how to connect mysql with spring boot
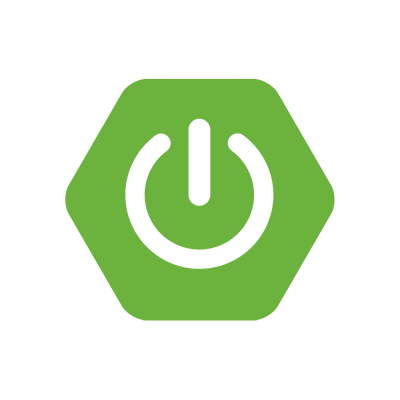
1 min read
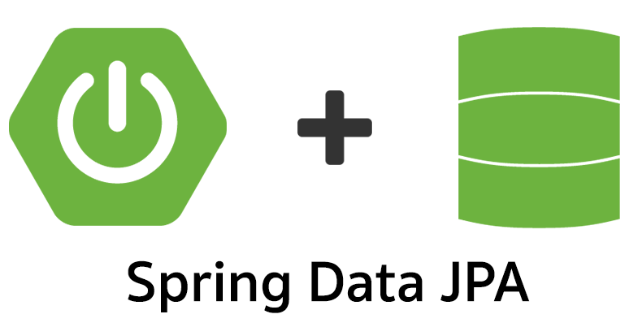
To connect MySQL with Spring Boot, you'll need to follow these steps:
Add MySQL dependencies to your project
Configure the database connection
Create a simple entity and repository
Here's a concise guide:
- Add dependencies to your
pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
- Configure the database connection in
application.properties
:
spring.datasource.url=jdbc:mysql://localhost:3306/your_database
spring.datasource.username=your_username
spring.datasource.password=your_password
spring.jpa.hibernate.ddl-auto=update
- Create a simple entity and repository:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
// Getters and setters
}
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
}
This setup should connect your Spring Boot application to MySQL.
0
Subscribe to my newsletter
Read articles from Nikhil Soman Sahu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
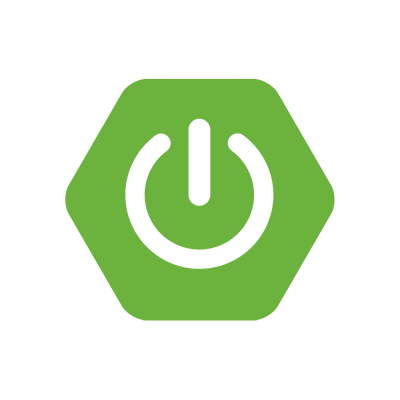
Nikhil Soman Sahu
Nikhil Soman Sahu
Software Developer