What’s New in Laravel 11: Key Updates You Need to Know
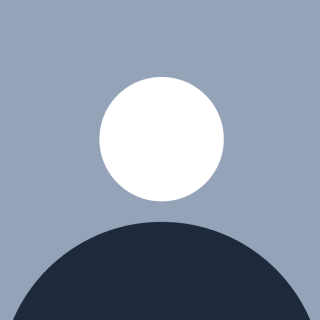
Table of contents
- List of the Features
- Php 8.2.
- Minimal Application Structure
- Streamlined Application Structure
- Config Changes
- Console Kernel Removed
- Laravel Reverb.
- Casts Method
- New Artisan Commands.
- Graceful encryption key rotation.
- Queue interaction testing
- Adjust Rate Limit
- Health Routing
- The Once Function
- Sqlite by Default
- Prompt Validation
- Summary
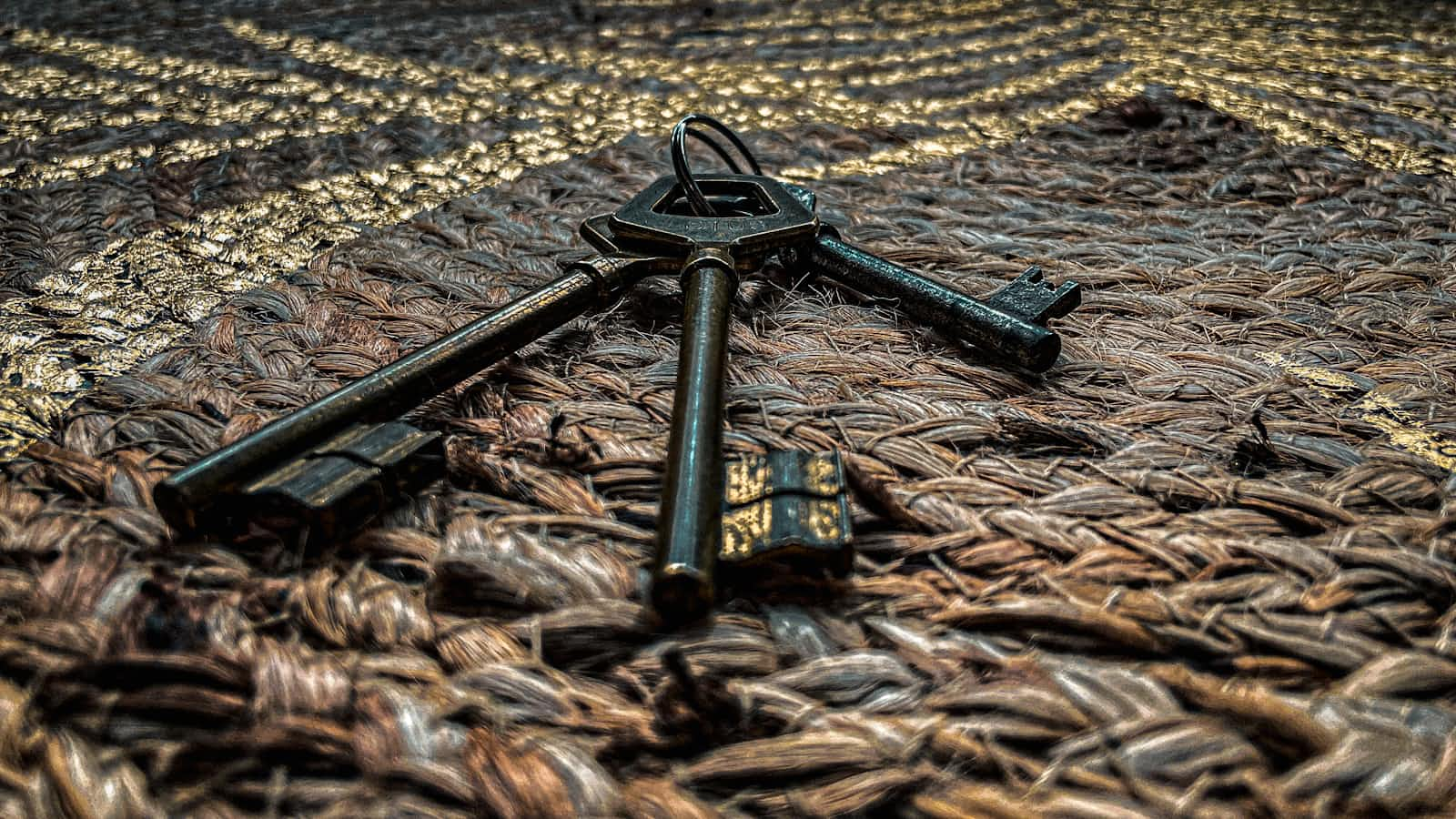
Laravel is a highly popular PHP framework, consistently chosen by many developers worldwide for its efficient web development capabilities. It adheres to the Model-View-Controller (MVC) architecture for organized and scalable project structures.
