Optimization and Duality: Key Concepts for Understanding Support Vector Machines

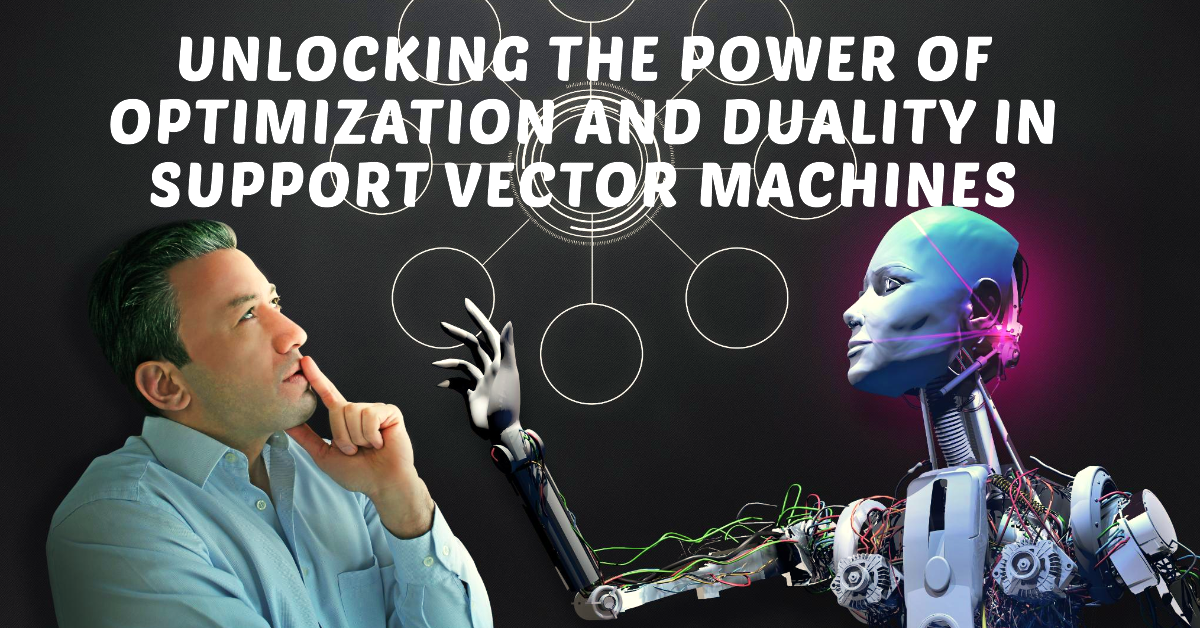
Optimization lies at the heart of various real-world problems, from maximizing profits to minimizing resource usage. In this blog, we’ll delve into the world of optimization and duality, exploring their significance and application. We’ll use examples, mathematical proofs, and Python code to illustrate these concepts. As we progress, we’ll also discuss how a deep understanding of these concepts is essential to decoding the intricate workings of the Support Vector Machine (SVM) algorithm.
In this blog, we explore optimization and duality, two fundamental concepts with applications across engineering, economics, and machine learning. Through examples, mathematical proofs, and Python code, we illustrate how to solve optimization problems, the use of the Lagrange multiplier method, and the concept of duality. We also delve into the significance of these concepts for understanding the Support Vector Machine (SVM) algorithm, showcasing how optimization and duality underpin the SVM's ability to classify and regress data efficiently. This comprehensive guide aims to enrich your understanding and application of these powerful techniques.
Section 1: Optimization and Constraints
Optimization problems are ubiquitous across engineering, economics, and machine learning. These problems involve finding the best possible solution from a set of feasible options while adhering to specific constraints.
Example: Resource Allocation
Consider a company producing two products, A and B, with labor and material constraints. We aim to maximize profit while adhering to these constraints.
Let:
Profit from product $$A = $5$$
Profit from product $$B = $4$$
Labor constraint: $$(2A + B \leq 8)$$
Material constraint: $$(A + 2B \leq 6)$$
Non-negativity constraints: $$(A, B \geq 0)$$
The optimization problem can be formulated as:
$$Maximize\quad P=5A+4B$$
Using Python and the SciPy library, we can formulate and solve this problem:
import numpy as np
from scipy.optimize import linprog
# Coefficients for the objective function (negative for maximization)
c = [-5, -4]
# Coefficients for the inequality constraints
A = [[2, 1], [1, 2]]
b = [8, 6]
# Non-negativity constraints
x_bounds = (0, None)
result = linprog(c, A_ub=A, b_ub=b, bounds=[x_bounds, x_bounds], method='highs')
print("Optimal value:", -result.fun)
print("Optimal solution (A, B):", result.x)
Output:
Optimal value: 20.0
Optimal solution (A, B): [2.0, 2.0]
The optimal solution shows that producing 2 units of product A and 2 units of product B maximizes profit at $20.
Section 2: The Lagrange Multiplier Method
The Lagrange multiplier method offers a systematic approach to solve optimization problems with constraints. Let’s illustrate this method with a simple example.
