A Guide to Breakpoints for Debugging in Visual Studio

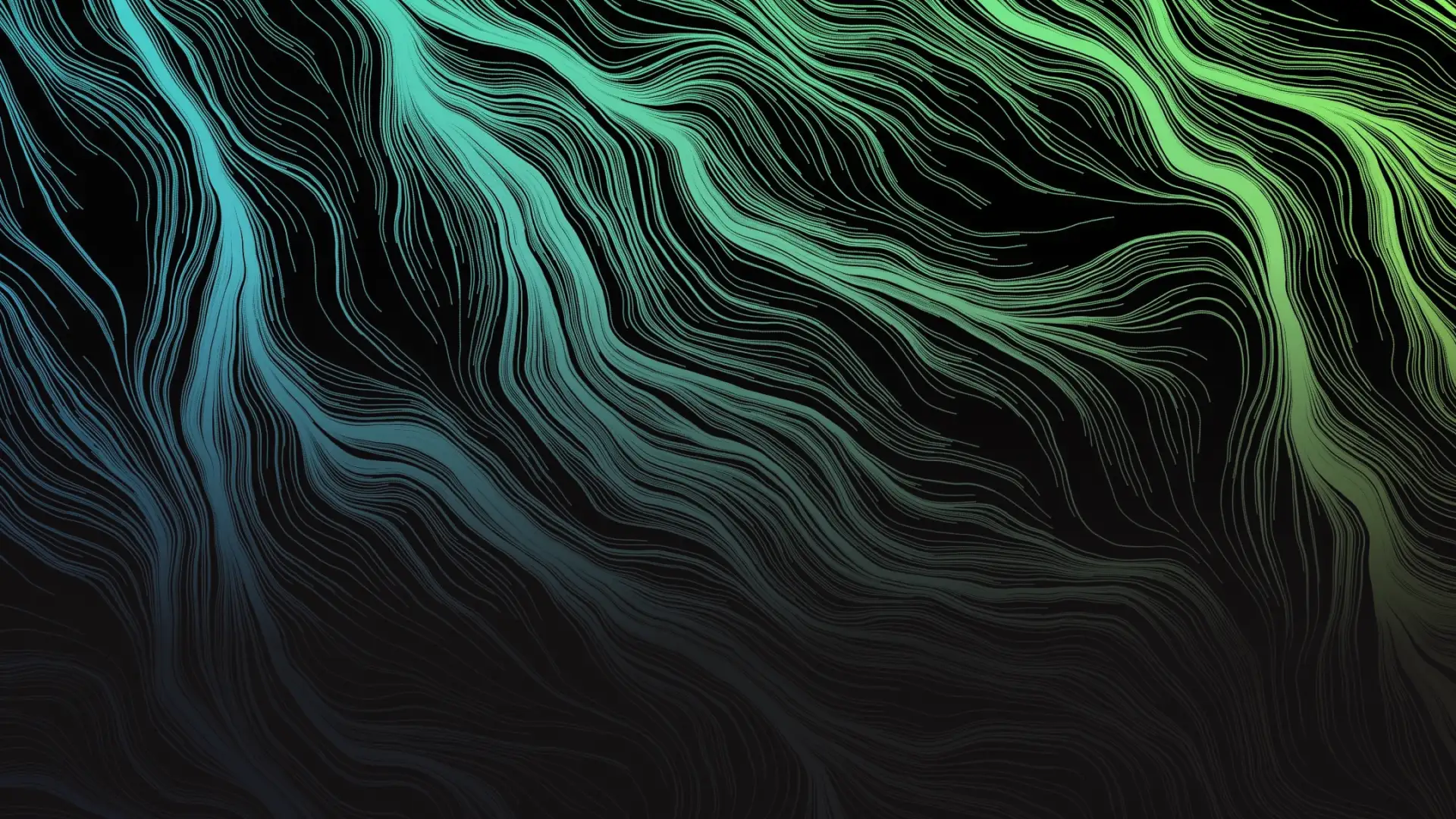
Debugging is a crucial aspect of software development, and breakpoints are one of the most powerful tools that developers have to halt code execution at a specific point for inspection. Visual Studio, one of the most popular Integrated Development Environments (IDE), offers a wide range of breakpoint types that can make the debugging process efficient and streamlined. In this blog, we'll explore the different types of breakpoints available in Visual Studio and when to use each one.
1. Standard Breakpoints
What It Is:
A standard breakpoint is the most basic form of breakpoint. You set it on a specific line of code, and when the program execution reaches that line, the execution is paused.
When to Use:
Standard breakpoints are ideal when you want to pause execution at a particular location and inspect the current state, variables, or flow of the program.
How to Set:
In the code editor, click on the left margin next to the line number where you want to pause the execution.
You can also press
F9
when the cursor is on the desired line.
