How to Boost Your Machine Learning Model with Feature Engineering
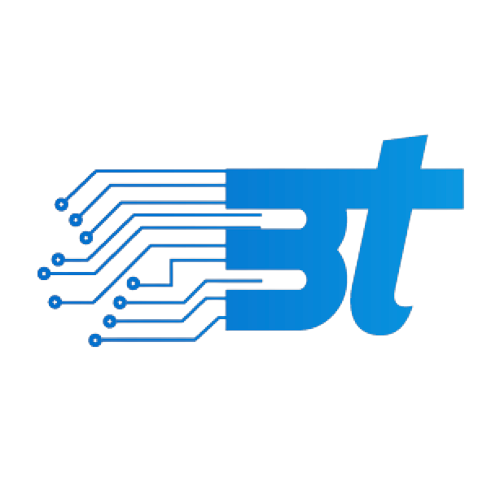
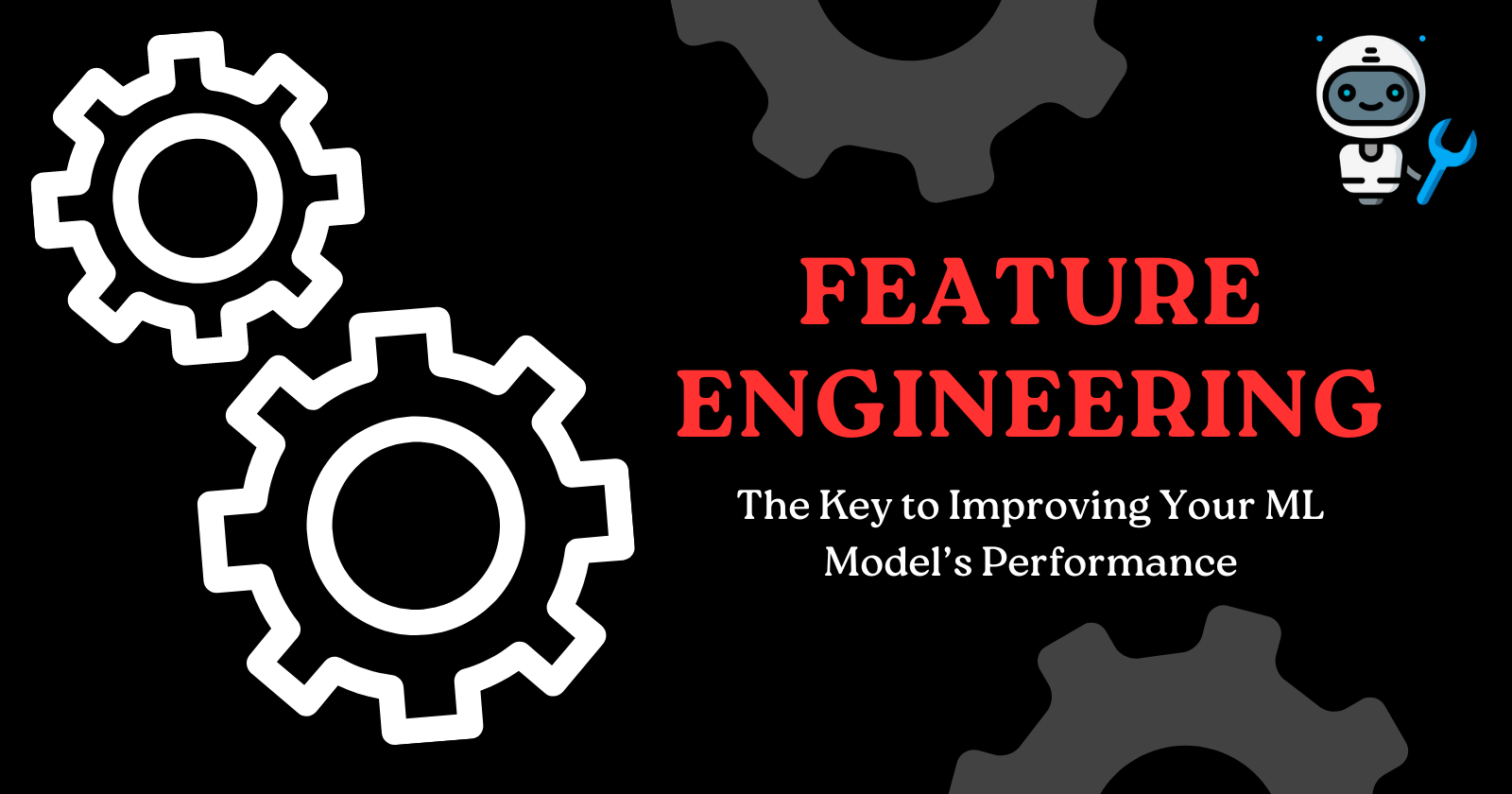
In machine learning, feature engineering is often considered the secret sauce that can dramatically improve model performance. While algorithms and models receive a lot of attention, the quality of features plays an equally—if not more—important role in determining how well a model performs. Feature engineering is the process of selecting, modifying, and creating new input features from raw data to make machine learning models more effective.
