Top Python Libraries for Data Science in 2024
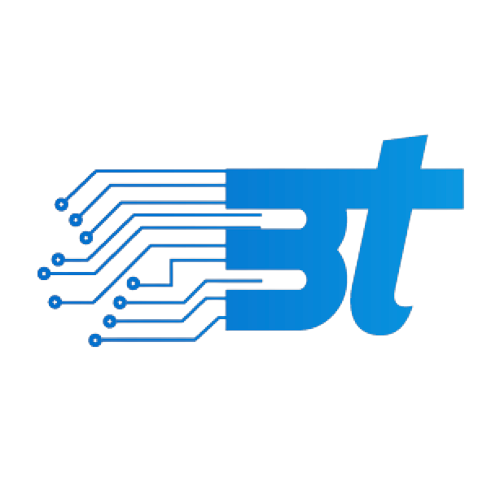
Table of contents
- 1. Pandas: Data Manipulation and Analysis
- 2. NumPy: Numerical Computing
- 3. Matplotlib: Data Visualization
- 4. Seaborn: Statistical Data Visualization
- 5. Scikit-learn: Machine Learning
- 6. TensorFlow: Deep Learning
- 7. PyTorch: Deep Learning
- 8. Statsmodels: Statistical Modeling
- 9. XGBoost: Extreme Gradient Boosting
- 10. Plotly: Interactive Visualization
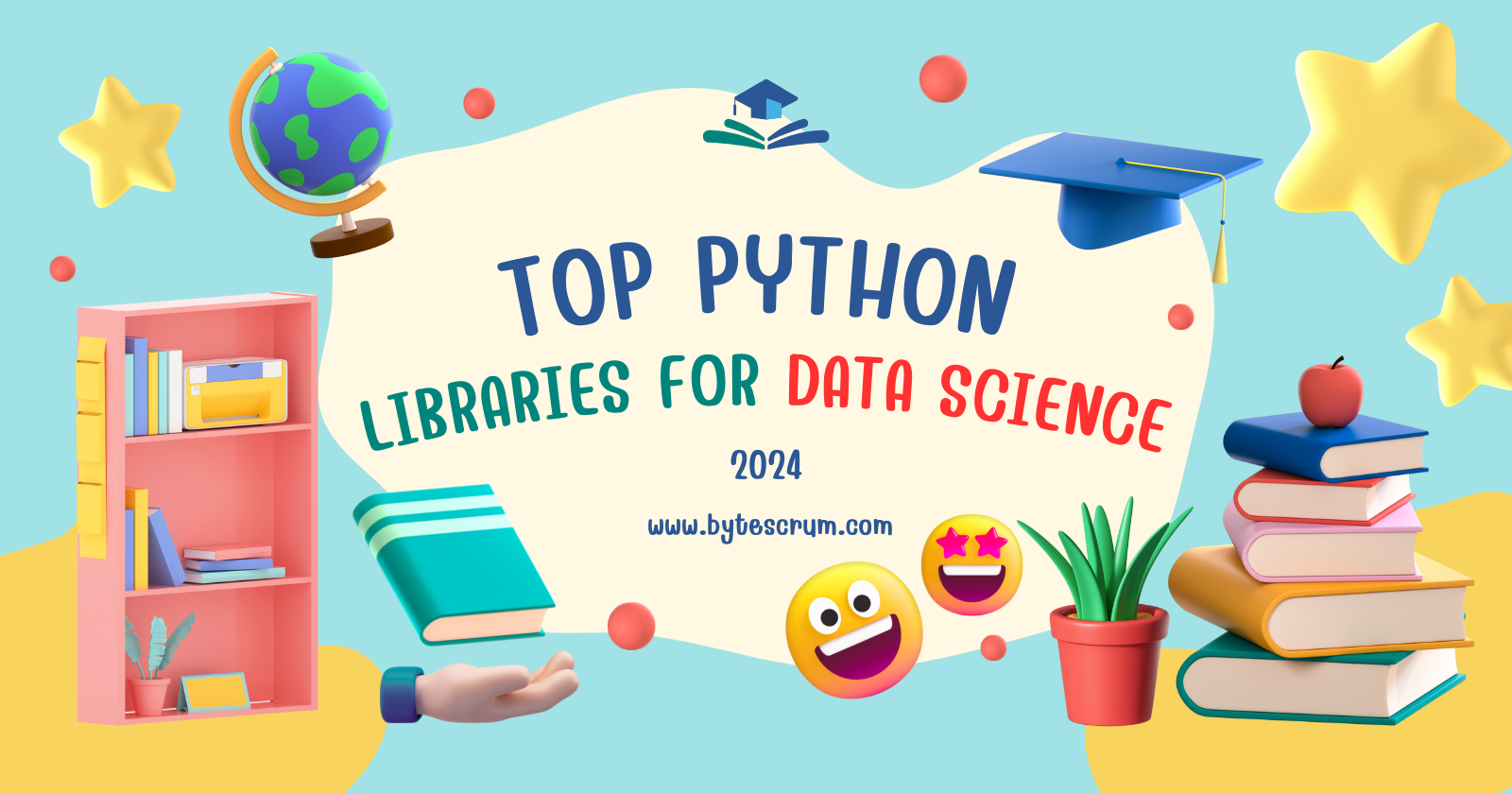
The rapid growth of data science has led to the emergence of a powerful ecosystem of Python libraries designed for every step of the data science workflow: data manipulation, visualization, machine learning, deep learning, and statistical analysis. In 2024, Python continues to dominate as the go-to language for data science due to its extensive and efficient libraries.
