React project with TypeScript, Tailwind CSS, Sass, Prettier, ESLint, and Firebase for authentication and Firestore:

Table of contents
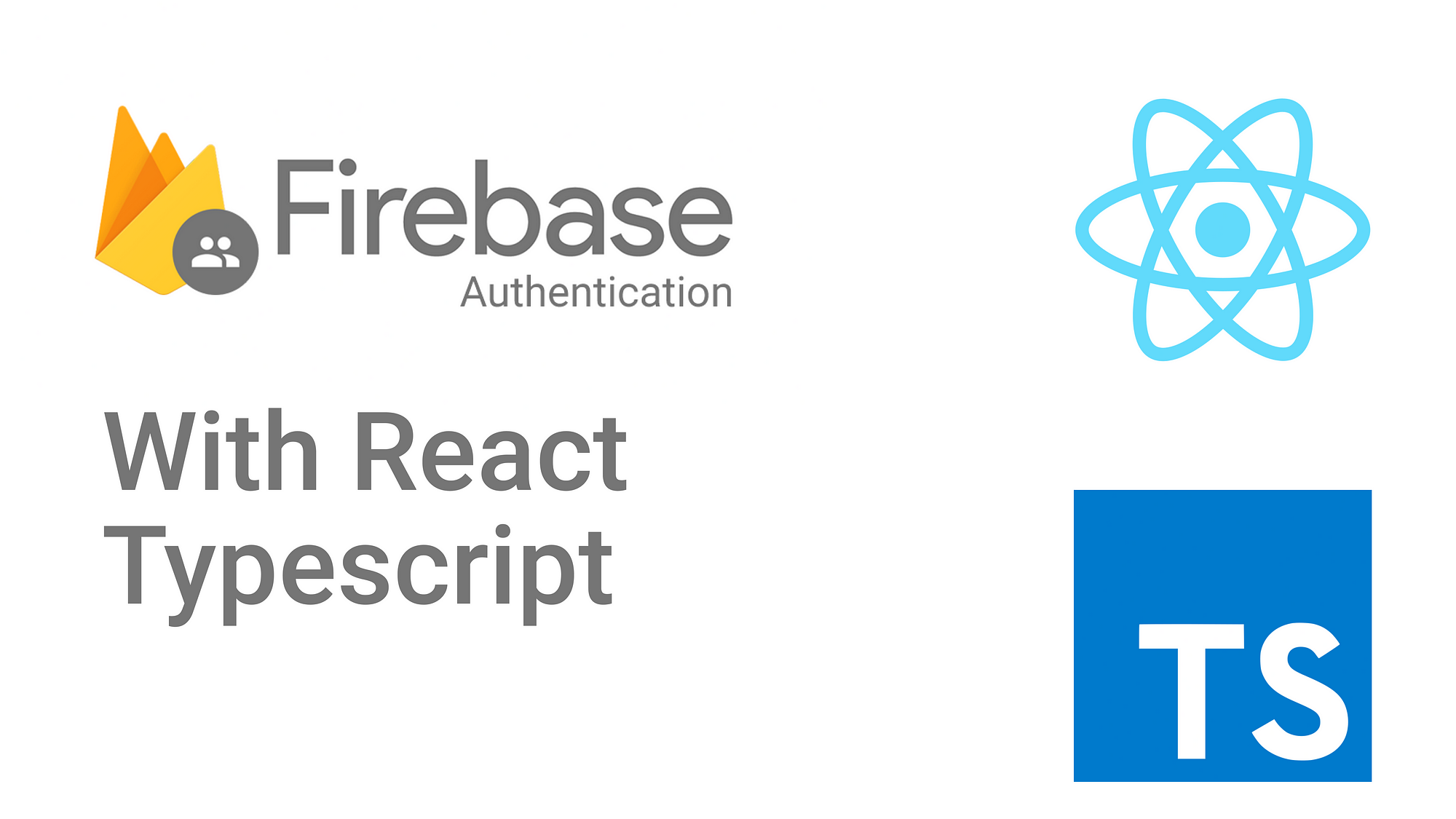
Step 1: Create a New React + TypeScript Project
First, create a new React project with TypeScript using create-react-app
:
npx create-react-app my-app --template typescript
cd my-app
Step 2: Install Tailwind CSS
Install Tailwind CSS:
npm install -D tailwindcss postcss autoprefixer
npx tailwindcss init -p
Configure tailwind.config.js
by adding the following:
/** @type {import('tailwindcss').Config} */
module.exports = {
content: [
"./src/**/*.{js,jsx,ts,tsx}",
],
theme: {
extend: {},
},
plugins: [],
}
In the src/index.css
file, include the Tailwind base, components, and utilities styles:
@tailwind base;
@tailwind components;
@tailwind utilities;
Step 3: Install Sass
Add Sass support:
npm install sass
Step 4: Install and Configure Prettier
Install Prettier for code formatting:
npm install --save-dev prettier
Create a .prettierrc
file in the root of your project:
{
"singleQuote": true,
"semi": false,
"trailingComma": "es5",
"tabWidth": 2
}
Add a .prettierignore
file to ignore specific files:
build
node_modules
Step 5: Install and Configure ESLint
Install ESLint and configure it with TypeScript support:
npm install --save-dev eslint @typescript-eslint/parser @typescript-eslint/eslint-plugin
Generate the ESLint configuration file:
npx eslint --init
Follow the prompts to set up ESLint for a TypeScript project. You can then update .eslintrc.json
to look like this:
{
"env": {
"browser": true,
"es2021": true
},
"extends": [
"eslint:recommended",
"plugin:react/recommended",
"plugin:@typescript-eslint/recommended",
"prettier"
],
"parser": "@typescript-eslint/parser",
"parserOptions": {
"ecmaFeatures": {
"jsx": true
},
"ecmaVersion": "latest",
"sourceType": "module"
},
"plugins": ["react", "@typescript-eslint"],
"rules": {}
}
Step 6: Set Up Firebase
Go to Firebase Console and create a new project.
Install Firebase in your project:
npm install firebase
Create a Firebase config file src/firebase.ts
:
import { initializeApp } from 'firebase/app';
import { getAuth } from 'firebase/auth';
import { getFirestore } from 'firebase/firestore';
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_AUTH_DOMAIN",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_STORAGE_BUCKET",
messagingSenderId: "YOUR_MESSAGING_SENDER_ID",
appId: "YOUR_APP_ID",
};
const app = initializeApp(firebaseConfig);
