Essential Docker Commands with examples

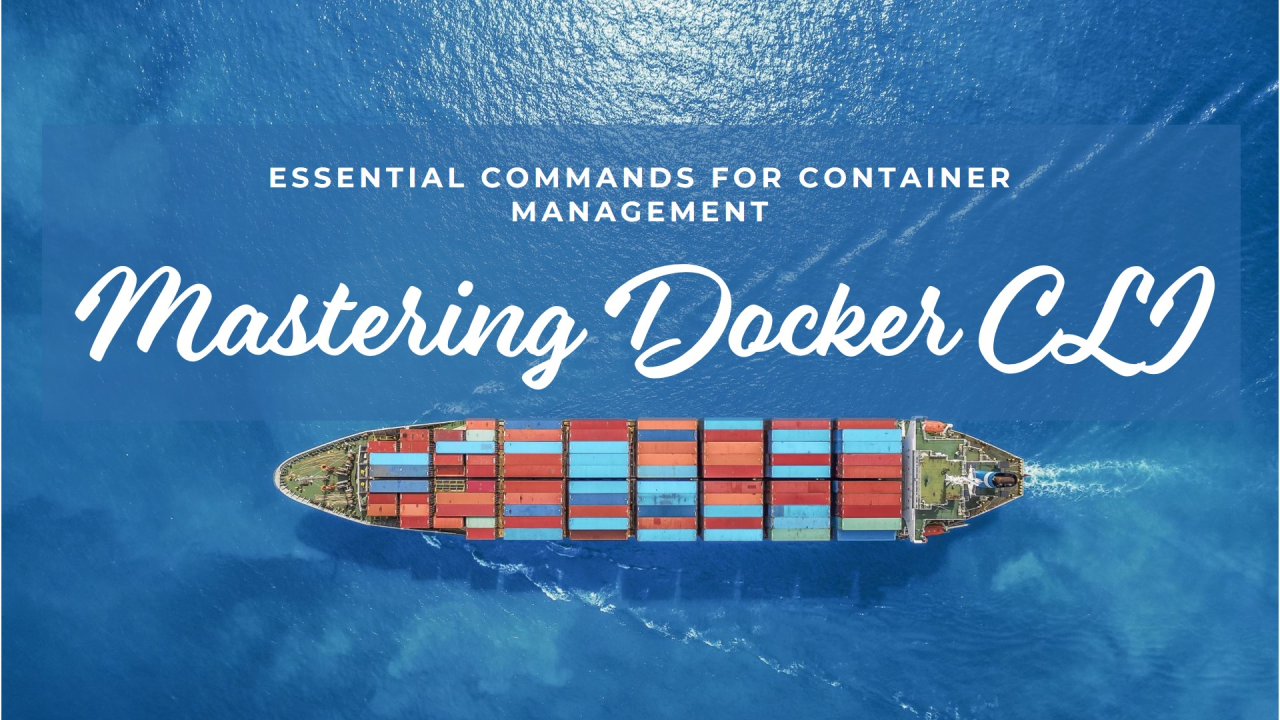
Introduction
In the fast-paced world of DevOps, Docker is a game-changer. It helps us build, ship, and run applications smoothly. Docker commands are the tools that make this possible. They let developers and DevOps pros manage containers, images, volumes, and networks easily. Whether you’re working on complex microservices or just trying to make your development process smoother, knowing these commands is key. This guide will walk you through the most important Docker commands, making your container journey easier and more efficient.
Key Docker Commands
Docker Version and Login Commands
docker --version
Explanation: Displays the installed Docker version.
Example:
docker --version
- Shows the current version of Docker installed on your system.
docker login
Explanation: Logs in to Docker Hub or a private registry.
Example:
docker login
- Prompts for Docker Hub credentials to authenticate your session.
docker login -u <username> -p <password>
Explanation: Logs in to Docker with the specified username and password.
Example:
docker login -u myusername -p mypassword
- Authenticates you to Docker Hub with provided credentials.
docker login <registry_url>
Explanation: Logs in to a specific Docker registry.
Example:
docker login
myregistry.com
- Authenticates you to a custom Docker registry.
Image Commands
docker pull <image>:<tag>
Explanation: Downloads an image from a Docker registry.
Example:
docker pull nginx:latest
- Fetches the latest version of the NGINX image from Docker Hub.
docker image ls
Explanation: Lists all images on your local system.