In this blog, we’ll explore the top Python libraries for data science in 2024, showcasing their features and providing code examples to help you get started.
1. Pandas: Data Manipulation and Analysis
Pandas is the most popular Python library for data manipulation. It provides data structures like DataFrame
and Series
to handle structured data. Pandas makes data cleaning, merging, reshaping, and analysis simple.
Key Features:
DataFrame: Two-dimensional, size-mutable, heterogeneous tabular data structure with labeled axes.
Data manipulation: Easy handling of missing data, merging datasets, and reshaping.
Time-series functionality: Efficient handling of time-indexed data.
Code Example:
import pandas as pd
# Creating a DataFrame
data = {'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [25, 30, 35, 40],
'Salary': [50000, 60000, 55000, 65000]}
df = pd.DataFrame(data)
# Basic data manipulation
df['Bonus'] = df['Salary'] * 0.10 # Adding a Bonus column
df_filtered = df[df['Age'] > 30] # Filtering rows where Age > 30
print(df_filtered)
2. NumPy: Numerical Computing
NumPy is the fundamental package for numerical computation in Python. It provides support for multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays.
Key Features:
n-dimensional arrays: Fast and efficient array operations.
Linear algebra: Tools for matrix and vector operations.
Random number generation: For simulations and probabilistic models.
Code Example:
import numpy as np
# Creating a NumPy array
arr = np.array([1, 2, 3, 4, 5])
# Basic operations
arr_squared = arr ** 2
matrix = np.random.rand(3, 3) # Generating a random 3x3 matrix
matrix_inverse = np.linalg.inv(matrix) # Calculating the inverse of a matrix
print(matrix_inverse)
3. Matplotlib: Data Visualization
Matplotlib is the most well-established plotting library in Python. It allows for the creation of static, animated, and interactive plots and is especially useful for basic visualizations and line graphs.
Key Features:
Flexible: Create any kind of chart (line, scatter, bar, etc.).
Customizable: Extensive customization for axis, labels, colors, and more.
Seamless with Pandas/NumPy: Works well with data from Pandas and NumPy.
Code Example:
import matplotlib.pyplot as plt
# Sample data
x = [0, 1, 2, 3, 4, 5]
y = [0, 1, 4, 9, 16, 25]
# Plotting a line graph
plt.plot(x, y, label='y = x^2')
plt.xlabel('x')
plt.ylabel('y')
plt.title('Quadratic Graph')
plt.legend()
plt.show()
4. Seaborn: Statistical Data Visualization
Seaborn is built on top of Matplotlib and provides a high-level interface for drawing attractive statistical graphics. It integrates closely with Pandas data structures and supports informative visualizations like heatmaps and pair plots.
Key Features:
Built-in themes: Enhances Matplotlib’s default aesthetics.
Statistical plots: Easily create visualizations like boxplots, violin plots, and pair plots.
Integration: Works seamlessly with DataFrames.
Code Example:
import seaborn as sns
import pandas as pd
# Sample dataset
df = pd.DataFrame({'Category': ['A', 'B', 'C', 'D'],
'Values': [4, 7, 1, 8]})
# Bar plot using Seaborn
sns.barplot(x='Category', y='Values', data=df)
plt.title('Category Value Distribution')
plt.show()
5. Scikit-learn: Machine Learning
Scikit-learn is the go-to library for implementing basic to intermediate machine learning models. It provides a vast collection of algorithms for classification, regression, clustering, and more.
Key Features:
Wide range of algorithms: Includes decision trees, support vector machines, k-means clustering, etc.
Model selection: Tools for cross-validation, hyperparameter tuning, and model evaluation.
Preprocessing: Handles scaling, normalization, and encoding.
