Supervised vs. Unsupervised Learning: When to Use Each?
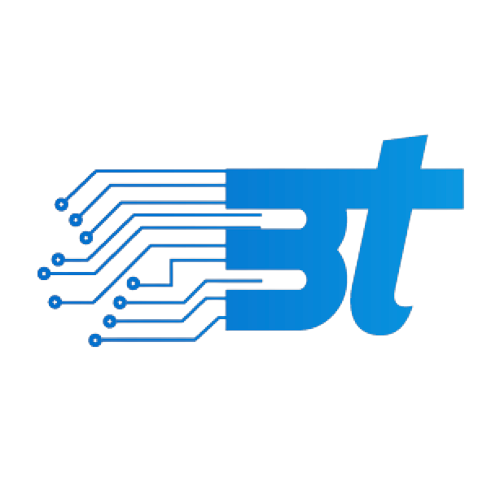
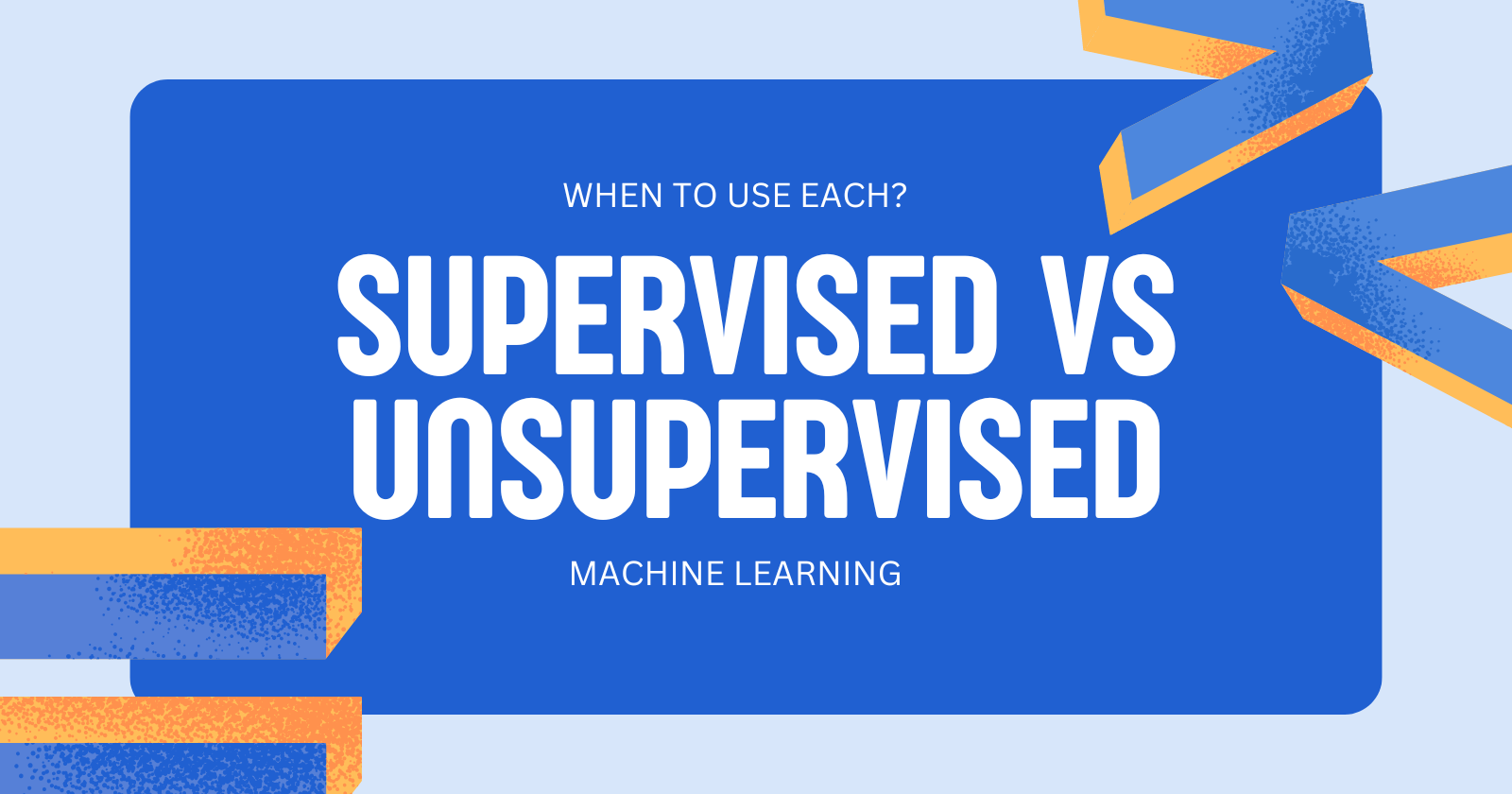
Machine learning is a rapidly growing field, and understanding the difference between supervised and unsupervised learning is crucial for anyone looking to leverage machine learning techniques effectively. These two types of learning algorithms serve distinct purposes, and knowing when to use each can greatly improve your data science or AI project.
