Strengthening API Security: A QA Engineer's Guide to the OWASP Top 10
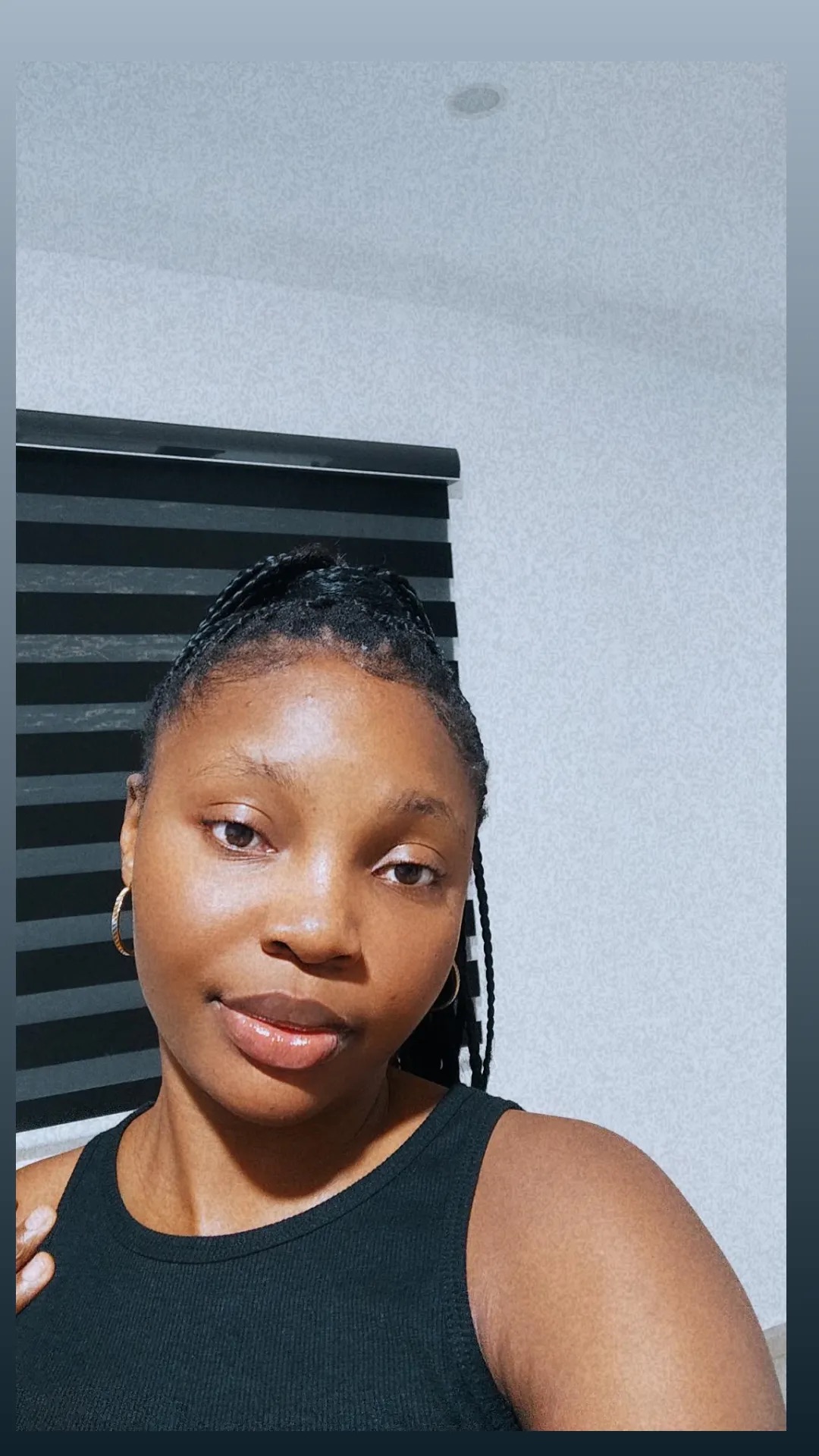
APIs are everywhere. They're the silent workhorses powering your favourite apps, from ordering food to streaming movies and so much more. But with great power comes great responsibility. And in the world of software, that responsibility falls partly on your shoulders. As a QA engineer, you're the last line of defence against a bad API.
Imagine this: a single vulnerability in your API could mean sensitive customer data leaked, financial losses, or even a damaged reputation. Scary, right? That's where I come in.
This guide is your toolkit to prevent those nightmares. We will delve into the OWASP Top 10 API Security Risks and provide practical guidance on how to address them. By following these best practices, you can significantly strengthen the security of your APIs and protect your organization from potential vulnerabilities.