Example:
int total = 0;
for (int i = 0; i < 10; i++)
{
total += i; // Set a breakpoint here to inspect the value of 'total' at each iteration.
}
2. Conditional Breakpoints
What It Is:
A conditional breakpoint only pauses the execution when a specified condition is met. For example, you can set a condition to break only when a variable reaches a certain value.
When to Use:
Conditional breakpoints are helpful when debugging loops or large datasets where you only care about specific cases or conditions.
How to Set:
Right-click on the existing standard breakpoint and select Conditions.
In the dialog box, set the condition based on an expression, variable, or hit count.
Example:
for (int i = 0; i < 100; i++)
{
// Break when i == 50
if (i == 50)
{
Console.WriteLine(i);
}
}
In this example, you can set a conditional breakpoint that will trigger when i == 50
.
3. Hit Count Breakpoints
What It Is:
A hit count breakpoint allows you to pause execution after a line of code has been executed a certain number of times. For instance, you can set a breakpoint to trigger after 10 iterations of a loop.
When to Use:
This type is useful in loops or repetitive code where the issue occurs after multiple iterations, and you don’t want to stop the execution every single time.
How to Set:
- Right-click on a standard breakpoint, select Hit Count, and enter the desired count (e.g., break after 5 hits).
Example:
for (int i = 0; i < 100; i++)
{
// Set a hit count breakpoint to trigger after 10 iterations
Console.WriteLine(i);
}
4. Function Breakpoints
What It Is:
A function breakpoint halts execution when a specific function is entered, regardless of where it’s called from.
When to Use:
This is useful when you're interested in tracking down bugs across multiple instances where a specific function is invoked, especially in large codebases where finding all function calls manually can be cumbersome.
How to Set:
Go to the Debug menu, select New Breakpoint, then choose Function Breakpoint.
Enter the function name you want to break on.
Example:
void CalculateTotal()
{
// Break whenever this function is called
Console.WriteLine("Calculating...");
}
5. Data Breakpoints (C++ Only)
What It Is:
A data breakpoint pauses the execution when the value of a specific variable changes, rather than when a line of code is reached.
When to Use:
Data breakpoints are excellent when you’re tracking down a bug caused by unintended modifications to a variable's value. This is especially useful when working with complex data structures.
How to Set:
- Right-click on the variable in the Watch or Autos window and select Break When Value Changes.
Example (C++ Only):
int myVar = 10;
// Set a data breakpoint to pause execution when 'myVar' is modified.
myVar = 20;
6. Exception Breakpoints
What It Is:
An exception breakpoint triggers when an exception is thrown in your code, regardless of where it occurs. You can choose to break on all exceptions or specific types of exceptions (e.g., null reference exceptions, divide-by-zero exceptions).
When to Use:
Use this when you're debugging an exception that isn't being handled as expected, or when you want to catch an exception at the moment it's thrown, rather than when it's caught.
How to Set:
Go to the Debug menu, choose Windows, and then select Exception Settings.
Check the box next to the exception type you want to break on.
Example:
try
{
int result = 10 / 0; // This will trigger a divide-by-zero exception.
}
catch (DivideByZeroException ex)
{
Console.WriteLine(ex.Message);
}
7. Tracepoints
What It Is:
A tracepoint is a special kind of breakpoint that doesn’t halt execution but logs a message to the output window when reached.
When to Use:
Tracepoints are useful when you want to log information during runtime, such as the value of variables or tracking how often a piece of code is executed, without interrupting the flow of the program.
How to Set:
Right-click on a standard breakpoint and select When Hit.
In the dialog, enter the message or variable values you want to print when the breakpoint is hit.
Example:
int total = 0;
for (int i = 0; i < 10; i++)
{
total += i; // Set a tracepoint here to log the value of 'total' at each iteration.
}
8. Temporary Breakpoints
What It Is:
A temporary breakpoint is a breakpoint that will automatically remove itself after being hit once.
When to Use:
This type of breakpoint is helpful when you only need to break at a specific point once, after which you want the program to continue uninterrupted.
How to Set:
Right-click on a standard breakpoint and choose Conditions.
Under the Action tab, select "Remove Breakpoint After It’s Hit."
Example:
int result = 0;
for (int i = 0; i < 5; i++)
{
result += i; // Set a temporary breakpoint here to hit once, then remove automatically.
}
Conclusion
Breakpoints are a core part of the debugging process in Visual Studio, and understanding the different types available can save you time and effort when troubleshooting. Whether you're stopping at specific lines with standard breakpoints, inspecting only under certain conditions, or tracking variable changes with data breakpoints, Visual Studio gives you a flexible set of tools for efficiently identifying and resolving bugs.
Mastering these breakpoints can significantly enhance your debugging skills and help you build more reliable, error-free applications. So next time you're debugging, experiment with these different types of breakpoints and find out which ones best suit your needs!
Subscribe to my newsletter
Read articles from Mohammed Omar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