In this blog post, we’ll dive deep into both supervised and unsupervised learning, provide easy-to-understand examples, and discuss when to use each. By the end, you’ll have a clear understanding of these two fundamental machine learning approaches.
What is Supervised Learning?
Supervised learning is a type of machine learning where the model is trained using labeled data. Labeled data means that each example in the dataset has both an input (features) and an output (label). The model learns by looking at these input-output pairs and makes predictions on new data based on what it has learned.
For example, imagine you're teaching a machine to recognize whether an email is spam or not. You would train it with emails that have already been labeled as either "spam" or "not spam." The machine looks at the words, links, and other features in the emails and learns to predict whether a new email is spam based on similar patterns.
Key Features of Supervised Learning:
Labeled Data: Requires input-output pairs.
Clear Objective: The goal is to predict a known outcome.
Performance Metrics: Accuracy, precision, recall, etc., are used to measure model performance.
Types of Supervised Learning:
Classification: Predicts discrete categories (e.g., "spam" vs. "not spam").
Regression: Predicts continuous values (e.g., predicting house prices).
Example of Supervised Learning: Predicting House Prices
Suppose you have a dataset with features like the size of the house, number of bedrooms, and location, along with the prices of these houses. In this case, you can use supervised learning to predict the price of a new house based on its features.
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.datasets import load_boston
# Load dataset
data = load_boston()
X, y = data['data'], data['target']
# Train-test split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train the model
model = LinearRegression()
model.fit(X_train, y_train)
# Make predictions
predictions = model.predict(X_test)
print(predictions[:5])
In this example, the Linear Regression model predicts house prices based on features like size and location.
When to Use Supervised Learning?
You have labeled data: If your dataset includes inputs with corresponding known outputs, supervised learning is the best approach.
Predictive tasks: When your goal is to make predictions, such as determining whether a customer will churn, classifying images, or forecasting sales.
Need for accuracy metrics: If you want to measure the performance of your model with specific metrics like accuracy, F1 score, or RMSE, supervised learning provides clear benchmarks for evaluation.
What is Unsupervised Learning?
Unsupervised learning is a type of machine learning that works with unlabeled data. Here, the model doesn’t have explicit labels or outcomes to predict. Instead, it seeks to find hidden patterns, groupings, or structures within the data.
For example, consider a retail company that wants to understand its customer base better. The company may not know exactly what segments exist, but by analyzing customer behavior (such as purchase history and browsing patterns), it can group customers into clusters with similar characteristics.
Key Features of Unsupervised Learning:
No Labeled Data: Works with datasets that do not have predefined labels.
Pattern Discovery: The main goal is to identify hidden patterns or groupings.
Exploratory in Nature: The outcome is not predefined.
Types of Unsupervised Learning:
Clustering: Grouping data points into clusters based on similarity (e.g., customer segmentation).
Dimensionality Reduction: Reducing the number of variables while preserving the essential information (e.g., Principal Component Analysis or PCA).