Understanding the OWASP Top 10
So, you know about API security, but the OWASP Top 10? Let's break it down. Imagine the Top 10 as your ultimate API security checklist. It's a curated list of the most common and dangerous threats lurking in your APIs.
Think of it like this: you wouldn't build a house without checking for structural integrity, right? Just as a house needs a strong foundation and sturdy walls, an API needs a solid security framework to withstand potential threats.
The OWASP Top 10 is your blueprint for building secure APIs. It covers everything from improper access control to weak encryption.
We'll dive into these ten critical areas, breaking them down into simple terms and providing practical examples. You'll learn how to spot potential vulnerabilities and, most importantly, how to fix them.
Security Misconfiguration
Security misconfiguration is like leaving the front door unlocked and the welcome mat out. It's an open invitation for troublemakers to waltz right in. In the digital world, it means your API is vulnerable to attack due to careless configuration. From default settings to exposed endpoints, these oversights can be a hacker's dream come true.
Common Examples of Misconfigurations
Default error messages: Revealing sensitive information about the API or system architecture.
Insecure HTTP methods: Allowing methods like PUT or DELETE without proper authentication.
Missing or weak input validation: Failing to sanitize user input, leading to injection vulnerabilities.
Exposed sensitive data: Exposing sensitive information in API responses.
Incorrect CORS configuration: Allowing unauthorized origins to access the API.
Weak encryption: Using outdated or weak encryption algorithms.
Test Cases for checking misconfigurations
To identify security misconfigurations, incorporate these test cases into your testing strategy:
Broken Object Level Authorization
This occurs when APIs do not properly validate user permissions, allowing unauthorized access to sensitive data.
For example, while RBAC (role-based access control) might grant a "customer" role read access to "orders," object-level authorization ensures a customer can only view their orders, not someone else's.
Common Vulnerabilities Related to Object Level Authorization
Insecure direct object references (IDORs): Directly manipulating object identifiers (e.g., changing a product ID) to access unauthorized data.
Insufficient access control checks: Failing to verify user permissions for each object accessed.
Horizontal privilege escalation: Exploiting vulnerabilities to access data or perform actions belonging to other users with the same role.
Data exposure: Exposing sensitive data through object retrieval.
Testing for Broken Object Level Authorization
ID manipulation: Modify object identifiers in API requests to test access controls.
Session hijacking: Attempt to access other users' data by stealing or manipulating session tokens.
Authorization bypass: Try to bypass authorization checks through different request methods or parameters.
Data exposure testing: Check if sensitive data is exposed when retrieving objects.
Test Cases for Implementing Object-Level Authorization
Injecting Security
Injection attacks occur when malicious code is inserted into an application. While SQL injection is the most well-known, other types include:
SQL Injection: Exploiting vulnerabilities in SQL queries to manipulate or extract data.
NoSQL Injection: Targeting NoSQL databases with malicious input to modify or extract data.
Command Injection: Injecting operating system commands into an application.
OS Command Injection: Similar to command injection, but specifically targets operating system commands.
LDAP Injection: Exploiting vulnerabilities in LDAP directories.
XML Injection: Injecting malicious XML content to manipulate application logic.
Impact of Injection Attacks
Injection attacks can have devastating consequences:
Data loss: Sensitive data can be stolen or deleted.
System compromise: Attackers can gain unauthorized access to the system.
Service disruption: Injection attacks can cause system crashes or denial-of-service conditions.
Financial loss: Unauthorized transactions or fraudulent activities can occur.
Reputation damage: Loss of customer trust and confidence.
Test Cases for Checking Injections
Mass Assignment: The Overwrite Threat
Mass assignment occurs when an application blindly assigns all incoming data to an object without proper validation. This can lead to severe security implications if the object contains sensitive fields.
Imagine a user profile with fields like name, email, and password. A malicious user could send a request to update their profile, but also include a field called "isAdmin" with the value "true." If the application isn't careful, the user could inadvertently be granted administrator privileges.
How Mass Assignment Can Lead to Data Breaches
Privilege escalation: Users can elevate their privileges by modifying sensitive fields.
Data corruption: Incorrect or malicious data can overwrite important information.
Unauthorized access: Attackers can bypass access controls by modifying sensitive fields.
Test Cases for Mass Assignment
Security Logging and Monitoring: Your Digital Watchtower
Importance of Logging and Monitoring for API Security
Logging and monitoring are the eyes and ears of your API. They provide invaluable insights into system behaviour, detect anomalies, and help you respond to security incidents promptly. Effective logging and monitoring can:
Identify threats: Detect suspicious activities and potential attacks.
