Binary Search Guide: Comparing Recursive and Iterative Methods
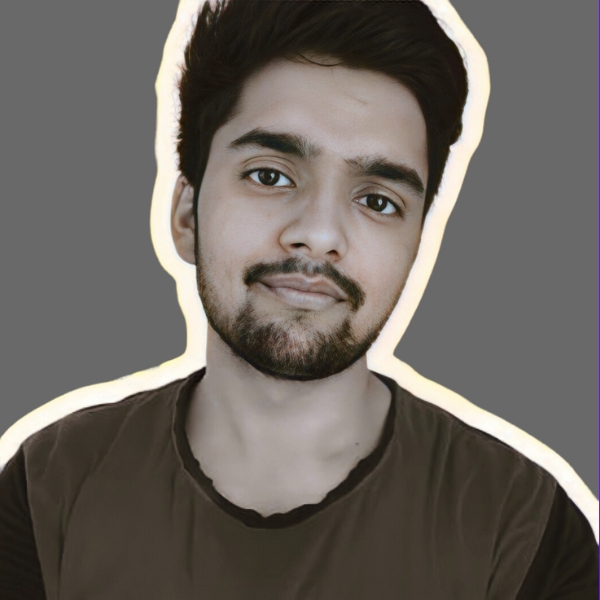
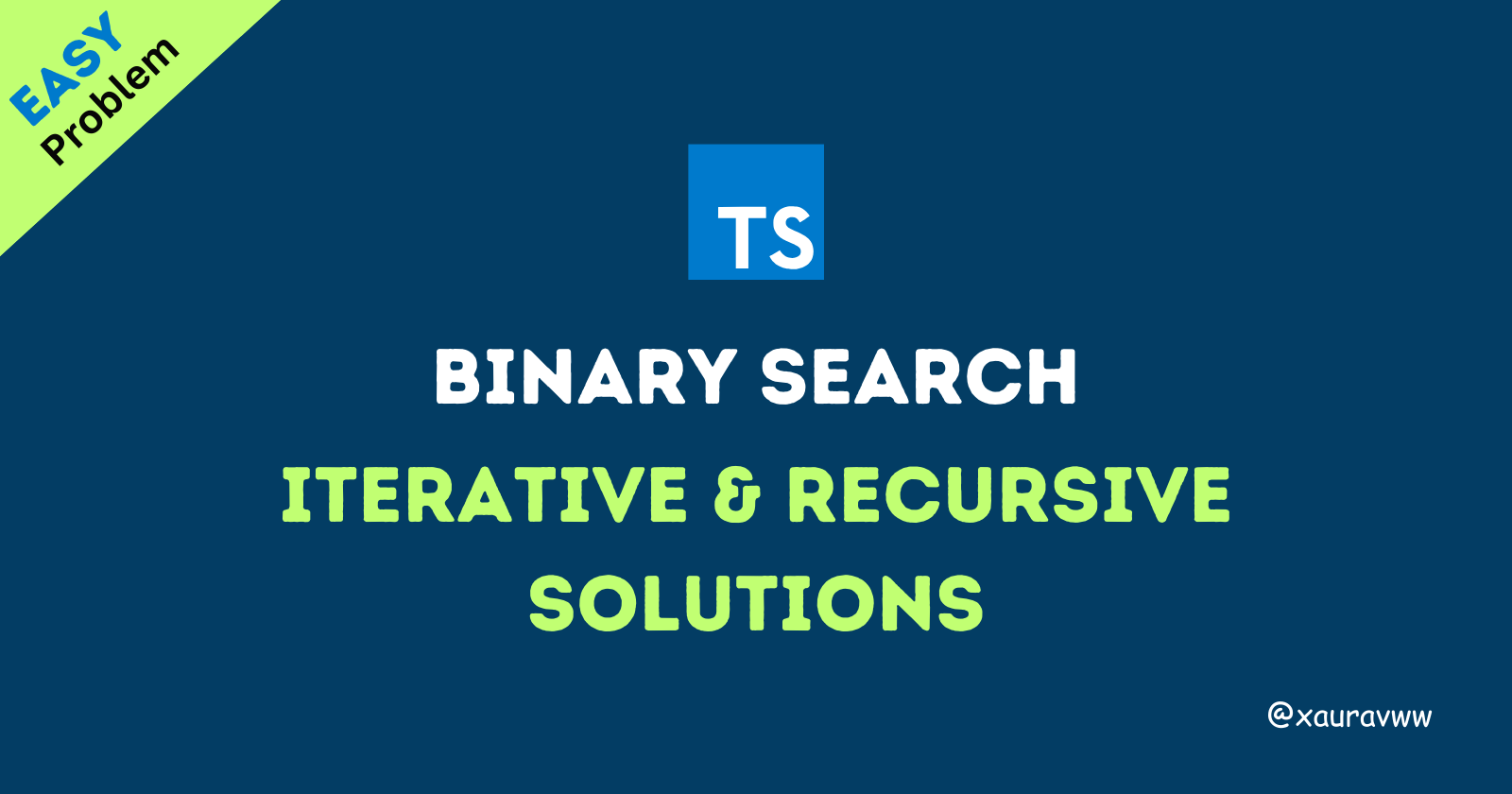
What is Binary Search?
