[2024] Guide: How to Convert TIFF to PDF and Back in Python

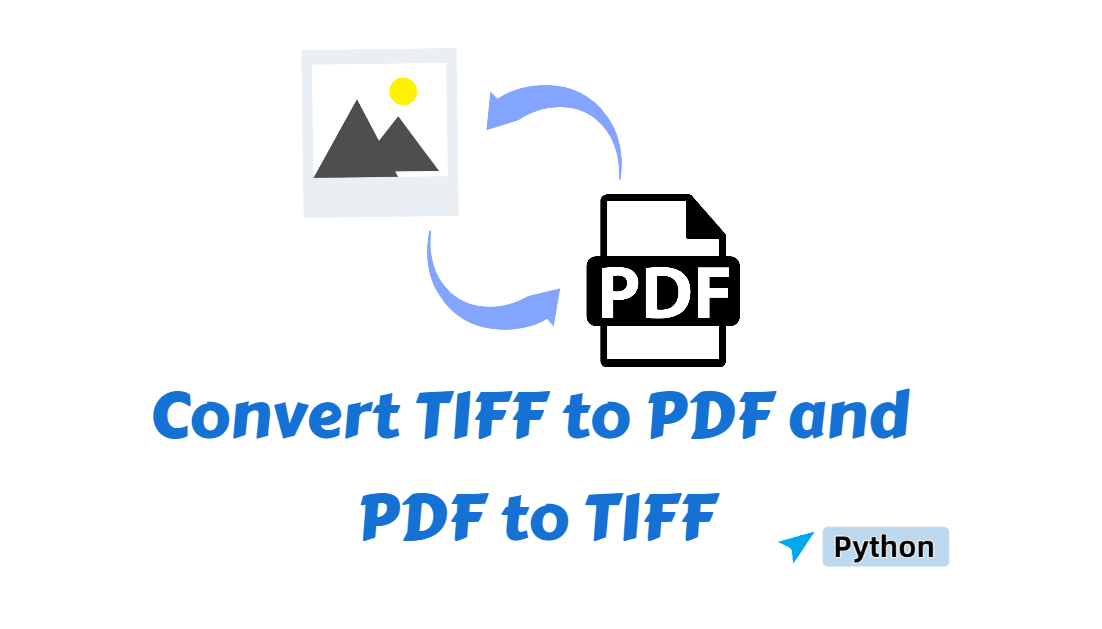
The TIFF (Tag Image File Format) format is commonly used in digital imaging and is popular among professionals for its high quality and ease of post-processing. However, due to its large file size, it is not the most convenient for sharing or printing. This is where converting it to PDF becomes an ideal solution. PDF files preserve the original image's quality while offering a more compact and easily shareable format, perfect for both transmission and printing. In this guide, we will explore how to convert TIFF to PDF and back in Python, enabling you to handle your documents easily.
Prepare for Tasks
To perform this feature in Python, we need to use Spire.PDF for Python, which is a popular Python PDF library. It supports many PDF-related operations, such as converting formats, adding or removing pages, extracting text or images, etc. This PDF component allows you to complete tasks efficiently without installing Adobe Acrobat.
You can install Spire.PDF for Python from PyPI using the pip command below:
pip install Spire.PDF
Adding another tool using the command: pip install pillow
TIF or TIFF: Learning File Formats
You might be wondering, what exactly is the TIFF format? And what about the TIF? They seem similar—so is there a difference between the two? In fact, according to Wikipedia, TIFF and TIF are just different ways of naming the same format. TIF is simply an abbreviation of TIFF. Both offer the same functionality and compatibility, so there’s no distinction between them in terms of usage or performance.
TIFF or TIF is an image file format for storing raster graphics images and is welcomed among graphic artists, the publishing industry, and photographers.
How to Convert TIFF to PDF in Python
Now that you're familiar with the TIFF format, let's get to the key point. When it comes to sharing processed images with colleagues or clients or printing them out on paper, the PDF format is often more suitable than TIFF. PDF files are easier to manage, ensure consistent formatting, and are generally more compact for the two purposes. In this section, we will guide you through how to convert TIFF files to PDFs in Python with detailed steps and a code example. Read on!