Code Example:
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.datasets import load_boston
from sklearn.metrics import mean_squared_error
# Load the Boston housing dataset
data = load_boston()
X = data['data']
y = data['target']
# Split the data
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train a linear regression model
model = LinearRegression()
model.fit(X_train, y_train)
# Predictions and evaluation
predictions = model.predict(X_test)
mse = mean_squared_error(y_test, predictions)
print(f'Mean Squared Error: {mse}')
6. TensorFlow: Deep Learning
TensorFlow is a popular library for building and training deep learning models. Developed by Google, it supports both CPU and GPU processing, making it highly scalable.
Key Features:
Flexible: Build machine learning models using neural networks.
Optimized for performance: Leverages GPU acceleration.
Keras integration: Simplified high-level API for neural network models.
Code Example:
import tensorflow as tf
# Creating a basic neural network
model = tf.keras.models.Sequential([
tf.keras.layers.Dense(64, activation='relu', input_shape=(100,)),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile and train the model
model.compile(optimizer='adam', loss='categorical_crossentropy')
# Assume X_train and y_train are prepared
# model.fit(X_train, y_train, epochs=10)
7. PyTorch: Deep Learning
PyTorch has grown in popularity due to its dynamic computation graph, which is intuitive and easy to debug. Used by Facebook’s AI research group, it has become a preferred choice for researchers and professionals alike.
Key Features:
Dynamic computation graph: Easier to experiment with complex architectures.
Autograd: Built-in automatic differentiation for gradients.
Large ecosystem: Extensive tools for deploying models and handling data.
Code Example:
import torch
import torch.nn as nn
# Define a simple feedforward neural network
class SimpleNN(nn.Module):
def __init__(self):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(10, 50)
self.fc2 = nn.Linear(50, 1)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# Instantiate and forward pass
model = SimpleNN()
input_data = torch.randn(5, 10) # Batch of 5 samples, each with 10 features
output = model(input_data)
print(output)
8. Statsmodels: Statistical Modeling
Statsmodels provides tools for statistical modeling, including linear models, time-series analysis, and hypothesis testing. It is perfect for those who need more advanced statistical analysis beyond Scikit-learn’s capabilities.
Key Features:
Descriptive statistics: Summarize datasets.
Estimation: Build linear and generalized linear models.
Time-series analysis: Includes ARIMA, SARIMAX, and Holt-Winters methods.
Code Example:
import statsmodels.api as sm
import numpy as np
# Sample data
X = np.random.rand(100, 3)
y = np.random.rand(100)
# Adding constant for intercept
X = sm.add_constant(X)
# OLS regression
model = sm.OLS(y, X).fit()
# Summary of the regression results
print(model.summary())
9. XGBoost: Extreme Gradient Boosting
XGBoost is an optimized machine learning library for gradient boosting, widely used in competitions and practical applications for its performance and accuracy.
Key Features:
Efficient and scalable: Outperforms other machine learning algorithms in terms of speed and accuracy.
Handles missing data: Robust to sparse datasets.
Feature importance: Helps with feature selection.
Code Example:
import xgboost as xgb
from sklearn.model_selection import train_test_split
from sklearn.datasets import load_boston
# Load dataset
data = load_boston()
X, y = data['data'], data['target']
# Train-test split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train an XGBoost regressor
model = xgb.XGBRegressor()
model.fit(X_train, y_train)
# Predictions
predictions = model.predict(X_test)
print(predictions[:5])
10. Plotly: Interactive Visualization
Plotly is a versatile library for creating interactive, web-based visualizations that are especially useful in exploratory data analysis. It integrates seamlessly with Pandas and provides dashboards.
Key Features:
Interactive visualizations: Create zoomable, responsive plots.
Wide variety of charts: From line charts to 3D plots and geographic maps.
Web-ready: Easily embed plots in web applications.
Code Example:
import plotly.express as px
# Sample data
df = px.data.iris()
# Create a scatter plot
fig = px.scatter(df, x='sepal_width', y='sepal_length', color='species', title='Iris Sepal Dimensions')
fig.show()
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
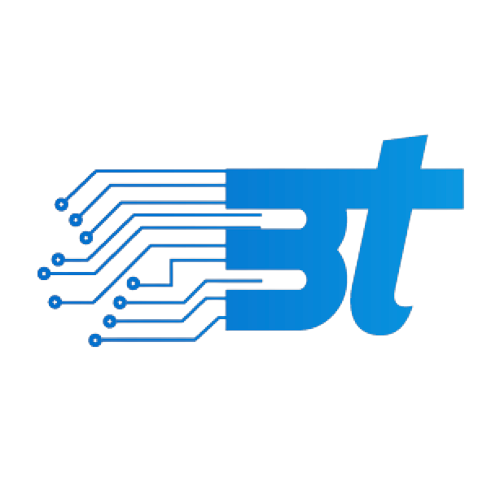
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.