This blog will dive deep into what feature engineering is, why it’s essential, and how it can elevate your machine learning projects. We'll also cover different types of feature engineering techniques and illustrate them with practical examples, ensuring that you can apply them to real-world problems.
What is Feature Engineering?
Feature engineering refers to the process of transforming raw data into features that better represent the underlying patterns to the machine learning algorithm, thus enhancing model accuracy and performance. Features are the input variables (or columns) used by models to make predictions. Feature engineering involves identifying and extracting the most relevant features or creating new ones that can help the model understand the data more effectively.
For example, suppose you're working with a dataset that includes a timestamp for each transaction. Rather than feeding the raw timestamp to the model, you could extract meaningful features like the hour of the day, day of the week, or month, which could give the model better context.
Why is Feature Engineering Important?
Feature engineering is one of the most crucial steps in the machine learning process for several reasons:
Improves Model Accuracy: Carefully engineered features can bring out patterns and trends in the data that are not easily captured by raw features, leading to improved model accuracy.
Reduces Overfitting: By adding features that better represent the data's patterns, models can generalize better to unseen data, thus reducing the risk of overfitting.
Simplifies Model Complexity: Good features can sometimes reduce the need for more complex models. Simple algorithms with well-engineered features can outperform complex algorithms with poorly chosen features.
Enhances Model Interpretability: Well-engineered features are often easier to interpret, making it more straightforward to understand how the model makes decisions.
Increases Model Efficiency: When the most relevant features are used, models can become more computationally efficient, reducing training and inference times.
In essence, feature engineering enables you to bridge the gap between raw data and machine learning algorithms, giving your models the best chance of success.
Types of Feature Engineering
1. Feature Selection
Feature selection involves selecting a subset of relevant features from the dataset and discarding irrelevant or redundant features. Irrelevant features can confuse the model and make it harder to find meaningful patterns, while redundant features can increase the risk of overfitting.
Techniques for Feature Selection:
Univariate Selection: Selects features based on their statistical significance, such as ANOVA or chi-square tests.
Recursive Feature Elimination (RFE): This iterative process eliminates the least important features until a desired number of features is reached.
Principal Component Analysis (PCA): Reduces the dimensionality of data by transforming it into a set of linearly uncorrelated features known as principal components.
from sklearn.feature_selection import RFE
from sklearn.linear_model import LogisticRegression
# Example of Recursive Feature Elimination
model = LogisticRegression()
rfe = RFE(model, n_features_to_select=5)
fit = rfe.fit(X, y)
print(f"Selected Features: {fit.support_}")
2. Feature Transformation
Feature transformation involves modifying the features to improve the accuracy of machine learning models. The idea is to transform the features into a new space or scale where the relationships between variables are more linear and easier for the model to capture.
Common Transformation Techniques:
- Scaling: Models like Support Vector Machines (SVMs) and K-Nearest Neighbors (KNN) are sensitive to feature scaling. Standardization and normalization are often used to transform features to a common scale.
from sklearn.preprocessing import StandardScaler
# Scaling features
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
- Log Transformation: For skewed distributions, a log transformation can be applied to make the data more normal.
import numpy as np
# Apply log transformation to a feature
X['Log_Feature'] = np.log(X['Feature'] + 1)
- Binning: This technique converts continuous data into categorical data by grouping them into bins. For instance, age can be divided into ranges like "Young," "Middle-aged," and "Senior."
# Binning a continuous variable
X['Age_Binned'] = pd.cut(X['Age'], bins=[0, 18, 35, 60, 100], labels=['Child', 'Young Adult', 'Adult', 'Senior'])
3. Feature Creation
Feature creation involves generating new features from existing ones, often by using domain knowledge. This is particularly valuable when the raw data doesn't explicitly provide the relationships the model needs to learn from.
Examples of Feature Creation:
- Polynomial Features: Generate higher-order interaction terms between features to capture non-linear relationships.
from sklearn.preprocessing import PolynomialFeatures
# Creating polynomial features
poly = PolynomialFeatures(degree=2)
X_poly = poly.fit_transform(X)
- Date and Time Features: Extract features like the day of the week, month, or time of day from timestamp data, which can help models capture temporal trends.