Binary search is a highly efficient algorithm used to locate a target value within a sorted array. By dividing the search space in half with each step, it reduces the time complexity to O(log n). In this blog, we'll explore both recursive and iterative solutions for binary search in TypeScript.
Recursive Solution
function search(nums: number[], target: number): number {
return binarySearch(nums, target, 0, nums.length - 1);
}
function binarySearch(nums: number[], target: number, left: number, right: number): number {
// Base case
if (left > right) return -1;
let mid = Math.floor((left + right) / 2);
if (nums[mid] === target) {
return mid;
}
else if (nums[mid] < target) {
return binarySearch(nums, target, mid + 1, right);
}
else {
return binarySearch(nums, target, left, mid - 1);
}
}
How the Recursive Solution Works:
Function Call:
search(nums, target)
initializes the search by callingbinarySearch
with the full array bounds.Base Case: If
left
exceedsright
, the target is not in the array, so return-1
.Middle Index: Compute the middle index,
mid = Math.floor((left + right) / 2)
.Comparison:
If
nums[mid]
equals the target, returnmid
.If
nums[mid]
is less than the target, search the right half by callingbinarySearch
with updated bounds.If
nums[mid]
is greater than the target, search the left half by callingbinarySearch
with updated bounds.
Iterative Solution
The iterative solution uses a loop instead of recursive calls, which can be more efficient in terms of space since it avoids the overhead of multiple function calls.
function search(nums: number[], target: number): number {
let left = 0;
let right = nums.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (nums[mid] === target) {
return mid;
}
else if (nums[mid] < target) {
left = mid + 1;
}
else {
right = mid - 1;
}
}
// Target is not found in the array
return -1;
}
How the Iterative Solution Works:
Initialize Pointers: Set
left
to the start of the array andright
to the end.Loop: Continue while
left
is less than or equal toright
.Middle Index: Compute the middle index,
mid = Math.floor((left + right) / 2)
.Comparison:
If
nums[mid]
equals the target, returnmid
.If
nums[mid]
is less than the target, updateleft
tomid + 1
.If
nums[mid]
is greater than the target, updateright
tomid - 1
.
Target Not Found: If the loop exits without finding the target, return
-1
.
Time and Space Complexity
Time Complexity: Both recursive and iterative binary search have a time complexity of O(log n), where
n
is the number of elements in the array.Space Complexity:
Recursive: O(log n) due to the call stack used in recursion.
Iterative: O(1) as it only uses a few variables.
Comment if I have committed any mistake. Let's connect on my socials. I am always open for new opportunities , if I am free :P
Subscribe to my newsletter
Read articles from Saurav Maheshwari directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
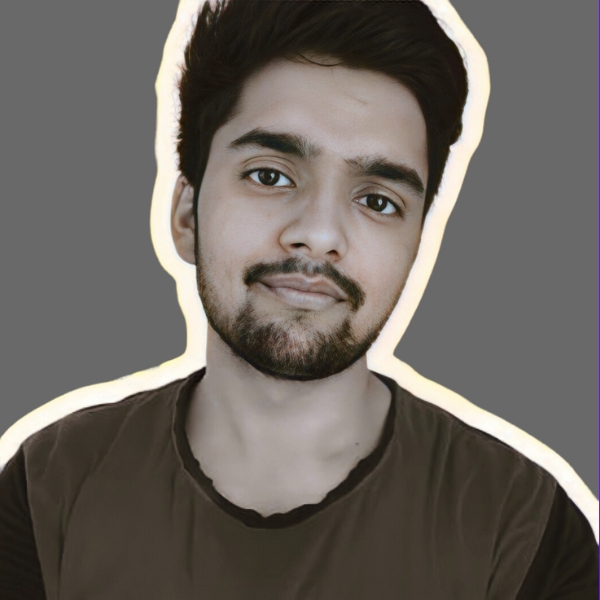