Efficiently Bypassing CORS in Next.js 14: A Practical Guide
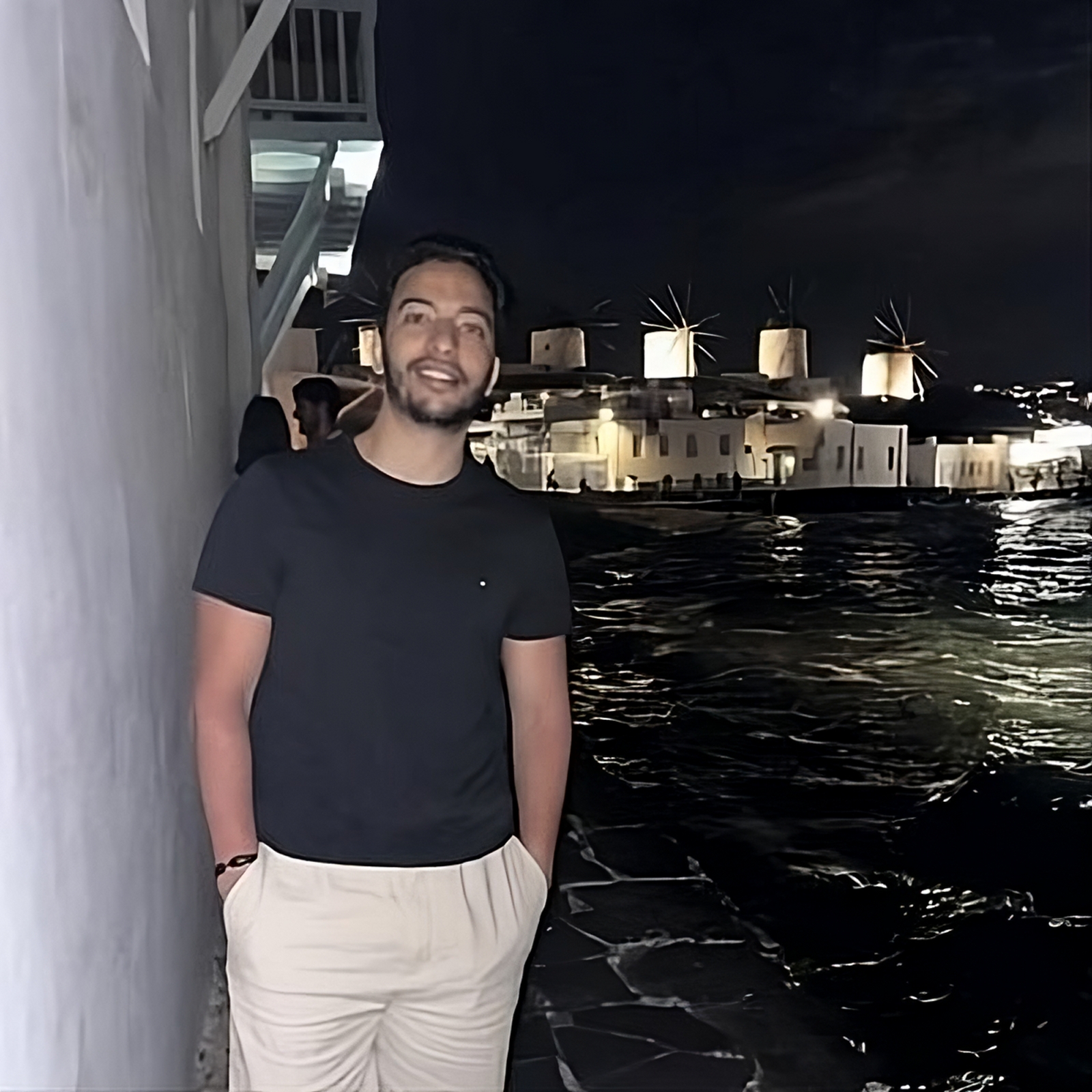
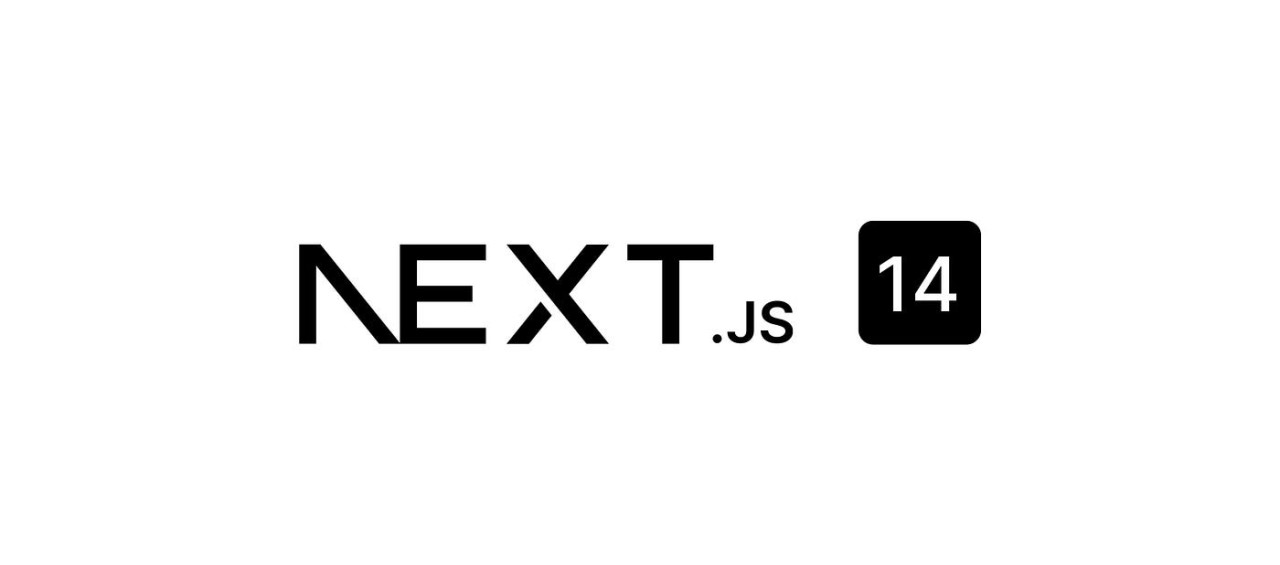
As a frontend developer, you've likely encountered CORS (Cross-Origin Resource Sharing) issues when working with external APIs. This guide will walk you through a straightforward solution to bypass CORS in Next.js 14, using a real-world example of fetching an RSS feed.
Understanding CORS
CORS is a security mechanism that restricts web pages from making requests to a different domain than the one serving the web page. While crucial for web security, it can become a hurdle during development, especially when working with third-party APIs or services.
The Challenge
Recently, while developing a portfolio with Next.js 14, I needed to fetch and display an RSS feed from a blog. However, I faced a CORS error because I was working solely on the frontend and didn't have control over the server hosting the RSS feed.
A Simple Solution in Next.js 14
Next.js 14 provides an elegant solution by allowing us to create an internal API route that acts as a proxy. Here's how to implement it:
- Create an API Route
First, create a file named route.ts
in the app/api/rss
directory:
import { NextResponse } from "next/server";
import Parser from "rss-parser";
export async function GET(request: Request) {
const parser = new Parser();
const feed = await parser.parseURL('https://blog.example.com/rss.xml');
const items = feed.items.slice(0, 3).map((item: any) => ({
title: item.title || "",
link: item.link || "",
source: "Example Blog",
contentSnippet: item.contentSnippet || "",
content: item.content || "",
image: feed.image?.url,
publishedAt: item.pubDate
}));
return NextResponse.json(items);
}
This API route fetches the RSS feed, parses it, and returns the first three items as JSON. By doing this server-side, we avoid CORS issues entirely.
