iOS app on Macs with Apple silicon
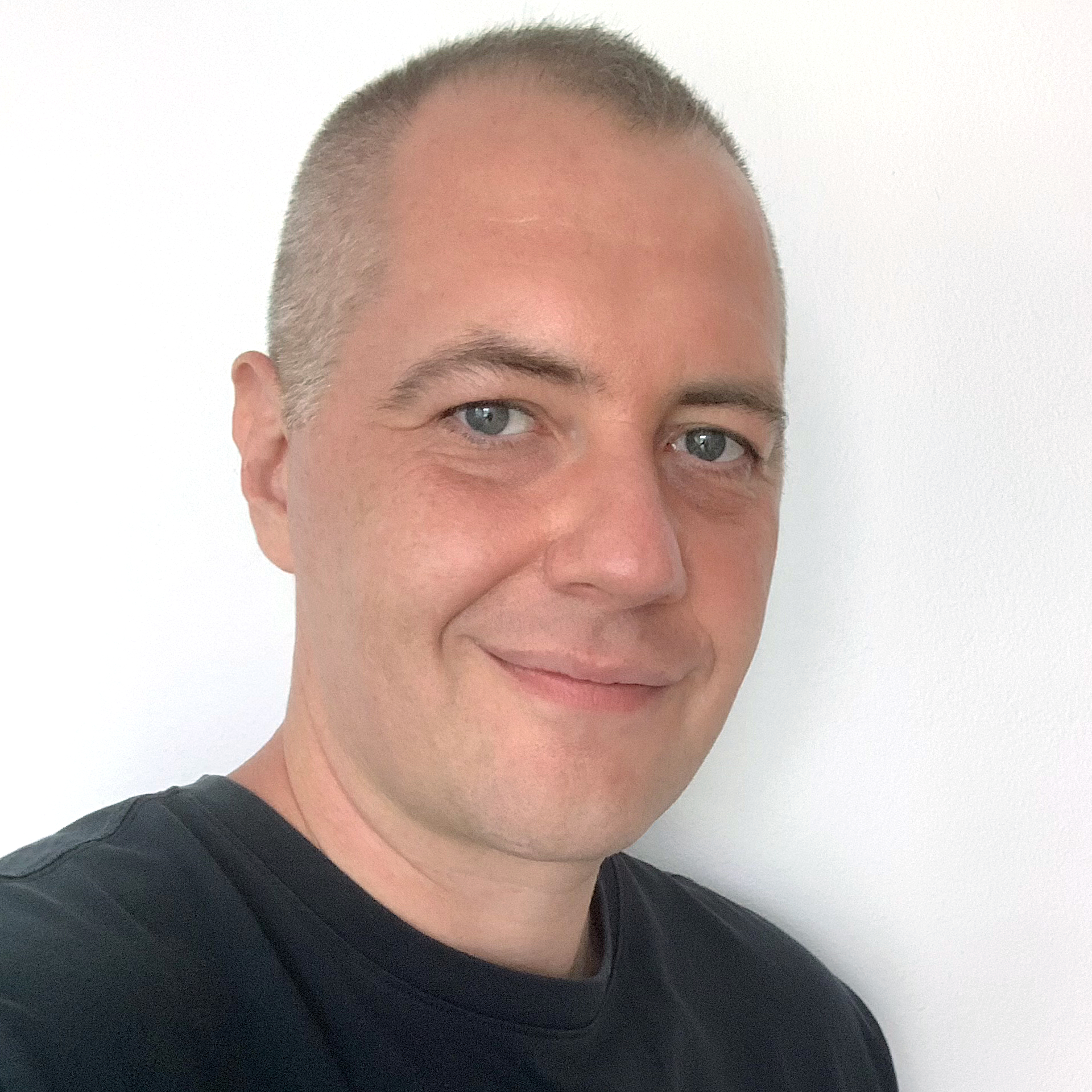
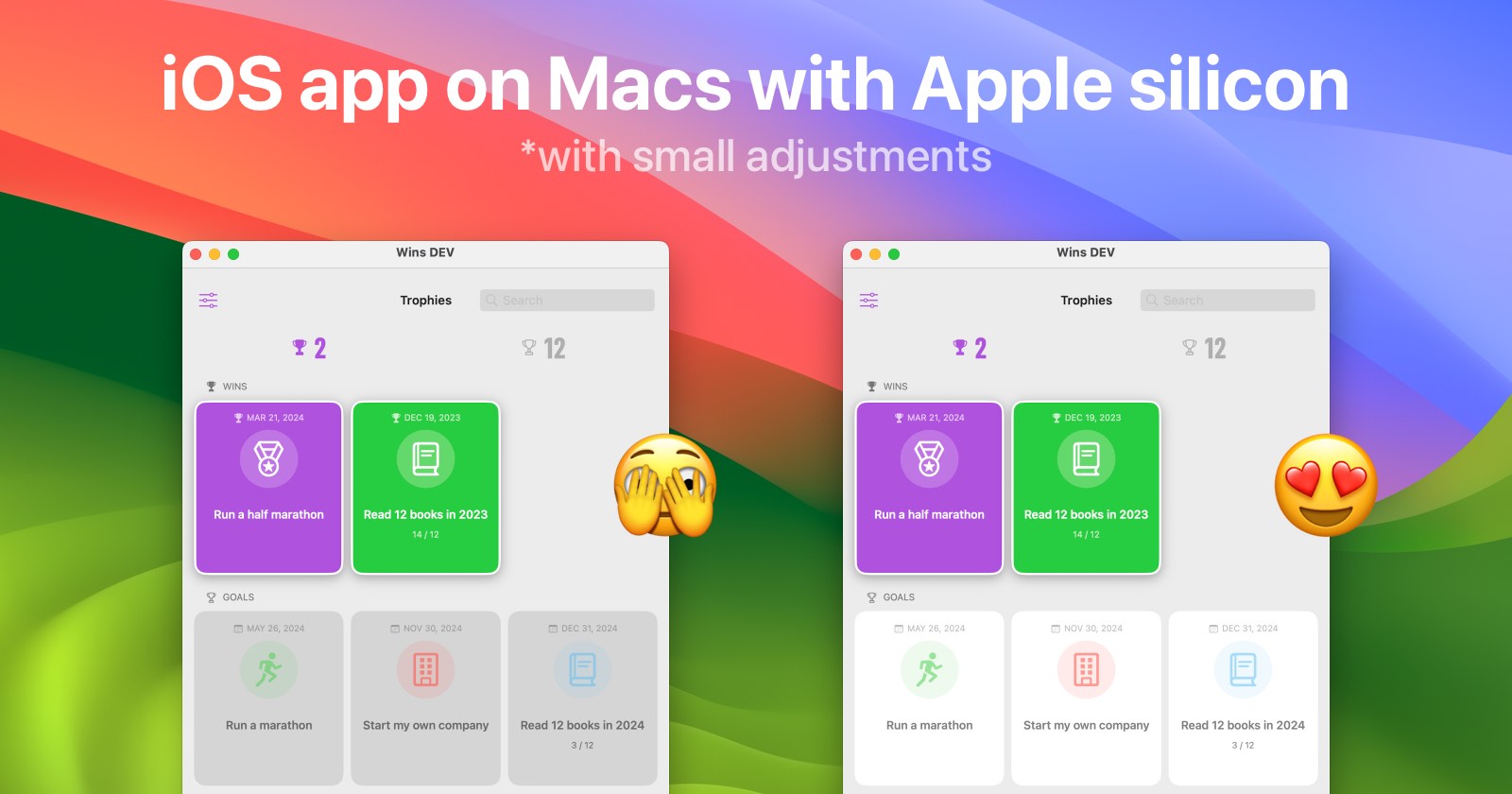
Before I make a native macOS version of my app Wins, I decided to implement a temporary solution: making the iPad version of my app available on Macs with Apple silicon. At least in my case, this turned out to be easier than expected.
