How to Implement Dark Mode and Light Mode in React

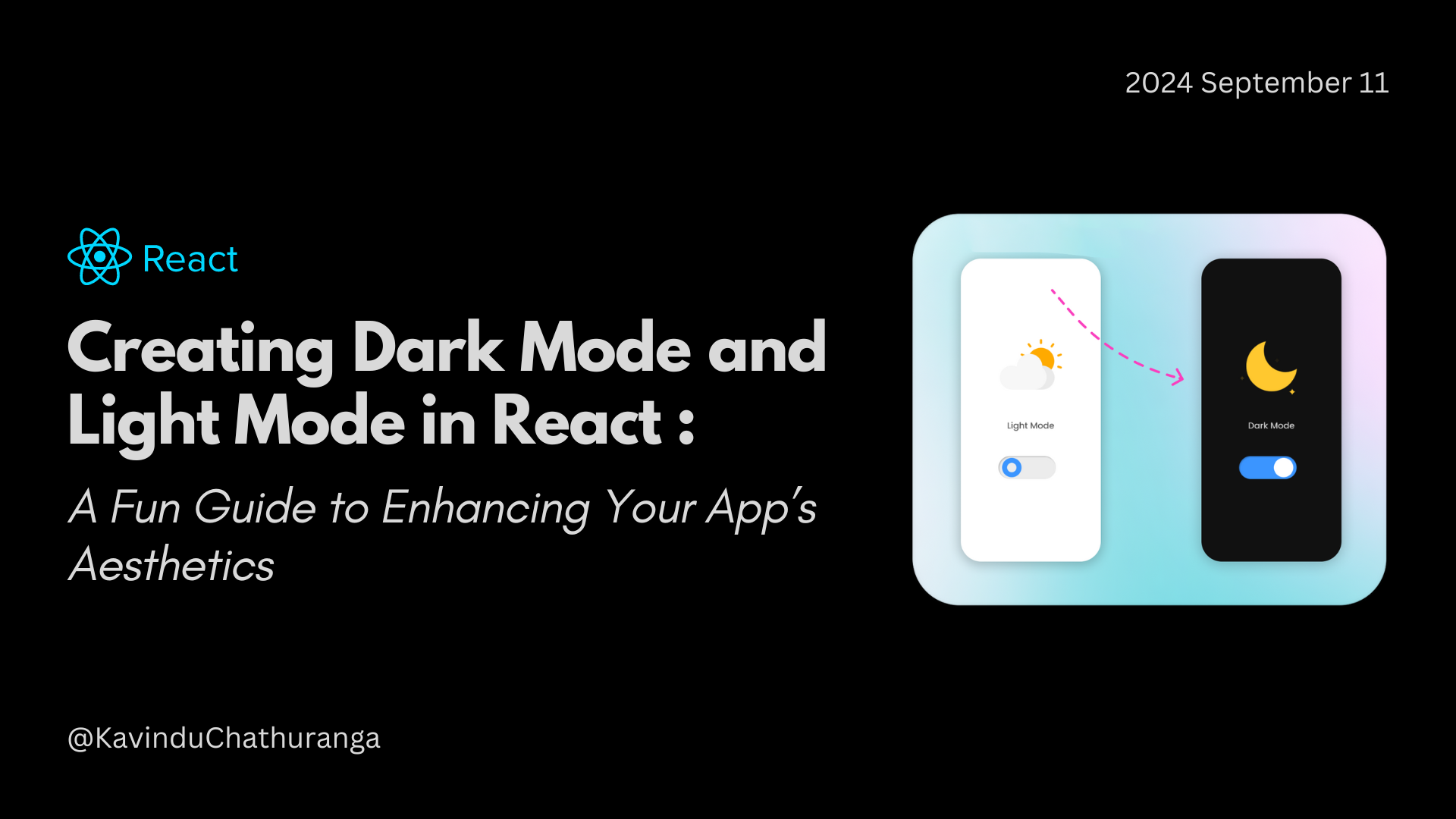
🌟 Introduction
In today's world, dark mode has become a beloved feature across many applications. It’s not only aesthetically pleasing but also reduces eye strain in low-light environments. In this tutorial, we’ll learn how to create a dark mode and light mode toggle in a React application from scratch.
📌 Why Implement Dark Mode?
Dark mode isn’t just a design trend—it's a practical feature that enhances user experience. Here’s why you should consider adding it to your website:
Reduced Eye Strain: Dark mode can be easier on the eyes, especially in low-light environments. It reduces the amount of blue light emitted by screens, which can help alleviate eye fatigue.
Improved Battery Life: For OLED screens, dark mode can significantly extend battery life because pixels use less power to display black or darker colors.