Investigate incidents: Gather evidence for forensic analysis and incident response.
Comply with regulations: Meet industry standards and regulatory requirements.
Improve performance: Identify bottlenecks and optimize system performance.
Key Information to Log
To effectively monitor your API, you should log:
API requests and responses: Include timestamps, request/response headers, and bodies.
User activity: Track user logins, logouts, failed attempts, and role changes.
Error messages: Record detailed error messages with timestamps and relevant context.
System events: Log system-level events like restarts, configuration changes, and software updates.
Security events: Capture security-related events such as failed logins, unauthorized access attempts, and intrusion detection alerts.
Testing Your Logging and Monitoring
While this is not traditional test cases, you can indirectly test the effectiveness of your logging and monitoring by:
API and Version Management
Best Practices for API Versioning
Effective API versioning is crucial for maintaining compatibility while allowing for evolution. Key best practices include:
Clear versioning scheme: Use a consistent and understandable versioning format (e.g., Major.Minor.Patch).
Breaking changes: Introduce new major versions for significant changes that break backward compatibility.
Deprecation policy: Clearly communicate deprecated endpoints and provide timelines for removal.
Documentation: Maintain detailed documentation for each API version.
Testing: Thoroughly test new API versions to ensure compatibility and functionality.
Migration plan: Develop a strategy for guiding consumers to adopt new API versions.
Risks Associated with Poor Version Management
Neglecting API version management can lead to:
Application failures: Incompatible API changes causing client errors.
Increased support costs: Handling issues related to API changes.
Lost revenue: Customers migrating to competitors due to API disruptions.
Security risks: Exploiting vulnerabilities in older, unsupported API versions.
Developer frustration: Difficulty managing multiple API versions.
Test Cases for API Version Compatibility
Insecure Authentication/Authorization
Strong authentication is the first line of defence against unauthorized access. It ensures that only legitimate users can access your system. A robust authentication mechanism protects sensitive data and prevents unauthorized actions.
Weak or compromised authentication mechanisms can enable attackers to gain unauthorized access to APIs.
Common Authentication Vulnerabilities
Weak passwords: Users often choose easily guessable passwords.
Brute-force attacks: Automated attempts to guess passwords.
Credential stuffing: Using stolen credentials from one site to access another.
Session hijacking: Stealing or manipulating session tokens.
Insufficient lockout policies: Allowing unlimited login attempts.
Missing or weak multi-factor authentication (MFA): Relying solely on passwords.
Test Cases for Authentication Mechanisms
Inadequate Privacy Controls
This refers to the lack of or improper authorization validation at the object property level, leading to information exposure or manipulation.
Excessive data exposure occurs when an API reveals more information than necessary. It's like leaving a treasure chest unlocked and unattended. Sensitive data, if exposed, can lead to significant consequences, including:
Identity theft: Exposure of personal information.
Financial loss: Disclosure of financial data.
Reputation damage: Loss of trust due to data breaches.
Regulatory penalties: Non-compliance with data protection regulations.
Protecting Sensitive Data
To safeguard sensitive data, implement the following measures:
Data minimization: Only expose data essential for the requested operation.
Data masking: Replace sensitive information with non-sensitive equivalents for testing and production environments.
Access controls: Enforce strict access controls based on user roles and permissions.
Encryption: Protect data at rest and in transit using strong encryption algorithms.
Regular audits: Conduct security audits to identify potential data exposure risks.
Test Cases for Data Exposure
Breaking Cryptography
Cryptography is the art of protecting information by transforming it into an unreadable format, accessible only to those with the correct decryption key. For APIs, cryptography is crucial for securing data transmission, authentication, and integrity.
Key concepts:
Encryption: Converting plaintext into ciphertext.
Decryption: Reversing the encryption process.
Symmetric encryption: Uses a single key for encryption and decryption.
Asymmetric encryption: Uses a public key for encryption and a private key for decryption.
Hashing: Creating a fixed-size representation of data for verification.
Digital signatures: Verifying the authenticity and integrity of data.
Common Cryptographic Vulnerabilities
While cryptography is powerful, it's susceptible to misuse:
Weak algorithms: Using outdated or insecure encryption algorithms.
Short key lengths: Using keys that are too short to resist brute-force attacks.
Improper key management: Storing or transmitting keys insecurely.
Oracle attacks: Exploiting information leaked through encryption processes.
Implementation errors: Incorrect use of cryptographic libraries or APIs.
Test Cases for Cryptographic Implementations
Testing Techniques and Best Practices
To address these risks, QA engineers can employ various testing techniques, including:
Vulnerability scanning: Use tools like OWASP ZAP, Burpsuite and others to identify potential vulnerabilities.