export const auth = getAuth(app);
export const db = getFirestore(app);
Step 7: Implement Firebase Authentication
For login and logout, you can use Firebase's Authentication service. Here is an example of how to implement it using React and TypeScript.
src/components/Login.tsx
(Login Component):
import { useState } from 'react'
import { signInWithEmailAndPassword } from 'firebase/auth'
import { auth } from '../firebase'
const Login = () => {
const [email, setEmail] = useState('')
const [password, setPassword] = useState('')
const [error, setError] = useState('')
const handleLogin = async () => {
try {
await signInWithEmailAndPassword(auth, email, password)
} catch (error: any) {
setError(error.message)
}
}
return (
<div>
<input
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
placeholder="Email"
/>
<input
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
placeholder="Password"
/>
<button onClick={handleLogin}>Login</button>
{error && <p>{error}</p>}
</div>
)
}
export default Login
src/components/Logout.tsx
(Logout Component):
import { signOut } from 'firebase/auth'
import { auth } from '../firebase'
const Logout = () => {
const handleLogout = async () => {
await signOut(auth)
}
return <button onClick={handleLogout}>Logout</button>
}
export default Logout
Step 8: Firestore Database Example
For Firestore integration, here's how you can read and write data:
Writing to Firestore:
import { collection, addDoc } from 'firebase/firestore'
import { db } from './firebase'
const addDocument = async () => {
try {
const docRef = await addDoc(collection(db, 'users'), {
name: 'John Doe',
email: 'johndoe@example.com',
})
console.log('Document written with ID: ', docRef.id)
} catch (e) {
console.error('Error adding document: ', e)
}
}
Reading from Firestore:
import { collection, getDocs } from 'firebase/firestore'
import { db } from './firebase'
const fetchUsers = async () => {
const querySnapshot = await getDocs(collection(db, 'users'))
querySnapshot.forEach((doc) => {
console.log(`${doc.id} => ${doc.data()}`)
})
}
Step 9: Run the Project
Finally, run the project to see everything in action:
npm start
This setup provides you with a robust environment for building React apps using TypeScript, Tailwind CSS, Sass, Prettier, and ESLint, with Firebase for authentication and Firestore as the database.
Subscribe to my newsletter
Read articles from Gauss Coder directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