Example:
docker image ls
- Displays a list of Docker images available on your machine.
docker image history <image>
Explanation: Shows the history of an image.
Example:
docker image history nginx
- Lists the layers and creation history of the NGINX image.
docker build -t <image_name>
Explanation: Builds an image from a Dockerfile.
Example:
docker build -t myapp:latest .
- Creates a Docker image from the Dockerfile in the current directory, tagging it asmyapp:latest
.
docker build -t <image_name> --no-cache
Explanation: Builds an image without using cache.
Example:
docker build -t myapp:latest --no-cache .
- Forces a fresh build of the image, ignoring any cached layers.
docker rmi <image_name>
Explanation: Removes an image by name.
Example:
docker rmi myapp:latest
- Deletes the Docker image namedmyapp:latest
from your system.
docker rmi <image_id>
Explanation: Removes an image by ID.
Example:
docker rmi a1b2c3d4e5f6
- Deletes the Docker image identified by the specified image ID.
docker rmi -f <image_id>
Explanation: Forcefully removes an image.
Example:
docker rmi -f a1b2c3d4e5f6
- Deletes the Docker image, even if it is being used by containers.
docker image prune
Explanation: Removes unused images.
Example:
docker image prune
- Cleans up images that are not associated with any containers.
docker rmi $(docker images -q)
Explanation: Removes all images at once (will not remove images used by running containers).
Example:
docker rmi $(docker images -q)
- Deletes all Docker images from your system that are not in use by running containers.
Container Commands
docker ps
Explanation: Lists currently running containers.
Example:
docker ps
- Displays details of containers that are currently running.
docker ps -a
Explanation: Lists all containers (running and stopped).
Example:
docker ps -a
- Shows all containers, including those that are stopped.
docker start <container_id/name>
Explanation: Starts a stopped container.
Example:
docker start mycontainer
- Restarts the container namedmycontainer
.
docker stop <container_id/name>
Explanation: Stops a running container.
Example:
docker stop mycontainer
- Shuts down the container namedmycontainer
.
docker container ls
Explanation: Lists all containers (running).
Example:
docker container ls
- Shows a list of containers currently running.
docker container pause <container_id/name>
Explanation: Pauses a container.
Example:
docker container pause mycontainer
- Pauses the container namedmycontainer
, stopping its execution without removing it.
docker container unpause <container_id/name>
Explanation: Unpauses a container.
Example:
docker container unpause mycontainer
- Resumes execution of the paused container namedmycontainer
.
docker container rm <container_id/name>
Explanation: Removes a stopped container.
Example:
docker container rm mycontainer
- Deletes the container namedmycontainer
, provided it is not running.
docker rm -vf <container_id/name>
Explanation: Forcefully removes a container and its volumes.
Example:
docker rm -vf mycontainer
- Deletes the container namedmycontainer
along with any associated volumes.
docker exec -it <container_id/name> <command>
Explanation: Executes a command inside a running container.
Example:
docker exec -it mycontainer /bin/bash
- Opens an interactive bash shell inside the container namedmycontainer
.
docker attach <container_id/name>
Explanation: Attaches to a running container.
Example:
docker attach mycontainer
- Connects your terminal to the container namedmycontainer
, allowing you to see its output.
docker logs <container_id/name>
Explanation: Fetches logs from a container.
Example:
docker logs mycontainer
- Displays the logs generated by the container namedmycontainer
.
docker commit <container_id/name> <image_name>:<tag>
Explanation: Creates a new image from a container's changes.
Example:
docker commit mycontainer myapp:latest
- Saves the current state of the container namedmycontainer
as a new imagemyapp:latest
.
docker run -it --name <container_name> <image>
Explanation: Creates and starts a container interactively.
Example:
docker run -it --name mycontainer ubuntu
- Runs a new container from theubuntu
image and opens an interactive terminal.
docker run -d -p <host_port>:<container_port> <image>
Explanation: Runs a container in detached mode with port mapping.
Example:
docker run -d -p 80:80 nginx
- Starts a new NGINX container in detached mode and maps port 80 on the host to port 80 in the container.
docker run -it --name <container_name> -v <host_dir>:<container_dir> <image>
Explanation: Runs a container with a volume mount.
Example:
docker run -it --name mycontainer -v /host/data:/container/data ubuntu
- Runs a new container from theubuntu
image with a volume mounted from/host/data
on the host to/container/data
in the container.
docker run -d --name <container_name> --mount type=<type>,source=<source>,target=<target> <image>
Explanation: Runs a container with a mount.
