Mastering JavaScript: 4 Essential Selector Methods You Need to Know

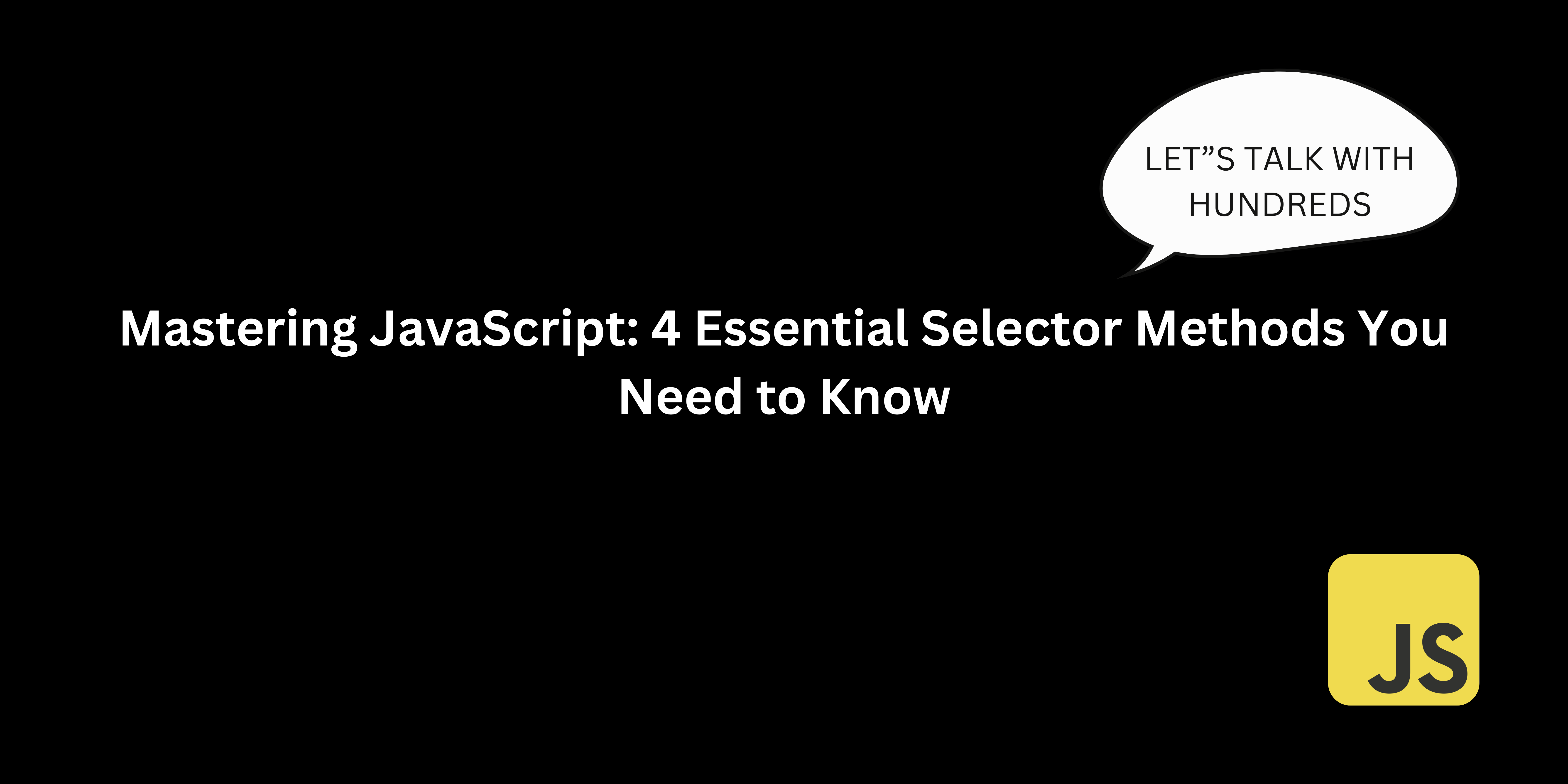
As a frontend developer, understanding how to work with the DOM is crucial, and selecting the right elements efficiently is half the battle. Over time, I’ve realized that choosing the right selector method can save not only time but also a lot of unnecessary code. If you want your website to feel dynamic and intuitive, mastering these four essential selector methods in JavaScript is a must.
Here’s a personalized take on how I use these methods in my projects and why they’re essential to every developer’s toolkit.
1. getElementById()
If there’s one method that I rely on when I need speed and precision, it’s getElementById()
. This method grabs an element using its unique ID, and because IDs are singular on a page, it’s the fastest way to get an element.
let header = document.getElementById('mainHeader');
Why I Use It:
Whenever I’m targeting something I know exists once on the page, like the main header or a unique section, I go for getElementById()
. It’s fast, it’s efficient, and I know it won’t accidentally grab multiple elements.
2. querySelector()
This is one of my favorite methods, especially when I want flexibility. querySelector()
lets you select the first element that matches any CSS selector. Whether you’re selecting by class, ID, or even an attribute, this method adapts to what you need.
let firstButton = document.querySelector('.btn-primary');
Why I Use It:
I reach for querySelector()
when I want to be a bit more selective, like when targeting a button within a specific section or styling based on nested classes. It allows me to be more precise, which is incredibly useful when building responsive and modular layouts.
3. querySelectorAll()
When I’m working with a group of elements and need to apply the same changes to all of them, querySelectorAll()
is my go-to. Unlike querySelector()
, this returns all matching elements in a NodeList, making it perfect for handling multiple items at once.
let allButtons = document.querySelectorAll('.btn');
Why I Use It:
Whenever I’m building something like a product grid or a list of interactive buttons, querySelectorAll()
helps me apply uniform changes, like adding an event listener to all buttons or updating the text on multiple items. It’s efficient and keeps my code clean.
4. getElementsByClassName()
If performance is a concern and you need speed, getElementsByClassName()
is a slightly faster alternative to querySelectorAll()
. It selects all elements that share a specific class name, and while it’s not as flexible, it’s great when you’re dealing with a straightforward class-based selection.
let items = document.getElementsByClassName('menu-item');
Why I Use It:
I use this method when I know exactly what class I’m targeting and don’t need the flexibility of a CSS selector. It’s ideal for situations where speed is crucial, like handling real-time updates on a high-traffic page.
Conclusion
In my experience, knowing which selector method to use can make or break your workflow. Each of these methods serves a unique purpose, and mastering them helps you write cleaner, faster, and more maintainable code. Whether you’re selecting a unique element with getElementById()
or applying changes to a group with querySelectorAll()
, these methods make your JavaScript code powerful and efficient.
Take the time to experiment with them, and you’ll see just how much control you can have over your DOM and, ultimately, your web projects.
Subscribe to my newsletter
Read articles from Anusiem Chidubem directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
