny URLs work behind the scenes? Whether you're sharing links on social media or simplifying a long link for your users, URL shorteners are super handy. In this blog post, we’ll walk through how URL shorteners work and how to build one from scratch using Node.js, Express, and MongoDB. But that’s not all—we’ll also explore how to enhance this with server-side rendering (SSR) using EJS to create a dynamic front end!
How Do URL Shorteners Work?
A URL shortener maps a long URL to a shorter one and stores that mapping in a database. When someone visits the short URL, the server looks up the original long URL and redirects the user to it. It’s that simple! Let’s break it down:
Database: Stores the long URL and its corresponding short URL.
Hashing: Generates a unique, shortened string (a short URL) from the long URL.
Routing: When the short URL is clicked, the app looks up the long URL and redirects the user to it.
Core Features of a URL Shortener
Short URL Creation: Takes a long URL, generates a short URL, and saves it in a database.
Redirection: When the short URL is visited, the server finds the original URL and redirects the user.
How to Build a URL Shortener
Let’s dive into building a URL shortener step-by-step.
Step 1: Setting up a Backend with Node.js and Express
To begin, we’ll create a basic Express app. Our backend will handle two primary routes:
One for shortening a URL (
POST /shorten
)Another for redirecting users based on the short URL (
GET /:shortId
)
Step 2: Adding Server-Side Rendering with EJS
At this point, our URL shortener works perfectly with just the backend. However, to make it user-friendly, we’ll add a front end using server-side rendering (SSR) with EJS (Embedded JavaScript
What is Server-Side Rendering?
SSR involves rendering HTML on the server and sending the fully-formed page to the browser. With SSR, we can create dynamic and interactive pages where users can input URLs, generate short links, and view the stats for their links
Why Use EJS?
EJS is a simple templating engine that integrates smoothly with Express. It allows us to embed JavaScript logic directly into our HTML and dynamically render content like the generated short URLs.
Step 3: Setting Up EJS and Express
First, install EJS and set it as the view engine for your Express app:
npm install ejs
Step 4: Creating a Dynamic Homepage with EJS
Now let’s create a view file (home.ejs
) that serves as the main page. Users will input their long URLs here, generate short URLs, and view stats.
Here’s the content of views/home.ejs
:
Step 5: Handling Form Submissions and Rendering Dynamic Data
When the form is submitted, the app will generate a short URL and store it. Here's how we handle form submissions and render the short URL on the homepage:
The res.render('home', { shortUrl, urls })
function injects the short URL and the list of all URLs into the home.ejs
template, making it easy to display them dynamically.
Step 6: Handle URL Redirection
Lastly, handle URL redirection in the app when users click on the short URL:
app.get('/:shortUrl', async (req, res) => { const url = await Url.findOne({ shortUrl: req.params.shortUrl }); if (url) { url.clicks++; await
url.save
(); res.redirect(url.longUrl); } else { res.status(404).send('URL not found'); } });
Conclusion:
In this post, we explored how URL shorteners work and built one from scratch using Node.js, Express, and MongoDB. To make the experience user-friendly, we integrated server-side rendering (SSR) using EJS, allowing us to create dynamic web pages that interact with our backend seamlessly.
By following these steps, you now have a basic URL shortener with a fully functional UI. You can easily expand this project by adding features like user authentication, analytics, or even a custom domain for the short links.
Code (Github):
Code Files: https://github.com/Rishabh6395/ShortURL
Subscribe to my newsletter
Read articles from Rishabh Bhatt directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
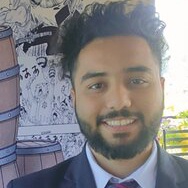
Rishabh Bhatt
Rishabh Bhatt
Hello, My name is Rishabh, a Web Developer who loves creating websites that are both simple and packed with great features. I have a solid foundation in Vanilla JavaScript and React.js, with some experience in Next.js and MongoDB. Recently, I took on a 180-day DSA challenge, where I committed to learning and posting my progress on LinkedIn every single day. It was a rewarding experience that strengthened my problem-solving skills and consistency.