# Extracting day, month, year from a timestamp
X['day'] = X['timestamp'].dt.day
X['month'] = X['timestamp'].dt.month
X['year'] = X['timestamp'].dt.year
- Interaction Terms: Create new features by combining two or more features that could capture relationships between them.
# Creating an interaction feature
X['interaction_feature'] = X['Feature1'] * X['Feature2']
4. Encoding Categorical Variables
Machine learning models typically cannot work with categorical variables directly, so it's necessary to encode them as numerical values.
Techniques for Encoding:
- One-Hot Encoding: Converts categorical variables into binary vectors, where each unique category gets its own column.
from sklearn.preprocessing import OneHotEncoder
# One-hot encoding
encoder = OneHotEncoder(sparse=False)
X_encoded = encoder.fit_transform(X[['Category']])
- Label Encoding: Converts each category into a unique integer. This is more useful when the categories have an inherent order, like "low," "medium," and "high."
from sklearn.preprocessing import LabelEncoder
# Label encoding
encoder = LabelEncoder()
X['Category_Encoded'] = encoder.fit_transform(X['Category'])
Steps for Effective Feature Engineering
1. Understand the Problem and Data
Before diving into feature engineering, thoroughly understand the problem you're trying to solve. Domain expertise can be critical in identifying which features are likely to be important. Exploratory Data Analysis (EDA) helps identify trends, outliers, and relationships in the data that may not be immediately obvious.
2. Clean the Data
Feature engineering starts with clean data. Handling missing values, outliers, and inconsistent data types is essential. Techniques like imputation for missing values and outlier removal help make the data suitable for modeling.
# Handling missing values
X.fillna(X.median(), inplace=True)
3. Choose the Right Features
Select features that contribute most to the problem at hand. You can use domain knowledge or automated feature selection techniques to identify important features.
4. Transform and Create New Features
Use feature transformation techniques like scaling, normalization, or binning, and create new features based on domain knowledge or patterns you see in the data.
5. Evaluate and Iterate
Always evaluate the model’s performance after feature engineering to ensure the new features have improved accuracy. Use cross-validation to ensure the model generalizes well to unseen data. Feature engineering is often an iterative process; the first attempt may not yield the best results.
Case Study: Predicting Housing Prices
Let’s put feature engineering into practice by building a model to predict house prices using the famous Boston Housing dataset. We will show how feature engineering improves model performance.
Step 1: Understanding the Data
The Boston Housing dataset contains features like the number of rooms, the age of the house, and the distance to employment centers. To improve the model, we can create new features, such as:
Rooms per household: A higher number of rooms per household may increase the house price.
Age of house: The older the house, the less likely it will have a high price.
# Creating new features
X['rooms_per_household'] = X['total_rooms'] / X['households']
X['age_house'] = 2024 - X['year_built']
Step 2: Feature Transformation
Some features, like the number of rooms and the distance to employment centers, may be skewed. We apply log transformation to these features to normalize them.
# Log transformation of skewed features
X['total_rooms_log'] = np.log(X['total_rooms'] + 1)
Step 3: One-Hot Encoding for Categorical Variables
The dataset contains categorical variables like "neighborhood." We can apply one-hot encoding to these categorical variables.
# One-hot encoding of the neighborhood feature
X = pd.get_dummies(X, columns=['neighborhood'], drop_first=True)
Step 4: Model Evaluation
After feature engineering, we train a regression model and evaluate its performance using Mean Squared Error (MSE).
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LinearRegression
from sklearn.metrics import mean_squared_error
# Train-test split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train model
model = LinearRegression()
model.fit(X_train, y_train)
# Evaluate model
y_pred = model.predict(X_test)
print(f"Mean Squared Error: {mean_squared_error(y_test, y_pred)}")
With carefully engineered features, the model's accuracy improves, highlighting the importance of feature engineering in machine learning projects.
Conclusion
So, whether you’re working on a regression task, a classification problem, or a complex deep learning project, remember that feature engineering is key to unlocking the full potential of your machine learning models!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
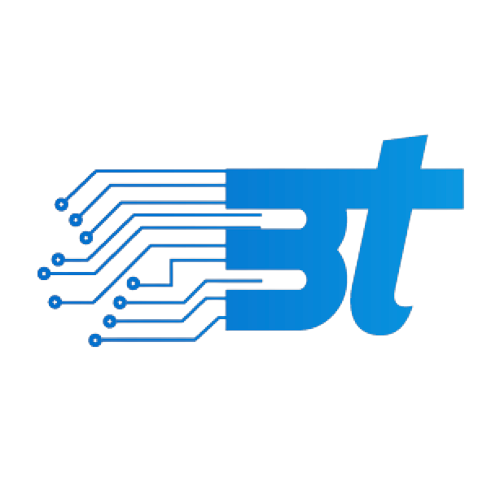
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.