Detailed steps to make a TIFF file into a PDF:
Create an instance of the PdfDocument and set the page margins with the PdfDocument.PageSettings.SetMargins() property.
Load a TIFF image from the file path using the PIL.Image.open() method.
Iterate through frames in this image.
Access the current frame and create a copy of it.
Save the frame as a PNG image.
Use the PdfImage.From file() method to load these PNG images.
Get the width of images using the PdfImage.PhysicalDimension.Width property, and the height with the PdfImage.PhysicalDimension.Height property.
Add a new page to the PDF document created the first time according to the image’s width and height.
Draw the image on the new page to create a PDF page with content using the PdfPageBase.Canvas.DrawImage() method.
Save the resulting PDF document by calling the PdfDocument.SaveToFile() method and release resources.
The following is the code example of converting a TIFF file to PDF:
from spire.pdf.common import *
from spire.pdf import *
from PIL import Image
import io
# Create a PdfDocument object
doc = PdfDocument()
# Set the page margins to 0
doc.PageSettings.SetMargins(0.0)
# Load a TIFF image
tiff_image = Image.open("/tiff samples/sample-2.tiff")
# Iterate through the frames in it
for i in range(tiff_image.n_frames):
# Go to the current frame
tiff_image.seek(i)
# Extract the image of the current frame
frame_image = tiff_image.copy()
# Save the image to a PNG file
frame_image.save(f"/output/output_frame_{i}.png")
# Load the image file to PdfImage
image = PdfImage.FromFile(f"/output/output_frame_{i}.png")
# Get image width and height
width = image.PhysicalDimension.Width
height = image.PhysicalDimension.Height
# Add a page to the document
page = doc.Pages.Add(SizeF(width, height))
# Draw the image at (0, 0) of the page
page.Canvas.DrawImage(image, 0.0, 0.0, width, height)
# Save the document to a PDF file
doc.SaveToFile("/output/TiffToPdf.pdf",FileFormat.PDF)
# Dispose resources
doc.Dispose()
How to Convert PDF to TIFF with Python
PDF is indeed great for file sharing and saving, yet when you need a high-quality image to use as a citation or need to manipulate this document, the TIFF format will be the better choice. TIFF files retain excellent image quality and are widely compatible with many image editing tools. This part will introduce how to convert PDF to TIFF in a programming way with Python. After learning this part, you will find that saving PDF as TIFF is very easy.
The instructions to convert PDF to TIFF image:
Import modules.
Create an object of the PdfDocument and use the PdfDocument.LoadFromFile() method to read a PDF document from the disk.
Create a list to store PIL images.
Loop through each page in the PDF.
Use the PdfDocument.SaveAsImage() method to convert PDF pages into images.
Make the image byte array with the imageData.ToArray() method and open it using Image.Open() method.
Add images to the list.
Save images to the disk and release the resource.
Below is an example of converting a three-page PDF to TIFF:
from spire.pdf.common import *
from spire.pdf import *
from PIL import Image
from io import BytesIO
# Create a PdfDocument object
doc = PdfDocument()
# Load a PDF document
doc.LoadFromFile("/input/sample.pdf")
# Create an empty list to store PIL Images
images = []
# Iterate through all pages in the document
for i in range(doc.Pages.Count):
# Convert a specific page to an image stream
with doc.SaveAsImage(i) as imageData:
# Open the image stream as a PIL image
img = Image.open(BytesIO(imageData.ToArray()))
# Append the PIL image to list
images.append(img)
# Save the PIL Images as a multi-page TIFF file
images[0].save("/output/ToTIFF.tiff", save_all=True, append_images=images[1:])
# Dispose resources
doc.Dispose()
The Conclusion
This article mainly clarifies TIFF and TIF and illustrates how to convert TIFF to PDF and PDF to TIFF in Python. The two solutions come with specified steps and a code example for your reference. When you finish reading, you will find out that handling the conversion between TIFF and PDF is just a piece of cake!
Subscribe to my newsletter
Read articles from Casie Liu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