Manual testing: Simulate attacks and test for weaknesses in API security.
Code review: Analyze code for security vulnerabilities.
Threat modeling: Identify potential threats and assess their impact.
Security testing frameworks: Utilize specialized frameworks to automate security testing.
Remember, API security is an ongoing journey. Stay updated on the latest threats and best practices. Keep testing, keep learning, and most importantly, keep your APIs safe! ๐ก๏ธ๐ช
Let's work together to build a safer digital world. Happy testing! ๐
Subscribe to my newsletter
Read articles from Esther Okafor directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
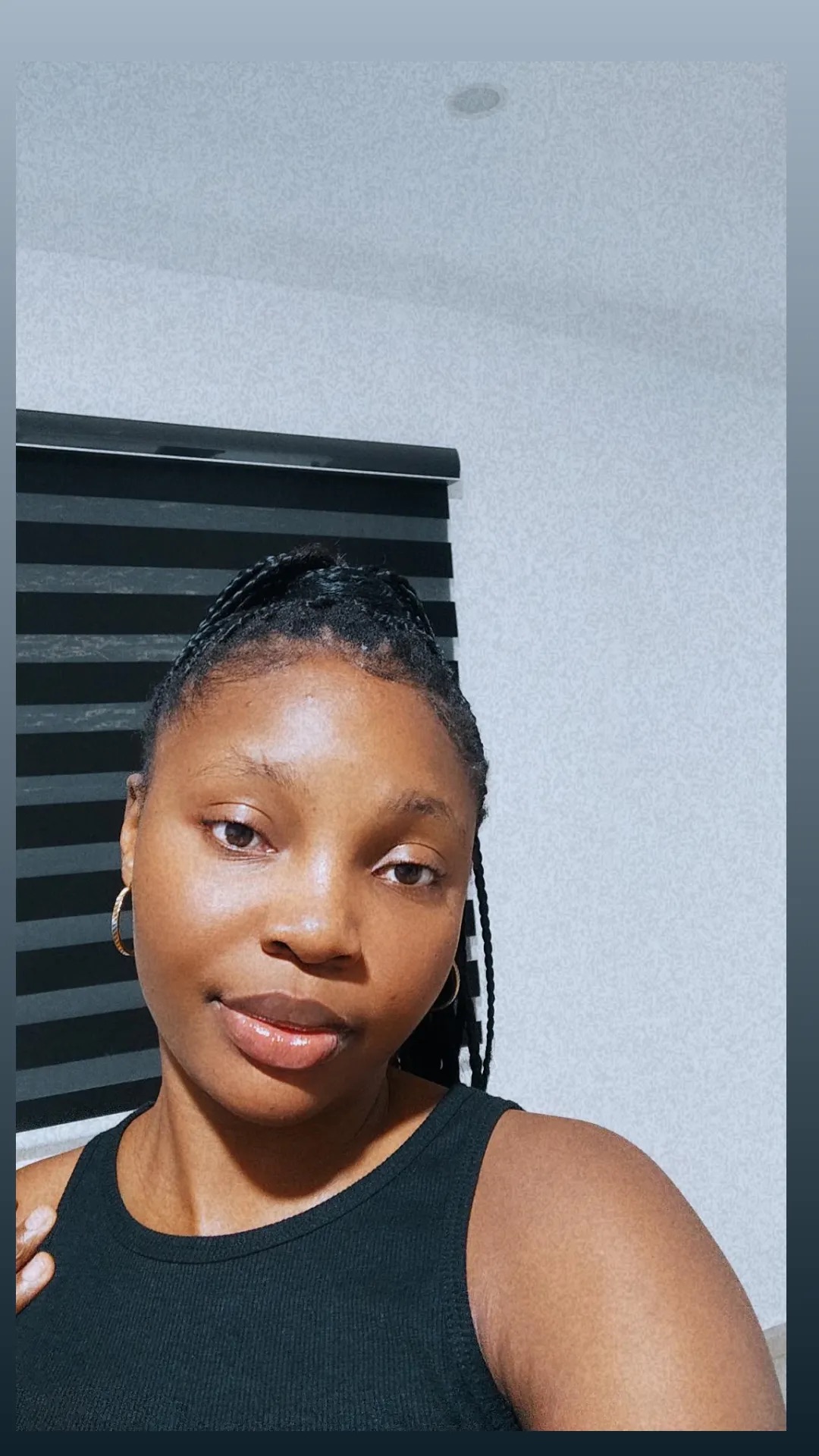
Esther Okafor
Esther Okafor
Esther Okafor is a Quality assurance engineer passionate about delivering great user experience applications. Prioritizing quality over speed