Introduction to Data Types, Variables, and Control Statements in Java :

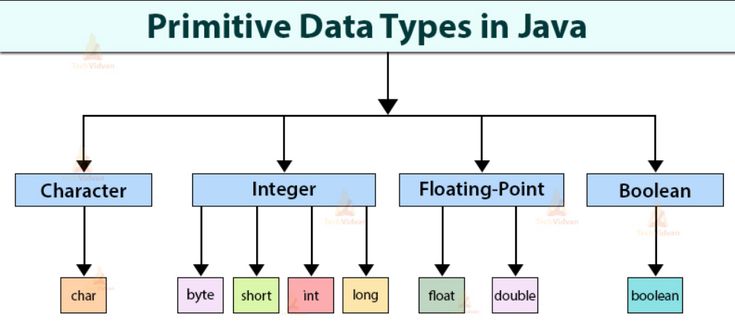
Java is one of the most popular programming languages, known for its simplicity and robustness. Whether you’re a beginner or an expert, understanding the fundamentals like data types, variables, and control statements is essential to writing efficient and maintainable code. In this blog, we’ll dive into these basic concepts with real-world examples and simple explanations that even a beginner can understand, but which also offer depth for more experienced developers.
"The journey of a thousand lines of code begins with a single variable."
Data Types in Java
In Java, data types specify the size and type of values that can be stored in a variable. Think of them like containers for information.
Primitive Data Types
Java has 8 primitive data types, which are predefined by the language. They fall into four main categories:
Integer Types:
byte: 1 byte, stores whole numbers from -128 to 127.
short: 2 bytes, stores whole numbers from -32,768 to 32,767.
int: 4 bytes, stores whole numbers from -2^31 to 2^31-1. (Most commonly used)
long: 8 bytes, stores very large whole numbers.
Floating-Point Types:
float: 4 bytes, stores fractional numbers (single precision).
double: 8 bytes, stores fractional numbers (double precision).
Character Type:
- char: 2 bytes, stores a single character/letter or ASCII values.
Boolean Type:
- boolean: 1 bit, stores true or false values.
Example:
Imagine you are building a calculator. You’d need:
int for simple whole number calculations.
double for precise calculations like 2.99 * 3.1415.
boolean to check if the user has entered valid input (true or false).
int apples = 5; double price = 2.99; boolean isFresh = true;
Non-Primitive Data Types (Reference Types) :
Non-primitive data types include String, Arrays, Classes, and Interfaces. These are more complex types and are derived from classes.
Example: A String holds a sequence of characters, like a name or a sentence.
String greeting = "Hello, world!";
"Variables are like boxes in the real world. The type of box determines what you can store inside."
Variables in Java
A variable is a name given to a memory location that holds data. Each variable has:
A data type (what type of data it holds).
A name (the identifier).
An assigned value.
Declaring a Variable
To declare a variable, you define its data type and name. You can also assign a value to it.
int age; // Declaring a variable
age = 25; // Assigning a value
// Or you can declare and assign together int score = 100;
Real-World Analogy:
Think of variables like fields in a form. The form has fields for age, name, and price, where each field only holds specific data.
String name = "John";
int age = 25;
double balance = 1000.50;
"Control statements are the traffic signals that guide the flow of your program."
Control Statements in Java :
Control statements dictate the flow of execution in a program. They help your code make decisions, repeat actions, and branch into different paths based on conditions.
1. Conditional Statements (if, else, else-if, switch) :
- if Statement: Used to execute a block of code only if a specified condition is true.
int age = 18;
if (age >= 18) {
System.out.println("You are eligible to vote.");
} else{
System.out.println("You are not eligible to vote.");
}
else-if :
The else if statement in Java is used to chain multiple conditions together when you want to test several possibilities in sequence. It comes after an if statement and before an optional else statement, allowing for more than two possible outcomes.