Laravel 11 is a significant upgrade that introduces a range of enhancements to streamline development and improve performance. Let’s dive into some of the key features that make Laravel 11 a must-try for developers.
List of the Features
Php 8.2.
The most significant change in Laravel 11 is the discontinuation of support for PHP 8.1. Now, PHP 8.2 is the minimum required version.
Minimal Application Structure
In Laravel 11, the app
folder has been made much simpler. Here’s what has changed in easy-to-understand terms:
- HTTP and Console Kernels Removed: These are no longer part of the
app
folder. They were responsible for handling incoming requests and console commands, but now Laravel handles this within the framework. You don’t need to worry about these files anymore. In earlier Version, the Kernel files were inside theHttp
andConsole
folders.
Earlier Version
Laravel 11
2. Middleware Moved to the Framework: The nine middleware files (which are used to process incoming requests) have been shifted into the framework. This change is because most developers rarely need to modify them.
3. Exception Handler Removed: The file that used to handle errors is no longer in the app folder, simplifying error management.
4. Single Provider: The Providers directory, which used to have multiple files for bootstrapping services, has been reduced to just a single provider file
We can still customize our application easily. We can do this through a file called bootstrap/app.php
. Here’s what we can adjust using this file:
Routing: We can change how our application handles different URLs and routes.
Middleware: We can set up or modify how requests are processed before they reach our application.
Service Providers: We can add or adjust services that our application uses.
Exception Handling: We can manage how our application deals with errors and exceptions.
In short, bootstrap/app.php
is where we go to tweak and set up these important parts of our application.
Laravel 11
Also, the routes
folder is now simpler. The api.php
and channels.php
route files are no longer included by default because many apps don’t need them.
If we need the api.php
or channels.php
file in the routes folder, we can create them using an Artisan command.
php artisan install:api
php artisan install:broadcasting
Streamlined Application Structure
In simple terms, one of the key features of Laravel 11 is its simplified and organized structure. Created by Taylor Otwell
and Nuno Maduro
, this new design makes the framework easier and more modern to use for developers. Even though it’s more streamlined, it still feels familiar and keeps the core ideas of Laravel the same, so developers don’t have to relearn everything.
Config Changes
In earlier versions of Laravel, there were many files inside the config folder. However, in Laravel 11, some of these files have been removed like
config/broadcasting.php
config/cors.php
config/hashing.php
config/sanctum.php
config/view.php
and the configuration options are now handled differently. The .env
file has been expanded to include all the options you need to set or configure.
But We can publish these files with the help of artisan commands.
php artisan config:publish
OR
php artisan config:publish --all
Console Kernel Removed
As we already discussed, the file app/Console/Kernel.php
is being removed in Laravel 11. But it's actually meant to make things easier. Instead of using that file to set up console commands, Laravel now has a simpler way to do it. So, there’s no need to worry—this just makes defining commands less complicated and more straightforward.
If we want to define the console command then we need to define it in routes/console.php
directly.
use Illuminate\Support\Facades\Schedule;
Schedule::command('emails:send')->daily();
Laravel Reverb.
It was developed by Joe Dixon
. Laravel Reverb allows our Laravel apps to have fast and scalable real-time communication using WebSockets. This means our app can instantly send and receive messages without delays. It works smoothly with Laravel’s event broadcasting tools, like Laravel Echo, making it easy to add real-time features to our app.
php artisan reverb:start
Reverb also allows you to handle more WebSocket traffic by using Redis’s publish/subscribe
capabilities. This means you can spread the WebSocket load across multiple Reverb servers, making it possible to support a large, high-demand application without slowing down. This helps our app run smoothly, even with lots of users at the same time.
Casts Method
In earlier versions of Laravel, casts
were defined as properties of the model. However, in Laravel 11, casts
are now defined as methods within the model. This change allows you to do more, like calling other methods directly from the casts.
