Set up Redux in a React TypeScript Project

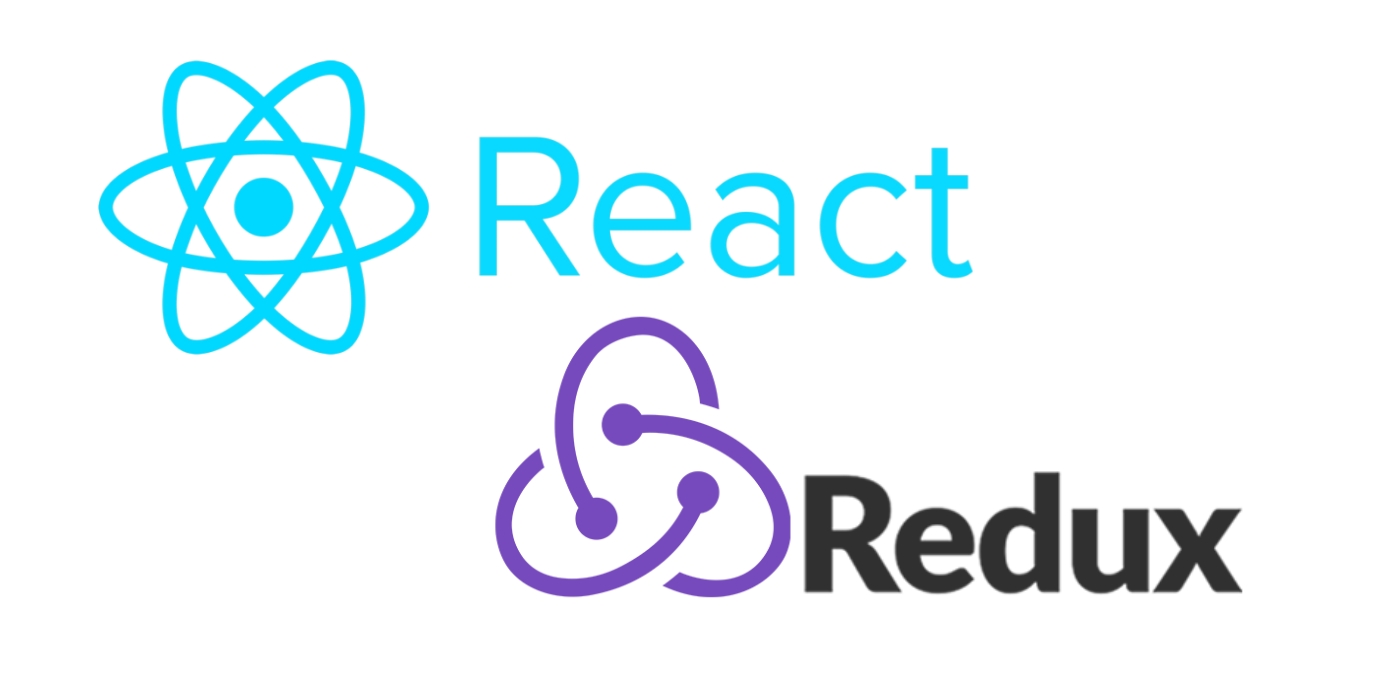
To set up Redux in a React TypeScript project, follow these steps:
Step 1: Install Dependencies
First, install Redux, React-Redux, and Redux Toolkit (recommended for modern Redux setups), along with TypeScript types for Redux:
npm install @reduxjs/toolkit react-redux
npm install --save-dev @types/react-redux
Step 2: Create the Redux Store
Create a
store
directoryCreate a directory for your Redux store and slice files. For example:
src/ └── store/ ├── index.ts └── counterSlice.ts
Set Up the Store
Create a file
src/store/index.ts
to configure your Redux store:// src/store/index.ts import { configureStore } from '@reduxjs/toolkit'; import counterReducer from './counterSlice'; export const store = configureStore({ reducer: { counter: counterReducer, }, }); // Optional: Define RootState and AppDispatch types for use in components export type RootState = ReturnType<typeof store.getState>; export type AppDispatch = typeof store.dispatch;
Create a Slice
Create a slice to manage your state. For example, a counter slice:
// src/store/counterSlice.ts
import { createSlice, PayloadAction } from '@reduxjs/toolkit';
interface CounterState {
value: number;
}
const initialState: CounterState = {
value: 0,
};
const counterSlice = createSlice({
name: 'counter',
initialState,
reducers: {
increment: (state) => {
state.value += 1;
},
decrement: (state) => {
state.value -= 1;
},
incrementByAmount: (state, action: PayloadAction<number>) => {
state.value += action.payload;
},
},
});
export const { increment, decrement, incrementByAmount } = counterSlice.actions;
export default counterSlice.reducer;
Step 3: Provide the Redux Store to Your Application
Wrap your application with the Provider
component from react-redux
to make the Redux store available to your components.
// src/index.tsx
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import { store } from './store';
import App from './App';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
Step 4: Connect Components to Redux
Use useSelector
and useDispatch
hooks from react-redux
to interact with the Redux store in your components.
Access State
// src/components/Counter.tsx import React from 'react'; import { useSelector, useDispatch } from 'react-redux'; import { RootState, AppDispatch } from '../store'; import { increment, decrement, incrementByAmount } from '../store/counterSlice'; const Counter: React.FC = () => { const dispatch: AppDispatch = useDispatch(); const count = useSelector((state: RootState) => state.counter.value); return ( <div> <h1>Counter: {count}</h1> <button onClick={() => dispatch(increment())}>Increment</button> <button onClick={() => dispatch(decrement())}>Decrement</button> <button onClick={() => dispatch(incrementByAmount(5))}>Increment by 5</button> </div> ); }; export default Counter;
Additional Tips
Type Safety: Using TypeScript with Redux enhances type safety, especially with actions and state. Ensure that all reducers, actions, and selectors are typed correctly.
Redux Toolkit: Redux Toolkit simplifies the process of writing Redux logic and reduces boilerplate. It provides utilities for creating slices, configuring the store, and more.
Async Actions: For handling async logic, you can use
createAsyncThunk
from Redux Toolkit. This is useful for making API calls and managing loading and error states.
By following these steps, you'll have a Redux setup integrated with TypeScript in your React project, allowing you to manage global state effectively.
Subscribe to my newsletter
Read articles from Gauss Coder directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