Example:
docker run -d --name mycontainer --mount type=bind,source=/host/data,target=/container/data ubuntu
- Starts a new container with a bind mount from/host/data
on the host to/container/data
in the container.
docker run --rm <image>
Explanation: Automatically removes the container and its file system when it exits.
Example:
docker run --rm nginx
- Runs a container from the NGINX image and removes it after it exits.
docker rename <container_name> <new_name>
Explanation: Renames a container.
Example:
docker rename oldname newname
- Changes the name of the container fromoldname
tonewname
.
docker rm -v <container_name>
Explanation: Removes a container and its associated volumes.
Example:
docker rm -v mycontainer
- Deletes the container namedmycontainer
and its associated volumes.
docker rm $(docker ps -a -f status=exited -q)
Explanation: Removes all exited containers.
Example:
docker rm $(docker ps -a -f status=exited -q)
- Deletes all containers that have exited.
docker port <container_name/id>
Explanation: Lists the port mappings for a container.
Example:
docker port mycontainer
- Shows the port mappings for the container namedmycontainer
.
Volume Commands
docker volume create <volume_name>
Explanation: Creates a new volume.
Example:
docker volume create myvolume
- Creates a Docker volume namedmyvolume
.
docker volume ls
Explanation: Lists all volumes.
Example:
docker volume ls
- Displays a list of all Docker volumes on your system.
docker volume inspect <volume_name>
Explanation: Shows detailed information about a volume.
Example:
docker volume inspect myvolume
- Provides information about the volume namedmyvolume
.
docker volume rm <volume_name>
Explanation: Removes a volume.
Example:
docker volume rm myvolume
- Deletes the Docker volume namedmyvolume
.
docker volume prune
Explanation: Removes all unused volumes.
Example:
docker volume prune
- Cleans up volumes that are not used by any containers.
Network Commands
docker network create <network_name>
Explanation: Creates a new network.
Example:
docker network create mynetwork
- Creates a Docker network namedmynetwork
.
docker network ls
Explanation: Lists all networks.
Example:
docker network ls
- Shows a list of all Docker networks on your system.
docker network inspect <network_name>
Explanation: Shows detailed information about a network.
Example:
docker network inspect mynetwork
- Provides information about the network namedmynetwork
.
docker network rm <network_name>
Explanation: Removes a network.
Example:
docker network rm mynetwork
- Deletes the Docker network namedmynetwork
.
System Commands
docker system df
Explanation: Displays disk usage of Docker components.
Example:
docker system df
- Shows the amount of disk space used by Docker images, containers, and volumes.
docker system prune
Explanation: Removes unused data (containers, networks, images).
Example:
docker system prune
- Cleans up unused Docker resources, but keeps stopped containers.
docker system prune -a
Explanation: Removes all unused containers, images, and networks.
Example:
docker system prune -a
- Cleans up all unused Docker resources, including images not referenced by any containers.
docker stats
Explanation: Displays real-time statistics of running containers.
Example:
docker stats
- Shows CPU, memory, and network usage of all running containers.
Conclusion
Docker commands are more than just tools; they are the foundation of modern app deployment and management. By mastering these commands, you can unlock Docker’s full potential, making your development and production processes smoother and more efficient. As you continue to explore and use these commands, you’ll be at the forefront of the containerization revolution, driving innovation and efficiency in your DevOps practices. Happy Dockering!
Subscribe to my newsletter
Read articles from Sandhya Babu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sandhya Babu
Sandhya Babu
🌟 Aspiring DevOps Engineer | Cloud & DevOps Enthusiast🌟 Hello! I’m Sandhya Babu, deeply passionate about DevOps and cloud technologies. Currently in my exploring phase, I’m learning something new every day, from tools like Jenkins, Docker, and Kubernetes to the concepts that drive modern tech infrastructures. I have hands-on experience with several Proof of Concept (POC) projects, where I've applied my skills in real-world scenarios. I love writing blogs about what I've learned and sharing my experiences with others, hoping to inspire and connect with fellow learners. With certifications in Azure DevOps and AWS SAA-C03, I’m actively seeking opportunities to apply my knowledge, contribute to exciting projects, and continue growing in the tech industry. Let’s connect and explore the world of DevOps together!