Syntax:
if (condition1) {
// Executes if condition1 is true
}else if(condition2){
// Executes if condition1 is false and condition2 is true
} else{
// Executes if both condition1 and condition2 are false
}
How it works:
The program evaluates the first if condition.
If it's true, the corresponding block of code runs, and the rest of the conditions are ignored.
If the first if condition is false, the program checks the else if condition.
If the else if condition is true, its block of code runs.
If neither the if nor else if conditions are true, the optional else block (if present) will execute.
This structure is useful when you need to check multiple conditions and execute different code based on which condition is met.
Example:
int number = 0;
if (number > 0){
System.out.println("Positive number");
}else if(number < 0){
System.out.println("Negative number");
}else{
System.out.println("Zero");
}
In this example, depending on the value of number, the program will output whether it's positive, negative, or zero.
Nested if-else Statements in Java :
Sometimes, you need to check multiple conditions before deciding which block of code to execute. In such cases, you can use nested if-else statements. A nested if-else is an if-else statement inside another if-else block.
"The beauty of code lies in its ability to make decisions, just like we do in life."
Example of Nested if-else Statement :
Let's look at a scenario where we build a system to determine a person's health based on their age and fitness level.
java int age = 25;
boolean isFit = true;
if (age < 18) {
System.out.println("You're a minor.");
} else {
// age >= 18
if (age <= 40) {
if (isFit) {
System.out.println("You're an adult in great shape.");
}else{
System.out.println("You're an adult, consider a healthier lifestyle.");
}
} else {
// age > 40
System.out.println("You're above 40, stay active to maintain your health.");
}
}
Real-World Analogy:
Think of this nested if-else like a decision-making process at a restaurant:
First, you check if the customer is eligible for a kids' meal (age check).
If they are an adult, you check their fitness level and offer health advice accordingly.
Nested if-else for a Coffee Shop Example:
Imagine a coffee shop where a customer can receive a discount based on their loyalty points and the type of coffee they are ordering.
int loyaltyPoints = 150;
String coffeeType = "Latte";
if(loyaltyPoints > 100){
if (coffeeType.equals("Latte")) {
System.out.println("You get a 20% discount on your Latte!");
}else{
System.out.println("You get a 15% discount on your coffee.");
}
} else{
if (coffeeType.equals("Latte")) {
System.out.println("You get a 5% discount on your Latte.");
} else {
System.out.println("No discount, but enjoy your coffee!");
}
}
In this case:
The system first checks if the customer has more than 100 loyalty points.
If true, it checks what type of coffee they are ordering and offers a discount based on that.
If loyalty points are less than 100, a smaller or no discount is applied.
"Coding is like solving a puzzle—piece by piece, condition by condition, until you see the bigger picture."
Nested if-else statements allow you to handle more complex decision-making processes by checking multiple conditions in a structured manner. However, be mindful that deep nesting can make code harder to read. If you find yourself creating too many nested conditions, it might be a sign to refactor your code or consider alternatives like switch statements.
By using nested if-else structures effectively, you can build flexible and dynamic programs that respond to different scenarios, just like real-life situations where decisions depend on multiple factors.
"The best way to predict the future is to create it—with code."
switch Statement: Used when you have multiple possible conditions for a single variable.
switch(Condition) { case 1: case 2: case 3: default : }
Syntax :
In the above syntax the condition is the statement for the switch block will be executed.
cases are the statements which will run if the condition matches the case and default case is the case where no case match the condition then default will be executed.
Example :
int day = 2;
switch(day) {
case 1: System.out.println("Monday");
break;
case 2: System.out.println("Tuesday");
break;
default: System.out.println("Weekend!");
}
Real-World Example:
Imagine you’re creating an app that provides recommendations based on the weather:
If it’s sunny, suggest going for a walk.
If it’s rainy, recommend staying inside.
String weather = "sunny";
if(weather.equals("sunny")){
System.out.println("It's a great day for a walk!");
}else if(weather.equals("rainy")){
System.out.println("How about a cozy movie indoors?");
}
2. Loops (for, while, do-while) :
Loops allow you to repeat a block of code multiple times. There are three main types of loops in Java:
for loop: Used when the number of iterations is known.