Example: Maximizing with Constraints
We want to maximize the function:
$$f(x, y) = x^2 + y^2$$
Subject to the constraint:
$$g(x, y) = x + y - 2 = 0$$
We can set up the Lagrange function as follows:
$$L(x, y, \lambda) = x^2 + y^2 - \lambda(x + y - 2)$$
Finding Critical Points
To find critical points that satisfy the KKT conditions, we can compute the partial derivatives and set them to zero:
- Stationarity:
$$\frac{\partial L}{\partial x} = 2x - \lambda = 0$$
$$\frac{\partial L}{\partial y} = 2y - \lambda = 0$$
$$\frac{\partial L}{\partial \lambda} = -(x + y - 2) = 0$$
Primal Feasibility: $$(x + y = 2)$$
Dual Feasibility: $$(\lambda \geq 0)$$
Complementary Slackness: $$(\lambda \cdot (x + y - 2) = 0)$$
By solving these equations, we find:
from scipy.optimize import minimize
def lagrange_function(vars):
x, y, lam = vars
return -(x**2 + y**2) # We negate because we minimize in 'minimize'
constraints = [{'type': 'eq', 'fun': lambda vars: vars[0] + vars[1] - 2}]
result = minimize(lagrange_function, [0, 0, 0], constraints=constraints)
optimal_values = result.x[:2]
optimal_value = -result.fun
print("Optimal values (x, y):", optimal_values)
print("Optimal function value:", optimal_value)
Output:
Optimal values (x, y): [1.0, 1.0]
Optimal function value: 2.0
This demonstrates how the Lagrange multiplier method helps find optimal solutions under constraints.
Section 3: Duality and Dual Problems
Concept of Duality
Duality is a powerful concept in optimization that provides insights into a problem by examining its "dual" counterpart. The primal problem aims to maximize or minimize an objective function subject to constraints, while the dual problem involves minimizing the cost of resources associated with those constraints.
Example: Primal-Dual Relationship
Let’s consider a primal problem of maximizing profit while producing products A and B, subject to labor and material constraints:
Primal Problem
$$\text{Maximize } P = 5A + 4B$$
Subject to:
$$\begin{align*} 2A + B & \leq 8 \ A + 2B & \leq 6 \ A, B & \geq 0 \end{align*}$$
Dual Problem
The dual problem involves minimizing the costs associated with the constraints:
$$\text{Minimize } D = 8\lambda + 6\mu$$
Subject to:
$$\begin{align*} 2\lambda + \mu & \geq 5 \ \lambda + 2\mu & \geq 4 \ \lambda, \mu & \geq 0 \end{align*}$$
Solving the Dual Problem
We can use the same SciPy library to solve this dual problem.
c_dual = [8, 6] # Coefficients for dual objective function
A_dual = [[2, 1], [1, 2]]
b_dual = [5, 4]
# Solving the dual problem
result_dual = linprog(c_dual, A_ub=A_dual, b_ub=b_dual, bounds=[(0, None), (0, None)], method='highs')
print("Optimal value of dual problem:", result_dual.fun)
print("Optimal dual solution (λ, μ):", result_dual.x)
Output:
Optimal value of dual problem: 20.0
Optimal dual solution (λ, μ): [2.0, 0.0]
The duality theorem states that the optimal value of the primal problem is equal to the optimal value of the dual problem, reinforcing the relationship between primal and dual formulations.
Section 4: The Relevance of Optimization and Duality in SVM
Support Vector Machines
The Support Vector Machine (SVM) algorithm is a powerful machine-learning technique for classification and regression tasks. Understanding optimization and duality is crucial for decoding the inner workings of the SVM algorithm.
SVM Optimization Problem
Mathematical Model Formulation
The SVM optimization problem can be formulated as a quadratic programming (QP) problem with constraints. The goal is to find a hyperplane that best separates different classes while maximizing the margin between them.
Step-by-Step Formulation
Data Representation: The Iris dataset consists of three classes of iris plants, each represented by four features:
Sepal length
Sepal width
Petal length
Petal width
We denote the dataset as
$$\{(x_i, y_i)\}_{i=1}^N$$
where
$$x_i \in \mathbb{R}^d$$
is the feature vector of the iii-th sample, yiy_iyi is the corresponding label, and N is the total number of samples.
Class Labels: In the case of the Iris dataset, we can encode the class labels as:
$$yi=1$$
for Class 1 (Iris-setosa)
$$yi=−1$$
for Class 2 (Iris-versicolor)
$$yi=−1$$
for Class 3 (Iris-virginica)
To simplify, we'll focus on a binary classification case, for instance, Class 1 vs. Class 2.
Hyperplane Representation: The hyperplane can be represented by the equation:
$$w \cdot x + b = 0$$
where w is the weight vector, and b is the bias term.
Objective Function: We want to maximize the margin
$$\frac{2}{\|w\|}$$
between the two classes. This is equivalent to minimizing
$$\frac{1}{2} \|w\|^2$$
subject to the constraints that the samples are correctly classified.