- Implement the Frontend Component
Now, let's create a React component to display the fetched articles using the useSWR
hook for data fetching and caching:
import React from "react";
import useSWR from 'swr';
import PostItem from "@/app/post-item";
const fetcher = (url: string) => fetch(url).then((res) => res.json());
interface Article {
title: string;
link: string;
source: string;
contentSnippet?: string;
content?: string;
image?: string;
publishedAt?: string;
}
export default function Articles() {
const { data, error } = useSWR("/api/rss", fetcher, {
revalidateOnFocus: false,
revalidateOnReconnect: false
});
if (error) return <div>Failed to load articles</div>;
return (
<section>
<h2 className="text-xl font-semibold mb-4">Latest Articles</h2>
<div className="space-y-4">
{data ? (
data.map((item: Article, index: number) => (
<PostItem key={index} {...item} />
))
) : (
<p className="text-sm text-gray-600">
Loading articles...
</p>
)}
</div>
</section>
);
}
Explanation of useSWR
and the Fetch Function
The useSWR
hook is a powerful tool for data fetching in React applications:
SWR (Stale-While-Revalidate): SWR is a React Hooks library for data fetching, created by the Vercel team. It's named after the HTTP Cache-Control extension "stale-while-revalidate". You can find more information Here
The
fetcher
function is a wrapper around the nativefetch
API, making a GET request and parsing the response as JSON.useSWR
takes the API route ("/api/rss") as its key and thefetcher
function as its second argument.It returns an object with
data
anderror
properties, handling loading and error states automatically.
Benefits of this Approach
CORS Bypass: The Next.js API route acts as a proxy, fetching data server-side where CORS restrictions don't apply.
Efficient Data Fetching: SWR provides automatic revalidation, caching, and error handling.