For Example if we need to print 1 to 5 .If we will write the numbers one by one till 5 then it might be possible to write 5 lines of codes but if we are given to print numbers from 1 to 1000 then it will be very lengthy and not a good practice. So here we will use loop statement to print the numbers from 1 to 1000 and here we know the number of iteration which is we have to run the loop 1000 times. So we will use for loop .
for(initialization ; condition ; increment/decrement){ //work which will be performed repeatedly }
SYNTAX :
To understand the syntax here initialization refers to the statement where the loop will begin
Condition refer to until which the loop will run
Increment / Decrement refers to in which way the loop will reach the condition
for (int i = 1; i <= 1000; i++) { System.out.println("Number is : " + i); }
while loop:
In the above case We are known to the number of iteration which is the number of time the loop will run. But if we don’t know the number of iteration then we should use while loop.
Let’s considering an example where we are having a basket full of fruits . But we don’t know how many number of fruits are inside the basket .So in that case we can use while loop. We will iterate the loop until the basket is empty . So don’t think much about how to get the size of the basket or how the basket will be empty .In coming sessions you will understand about this in more details.
Let’s understand another example : Suppose we are given a task to count the number of prime numbers from 1 to 1000 then here we don’t know how many prime numbers lies in between 1 to 1000. So in this case we will use for loop and give a condition which will check whether the number is prime and lies between 1 to 1000. So in cases like this we will use while loop .
In conclusion if we are given to perform some operation without knowing the number of iteration then we should use the while loop.
Syntax :
while(condition){ //task that will be performed untill the task get completed }
int count = 0;
while (count < 5) {
System.out.println("Count: " + count);
count++;
}
do-while loop:
Similar to while, but the condition is checked after each iteration.
In do while loop the statement will run at least 1 time before the condition get checked.
Syntax:
do { //statement or work to perform Increment/Decrement }while(condition);
In the above syntax the code will run at least 1 time before we check the condition.
int num = 1;
do {
System.out.println(num);
num++;
} while (num <= 5);
"Good code is like a well-prepared meal; you control the flow, add the right ingredients, and repeat steps until perfection."
Control Flow Statements : (break, continue )
In Java, break and continue are control flow statements used to alter the normal flow of loops.
break: This statement is used to exit the loop immediately, regardless of the loop's condition. When a break is encountered, the control exits the current loop and moves to the statement following the loop. It's often used when a certain condition is met, and there's no need to continue the loop any further.
Example:
for (int i = 0; i < 10; i++){ if(i == 5){ break; // Exits the loop when i equals 5 } System.out.println(i); }
continue: This statement skips the current iteration of the loop and moves the control back to the beginning of the loop for the next iteration. It’s used when you want to skip certain conditions but still keep the loop running.
Example:
for(int i = 0; i < 10; i++){ if(i == 5){ continue;// Skips the rest of the code when i equals 5 } System.out.println(i); }
Both of these statements are helpful in controlling the flow of loops efficiently.
Conclusion
Java’s data types, variables, and control statements form the backbone of any Java application. For beginners, understanding these concepts is the first step in learning how to write structured and logical programs. For experts, these are the building blocks to creating more complex applications.
Mastering the use of variables and control flow will allow you to design efficient programs that solve real-world problems, like calculating prices, automating tasks, and even building large-scale applications.
“Learn the rules like a pro, so you can break them like an artist."
Subscribe to my newsletter
Read articles from Akash Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Akash Das
Akash Das
Java Developer | Tech Blogger | Spring Boot Enthusiast | DSA Advocate*I specialize in Java, Spring Boot, and Data Structures & Algorithms (DSA). Follow my blog for in-depth tutorials, best practices, and insights on Java development, Spring Boot, and DSA. Join me as I explore the latest trends and share valuable knowledge to help developers grow.