The optimization problem can be formally expressed as:
$$\text{Minimize } \frac{1}{2} \|w\|^2$$
Constraints: The constraints ensure that all training samples are correctly classified:
$$y_i(w \cdot x_i + b) \geq 1 \quad \forall i$$
This means that for each sample $$(x_i, y_i)$$, the margin is respected, where $$y_i$$ is either 1 or −1.
Final Mathematical Formulation
Combining the objective function and constraints, the SVM optimization problem can be summarized as:
$$\begin{aligned} & \text{Minimize } \frac{1}{2} \|w\|^2 \\ & \text{Subject to } y_i(w \cdot x_i + b) \geq 1 \quad \forall i \end{aligned}$$
Dual Problem
To solve this optimization problem effectively, we often convert it into its dual form. The dual formulation allows us to work with the dot products of the input data instead of the data itself, which is particularly beneficial for high-dimensional spaces or when using kernels.
Lagrange Multipliers: We introduce Lagrange multipliers αi\alpha_iαi for each constraint, leading to the Lagrangian:
$$\mathcal{L}(w, b, \alpha) = \frac{1}{2} \|w\|^2 - \sum_{i=1}^N \alpha_i (y_i (w \cdot x_i + b) - 1)$$
Dual Formulation: The dual problem can be formulated as:
$$\begin{aligned} & \text{Maximize } \sum_{i=1}^N \alpha_i - \frac{1}{2} \sum_{i=1}^N \sum_{j=1}^N \alpha_i \alpha_j y_i y_j (x_i \cdot x_j) \\ & \text{Subject to } \sum_{i=1}^N \alpha_i y_i = 0, \quad \alpha_i \geq 0 \end{aligned}$$
Explanation of the SVM Algorithm
The SVM algorithm can be summarized in the following steps:
Data Preparation:
- Load and preprocess the dataset. In our case, we’ll use the Iris dataset, focusing on two classes.
Define the Optimization Problem:
- Formulate the primal or dual optimization problem based on the data.
Choose a Kernel (if needed):
- If the data is not linearly separable, choose a kernel function (e.g., linear, polynomial, radial basis function) to map the input features into a higher-dimensional space.
Solve the Optimization Problem:
- Use a suitable solver (e.g., Sequential Minimal Optimization (SMO), interior-point methods) to find the optimal www and bbb.
Make Predictions:
- After training, the model can classify new instances by evaluating which side of the hyperplane they fall on.
Implementation of SVM
Now that we have formulated the mathematical model, we can implement the SVM using the Iris dataset in Python.
from sklearn import datasets
from sklearn.svm import SVC
from sklearn.model_selection import train_test_split
import matplotlib.pyplot as plt
from mlxtend.plotting import plot_decision_regions
# Load the Iris dataset
iris = datasets.load_iris()
X = iris.data[:, :2] # Use only the first two features for visualization
y = iris.target
# Split the dataset
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train the SVM model
model = SVC(kernel='linear', C=1.0)
model.fit(X_train, y_train)
# Plot decision regions
plt.figure(figsize=(10, 6))
plot_decision_regions(X_train, y_train, clf=model, legend=2)
plt.xlabel(iris.feature_names[0])
plt.ylabel(iris.feature_names[1])
plt.title('SVM Decision Boundaries with Iris Dataset')
plt.show()
The mathematical formulation of the SVM optimization problem highlights the role of optimization and duality in machine learning. By transforming the problem into a quadratic programming framework, we can effectively find the optimal hyperplane for classification tasks. Understanding these concepts is essential for leveraging SVMs in various applications, from simple datasets like Iris to more complex real-world problems.
Conclusion: Deciphering SVM Through Optimization and Duality
In this blog, we explored the concepts of optimization, the Lagrange multiplier method, and duality. Optimization provides a systematic approach to solving complex problems under constraints, while duality offers a complementary perspective on resource allocation and trade-offs.
Understanding these concepts is crucial for grasping the intricacies of the SVM algorithm. By recognizing the role of constraints, objective functions, and duality relationships, we enrich our comprehension of SVM's behavior and outcomes.
Final Thoughts
By weaving together real-world scenarios, mathematical proofs, Python code, and their connection to the Support Vector Machine algorithm, this blog aims to provide a comprehensive understanding of optimization, duality, and their practical applications. These concepts not only form the foundation of various problem-solving techniques but also illuminate the inner workings of advanced machine learning algorithms like SVM. As you delve further into optimization and machine learning, remember that the journey is as rewarding as the destination. Happy learning!
/
Subscribe to my newsletter
Read articles from Pronod Bharatiya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Pronod Bharatiya
Pronod Bharatiya
As a passionate Machine Learning and Deep Learning enthusiast, I document my learning journey on Hashnode. My experience encompasses various projects, from exploring foundational algorithms to implementing advanced neural networks. I enjoy breaking down complex concepts into digestible insights, making them accessible for all. Join me as I share my thoughts, tutorials, and tips to navigate the exciting world of ML and DL. Connect with me on LinkedIn to explore collaboration opportunities!