Making the app available on Macs is a simple process. The first step is to add the correct destination for the app target in Xcode:
Select the app target (in my case, Wins).
Go to General tab and the Supported Destinations section.
Click the plus button.
Choose: Mac / Designed for iPad (or "Designed for iPhone" if the app is only for iPhone).
Now when I select My Mac (Designed for iPad) as the Run Destination and run the app, I can see how my iPad app looks on a Mac.
Mac and iPad apps are not the same
Let’c clarify something - an iPad app on a Mac doesn’t look exactly like it does on an iPad. It looks very similar, but there are some differences:
UI components are a bit different on macOS (Search, pickers, etc.)
animations are different (openine and closing sheets, for example)
colors (list backgrounds, for example)
While most colors look the same, I am using Color(.tertiarySystemBackground)
as the background color for some UI elements in my app. Why? Because I needed a white background in Light Mode and gray background in Dark Mode and thanks to system colors it’s easy to achieve without defining custom colors.
But… surprise, surprise - tertiarySystemBackground
on macOS is not white 😅
To fix this, I needed to conditionaly change the background color to Color(.secondarySystemGroupedBackground)
, which on macOS is white in Light Mode and gray in Dark Mode - exactly what I need.
But I’ve encountered a small problem…
How to conditionally change the UI on macOS
My first reaction was to use Swift’s compiler directive #if
, like this:
#if os(macOS)
.background(Color(.secondarySystemGroupedBackground))
#else
.background(Color(.tertiarySystemBackground))
#endif
But… since this condition runs at compile time and the iPad and Mac use the same build in this case, it doesn’t solve the problem.
Another idea I had was to use userInterfaceIdiom
:
var backgroundColor: Color {
if UIDevice.current.userInterfaceIdiom == .mac {
Color(.secondarySystemGroupedBackground)
} else {
Color(.tertiarySystemBackground)
}
}
// And later use backgroundColor in the modifier like this:
.background(backgroundColor)
But again, this doesn’t work. When an iPad app runs on a Mac, userInterfaceIdiom
returns .pad
, which means that backgroundColor
would always be set to tertiarySystemBackground
, regardless of whether the app is running on an iPad or a Mac.
Fortunately, there is another option: we can use the ProcessInfo class to handle this. Here’s how:
var backgroundColor: Color {
if ProcessInfo.processInfo.isiOSAppOnMac {
Color(.secondarySystemGroupedBackground)
} else {
Color(.tertiarySystemBackground)
}
}
// And later use backgroundColor in the modifier like this:
.background(backgroundColor)
And now, colors in my app look the same as I wanted on both iPad and Mac 😍
App Store Connect
The last step was to set the availability on App Store Connect. I needed to check “Make this app available” on the Pricing and Availability page, under the section iPhone and iPad Apps on Apple Silicon Macs.
After I checked the “Make this app available”, I also needed to click the Verify Compatibility button. The prompt says: “(…) If you've tested your app on Apple silicon Mac, and it functions as intended, verify it below.”
So, it’s up to the developer to test whether the app works as intended. If it does, we simply need to confirm that we have verified compatibility, which removes the “Not verified for macOS” label on the App Store that you may see on some apps.
I set my app’s availability after my new build with the color corrections was approved, and the new version of my app was released and… Voilà! My iOS and iPadOS app now works on Macs with Apple silicon and looks as intended. Next step: native macOS app! 😀
Thank you for reading!
If you want to support my work, please like, comment or share the article.
And most importantly...
