Common Misconceptions About React Server Components

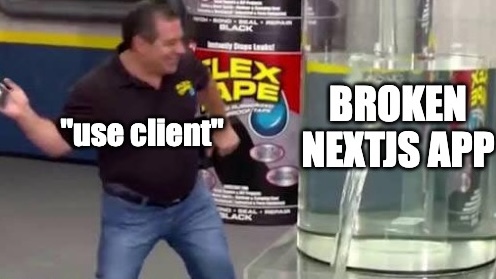
React Server Components are a new way of writing React components that speed up server-side & full-stack app development. But let's be real, they've also caused a lot of confusion.
Common Misconceptions
1. Client components are rendered only on the browser
Client components are rendered in both the server and browser.
๐๐จ๐ฐ ๐๐๐ฑ๐ญ.๐ฃ๐ฌ ๐ซ๐๐ง๐๐๐ซ๐ข๐ง๐ ๐ฐ๐จ๐ซ๐ค๐ฌ
- Server generates initial HTML, including Server Components and the initial state of Client Components.
- The initial HTML is sent to the browser with the JS files of Client components.
- The initial HTML is displayed on the browser.
- Components marked with 'use client' become interactive.
๐๐ก๐ฒ ๐ญ๐ก๐ ๐๐จ๐ฎ๐๐ฅ๐ ๐ซ๐๐ง๐๐๐ซ๐ข๐ง๐ ?
If components are only rendered in the browser, it can lead to poor user experience. Users might see a blank page at first and have to wait for JavaScript to run before seeing any content.
This is more likely to happen on slow networks and old devices. It also reduces the website's visibility in search engines.
Next.js solves this by pre-rendering client components on the server. This shows content immediately, improving user experience and SEO.
2. Server components can't be nested inside Client components
You can't put Server Components directly inside Client Components. Doing so turns the Server Component into a Client Component, losing its server-side benefits.
But you can pass Server Components as props to Client Components. This keeps their server-rendered nature.
A common pattern is to pass them as the children prop, as shown in the code example below.
This allows you to handle data fetching and heavy tasks on the server, making your app faster while still having interactive client components.
3. Every interactive Component needs to be a client component
You don't need use client
in every component that needs to be rendered on the client.
When you import a Server component inside a Client Component, the server component is rendered as a client component.
Also, all the nested components of that server component, become client components, including their utilities and functions.
Therefore, you only need to make the top-level parent component a "Client Component", then all the nested components are treated as a client component.
In this example, we have a client component 'Home'. The footer and Header are server components. Since they are nested from 'Home', they are treated as client components.
๐จ Why do you need to know this?
You might accidentally poison the frontend with a code that's meant to be used only on the backend.
For instance, if you imported a server component that has a getData
function into a client component, that getData
function would now be exposed to the frontend.
const API_SECRET = process.env.API_SECRET;
function fetchData() {
return fetch(`https://api.example.com/data?key=${API_SECRET}`);
}
This function fetches data, it uses an API_SECRET
. If this is imported in frontend, it may leak sensitive information.
This won't work on the Client because Next.js prevents accessing private variables (from the Client).
Note:** Private variables mean variables that don't start with NEXT_PUBLIC
.
Luckily, You could prevent this from happening by using server-only
, it will throw an error at build time if you try to share a backend code in the frontend.
'server-only'
const API_SECRET = process.env.API_SECRET;
function fetchData() {
return fetch(`https://api.example.com/data?key=${API_SECRET}`);
}
Key Takeaways
Client Components render on both the server and the client.
Server Components can be nested inside Client Components, as a prop.
Not all interactive components need
use client
, only top-level parent.Beware of exposing server-side code in client components.
Best Practices
Understand deeply RSC (React Server Components) before production use.
Use
server-only
to prevent code exposure.Structure apps for optimal performance and clear server/client separation.
Subscribe to my newsletter
Read articles from Hamza Mellahi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Hamza Mellahi
Hamza Mellahi
I am Hamza, a front-end developer based in Morocco ๐ฒ๐ฆ. I specialize in crafting engaging interfaces and optimizing web performance.