⚡️Boost Your Testing with Playwright’s Multi-Browser Capabilities ⚡️
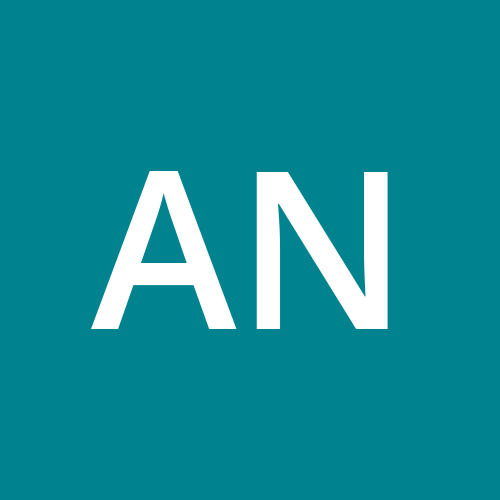
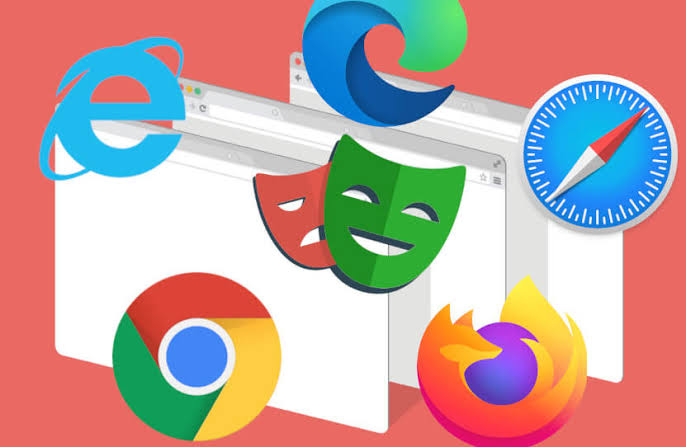
Introduction 🔍
In the fast-paced world of web development, ensuring that your web applications perform seamlessly across different browsers is crucial. Playwright offers powerful features to help you achieve this with ease. In this blog, we’ll explore how to set up multi-browser testing using Playwright, leveraging environment variables and a switch statement to streamline your testing process.
Setting Up Playwright 🛠️
Before diving into multi-browser testing, ensure you have Playwright installed. If not, you can install it using the following command:
npm install dotenv
We’ll also use the dotenv
package to manage environment variables, making it easier to switch between browsers without changing your code.
Configuring Environment Variables 🌍
Create a .env
file in your project’s root directory to define the browser you want to use for testing. This file allows you to easily switch browsers by changing a single line. Here’s how you set it up:
Create the
.env
File:BROWSER_TYPE=chromium
You can replace
chromium
withfirefox
orwebkit
depending on the browser you want to test with.Load Environment Variables in Your Script:
Update your Playwright script to use these variables. Below is an example script that utilizes a switch statement to select the browser based on the
BROWSER_TYPE
environment variable.
Multi-Browser Testing with Playwright 🌐
Here’s a Playwright script that demonstrates how to set up multi-browser testing using the dotenv
package and a switch statement:
require('dotenv').config(); // Load environment variables from .env file
const { chromium, firefox, webkit } = require('playwright');
(async () => {
// Retrieve the browser type from environment variables
const browserType = process.env.BROWSER_TYPE || 'chromium'; // Default to 'chromium' if not specified
let browser;
switch (browserType) {
case 'firefox':
browser = await firefox.launch();
break;
case 'webkit':
browser = await webkit.launch();
break;
case 'chromium':
default:
browser = await chromium.launch();
break;
}
const context = await browser.newContext();
const page = await context.newPage();
await page.goto('https://example.com');
// Example interaction
console.log('Page Title:', await page.title());
// Add more test steps here
await browser.close();
})();
Explanation of the Script:
Loading Environment Variables:
- The script starts by loading environment variables from the
.env
file usingdotenv
.
- The script starts by loading environment variables from the
Selecting the Browser:
- It then uses a switch statement to launch the browser specified in the
BROWSER_TYPE
variable.
- It then uses a switch statement to launch the browser specified in the
Running Tests:
- After launching the browser, the script creates a new page, navigates to a URL, and performs interactions.
Closing the Browser:
- Finally, it closes the browser to clean up resources.
Running Your Test Script ⚙️
To execute your script, simply run:
npx playwright test your-script.ts
To test with different browsers, update the .env
file with your desired browser type and re-run the script. For example:
To test with Firefox, set
BROWSER_TYPE=firefox
.To test with WebKit, set
BROWSER_TYPE=webkit
.
By using environment variables and switch statements, you can efficiently manage multi-browser testing with Playwright. This method simplifies your testing setup, allowing you to switch between different browsers without changing your codebase. Happy testing! 🎈
Subscribe to my newsletter
Read articles from Anandkumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
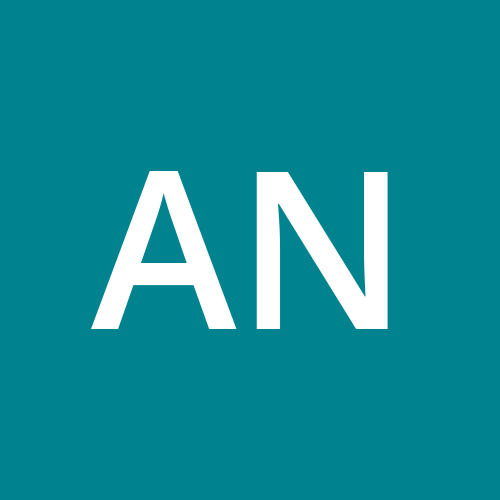
Anandkumar
Anandkumar
"Passionate playwright dedicated to refining scripts through innovative methods. Embracing automated testing to enhance creativity and efficiency in crafting compelling narratives. Let's revolutionize storytelling together!"