Simplifying the forEach Method : A Beginner’s Guide

Table of contents
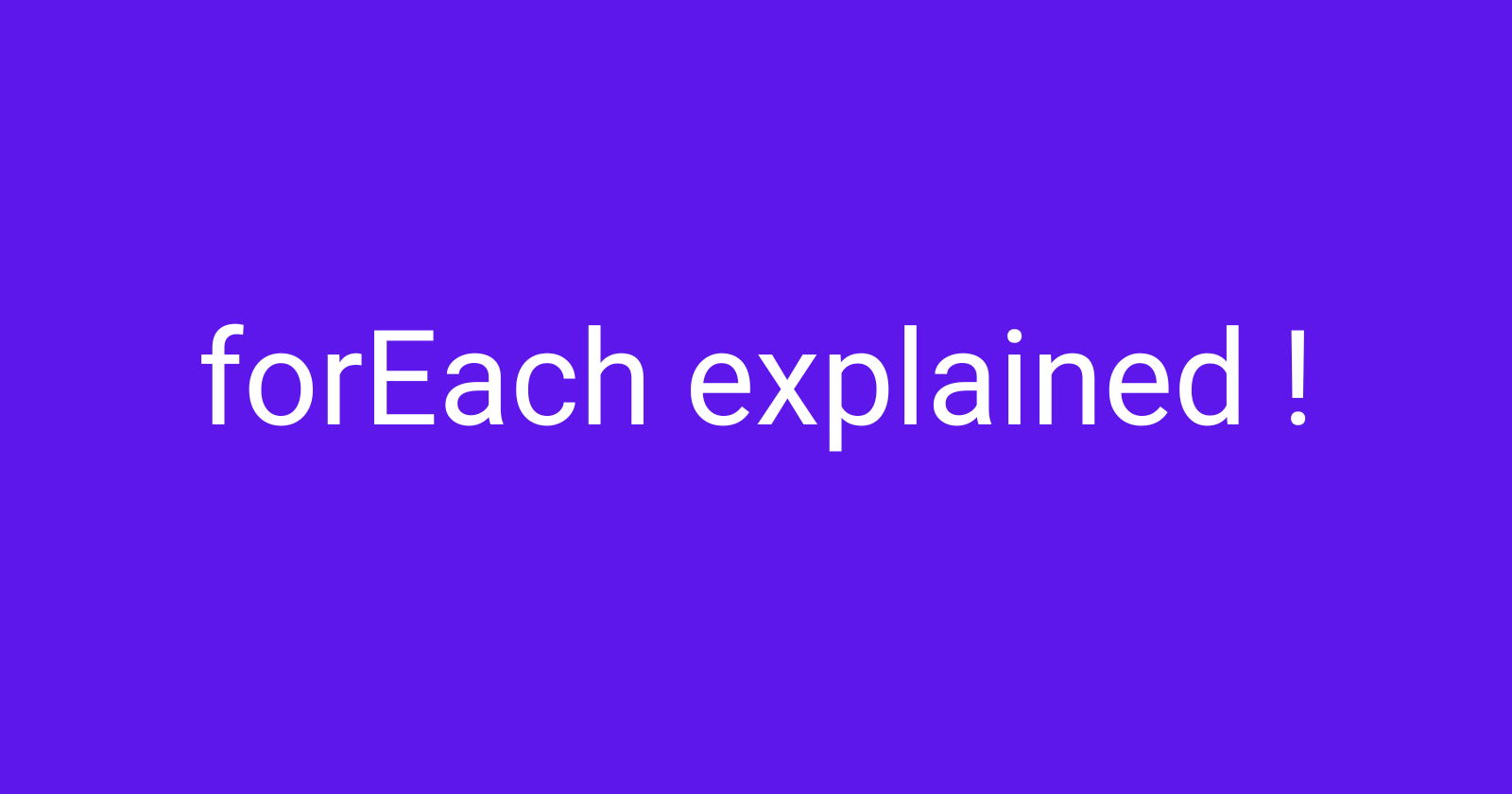
If you are a JavaScript beginner, you must have come across the forEach
method. It might look a little bit quirky at first, but believe me, you will be using this method more often after learning it.
Hi fellow developers, my name is Sandeep, and I am an aspiring developer. As part of my development journey, I hardly use traditional loops such as for
loops and while
loops, because JavaScript (yes, I love this language a lot 😁) provides a lot of out-of-the-box tools to solve problems including array handling and that makes our life a lot easier!
In this article, I will try to break down the forEach
method and make it as easy to understand as possible.
The Syntax
let’s take a look at its syntax first…
//define an array first
const ourArray=[a,b,c,d,e];
//now let's define forEach
ourArray.forEach ( function() ) ;
We use forEach method to execute a FUNCTION
for every single array element. This function can take three arguments .
ourFunction ( currentElement , index , array ) {
//code to be executed
}
These argument are -
currentElement - the array element
index - index of array element
array - the array itself
You mostly be working with first two arguments i.e currentElement and index.
ourArray.forEach ( function(currentElement , index ) {
//code to be executed for each element
} ) ;
Now let’s implement the above syntax with a simple code example.
const ourArray = [1, 2, 3, 4, 5];
ourArray.forEach( function(element , index) {
console.log('Element:' , element); //to console each element [1,2,3,4,5]
console.log ('index:' , index); // to console indexs [0,1,2,3,4]
});
yeah , this is what forEach method is . it simply runs a function for each array element.
let’s take a look at another example . Here we will run a function which will double the each array element and we will be using arrow function syntax this time (arrow functions are awesome 😎 )
const array = [2,4,6,8,10];
array.forEach( (element)=> {
let double= 2*element; //double the array element
console.log(double);
});
// 4,8,12,16,20
Please , try these codes in your VsCode ( i know you are using Vs code😂 ) .
This is pretty much what the forEach
method is.
Now let’s understand where you will be using this forEach method for practical purpose.
Suppose you have four buttons in your html
<button>btn 1</button>
<button>btn 2</button>
<button>btn 3</button>
<button>btn 4</button>
and you want to display an alert message whenever you click on a button.
will you attach a separate event handler to each button like this ?
const btn= document.querySelectorAll('button');
//querySelectorAll returns a node list , which looks like array !
btn[0].addEventListener('click' , ()=>alert("hello bro"));
btn[1].addEventListener('click' , ()=>alert("hello bro"));
btn[2].addEventListener('click' , ()=>alert("hello bro"));
btn[3].addEventListener('click' , ()=>alert("hello bro "));
Definitely not! Here we want to execute the same function for each button, so why not use forEach
? Don’t we exactly use the forEach
method for this purpose??
so , let’s use this
const btn= document.querySelectorAll('button');
//btn is an array , well not exactly an array , it's node list !
btn.forEach ( (element)=>{
element.addEventListener('click' , ()=>alert("hello bro"));
});
Now you can compare for yourself which one is easier.
I hope you have tried the above codes alongside and have understood them well and practise until you master it.
Well, that’s all for today. I will be back with more JavaScript and development-related topics.
Follow me if you like this post 👋
Subscribe to my newsletter
Read articles from Sandeep Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