Enhanced Readability: In dark mode, text can stand out more clearly against a darker background, which can be particularly beneficial for users with visual impairments.
Modern Aesthetic: Dark mode offers a sleek, modern look that many users find visually appealing and trendy. It can give your site a contemporary edge.
User Preference: Many users prefer dark mode for various reasons, including personal comfort and aesthetic preference. Offering both modes can enhance user satisfaction and retention.
We’ll explore:
Setting up a simple React app.
Adding a toggle switch for dark and light mode.
Let’s dive in!
🛠️ Prerequisites
Before we get started, make sure you have a basic understanding of the following:
React fundamentals (components, state, and props).
CSS for basic styling.
A React project already set up (or follow along using
create-react-app
).
📋 Project Setup: Let’s Get Started!
Before we dive into the code, make sure you have a React app ready to go. If not, set it up with:
npx create-react-app dark-light-mode
cd dark-light-mode
Step 1: Crafting the Toggle Component
Create a file named Toggle.js
in the src/Components
directory. This component will be our magical switch between light and dark modes:
import "./toggle.css";
export const Toggle = ({ handleChange, isChecked }) => {
return (
<div className="toggle-container">
<input
type="checkbox"
id="check"
className="toggle"
onChange={handleChange}
checked={isChecked}
/>
<label htmlFor="check">Dark Mode</label>
</div>
);
};
Style It Up
Add some style magic to toggle.css
:
.toggle-container {
position: absolute;
top: 2em;
right: 2em;
}
.toggle {
visibility: hidden;
}
.toggle + label {
display: flex;
align-items: center;
font-size: 1.5em;
cursor: pointer;
color: var(--primary-text-color);
position: relative;
}
.toggle + label::before {
content: "";
height: 1em;
width: 2em;
border-radius: 1em;
background-color: var(--toggle-bg);
margin-right: 0.5em;
transition: background-color 250ms ease-in-out;
position: relative;
}
.toggle + label::after {
content: "";
height: 0.8em;
width: 0.8em;
border-radius: 50%;
background-color: var(--toggle-fg);
position: absolute;
left: 0.2em;
top: 50%;
transform: translateY(-50%);
transition: background-color 250ms ease-in-out, transform 250ms ease-in-out;
}
.toggle:checked + label::after {
transform: translate(1.2em, -50%);
}
Step 2: The App Component – Where the Magic Happens
Now, let’s sprinkle some magic into our App.js
:
import React, { useState } from 'react';
import './App.css';
import { Toggle } from "./Components/Toggle";
const App = () => {
const [isDark, setIsDark] = useState(true);
const handleToggle = () => {
setIsDark(!isDark);
};
return (
<div className="App" data-theme={isDark ? "dark" : "light"}>
<Toggle
isChecked={isDark}
handleChange={handleToggle} // Pass the toggle handler as a prop
/>
<h1 className='title'>
Hello World
</h1>
<div className="box">
<h2>
This is a box
</h2>
</div>
</div>
);
}
export default App;
Step 3: Global Styles – Light & Dark Themes
Set up your global styles in index.css
to define the light and dark themes:
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: 'Arial';
}
:root {
--background-color: #ffffff;
--foreground-color: #003cff;
--primary-text-color: black;
--secondary-text-color: white;
--toggle-bg: #f0eb9d;
--toggle-fg: #ffd000;
}
[data-theme="dark"] {
--background-color: #0a0a0a;
--foreground-color: #567aff;
--primary-text-color: #f6f6f6;
--secondary-text-color: #0a0a0a;
--toggle-bg: #283452;
--toggle-fg: #00a6ff;
}
.App {
width: 100%;
height: 100vh;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
background-color: var(--background-color);
}
.title {
color: var(--primary-text-color);
margin-bottom: 50px;
}
.box {
padding: 20px;
border-radius: 10px;
background-color: var(--foreground-color);
color: var(--secondary-text-color);
}
7. Testing the Toggle
Launch your app by running
npm start
.Toggle between light and dark modes.
Refresh the page to see that the theme persists (thanks to
localStorage
).
💡 Final Thoughts
Congratulations! Your React app is now equipped with both dark mode and light mode. This feature not only makes your app more dynamic but also enhances user experience by allowing users to choose their preferred viewing mode. Customize the colors and styles to fit your app’s design, and enjoy coding in style!
📂 Source Code
You can find the complete source code for this project on my GitHub repository.
Happy coding! 💻✨
Subscribe to my newsletter
Read articles from Kavindu Chathuranga Dasanayaka directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Kavindu Chathuranga Dasanayaka
Kavindu Chathuranga Dasanayaka
Passionate full-stack developer and UI/UX enthusiast, shaping intuitive digital experiences with innovative solutions