How to Develop a Basic Discord Bot in Python

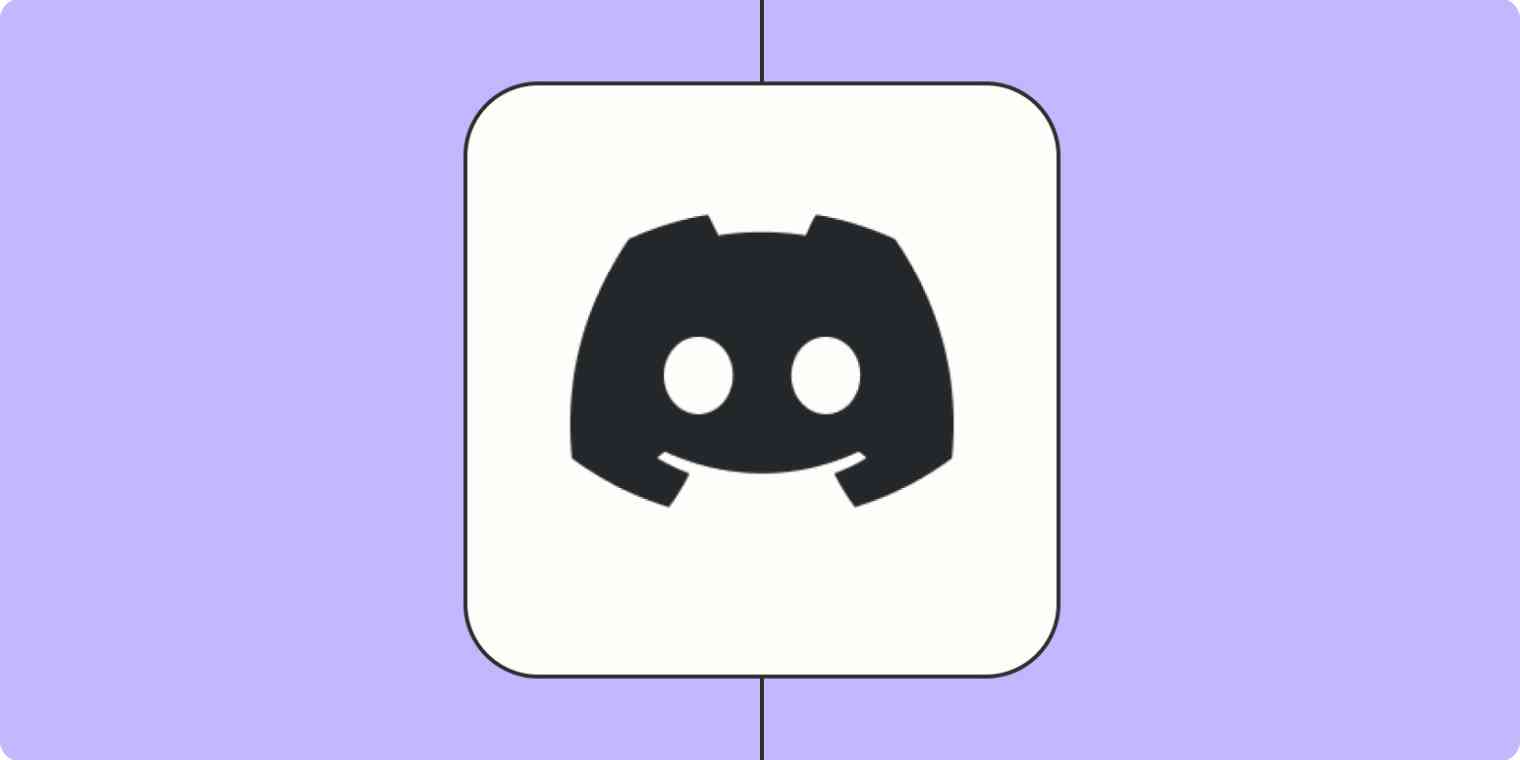
Install
discord.py
package
Begin with installing the discord.py
package, it is required for the bot as it serves as the core of the bot. It provides the essential tools and functionalities needed to interact with the Discord platform, enabling the bot to send messages, respond to commands, and manage server activities.
Windows:
pip install discord
Linux/MacOS:
pip3 install discord
Great, now that we have the core of the bot installed, we can start coding.
Coding the bot
Let’s start coding the actual functionality of the bot. Create a new file named main.py
in your chosen directory and open it in your preferred text editor.
Import the package we downloaded earlier:
import discord
from discord.ext import commands
bot = commands.Bot(command_prefix=commands.when_mentioned_or('!'), intents=discord.Intents.all())
Great, our command prefix will be !
. You can change this to whatever you want. You might have noticed that I added when_mentioned_or
. This means the bot will listen to commands with !
and also when mentioned. For example, both !command
and @bot command
will work. Now, let's add our first command and make the bot actually work.
@bot.event
async def on_ready():
print('Succesfully logged in!')
@bot.command()
async def hello(ctx):
await ctx.channel.send('World!')
bot.run('BOTTOKEN') # Replace with your own
Great! Our bot should be working now! Before I explain what it does, make sure to replace BOTTOKEN
with your own token from the developer panel.
Alright, async def on_ready():
runs when our bot starts. It will print "Successfully logged in!" when it does. bot.run('BOTTOKEN')
starts the bot and changes its status from offline to online. Without this, the bot can't do anything.
Now for the fun part, async def hello(ctx):
. This sets up the command. You can change "hello" to any word you want. The same goes for "World!". await ctx.channel.send('World!')
will send "World!" in the channel where you used the command.
I hope this guide has helped you understand how to create a basic Discord bot in Python. With the discord.py
package, you can add many features to improve your Discord server. Keep experimenting and adding new commands to make your bot even better. Happy coding!
Subscribe to my newsletter
Read articles from bytes directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