Example of Unsupervised Learning: Customer Segmentation
Suppose a retail company wants to divide its customers into distinct groups based on their purchasing habits. Since there are no predefined labels (e.g., no pre-existing customer segments), unsupervised learning techniques like clustering can be used to group customers based on their behavior.
from sklearn.cluster import KMeans
import matplotlib.pyplot as plt
from sklearn.datasets import make_blobs
# Generate sample data
X, _ = make_blobs(n_samples=300, centers=4, cluster_std=0.60, random_state=42)
# Apply K-Means clustering
kmeans = KMeans(n_clusters=4)
kmeans.fit(X)
# Predict cluster labels
y_kmeans = kmeans.predict(X)
# Plot the clusters
plt.scatter(X[:, 0], X[:, 1], c=y_kmeans, s=50, cmap='viridis')
plt.scatter(kmeans.cluster_centers_[:, 0], kmeans.cluster_centers_[:, 1], s=200, c='red', label='Centroids')
plt.legend()
plt.show()
In this example, K-Means clustering identifies four distinct groups of customers based on their data points, which might correspond to different customer segments.
When to Use Unsupervised Learning?
You don’t have labeled data: If your dataset doesn’t have clear labels or outcomes, unsupervised learning is ideal.
Exploring hidden patterns: When the goal is to discover unknown structures or groupings, such as clustering customers based on behavior or finding anomalies in network traffic.
Dimensionality reduction: When working with high-dimensional data, unsupervised learning can help reduce complexity while retaining important information.
Supervised vs. Unsupervised Learning: Key Differences
Aspect | Supervised Learning | Unsupervised Learning |
Data | Requires labeled data | Uses unlabeled data |
Objective | Predict outcomes based on input data | Discover hidden patterns or groupings |
Examples | Spam detection, house price prediction | Customer segmentation, anomaly detection |
Types of Tasks | Regression, Classification | Clustering, Dimensionality Reduction |
Evaluation Metrics | Accuracy, precision, recall | Not always clear, exploratory in nature |
Complexity | Often more complex due to labeled data | More exploratory, requires less data preparation |
Real-world Use Cases: Supervised vs. Unsupervised Learning
Supervised Learning Examples:
Medical Diagnosis: Classifying diseases based on patient symptoms and medical tests.
- The model learns from historical data labeled with known diagnoses to predict diseases for new patients.
Email Spam Detection: Identifying spam emails using labeled datasets of emails marked as spam or not spam.
- Supervised learning models can classify incoming emails based on past examples.
House Price Prediction: Predicting real estate prices based on features like location, size, and amenities.
- Regression models use labeled data with house prices to predict future sales prices.
Unsupervised Learning Examples:
Customer Segmentation: Grouping customers into segments based on purchasing behavior.
- Retailers can use unsupervised learning to create targeted marketing campaigns by understanding the behaviors of different customer groups.
Anomaly Detection in Networks: Detecting unusual patterns in network traffic that could indicate cyber-attacks.
- Unsupervised learning models find outliers or anomalies that deviate from normal traffic patterns.
Recommendation Systems: Grouping similar products or users to provide personalized recommendations.
- Unsupervised learning is often used in collaborative filtering to suggest products based on clustering of similar items or users.
Challenges and Considerations
Challenges in Supervised Learning:
Data Labeling: Labeled data is often expensive and time-consuming to collect.
Overfitting: If the model learns the noise in the data too well, it can perform poorly on new, unseen data.
Challenges in Unsupervised Learning:
Evaluation: Without labeled data, it’s hard to know if the model’s results are correct or useful.
Interpretability: The patterns or groupings found may not always be intuitive or easy to interpret.
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
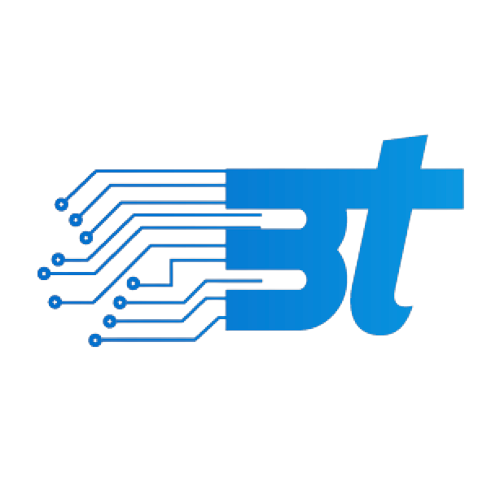
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.