Clean Implementation: This solution requires minimal setup and integrates smoothly with Next.js projects.
Conclusion
This method offers a practical solution for bypassing CORS issues in Next.js 14 applications. It's particularly useful for scenarios where you need to fetch data from external sources without modifying the backend.
While effective for many use cases, always consider the implications of proxying requests through your server, especially for high-traffic applications. In some scenarios, implementing proper CORS headers on your API might be more appropriate.
By leveraging Next.js API routes and SWR, you can create robust, CORS-free data fetching solutions in your Next.js applications.
Subscribe to my newsletter
Read articles from Ayoub Touba directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
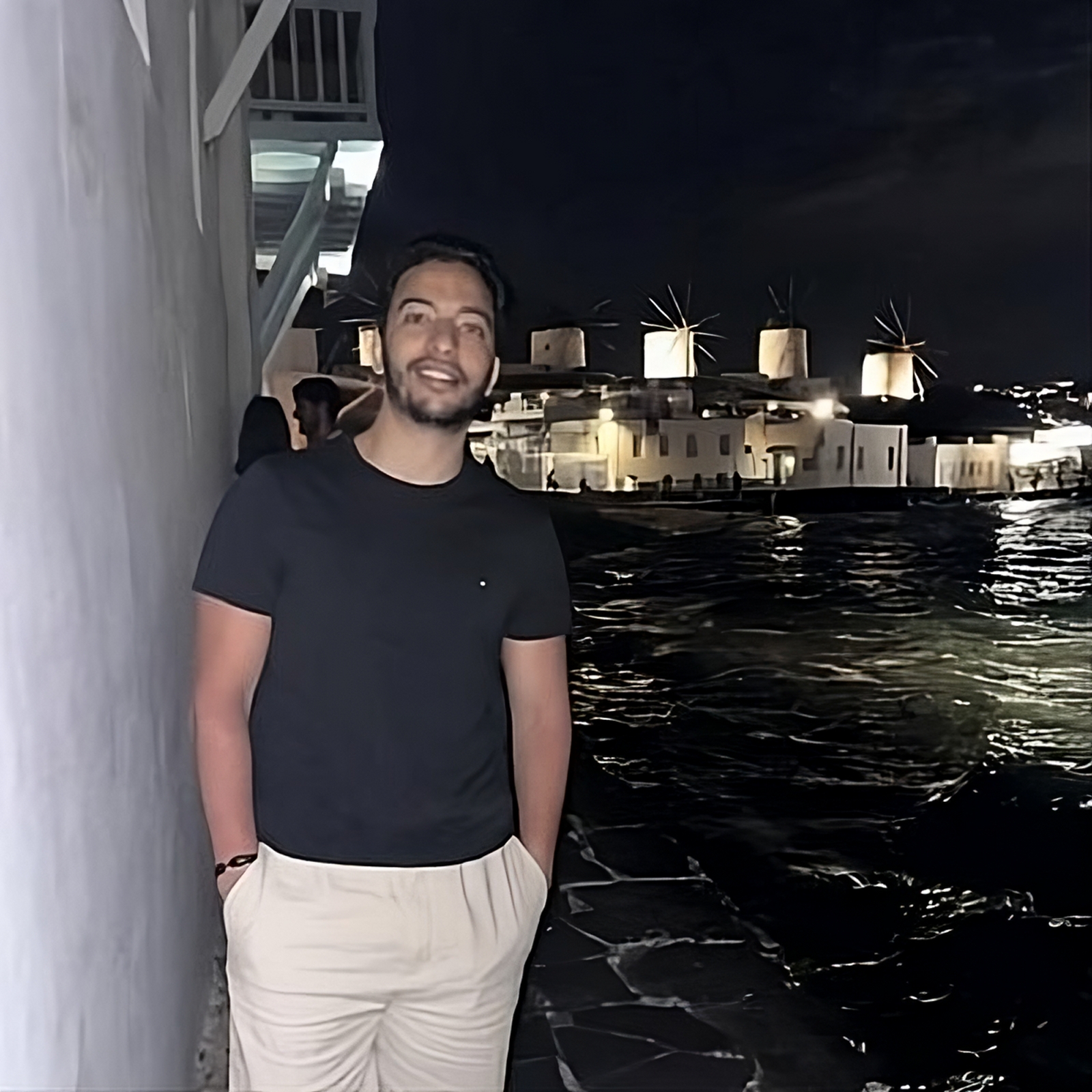
Ayoub Touba
Ayoub Touba
With over a decade of hands-on experience, I specialize in building robust web applications and scalable software solutions. My expertise spans across cutting-edge frameworks and technologies, including Node.js, React, Angular, Vue.js, and Laravel. I also delve into hardware integration with ESP32 and Arduino, creating IoT solutions.