📱Check out my apps on the App Store:
https://apps.apple.com/developer/next-planet/id1495155532
Subscribe to my newsletter
Read articles from Kris Slazinski directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
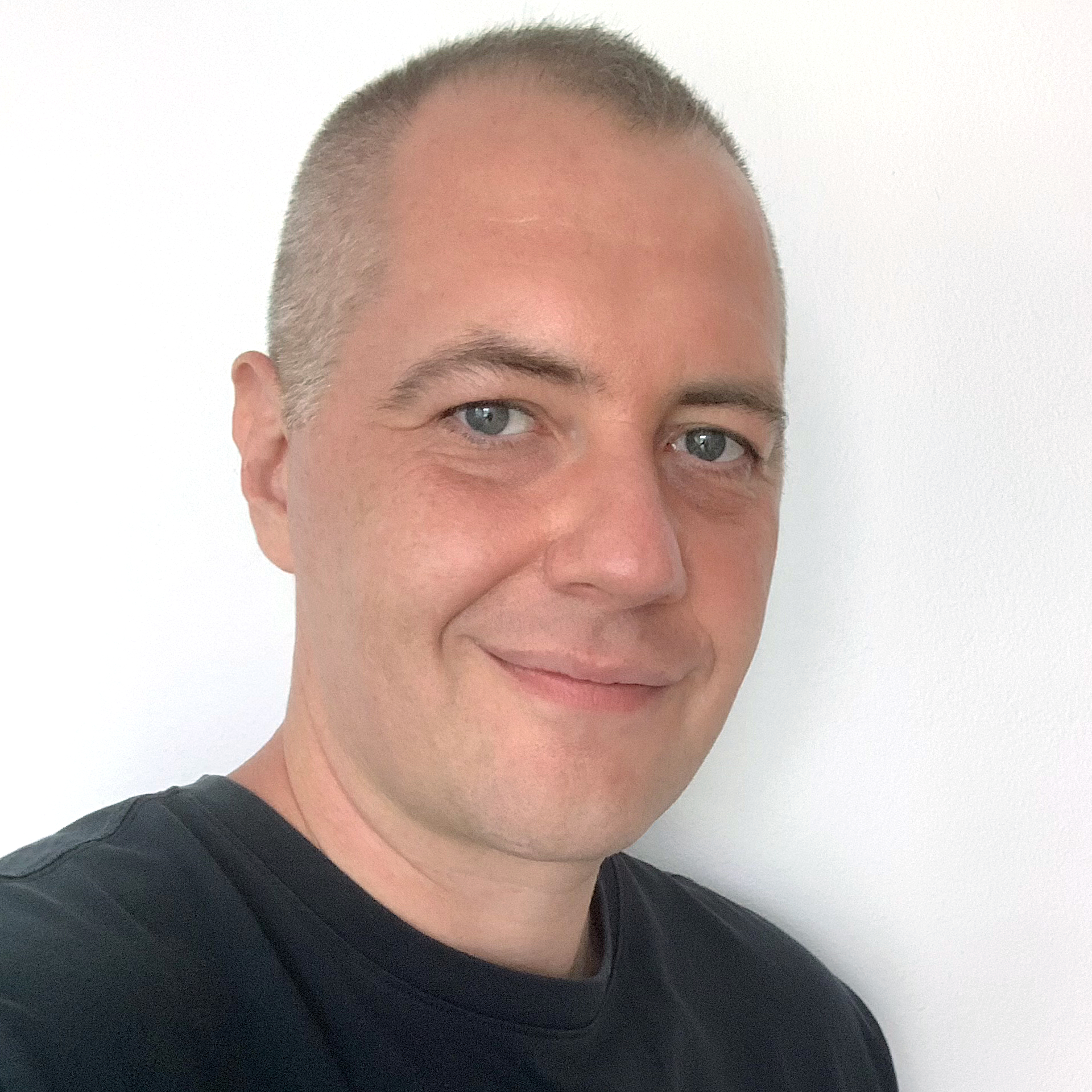
Kris Slazinski
Kris Slazinski
👨🏻💻 UX Designer, self-employed at NEXT PLANET - small design studio. 👨🏻💻 Indie iOS developer 📱 Creator of: Moons, Numi, Skoro, Wins and Emo. 🎸Guitarist at ZERO and Quadroom 🎨 MA in art 🌱 Vegan