Typically, Laravel requires defining attribute casting in an Eloquent model as shown below.
<?php
namespace App\Models;
// use Illuminate\Contracts\Auth\MustVerifyEmail;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
use Laravel\Sanctum\HasApiTokens;
class User extends Authenticatable
{
use HasApiTokens, HasFactory, Notifiable;
/**
* The attributes that should be cast.
*
* @var array<string, string>
*/
protected $casts = [
'email_verified_at' => 'datetime',
'password' => 'hashed',
];
}
Now In Laravel 11, we can specify our casts as an array, as given below:
<?php
namespace App\Models;
// use Illuminate\Contracts\Auth\MustVerifyEmail;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
class User extends Authenticatable
{
use HasFactory, Notifiable;
/**
* Get the attributes that should be cast.
*
* @return array<string, string>
*/
// Without Array
protected function casts(): array
{
return [
'email_verified_at' => 'datetime',
'password' => 'hashed',
];
}
//Using Array
protected function casts() : array
{
return [
'foo' => [PostCollection::class, LikeCollection::class],
];
}
}
New Artisan Commands.
Laravel 11
added some new commands to allow the quick creation of classes, enums, interfaces, and traits:
php artisan make:class
php artisan make:enum
php artisan make:interface
php artisan make:trait
Graceful encryption key rotation.
In Laravel
, all cookies, including session cookies, are encrypted, meaning that every request to a Laravel application depends on encryption. Previously, if you changed the encryption key (APP_KEY
), it would log out all users and make it impossible to decrypt data encrypted with the old key.
Laravel 11
solves this problem by introducing a new feature that lets you list previous encryption keys using the APP_PREVIOUS_KEYS
environment variable. This allows our application to decrypt data encrypted with old keys, ensuring a smooth transition when rotating encryption keys without logging users out or breaking data.
Queue interaction testing
Laravel’s queue system is great for handling tasks in the background. In Laravel 11, testing these queues is much easier with improved features like withFakeQueueInteractions
. This lets you quickly test if jobs were released, deleted, or failed, ensuring our background jobs are reliable and our tests are more thorough.
use App\Jobs\ProcessPodcast;
$job = (new ProcessPodcast)->withFakeQueueInteractions();
$job->handle();
$job->assertReleased(delay: 30);
Adjust Rate Limit
Laravel 11 now supports per-second rate limiting, meaning developers can set more precise limits on how often HTTP requests and queued jobs are allowed each second. This gives better control over managing traffic and job processing.
RateLimiter::for('invoices', function (Request $request) {
return Limit::perSecond(1);
});
Health Routing
In Laravel 11
, new applications come with a built-in health-check route. This route is a simple way to check if our application is running properly. It’s set up /up
by default and can be used by monitoring tools or systems like Kubernetes to check the health of our app.
The Once Function
The once
helper function runs a given callback function and saves its result in memory for the duration of the current request. If you call once
with the same callback function again, it will return the saved result instead of running the callback again.
function random(): int
{
return once(function () {
return random_int(1, 1000);
});
}
random(); // 123
random(); // 123 (cached result)
random(); // 123 (cached result)
Sqlite by Default
By default, new Laravel applications use SQLite for the database, which is also used for storing session data, cache, and queue information. When we create a new project with the composer create-project
command or the Laravel Installer, it automatically sets up the SQLite database file and runs the initial database setup for you.
Prompt Validation
Laravel 11
integrates with the Laravel Prompts package, which adds stylish and user-friendly forms to our command-line tools. It includes features like placeholder text and validation, making it easy to validate inputs just like in a web browser.
$name = text('What is your name?', validate: [
'name' => 'required|min:3|max:255',
]);
If you want to understand in detail then we can refer to Laravel Prompt Validation.
Summary
These are just a few of the exciting new features and improvements in Laravel 11. For more details and instructions on upgrading your existing applications, check out the official Laravel documentation.
Wow, what a ride! 🚀 If this article sparked your interest or helped you out, please drop a comment and hit that clap button to show your support. Your feedback keeps me going and helps me create even more awesome content. Don’t forget to follow for more exciting updates and feel free to reach out if you need any help. Let’s keep the excitement and support flowing together! 🌟🙌
Subscribe to my newsletter
Read articles from Aman jain directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Aman jain
Aman jain
I'm a developer who shares advanced insights and expertise through technology-related articles. Passionate about creating innovative solutions and staying up-to-date with the latest tech trends. Let's connect and explore the world of technology together!